Overcoming Java EE 6 Galleria Performance Issues
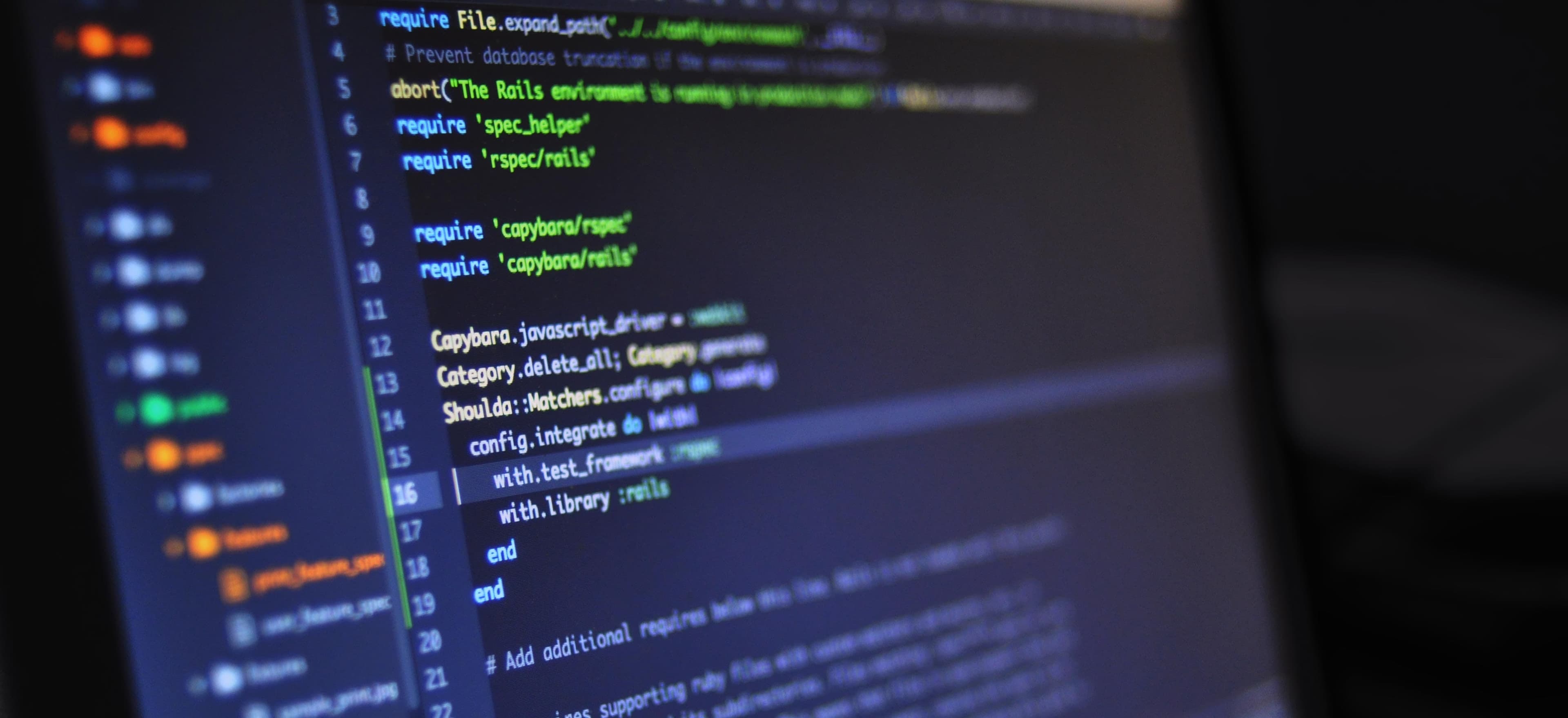
- Published on
Overcoming Java EE 6 Galleria Performance Issues
Java EE (Enterprise Edition) has long been a cornerstone for building robust, scalable, and efficient web applications. However, as with any technology, performance issues can arise—especially in complex applications like the Java EE 6 Galleria. In this blog post, we will dive into common performance bottlenecks associated with Java EE 6 Galleria applications and explore strategies to overcome them.
Understanding Java EE 6 Galleria
The Java EE 6 Galleria is a sample application designed to showcase the capabilities of Java EE technologies. It demonstrates how to use various components like Servlets, JSP, and EJBs in a cohesive manner. Though it serves as an excellent educational tool, the Galleria can encounter performance issues under certain conditions, such as high traffic loads or inefficient resource utilization.
Identifying Performance Issues
Before we can tackle performance problems, we first need to identify them. Common symptoms include:
- Slow Response Times: Users experience delays when navigating through the application.
- High Memory Usage: The application consumes excessive memory, leading to potential OutOfMemoryError exceptions.
- Increased CPU Usage: The server runs at high CPU levels, impacting the overall system performance.
- Database Bottlenecks: Slow database operations can severely affect application responsiveness.
To diagnose these issues, developers can utilize profiling tools like VisualVM or JProfiler to analyze the application performance and identify resource-consuming operations.
Strategies to Overcome Performance Issues
With a better understanding of the Galleria and its potential pitfalls, let’s explore targeted strategies to enhance performance.
1. Optimize Database Interactions
Database interactions are often the primary bottleneck in enterprise applications. Here are several strategies to streamline database access:
- Connection Pooling: Utilizing connection pools (e.g., through a Java EE resource adapter) reduces the overhead of establishing new connections for each request.
import javax.annotation.Resource;
import javax.sql.DataSource;
@Resource(lookup = "java:jboss/datasources/MainDS")
private DataSource dataSource;
public Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
Why: This optimizes resource utilization, as connection pooling minimizes latency in database connections.
- Batch Processing: When performing bulk insertions or updates, batching can considerably decrease the number of database calls.
public void batchInsert(List<Item> items) {
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement("INSERT INTO orders VALUES (?, ?)")) {
for (Item item : items) {
pstmt.setInt(1, item.getId());
pstmt.setString(2, item.getName());
pstmt.addBatch();
}
pstmt.executeBatch(); // Executes the batch of commands.
}
}
Why: This approach reduces the number of round trips to the database, enhancing performance for bulk operations.
2. Implement Caching
Caching frequently accessed data can drastically cut down on database queries and speed up application response times.
- Use Java EE Caching: Java EE provides mechanisms like JCache to cache objects in memory.
import javax.cache.Cache;
import javax.cache.CacheManager;
import javax.cache.Caching;
CacheManager cacheManager = Caching.getCachingProvider().getCacheManager();
Cache<Integer, Item> cache = cacheManager.getCache("itemCache", Integer.class, Item.class);
// Check if the item is in the cache before querying the database
if (!cache.containsKey(itemId)) {
Item item = database.getItem(itemId);
cache.put(itemId, item);
}
Why: This reduces the need to fetch data from the database frequently, speeding up access for repeated requests.
3. Optimize EJB Usage
Enterprise JavaBeans (EJB) can be a performance concern when not used judiciously. Here are key points to consider:
-
Stateless vs. Stateful: Prefer stateless EJBs for most operations. Stateful beans maintain state between invocations, consuming more resources.
-
Reduce the Number of EJB Calls: Try to minimize remote calls. When possible, use local interfaces to reduce the overhead associated with remote method invocations.
4. Asynchronous Processing
For operations that can be performed independently of the main request-response cycle, consider using asynchronous processing. This allows the server to free up resources for handling other requests.
import javax.ejb.Asynchronous;
import javax.ejb.Stateless;
@Stateless
public class ItemProcessor {
@Asynchronous
public void processItem(Item item) {
// Perform operations that do not block user requests.
}
}
Why: Asynchronous processing helps to improve application responsiveness and scale under heavy loads.
5. Utilize Profiling and Monitoring Tools
Monitoring and profiling are critical for maintaining and optimizing performance. Tools such as JVisualVM, YourKit, or APM solutions like New Relic can provide insights into application behavior, allowing for data-driven decisions regarding optimization.
- Real-Time Monitoring: Track metrics such as memory usage, thread count, and request latency.
- Identify Hot Spots: Use profiling tools to determine which methods are consuming the most CPU or memory.
6. Spring Integration
While Java EE has robust capabilities, some developers opt to integrate Spring for simplified configurations and a range of powerful features like Spring Boot for application-specific optimizations. For optimal performance, consider leveraging Spring's component scanning and dependency injection capabilities to avoid some of the performance issues found in Java EE.
For further insights on enhancing Java EE performance, check out Java EE 6 Overview and Improving Java EE Performance.
My Closing Thoughts on the Matter
Overcoming performance issues in Java EE 6 Galleria applications involves a multifaceted approach. By optimizing database interactions, implementing caching, fine-tuning EJB usage, leveraging asynchronous processing, and utilizing monitoring tools, developers can significantly enhance the efficiency and responsiveness of their applications.
Always remember that tuning performance is an iterative process. Regular profiling and monitoring will ensure that your application continues to meet the growing demands of users and maintains its edge in performance.
Implementing these strategies will not only improve user experience but also reduce operational costs associated with resource consumption. The Java EE 6 Galleria can indeed shine as a stellar example of efficient web applications when given the right attention to performance details.