The DateTime API: Is it Really What Java Developers Need?
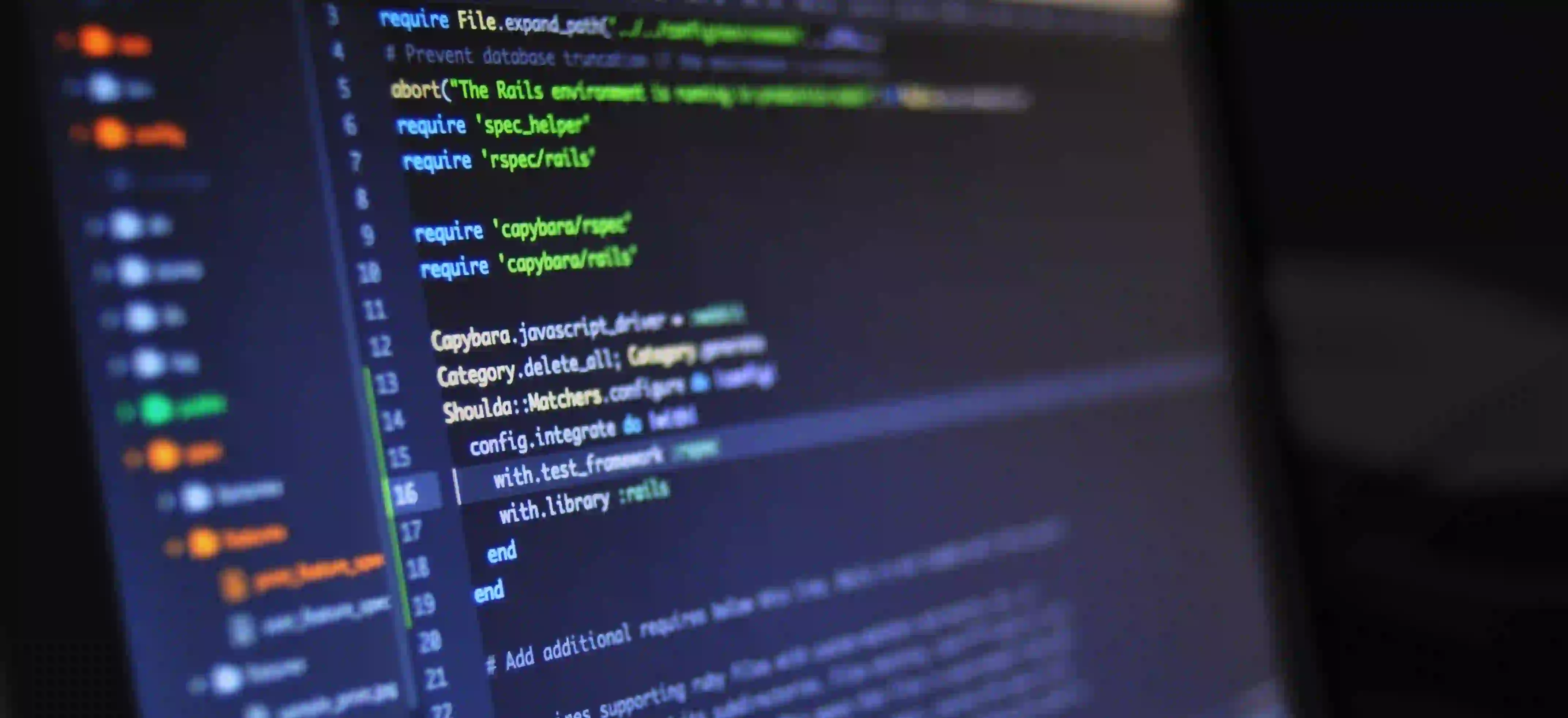
The DateTime API: Is it Really What Java Developers Need?
Java development has undergone significant transformations since its inception in the mid-'90s. One of the most notable additions is the DateTime API introduced in Java 8. This API aims to streamline date and time manipulation with a more robust and user-friendly approach compared to its predecessors. In this blog post, we will explore the DateTime API, why it was introduced, its key features, and whether it truly meets the needs of Java developers today.
A Brief History of Date and Time in Java
Before the introduction of the DateTime API, Java developers primarily relied on the java.util.Date
and java.util.Calendar
classes. However, these classes had significant limitations:
- Mutability:
Date
andCalendar
objects can be changed, which can lead to bugs in a multi-threaded environment. - Time Zones: Managing time zones with
Calendar
was cumbersome and error-prone. - Lack of Clarity: The API was not very intuitive, leading to code that was hard to read and maintain.
To address these issues, the DateTime API, based on the JSR-310 specification, was created. This new API drew inspiration from the widely used Joda-Time library, providing more powerful and flexible types for date and time manipulation.
Key Features of the DateTime API
The DateTime API consists of several key classes residing in the java.time
package. Here are some essential features:
1. Immutability
Classes like LocalDate
, LocalTime
, and LocalDateTime
are immutable. This means that once you create a date-time object, it cannot be altered. For instance:
import java.time.LocalDate;
LocalDate date1 = LocalDate.of(2023, 10, 31); // Create a LocalDate for Halloween
LocalDate date2 = date1.plusDays(1); // Create a new date for November 1st
System.out.println("Original date: " + date1); // Outputs: 2023-10-31
System.out.println("New date: " + date2); // Outputs: 2023-11-01
Why Immutability Matters: Immutability promotes safer code and helps avoid side effects, making your code easier to understand. In concurrent programming, immutability reduces the risk of data inconsistency.
2. Comprehensive Time Zones Support
Dealing with time zones is often a nightmare, but the DateTime API simplifies this with the ZonedDateTime
class.
import java.time.ZonedDateTime;
import java.time.ZoneId;
ZonedDateTime zonedDateTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("Current date and time in New York: " + zonedDateTime);
By passing a specific time zone (ZoneId
), you can easily manage problems associated with date-time transitions, such as daylight saving time.
Why Effective Time Zone Management Matters: Time zones can introduce complexities in applications that operate globally. Using a robust system helps ensure accuracy and prevents bugs in date-time calculations.
3. Fluent API Design
The DateTime API uses a fluent design pattern, making it easy to chain methods together for cleaner code.
import java.time.LocalDateTime;
LocalDateTime now = LocalDateTime.now();
LocalDateTime futureDate = now.plusDays(10).withHour(12).withMinute(30);
System.out.println("Future Date and Time: " + futureDate);
Why Clean Code Matters: Readable and maintainable code is vital in software development. A fluent API allows developers to express complex operations more clearly, enhancing collaboration and reducing the learning curve for new team members.
4. Comprehensive Formatting and Parsing
Formatting and parsing dates can often lead to tedious code. The DateTime API provides a dedicated formatter:
import java.time.format.DateTimeFormatter;
LocalDate date = LocalDate.of(2023, 10, 31);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
String formattedDate = date.format(formatter);
System.out.println("Formatted date: " + formattedDate);
Why Formatting is Important: Proper formatting ensures that date-time information is conveyed clearly, especially in user interfaces and reports.
5. Period and Duration
The DateTime API differentiates between periods (dates measured in years, months, days) and durations (time measured in hours, minutes, seconds). This distinction adds to the clarity:
import java.time.Period;
import java.time.Duration;
LocalDate start = LocalDate.of(2023, 1, 1);
LocalDate end = LocalDate.of(2024, 1, 1);
Period period = Period.between(start, end);
System.out.println("Period in days: " + period.getDays());
Duration duration = Duration.ofHours(5);
System.out.println("Duration in seconds: " + duration.getSeconds());
Why Distinction is Useful: Understanding the difference between periods and durations allows you to select the appropriate calculations for your application, enhancing precision and reducing bugs.
Are Java Developers Embracing the DateTime API?
The transition to the DateTime API reflects a broader trend as Java developers adapt to modern programming paradigms. Many developers praise the API for its clarity, convenience, and enhanced capabilities compared to the older Date and Calendar classes.
However, like any new feature, the DateTime API comes with a learning curve. Developers accustomed to the older classes may initially find the transition challenging. Nonetheless, the long-term benefits far outweigh the short-term hurdles.
Final Thoughts: Is It What Java Developers Need?
Absolutely! The Java DateTime API is a substantial improvement over its predecessors. It offers:
- Robustness through immutability.
- Ease of use with a fluent interface.
- Comprehensive support for time zones.
- Clarity through period and duration management.
- Flexibility in formatting and parsing.
As Java continues to evolve, maintaining and enhancing date and time handling will be critical. The DateTime API aligns with modern software engineering practices and supports developers in writing clear, effective, and efficient code.
For more information about the DateTime API, you can refer to the official documentation.
In closing, adopting the Java DateTime API can significantly enhance both the quality and maintainability of your code. It's a shift well worth making—so why not start today?