Scaling Java Frameworks: Tackling Mono-Repo Challenges
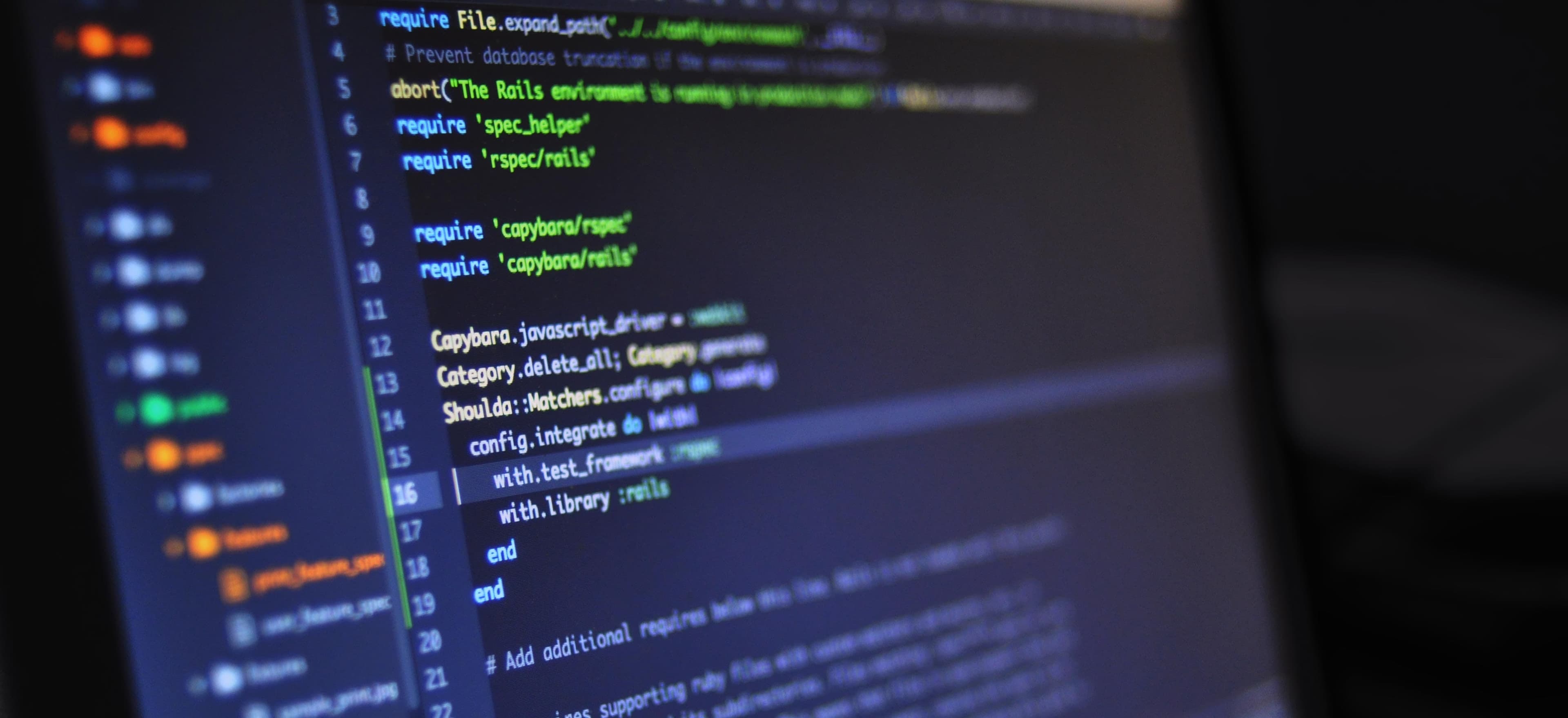
- Published on
Scaling Java Frameworks: Tackling Mono-Repo Challenges
In the world of software engineering, particularly with expansive projects, Java developers frequently encounter the complexities of managing large mono-repositories, or mono-repos. Mono-repos can enhance collaboration but also present unique challenges, especially when focused on scalability. In this blog post, we'll explore effective strategies for addressing scalability issues in mono-repos with Java frameworks, while linking back to insightful discussions in an existing article titled Overcoming Scalability Issues in Large Mono-Repos.
Understanding Mono-Repos
A mono-repo is a single repository that houses multiple related projects. The allure of mono-repos lies in their ability to facilitate code sharing, enforce uniformity, and streamline dependency management among teams. However, as projects scale, issues can arise, such as:
- Slower build times
- Dependency conflicts
- Codebase bloat
Given the Java framework's stronghold in enterprise applications, understanding how to optimize these challenges becomes imperative.
Build Optimization Techniques
One primary issue with large mono-repos is prolonged build times. Here are key strategies to mitigate this.
1. Incremental Builds
Incremental builds only compile code that has changed, rather than the entire repository. This can significantly reduce build times.
// Example using Gradle for incremental builds
tasks.register("myTask") {
outputs.upToDateWhen {
// Check if the outputs are up-to-date
false // Logic for determining if the output is new
}
}
Using Gradle's outputs.upToDateWhen
, you can customize the condition under which your build outputs are considered fresh.
Why? This approach ensures that your development cycle is more efficient, meaning less waiting and more coding.
2. Parallel Builds
Enable parallel builds wherever possible. Tools like Maven and Gradle inherently support parallel execution.
<!-- Maven POM for parallel builds -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<forkCount>2</forkCount>
</configuration>
</plugin>
</plugins>
</build>
Why? This feature allows multiple tasks to run at the same time, significantly speeding up the overall process.
3. Dependency Management
Implementing granular dependency management can greatly aid in building efficiency.
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
</dependency>
Using tools like Maven Dependency Plugin helps visualize dependencies and helps avoid conflicts or redundant dependencies.
Why? Keeping your dependency tree clear and concise minimizes conflicts and build issues.
Refactoring and Modularization
Large codebases can quickly become unwieldy. Refactoring is a proactive solution.
1. Extract Modules
Break down monolithic applications into smaller, manageable modules. Java's support for modules through Project Jigsaw adds structure to codebases.
module com.example.myapp {
requires com.example.myothermodule;
exports com.example.myapp.services;
}
Why? Encapsulating features into distinct modules allows you to isolate and manage changes effectively, enhancing both maintainability and scalability.
2. Use Version Control Wisely
Make use of version tags and branching to keep track of changes across different modules.
Why? This facilitates faster troubleshooting and provides clearer paths for collaborative development.
Efficient CI/CD Strategies
Integrating Continuous Integration (CI) and Continuous Deployment (CD) practices can streamline workflow.
1. Pipeline Optimization
Establish an efficient CI/CD pipeline that can identify and run only the necessary tests when code changes.
# Example CI configuration
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run Tests
run: ./gradlew test
Using CI tools like GitHub Actions or Jenkins efficiently enables only altered parts of the monorepo to be tested, rather than the entire codebase.
Why? This reduces resource allocation and speeds up development cycles.
2. Efficient Docker Usage
Utilizing Docker containers can standardize development environments and significantly decrease onboarding times.
# Example Dockerfile
FROM openjdk:11-jre-slim
COPY target/myapp.jar /app/
CMD ["java", "-jar", "/app/myapp.jar"]
By crafting succinct Docker images tailored for your Java applications, you reduce inconsistencies across environments.
Why? This streamlines deployments and ensures consistency, crucial in collaborative projects.
The Last Word
Navigating the scalability challenges within Java frameworks, particularly in mono-repos, requires strategic planning and execution. With techniques such as incremental builds, modularization, and efficient CI/CD practices, teams can foster a collaborative environment without sacrificing performance.
To explore more on the nuances of scalability in mono-repos, check out the insightful article Overcoming Scalability Issues in Large Mono-Repos. Embracing these techniques will not only smoothen the path for developers but also lead to more robust and scalable applications in Java.
Happy coding!
Checkout our other articles