Mastering Error Handling in Storm Trident Topologies
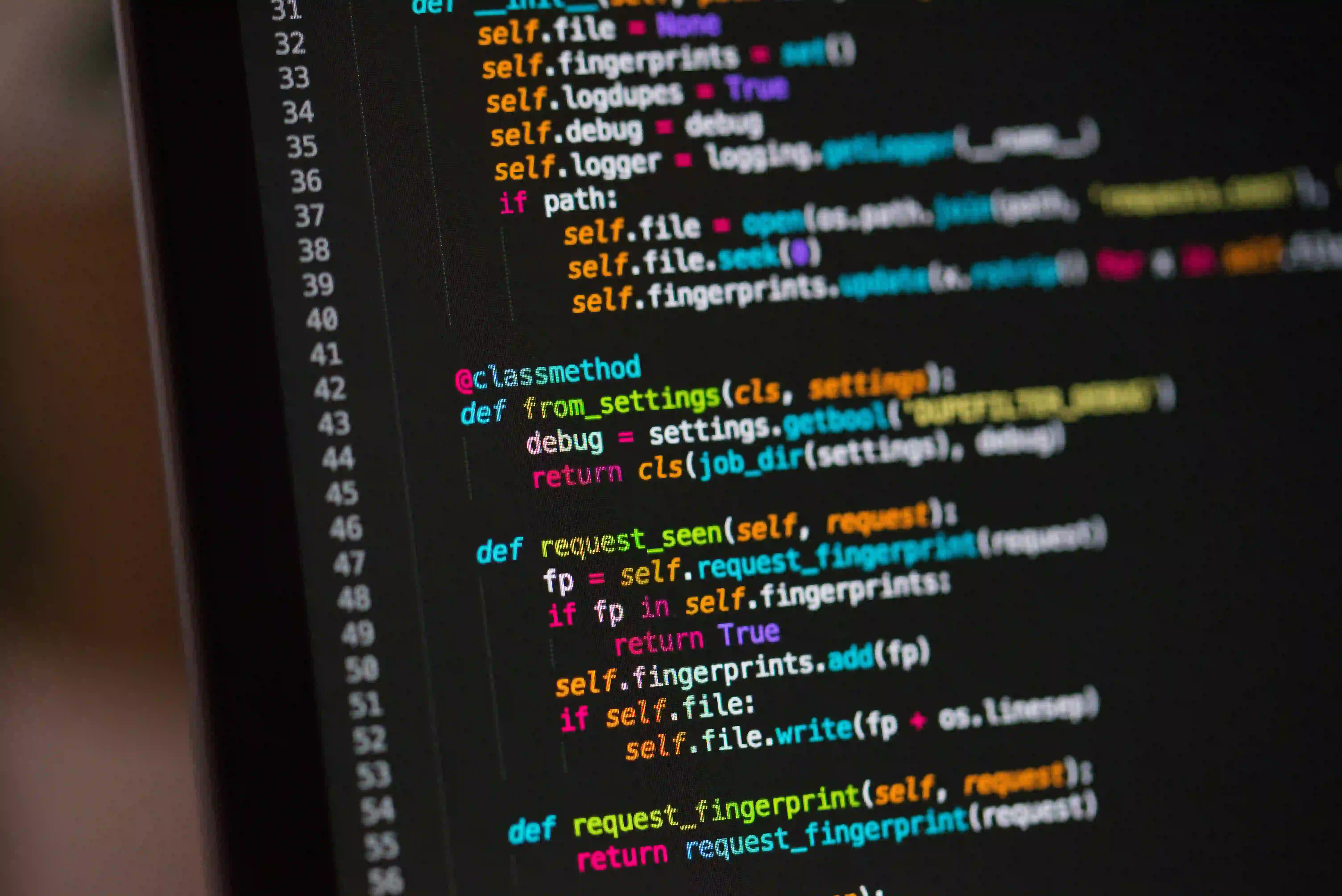
Mastering Error Handling in Storm Trident Topologies
In the realm of big data and stream processing, Apache Storm offers a robust framework for real-time computation. Within this framework, Trident serves as an abstraction layer that simplifies stateful processing. However, even with such powerful tools, error handling is paramount to ensure the reliability and maintainability of applications. In this blog post, we will explore effective strategies for implementing error handling in Storm Trident topologies, providing you with actionable insights and code snippets that can be applied in real-world projects.
Understanding Apache Storm and Trident
Before diving into error handling, let's briefly revisit what Apache Storm and Trident offer.
Apache Storm is a distributed real-time computation system that processes unbounded streams of data. It allows for the processing of millions of messages per second with low latency. However, this comes with challenges in error management.
Trident is built on top of Storm and adds a layer that simplifies stateful stream processing. With structured data and built-in guarantees, such as exactly-once processing, Trident enhances the capabilities of traditional Storm applications.
For more detailed insights on Storm, check out the official Apache Storm documentation.
Why Error Handling is Critical
In the world of stream processing, errors are inevitable — from data format mismatches to system failures. Proper error handling ensures that:
- The system maintains uptime.
- Data integrity is preserved.
- Applications can recover gracefully from failures.
Common Types of Errors in Storm Trident
Understanding the kinds of errors you might encounter will allow you to create efficient error handling strategies. Below are common categories:
- Transient Errors: Issues that are temporary and typically resolve on their own (e.g., network timeouts).
- Fatal Errors: Unrecoverable errors that require the entire process to be halted (e.g., application crashes).
- Data Errors: Data quality issues like malformed records or missing fields.
Strategies for Error Handling
Here are some strategies you can implement to enhance error handling in your Storm Trident applications.
1. Using Try-Catch Blocks
Try-catch blocks are fundamental in Java for managing exceptions. In a Storm Trident topology, you can use them to encapsulate code that may throw an error.
Stream stream = tridentTopology
.newStream("source", new MySpout())
.each(new Fields("data"), new EachFunction() {
@Override
public void execute(TridentTuple tuple, TridentCollector collector) {
try {
String data = tuple.getString(0);
// Process data...
collector.emit(new Values(processedData));
} catch (Exception e) {
// Handle the error
System.err.println("Error processing tuple: " + e.getMessage());
// You might log this error or send it to a dead letter queue
}
}
});
Why: This allows you to gracefully handle exceptions and potentially log them or direct them to a monitoring system. It prevents the entire stream from stopping due to one faulty tuple.
2. Implementing Retry Mechanisms
Transient errors can often be resolved with retries. Here's how you can implement retries with exponential backoff.
public void processDataWithRetry(String data) {
int attempts = 0;
while (attempts < MAX_ATTEMPTS) {
try {
// Attempt to process the data.
processData(data);
return; // Exit if processing is successful.
} catch (TemporaryException e) {
attempts++;
try {
Thread.sleep((long) Math.pow(2, attempts) * 100); // Exponential backoff
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
// Log and handle failure after maximum attempts.
System.err.println("Failed to process after retries: " + data);
}
Why: This retry strategy helps mitigate issues caused by temporary glitches in the system or network. The exponential backoff adds a delay that can relieve system stress during high-load periods.
3. Dead Letter Queues (DLQs)
Implementing Dead Letter Queues is a best practice for handling messages that cannot be processed after several attempts. You can leverage systems like Apache Kafka or RabbitMQ for this.
public void processDataWithDLQ(String data) {
boolean isProcessed = false;
int attempts = 0;
while (attempts < MAX_ATTEMPTS) {
try {
// Attempt to process the data.
processData(data);
isProcessed = true;
break; // Processing success
} catch (Exception e) {
attempts++;
if (attempts >= MAX_ATTEMPTS) {
sendToDLQ(data); // Send to Dead Letter Queue
}
}
}
}
Why: DLQs enable you to handle problematic tuples by storing them for later analysis or reprocessing. This ensures that the rest of your system continues functionally, without allowing a single tuple to disrupt the overall flow.
4. Logging and Monitoring
Having a robust logging and monitoring system is essential. Utilize logging frameworks like SLF4J or Log4J for structured logging.
public void logError(Exception e, String message) {
logger.error("Error processing data: {}. Exception: {}", message, e.getMessage());
}
Why: Logging is critical for diagnosing issues in your topology. A well-structured log entry can drastically reduce the time it takes to identify and resolve errors.
5. Custom Error Handlers
For more complex error scenarios, implementing custom error handlers can give you control over how different types of errors are processed.
public class MyErrorHandler implements ErrorHandler {
@Override
public void handleError(TridentTuple tuple, Exception e) {
// Custom logic for handling the error
logError(e, tuple.toString());
// Take necessary recovery actions, such as sending to DLQ
}
}
// In your topology
tridentTopology.setErrorHandler(new MyErrorHandler());
Why: Custom error handlers provide a tailored way to manage errors that fit your specific business logic. They help ensure your response to errors aligns with organizational priorities.
To Wrap Things Up
Effective error handling is crucial for building robust Storm Trident applications. By employing strategies such as try-catch blocks, retries, Dead Letter Queues, logging, and custom error handlers, you can ensure that your system is resilient to various errors.
As you develop your own stream processing applications, remember to monitor exceptions actively and periodically review error handling strategies. This proactive measure will promote a reliable and efficient data flow in your system.
For further reading on Storm and its components, check out the Storm Trident section of the documentation.
By mastering error handling, you can maximize the performance of your Storm Trident topologies and ensure that they deliver actionable insights to your business in real-time. Happy coding!