Mastering Debugging: Troubleshooting Finalizer Issues
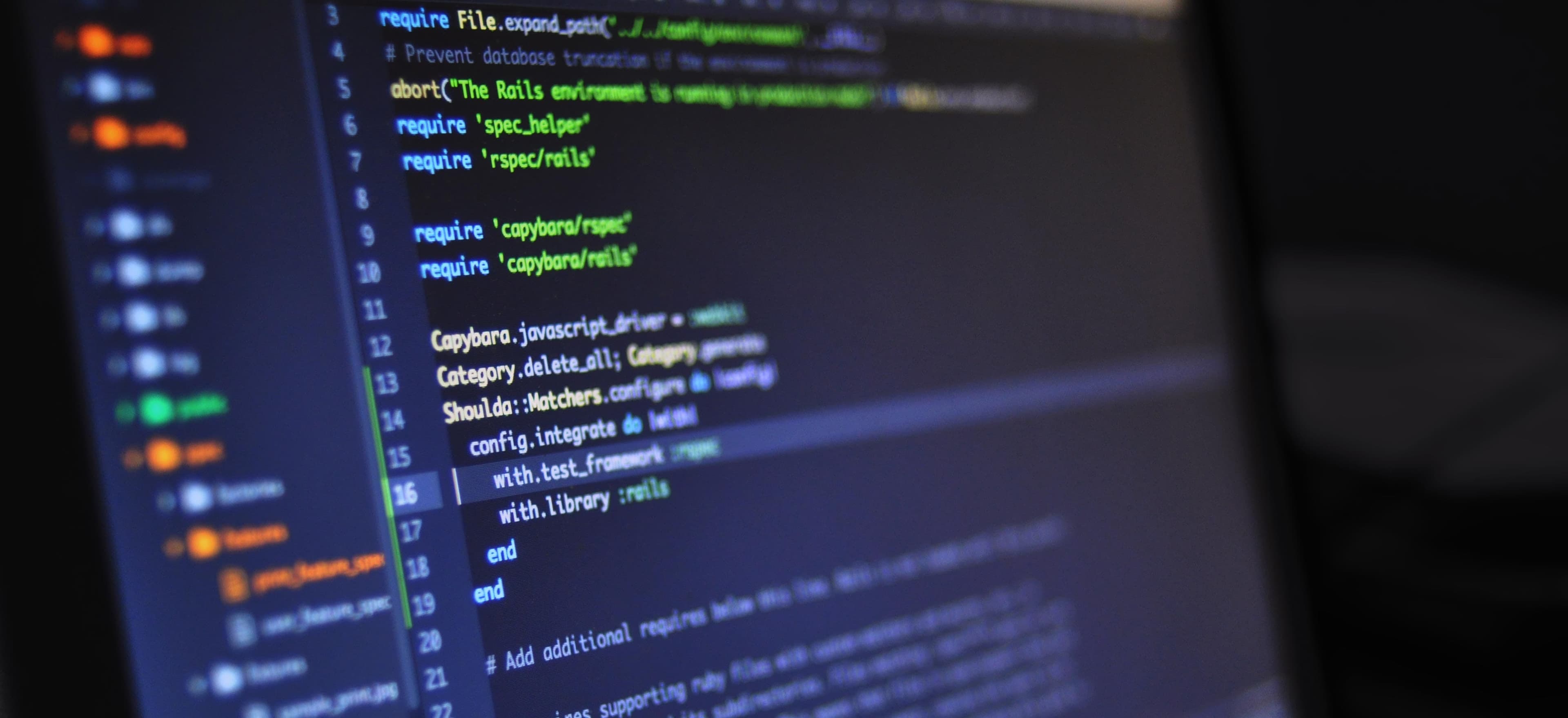
- Published on
Mastering Debugging: Troubleshooting Finalizer Issues in Java
Debugging is an essential skill for any developer, and mastering it can significantly enhance your productivity. In Java, finalizers can present unique challenges that may hinder your application’s performance. This blog post aims to delve into these issues, offering insights and techniques for effective troubleshooting.
Understanding Finalizers in Java
Before we can troubleshoot finalizer issues, we must first understand what finalizers are. In Java, every object is eligible for garbage collection once there are no strong references to it. However, if a class defines a finalizer using the finalize()
method, this method will be invoked by the garbage collector before the memory occupied by the object is reclaimed.
Example of a Finalizer
public class FinalizerExample {
@Override
protected void finalize() throws Throwable {
try {
// Cleanup code before garbage collection
System.out.println("Finalizer is being called for: " + this);
} finally {
super.finalize(); // Always call the superclass finalize
}
}
}
In this example, the finalize()
method prints a message when the object is being finalized. It's paramount to call super.finalize()
to ensure that the superclass’s finalizer is also executed.
Why Finalizers Can Be Problematic
While finalizers seem useful, they introduce several issues:
-
Unpredictable Timing: Finalizer execution is unpredictable. The garbage collector does not guarantee when (or even if) the finalizer will run. This can lead to resource leaks.
-
Performance Overhead: Objects with finalizers can incur a performance penalty because the garbage collector must keep track of them. This delay can lead to slower application performance.
-
Debugging Difficulty: Issues related to finalizers can be hard to debug. If an object’s finalize method throws an exception, the garbage collector will ignore the exception and move on, potentially leaving behind unreachable resources.
The combination of these issues makes finalizers often discouraging in practice, prompting many developers to use alternative approaches such as try-with-resources or explicit resource management.
Alternatives to Finalizers
Given the aforementioned downsides of finalizers, consider using the following alternatives:
1. Try-With-Resources
This construct is a reliable way to manage resources that implement the AutoCloseable
interface.
public class ResourceExample implements AutoCloseable {
public ResourceExample() {
// Resource Acquisition
System.out.println("Resource Acquired");
}
@Override
public void close() {
// Resource Cleanup
System.out.println("Resource Released");
}
public void performAction() {
System.out.println("Action Performed");
}
}
// Using try-with-resources
try (ResourceExample resource = new ResourceExample()) {
resource.performAction();
}
In the above example, the ResourceExample
class implements AutoCloseable
, allowing automatic resource release when the try block completes.
2. Explicit Resource Management
For more complex scenarios that don’t fit neatly into the try-with-resources
paradigm, consider explicitly managing your resources through your own method calls.
public class ResourceHandler {
private ResourceExample resource;
public void allocateResource() {
resource = new ResourceExample();
}
public void releaseResource() {
if (resource != null) {
resource.close();
resource = null; // Avoid memory leaks
}
}
}
Here, resource management is performed explicitly with careful attention to resource cleanup.
Debugging Finalizer Issues
If you decide to use finalizers—or are tasked with troubleshooting existing code that does—it's essential to employ effective debugging strategies.
1. Logging Finalizer Execution
Add logging to your finalizer to track execution timing and the state of your objects. This is invaluable for seeing what happens when garbage collection attempts to finalize objects.
@Override
protected void finalize() throws Throwable {
try {
log("Finalizing object: " + this);
} finally {
super.finalize();
}
}
private void log(String message) {
System.out.println(message);
}
2. Monitor Object References
Use profiling tools such as VisualVM or Eclipse Memory Analyzer to monitor object references and identify memory leaks. These tools help to visualize how objects are being held in the heap.
3. Check for finalize
Exceptions
Be vigilant about exceptions thrown within the finalize()
method. While these exceptions won't normally crash your application, they can prevent proper resource management.
@Override
protected void finalize() throws Throwable {
try {
// Perform cleanup
} catch (Exception e) {
log("Exception in finalize: " + e);
} finally {
super.finalize();
}
}
Bringing It All Together
While finalizers in Java might seem useful at first glance, they carry substantial downsides that can complicate debugging and degrade performance. Instead, consider alternatives like try-with-resources or explicit resource management to handle resource cleanup efficiently.
If you find yourself debugging finalizer issues, employ logging, monitoring tools, and exception handling to ease your task. With these practices, you can ensure your Java applications are both efficient and clean, leading to smoother development processes.
Further Reading
For a deeper dive into effective resource management in Java, check out:
- Effective Java by Joshua Bloch
- Java Concurrency in Practice
- Java Performance: The Definitive Guide
By mastering debugging and understanding finalizer issues thoroughly, you prepare yourself to create robust, high-performing Java applications. Happy coding!
Checkout our other articles