Mastering Global Exception Handling in Java EE 8 MVC
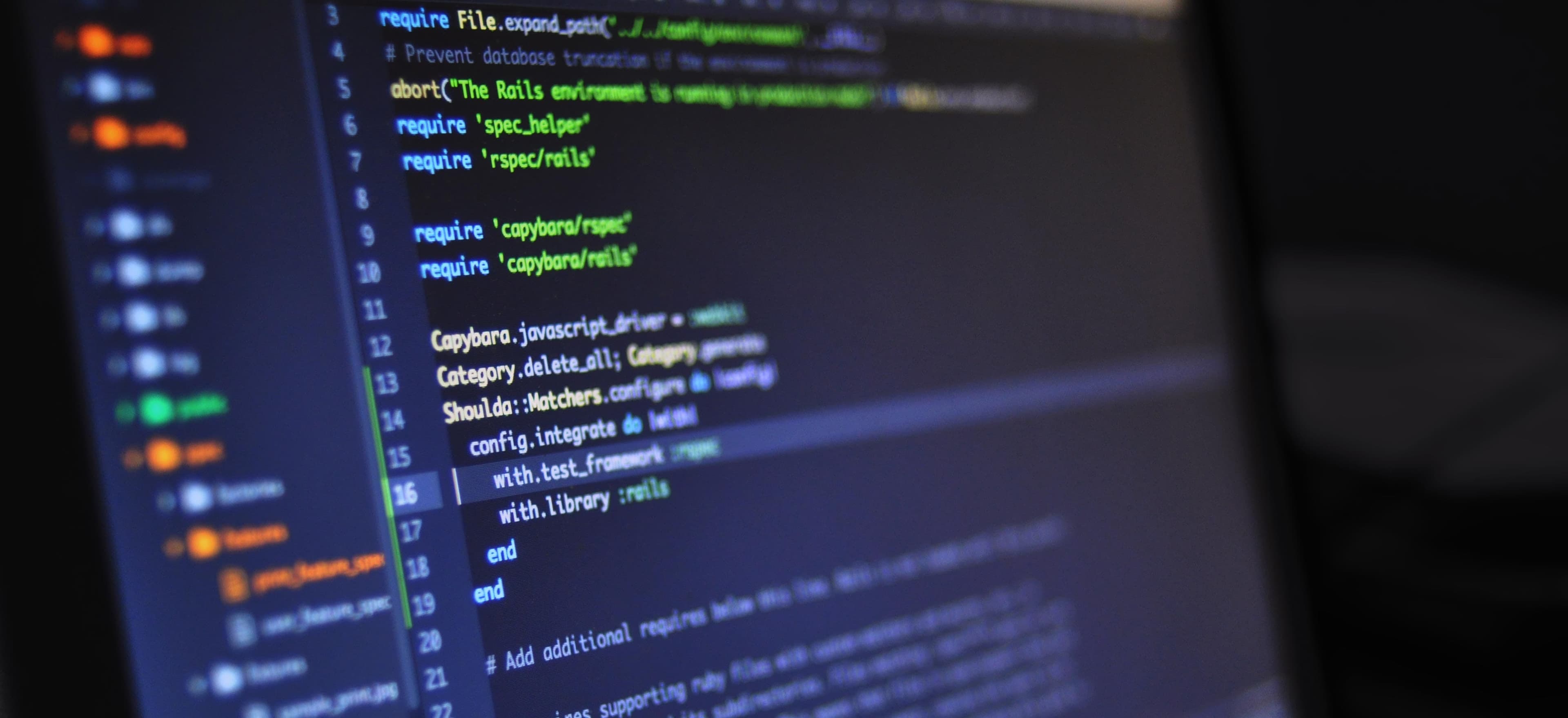
- Published on
Mastering Global Exception Handling in Java EE 8 MVC
Exception handling is a critical aspect of any application. For developers working with Java EE 8 MVC, effectively managing exceptions can greatly enhance the robustness and maintainability of web applications. In this blog post, we'll delve into global exception handling in Java EE 8 MVC, exploring the framework's mechanisms, best practices, and providing code snippets to illustrate these concepts.
Understanding Exception Handling
Exception handling is the process of responding to the occurrence of exceptions. Exceptions are unwanted or unexpected events that can occur during the execution of a program. In a web application, various types of exceptions may arise—from unexpected user input to system errors.
In Java EE, the default behavior when an exception occurs is to log it and display a generic error page to the user. This approach can be insufficient, as it often lacks clarity and provides no guidance on how to rectify the issue.
Additionally, proper exception handling also enhances the user experience by providing informative feedback rather than exposing internal server errors.
Why Global Exception Handling?
With Java EE 8 MVC, you can implement global exception handling to catch and manage exceptions across your application. Here are some primary reasons to consider global exception handling:
- Consistency: It enforces a uniform strategy for managing exceptions across different controllers.
- Clarity: It allows you to present users with a defined, user-friendly error message.
- Separation of Concerns: It helps maintain clean controllers by separating error handling from business logic.
- Centralized Logging: It enables centralized logging of errors, making it easier to maintain and troubleshoot application issues.
Setting up Global Exception Handling
Step 1: Creating Custom Exception Classes
First, let's create a custom exception to handle specific application errors. This adds clarity to the exception handling process.
package com.example.exceptions;
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
In this code snippet, we define a ResourceNotFoundException
which extends RuntimeException
. This can be thrown when a requested resource cannot be found.
Step 2: Implementing the Global Exception Handler
Next, we will create a global exception handler using the @ControllerAdvice
annotation:
package com.example.controllers;
import com.example.exceptions.ResourceNotFoundException;
import javax.ws.rs.core.Response;
import javax.ws.rs.ext.ExceptionMapper;
import javax.ws.rs.ext.Provider;
import javax.ws.rs.core.MediaType;
@Provider
public class GlobalExceptionHandler implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
String errorMessage = exception.getMessage();
if (exception instanceof ResourceNotFoundException) {
return Response.status(Response.Status.NOT_FOUND)
.entity(errorMessage)
.type(MediaType.APPLICATION_JSON)
.build();
}
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("An unexpected error occurred.")
.type(MediaType.APPLICATION_JSON)
.build();
}
}
Points to Note:
- We implement
ExceptionMapper
, allowing for handling of all exceptions. - This handler provides a consistent response for
ResourceNotFoundException
and defaults for any unhandled exceptions. - The API returns responses organized as JSON for better integration with frontend applications.
Step 3: Registering the Exception Handler
Now that we have the global exception handler, the next step is registering it with the application's context. This is usually achieved in the web.xml
configuration:
<context-param>
<param-name>javax.ws.rs.Application</param-name>
<param-value>com.example.config.ApplicationConfig</param-value>
</context-param>
And in your application class, register your handler:
package com.example.config;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class ApplicationConfig extends Application {
// Additional configuration can be provided here.
}
Where ApplicationConfig
is the class that registers your resource and exception handler.
Enhancing User Experience with Custom Error Pages
While JSON responses are great for APIs, it is essential to provide HTML error pages for web applications. Here’s how you can customize your error pages:
Step 4: Creating Custom Error Pages
Creating a custom error page is straightforward. You can create a JSP file or an HTML file such as error.html
in the resources directory.
<!DOCTYPE html>
<html>
<head>
<title>Error Page</title>
</head>
<body>
<h1>An error occurred</h1>
<p>We're sorry, but something went wrong on our end.</p>
</body>
</html>
Step 5: Modifying the Global Exception Handler for HTML Responses
You can modify the handler to return a full HTML response when necessary:
@Override
public Response toResponse(Throwable exception) {
if (exception instanceof ResourceNotFoundException) {
return Response.seeOther(URI.create("/error.html"))
.status(Response.Status.NOT_FOUND)
.build();
}
return Response.seeOther(URI.create("/error.html"))
.status(Response.Status.INTERNAL_SERVER_ERROR)
.build();
}
In this case, when a ResourceNotFoundException
occurs, the user is redirected to a custom error page, enhancing interaction.
Testing Your Global Exception Handling
It is crucial to test your global exception handling thoroughly. You can utilize tools like JUnit along with Mockito to create tests that mimic exceptions.
Here is a simple JUnit test for our GlobalExceptionHandler
:
package com.example.controllers;
import org.junit.Test;
import javax.ws.rs.core.Response;
import static org.junit.Assert.assertEquals;
public class GlobalExceptionHandlerTest {
private final GlobalExceptionHandler handler = new GlobalExceptionHandler();
@Test
public void testResourceNotFoundException() {
Response response = handler.toResponse(new ResourceNotFoundException("Resource not found"));
assertEquals(Response.Status.NOT_FOUND.getStatusCode(), response.getStatus());
assertEquals("{\"message\":\"Resource not found\"}", response.getEntity());
}
// Additional tests can be added here.
}
Summary
Global exception handling is an essential feature in Java EE 8 MVC applications. It not only improves the user experience by providing clear responses but also ensures that your application's error handling strategy is consistent.
Additional Resources
If you want to delve deeper into Java EE specifications and best practices, consider checking out the Java EE 8 Documentation and Java EE on JBoss. Both will provide a broader context and additional guidelines.
By implementing global exception handling and following the best practices outlined in this post, you can create more reliable, user-friendly web applications that effectively manage errors. Remember, every error is an opportunity to enhance your application's resilience!