Mastering Java: Tackling Scalability in Mono-Repo Development
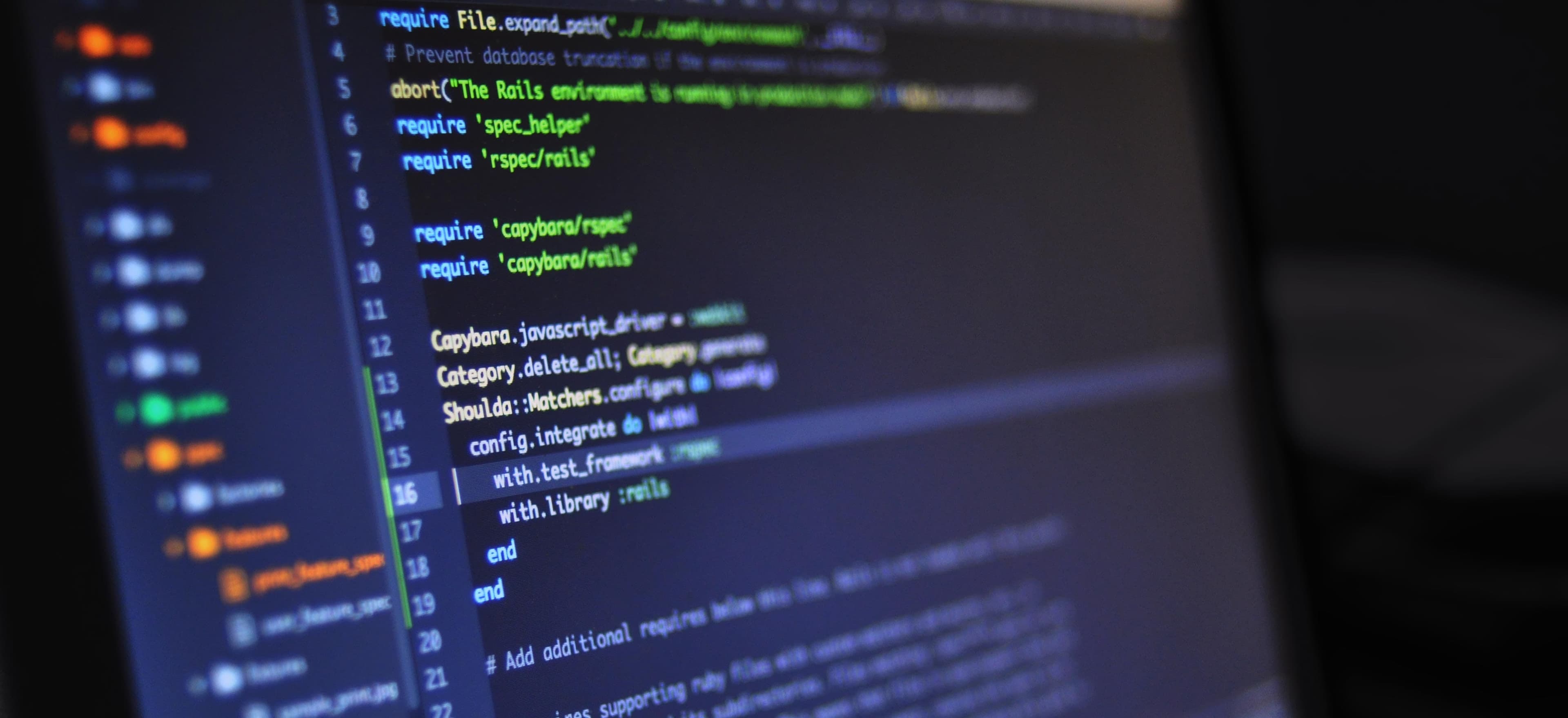
- Published on
Mastering Java: Tackling Scalability in Mono-Repo Development
In the ever-evolving landscape of software development, scalability is a critical concern. For teams managing large projects, especially those using mono-repositories (mono-repos), understanding how to overcome scalability challenges is crucial. This blog post will delve into mastering scalability in Java applications using a mono-repo strategy, drawing insights from existing resources, including the article "Overcoming Scalability Issues in Large Mono-Repos". You can read it here for an in-depth exploration.
What is a Mono-Repo?
A mono-repo, short for monolithic repository, is a software development strategy where multiple projects or services are stored in a single version-controlled repository. This approach can streamline dependency management and foster collaboration across teams. However, it brings its own set of challenges, particularly when it comes to scalability.
Advantages of Using a Mono-Repo
- Centralized Management: All code is stored in one place, making it easier for teams to manage and share resources.
- Synchronized Versioning: With all projects using the same repository, dependencies between projects can be aligned, reducing version mismatch issues.
- Improved Collaboration: Teams can more easily collaborate, as the entire codebase is visible to everyone.
Disadvantages of Using a Mono-Repo
- Build Performance: As the repository grows, build times can spike. This can be particularly challenging for large Java projects.
- Complex Dependency Management: With multiple services and libraries, dependency conflicts may arise.
- Tooling Limitations: Some CI/CD tools may struggle with very large repositories, leading to bottlenecks.
Java's Role in Mono-Repos
Java, known for its robustness and performance, is a popular choice for building large-scale applications. However, when an organization decides to adopt a mono-repo strategy, specific practices can enhance scalability. Below are essential strategies that developers can implement.
Modularization Through Java Modules
Java 9 introduced the module system, allowing developers to break applications into smaller, distinct modules. This modularity aids scalability by:
- Streamlining dependency management.
- Reducing the chances of version conflicts.
- Enabling developers to work on different modules independently.
Example: Creating a Module
module com.example.myapp {
requires java.logging; // Declares a dependency on the logging module
exports com.example.myapp.service; // Exports the service package
}
In this example:
- The
requires
keyword specifies that this module needs thejava.logging
module. - The
exports
keyword makes thecom.example.myapp.service
package accessible to other modules.
Using modules, teams can efficiently manage dependencies without compromising the build's overall performance.
Build Tools: Gradle and Maven
Using a build tool is crucial in managing a mono-repo. Gradle and Maven are two popular choices for Java projects.
- Gradle: It is flexible, allows incremental builds, and parallel execution across tasks, helping to speed up the build time.
- Maven: It provides a standardized project structure and dependency management that automates project builds.
Gradle Example
Below is a sample build.gradle
configuration for a Java project.
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0' // Example dependency
}
tasks.withType(JavaCompile) {
options.encoding = 'UTF-8'
}
In this configuration:
- The
repositories
block specifies the use of Maven Central for fetching dependencies. - The
dependencies
block defines the libraries used in the project. - The
tasks
block customizes the Java compilation process, ensuring that file encoding is set to UTF-8.
Optimizing Your CI/CD Pipeline
When employing a mono-repo strategy, scaling your Continuous Integration/Continuous Deployment (CI/CD) pipeline is essential. Here are some strategies to optimize it:
-
Use Caching: Enable caching to avoid redundant builds. For instance, caching dependencies ensures that they are not downloaded multiple times during CI runs.
-
Parallel Builds: Utilize parallel execution when deploying or testing different modules. This significantly speeds up the testing and deployment process.
-
Selective Builds: Only trigger builds for the modules impacted by recent changes instead of rebuilding the entire repository. This strategy minimizes wait times and unnecessary resource usage.
A CI/CD tool like Jenkins or GitHub Actions can be configured to utilize these strategies effectively, increasing the overall efficiency of your development process.
Monitoring and Feedback Loops
To truly tackle scalability issues, robust monitoring becomes essential. Integrating monitoring tools helps to pinpoint bottlenecks, poor performance, and other issues that may affect scalability.
Implementing Application Performance Monitoring (APM)
Integrating APM tools into your Java applications can provide real-time performance metrics. Tools such as New Relic or Prometheus can help monitor application performance, enabling proactive responses to scaling issues.
import com.newrelic.api.agent.NewRelic;
public class MyService {
public void processRequest() {
NewRelic.incrementCounter("Custom/ProcessRequest");
// Processing logic here
}
}
In this snippet, New Relic's API records a custom counter every time processRequest
is invoked. This data can later be analyzed to measure how many requests are being processed successfully, aiding in performance evaluation and forecasting potential scalability needs.
Agile Feedback Loops
With a mono-repo strategy, use Agile practices to create effective feedback loops. Regularly solicit feedback from team members about build performance and code quality. Incorporate tools for tracking technical debt and ensure timely remediation.
Final Thoughts
Tackling scalability challenges in mono-repo development requires strategic planning and effective implementation of Java features and tools. By modularizing your application, optimizing your build process, enhancing your CI/CD pipeline, and integrating robust monitoring, you can make significant strides in overcoming scalability issues.
For more insights on managing scalability in mono-repo projects, be sure to check out "Overcoming Scalability Issues in Large Mono-Repos" here. Embarking on this journey will not only improve your application's performance but also enhance collaboration and productivity across your teams.
By mastering these concepts and practices, you can ensure that your Java applications remain scalable and maintainable in the long run. Happy coding!
Checkout our other articles