Solving JavaScript Compatibility for Dropdown Menus in IE
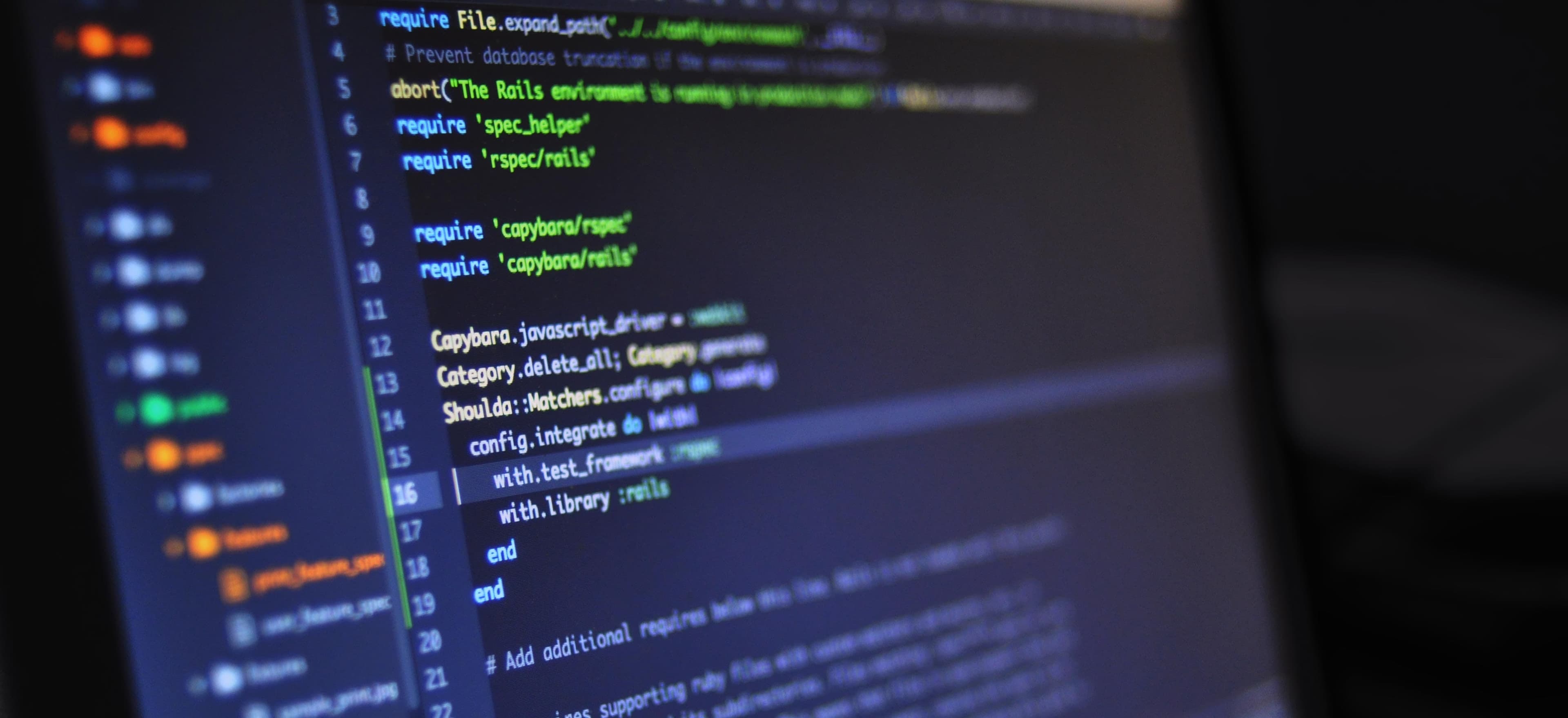
- Published on
Solving JavaScript Compatibility for Dropdown Menus in Internet Explorer
If you are a web developer, you have likely encountered compatibility issues with Internet Explorer (IE). One prevalent issue involves dropdown menus, particularly when dealing with transparency effects. In this blog post, we will delve into some practical JavaScript solutions that help create smooth and functional dropdown menus even in the notoriously finicky browser, Internet Explorer.
Understanding the Dropdown Menu Problems in IE
Dropdown menus can fail to display correctly in Internet Explorer due to various reasons, including improper handling of CSS styles or JavaScript logic that doesn't account for the quirks of IE. If you are using modern web technologies like CSS Flexbox or Grid, these may not render correctly in IE without specific workarounds.
A common issue is the transparent dropdown that disappears when hovered over. For a deeper understanding of some associated challenges, you might want to check out the article titled "Fixing Transparent Dropdown Menu Issues in Internet Explorer" at infinitejs.com/posts/fix-transparent-dropdown-ie-issues.
How to Approach Dropdown Menus with JavaScript
1. Structure Your HTML
Your HTML serves as the foundation for your dropdown menu. Here is an example of a basic structure:
<nav>
<ul class="menu">
<li class="menu-item">Home</li>
<li class="menu-item">
Services
<ul class="dropdown">
<li class="dropdown-item">Web Development</li>
<li class="dropdown-item">Graphic Design</li>
</ul>
</li>
<li class="menu-item">About</li>
<li class="menu-item">Contact</li>
</ul>
</nav>
In this layout, the dropdown menu is nested within a list item. Structuring your HTML this way is important because it provides a clear hierarchy, essential for dropdown menus.
2. Styling with CSS
Use CSS to ensure that the dropdown menu is hidden by default and only appears when the parent menu item is hovered over:
.menu {
list-style: none;
background-color: #333;
}
.menu-item {
position: relative;
display: inline-block;
padding: 15px;
color: white;
}
.dropdown {
display: none;
position: absolute;
background-color: #444;
list-style: none;
padding: 0;
margin: 0;
}
.menu-item:hover .dropdown {
display: block; /* This shows the dropdown on hover */
}
The use of position: absolute;
allows the dropdown to appear directly below the parent menu item. However, there is a drawback for certain versions of IE: the dropdown might not remain visible when using transparency. That’s where JavaScript comes in handy.
3. Implementing JavaScript
With JavaScript, we can add event listeners that manage the dropdown's display state, ensuring better compatibility across browsers. Here's a simple example to enhance the dropdown functionality:
document.querySelectorAll('.menu-item').forEach(item => {
item.addEventListener('mouseenter', () => {
const dropdown = item.querySelector('.dropdown');
if (dropdown) {
dropdown.style.display = 'block'; // Display the dropdown on hover
}
});
item.addEventListener('mouseleave', () => {
const dropdown = item.querySelector('.dropdown');
if (dropdown) {
dropdown.style.display = 'none'; // Hide the dropdown when cursor leaves
}
});
});
Why This Approach Works
- Event Listeners: By attaching event listeners for mouse enter and leave events, we have more granular control than the CSS hover approach, which can conflict with IE's rendering.
- Dynamic Style Changes: Directly manipulating the
style.display
property avoids potential issues with visibility and rendering that IE faces.
4. Testing for IE Compatibility
To ensure that your dropdown menus work in all browsers, including IE, it's essential to test your implementation. Here are some common testing methods:
- Emulation: Use IE's built-in developer tools to emulate various versions.
- Cross-Browser Testing Tools: Consider using tools like BrowserStack or Sauce Labs to run tests across different browser versions.
5. Enhancements for Functionality
Once the basic dropdown functionality is working, you might want to enhance it further. For instance, you can add animations to make the dropdown appear more dynamically. Here’s how you can implement a simple fade-in effect:
function fadeIn(element) {
element.style.opacity = 0;
element.style.display = 'block';
let last = +new Date();
const tick = () => {
element.style.opacity = +element.style.opacity + (new Date() - last) / 400;
last = +new Date();
if (+element.style.opacity < 1) {
requestAnimationFrame(tick);
}
};
tick();
}
document.querySelectorAll('.menu-item').forEach(item => {
item.addEventListener('mouseenter', () => {
const dropdown = item.querySelector('.dropdown');
if (dropdown) {
fadeIn(dropdown);
}
});
item.addEventListener('mouseleave', () => {
const dropdown = item.querySelector('.dropdown');
if (dropdown) {
dropdown.style.display = 'none'; // Hides dropdown
dropdown.style.opacity = 1; // Reset opacity for next time
}
});
});
This implementation creates a more visually appealing dropdown. The fadeIn
function smoothly transitions the menu's opacity, making it seem more polished.
A Final Look
Navigating the quirks of Internet Explorer doesn't need to be a daunting task. With the proper structuring of HTML, thoughtful CSS styling, and a sprinkle of JavaScript enhancement, you can ensure that dropdown menus work seamlessly, even on outdated browsers.
Remember that thorough testing is key. As web development continues to evolve, staying informed about browser compatibility issues and solutions will ensure a smoother user experience across the board.
For additional insights into dropdown menu issues specific to Internet Explorer, check out the article "Fixing Transparent Dropdown Menu Issues in Internet Explorer" at infinitejs.com/posts/fix-transparent-dropdown-ie-issues.
Happy coding!