Tackling CORS Issues in Java Web Applications with Azure
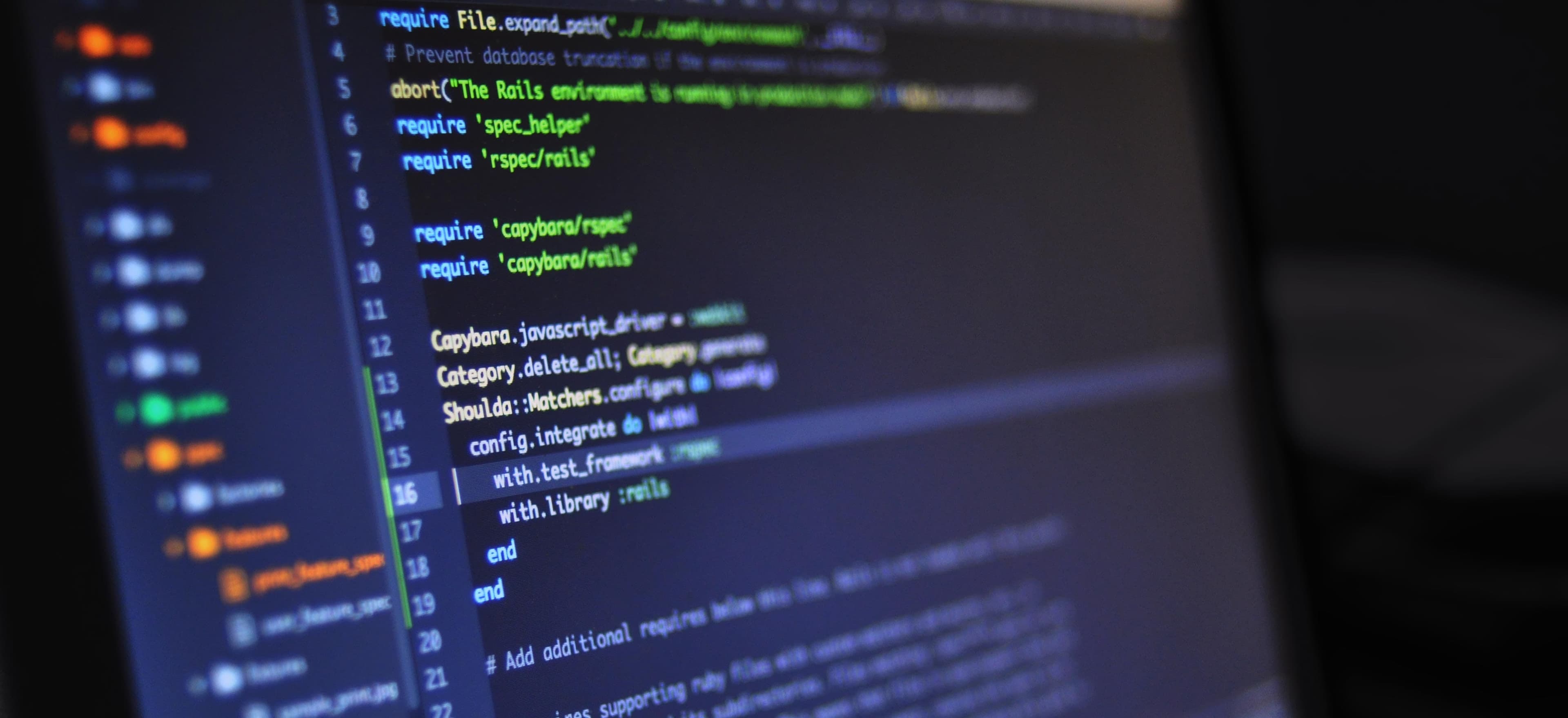
- Published on
Tackling CORS Issues in Java Web Applications with Azure
Cross-Origin Resource Sharing (CORS) is an essential aspect of web security. It dictates how your web application can interact with resources from different origins. In this blog post, we'll tackle CORS issues that developers often face in Java web applications deployed in Azure.
If you are looking for a comprehensive understanding of CORS issues specifically in Azure, you can find valuable insights in this article: Understanding CORS Issues in Azure: A Simple Guide.
What is CORS?
CORS is a security feature implemented by browsers to prevent malicious sites from reading sensitive data from another site. In simpler terms, it helps control how resources with different origins can communicate. When a web application tries to make a request to a resource from a different origin, the browser checks for CORS headers to determine whether the request should be allowed.
Common CORS Issues in Java Web Applications
When working with Azure, Java developers frequently encounter CORS-related challenges, particularly when they try to connect their frontend applications, like React or Angular, with a backend Java service (Spring Boot, for instance). Here are some frequent errors you may encounter:
- No 'Access-Control-Allow-Origin' Header: This indicates that the response from your API did not include the necessary CORS headers.
- CORS Preflight Request Failed: This indicates a failure during the mechanism called the "preflight" request, which is part of the CORS checks.
Why is Handling CORS Important?
Understanding and managing CORS settings in your Java application is crucial for several reasons:
- User Experience: Users expect smooth interactions across different domains. CORS misconfigurations can lead to HTTP errors, breaking functionality.
- Security: Properly configured CORS policies can prevent data leaks and unauthorized access.
- Performance: A well-optimized CORS setup can enhance the performance of your web application by reducing unnecessary round trips.
How to Resolve CORS Issues in Your Java Web Application
Updating Your Spring Boot Application
Most Java applications use frameworks like Spring Boot. To enable CORS, you can use the @CrossOrigin
annotation. This allows you to specify the origins that are permitted to access your resources.
Here is a straightforward example:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin(origins = "http://example.com") // Replace with your frontend origin
public class MyController {
@GetMapping("/api/data")
public String getData() {
return "Hello, CORS!";
}
}
Why Use @CrossOrigin?
Using the @CrossOrigin
annotation simplifies the process of enabling CORS for specific endpoints. This is beneficial in a development environment where you might be testing with different origins.
Global CORS Configuration
If you want to enable CORS for your entire application, you can create a global configuration class. This is especially useful if you have multiple controllers and need a consistent CORS policy across them.
Here's a code snippet demonstrating how to set it up globally:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://example.com") // Specify your frontend origin
.allowedMethods("GET", "POST", "PUT", "DELETE")
.allowedHeaders("*");
}
}
What Does This Code Do?
- addMapping("/")**: This allows CORS on all endpoints.
- allowedOrigins(...): This specifies which origins can access your API. Replace "http://example.com" with your frontend's actual URL.
- allowedMethods(...): This defines which HTTP methods are allowed. Customize it according to your needs.
- allowedHeaders("*"): This permits all headers. You can refine this for better security.
Handle CORS in Azure API Management
If you are hosting your Java application in Azure, you may also want to configure CORS in Azure API Management. This is especially useful if you are exposing APIs to third-party developers.
To enable CORS in Azure API Management:
- Navigate to your API Management instance in Azure.
- Click on "APIs" and select your API.
- In the settings, find the "CORS" tab.
- Set the allowed origins, methods, and headers based on your requirements.
Why Set CORS in API Management?
Setting CORS at the Azure API Management level centralizes your configuration. It reduces the overhead of adding CORS settings in all your services and ensures consistent behavior across your APIs.
Testing CORS
Once you've implemented CORS in your application, you should test it to ensure it behaves as expected. Tools like Postman or your browser’s developer tools can be used to make cross-origin requests and check for appropriate CORS headers in the response.
Troubleshooting CORS Issues
- Check the Network Tab: Use your browser's developer console to inspect the headers. Look for missing or incorrect CORS headers.
- Inspect CORS Policy Violations: Review error messages carefully to identify which CORS policy is violating.
- Debug Logging: Implement logging in your Java application to capture CORS-related requests and responses for deeper insights.
The Last Word
Resolving CORS issues in Java web applications deployed in Azure may seem daunting, but with a comprehensive understanding of how to configure CORS, it can be straightforward. Whether you choose to use annotations, global configurations, or Azure API Management settings, remember that proper CORS configuration not only enhances security but also optimizes the user experience.
For further insights into Azure-specific CORS challenges, check out the article Understanding CORS Issues in Azure: A Simple Guide.
In summary, securing your application with correctly tuned CORS policies is essential for seamless integration and user satisfaction. Rest assured, with the right configurations, you'll empower your Java web application to communicate across domains without any hitches.
Additional Resources
Make sure to implement these strategies into your Java applications, and embrace the complexity of CORS with confidence. Happy coding!