Managing Temporary Directories in JUnit 5 Tests
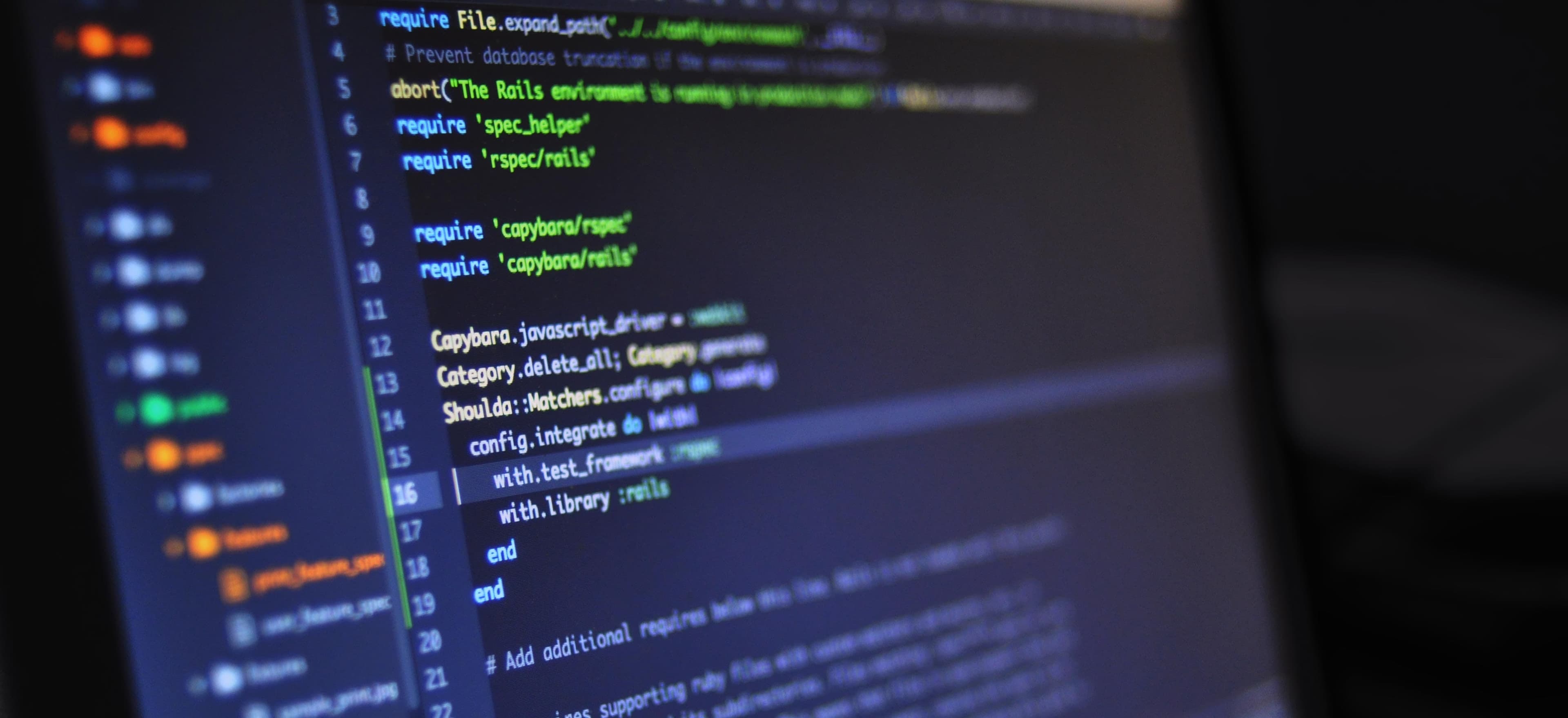
- Published on
Managing Temporary Directories in JUnit 5 Tests
When it comes to writing tests in Java, especially those that deal with file I/O, managing temporary directories effectively is crucial. This ensures that tests run in isolation, preventing data from corrupting the filesystem or carrying over between tests. In this blog post, we will explore how to manage temporary directories in JUnit 5 tests effectively.
Why Use Temporary Directories?
Engaging with file systems can lead to several issues, such as:
- Data Contamination: Tests that write to the same file can overwrite data and lead to unpredictable test results.
- Resource Cleanup: Leaving behind temporary files leads to resource leakage, which can cause problems in longer-running builds or tests.
- Isolation: Keeping tests independent prevents changes in one test from affecting others.
Using temporary directories allows your tests to be self-contained. Each test creates its own directory, using it to read/write, ensuring that tests are isolated from one another.
Setting Up JUnit 5
Before we dive into managing temporary directories, make sure you have the necessary dependencies for JUnit 5 in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.9.1</version>
<scope>test</scope>
</dependency>
Make sure to adjust the version according to the latest stable release.
Creating Temporary Directories
JUnit 5 provides a built-in mechanism to handle temporary files and directories using the @TempDir
annotation. Let's see how to implement this in our tests.
Creating a Temporary Directory
Here's how to create a temporary directory for your tests using the @TempDir
annotation:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.io.TempDir;
import java.io.File;
import java.io.IOException;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class TempDirExampleTest {
@TempDir
Path tempDir;
@Test
void testTemporaryDirectory() throws IOException {
// Create a new file in the temporary directory
File tempFile = tempDir.resolve("tempFile.txt").toFile();
boolean fileCreated = tempFile.createNewFile();
// Assert that the file was created
assertTrue(fileCreated, "Temporary file should be created");
// Ensure that the tempDir has created the file
assertTrue(tempFile.exists(), "The temporary file should exist");
// The temporary directory will be deleted after this test
}
}
Why This Code Works
- @TempDir: This annotation automatically manages a temporary directory. It is created before the test method and deleted after.
- Path tempDir: The
Path
type is part of the Java NIO package which provides a convenient way to interact with the filesystem. - File Creation: Using
tempDir.resolve("tempFile.txt").toFile()
creates a newFile
object in the temporary directory. - Assertions: We check that the file was created using assertions, ensuring that our test behaves as expected.
Nested Temporary Directories
Sometimes, you may need a more complex setup, such as nested temporary directories. Here's how to handle that:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.io.TempDir;
import java.nio.file.Files;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class NestedTempDirTest {
@TempDir
Path outerTempDir;
@Test
void testNestedTempDirectory() throws Exception {
// Create a nested temporary directory
Path innerTempDir = outerTempDir.resolve("innerDir");
Files.createDirectories(innerTempDir);
// Create a file in the nested directory
Path nestedFile = innerTempDir.resolve("nestedFile.txt");
Files.createFile(nestedFile);
// Assert that the nested file exists
assertTrue(Files.exists(nestedFile), "Nested file should exist");
// The entire outerTempDir with its contents will be deleted automatically
}
}
Understanding the Nested Structure
- Nested Directories: By resolving paths, you can create a logical hierarchy of directories.
- Files.createDirectories: This convenient method creates all nonexistent parent directories if necessary.
- File Existence: The test checks that the file exists within the nested structure.
Temporary Files Management
Besides managing directories, you might often need to create temporary files. Let's extend our test cases to include temporary file creation:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.io.TempDir;
import java.nio.file.Files;
import java.nio.file.Path;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class TempFileExampleTest {
@TempDir
Path tempDir;
@Test
void testTemporaryFile() throws Exception {
// Create a temporary file
Path tempFile = tempDir.resolve("tempFile.txt");
String content = "Hello, World!";
Files.write(tempFile, content.getBytes());
// Read back the content from the temporary file
String readContent = Files.readString(tempFile);
// Assert that the content is as expected
assertEquals(content, readContent, "The content should match the initial value");
}
}
Explanation of Temporary File Management
- Files.write: This method allows you to write data to a file atomically, creating the file if it doesn’t exist or replacing it if it does.
- Files.readString: Conveniently reads all bytes from a file and converts them into a string. Easy to use for tests.
Cleaning Up Resources
While JUnit manages the temporary directories, it's still good practice to ensure that you explicitly manage other kinds of resources, such as network connections or database sessions. Wrapping setup and teardown logic using methods annotated with @BeforeEach
and @AfterEach
can prepare your tests adequately.
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
public class ResourceManagementTest {
// Some resource you need to manage
DatabaseConnection connection;
@BeforeEach
void setup() {
// Initialize the connection
connection = new DatabaseConnection();
}
@AfterEach
void cleanup() {
// Close the connection
connection.close();
}
}
Resource Management in JUnit
- Lifecycle Annotations: Using
@BeforeEach
and@AfterEach
, we maintain control over the setup and teardown processes, ensuring that resources are allocated and released correctly.
Final Thoughts
In this blog post, we've explored how to manage temporary directories in JUnit 5 tests efficiently. The @TempDir
annotation simplifies creating and managing temporary files and directories. It enhances test reliability by ensuring tests remain isolated and self-contained. By integrating resource management strategies, you guarantee that your tests are robust and maintainable.
For additional resources on file I/O in Java, you can refer to Java's NIO package and JUnit 5 User Guide for further reading.
By following the best practices outlined here, you can ensure that your tests run smoothly and reliably, greatly improving the quality of your Java applications. Happy testing!
Checkout our other articles