Mastering CORS in Java Applications on Azure
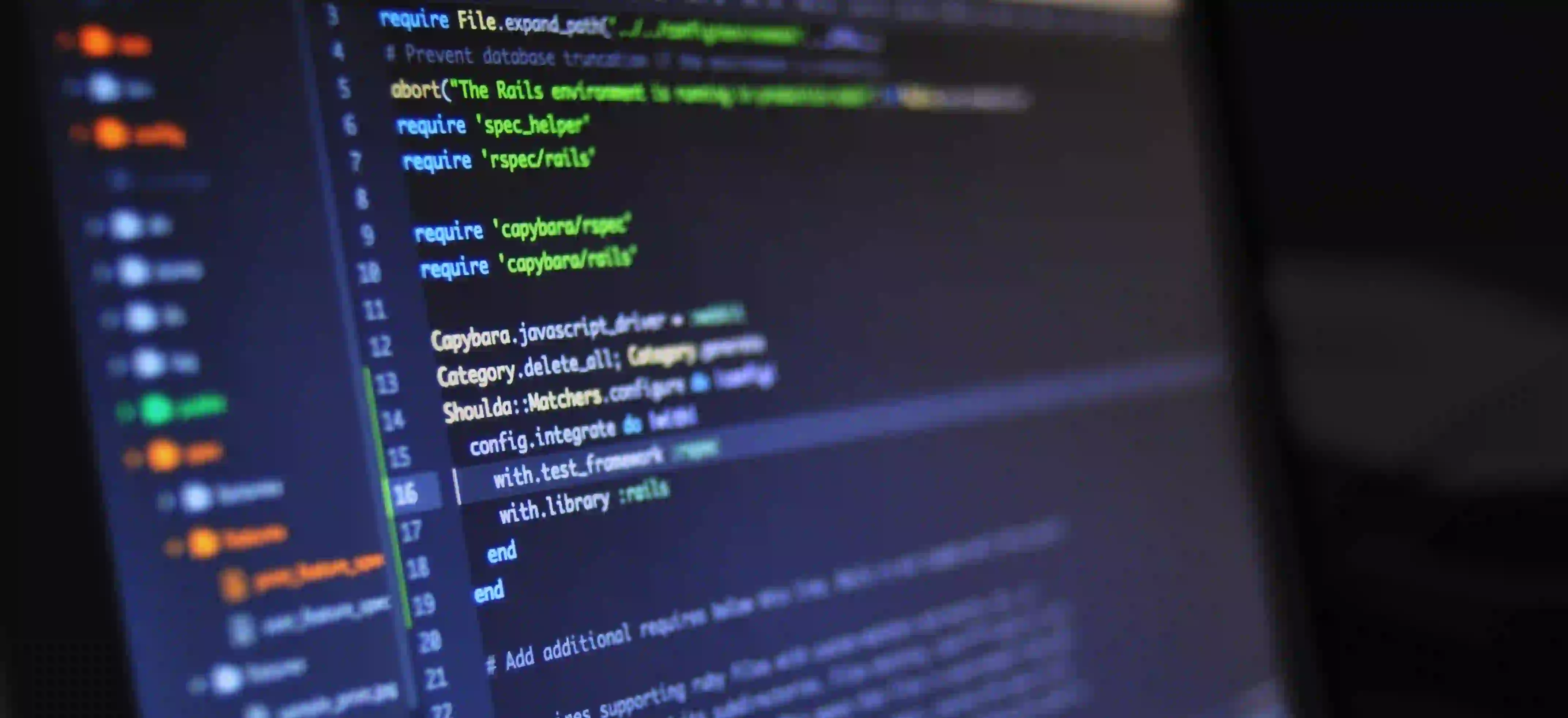
Mastering CORS in Java Applications on Azure
Cross-Origin Resource Sharing (CORS) is a critical web security feature that allows or restricts resources requested from another domain outside the current origin. While working with Java applications deployed on Azure, understanding and managing CORS effectively can be the difference between a seamless user experience and frustrating access issues. In this blog post, we’ll explore CORS and how to implement it in your Java applications on Azure, alongside best practices and troubleshooting tips for common issues.
What is CORS?
CORS is a mechanism that uses HTTP headers to tell browsers to give a web application running at one origin (domain) permission to access resources from a different origin. This is important for web applications that communicate with external APIs or services hosted on different domains.
For instance, your Java application running on https://myapp.azurewebsites.net
may need to access an API hosted at https://api.external-service.com
. Without CORS, the browser would block these requests because of the same-origin policy.
Key Terms:
- Origin: A combination of URL scheme, hostname, and port number.
- Preflight Request: An initial request sent using the OPTIONS method to determine if the actual request is safe to send.
Why CORS is Important
CORS is essential for web applications due to security implications. It ensures that malicious websites do not have access to sensitive user data on other domains without explicit permission.
Benefits of CORS
- Improves Security: Helps control which domains can access your resources.
- Enhances User Experience: Allows seamless integrations with third-party APIs.
- Enables Modern Web Applications: Essential for single-page applications (SPAs) that interact with backend services.
CORS Configuration in Java Applications
Now that we've covered what CORS is and why it matters, let's dive into how to implement it in Java applications deployed on Azure.
Setting Up CORS in a Spring Boot Application
Spring Boot is one of the most popular frameworks for developing Java applications. Here’s a simple guide to enable CORS in a Spring Boot application.
Step 1: Add Dependencies
If you're using Spring Boot, you typically don’t need to include any additional dependencies for CORS support as it is built into Spring Framework.
Step 2: Enable CORS Globally
You can configure CORS at a global level in your @Configuration
class. Here's how:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // Allow all endpoints
.allowedOrigins("https://example.com") // Specify allowed origin
.allowedMethods("GET", "POST", "PUT", "DELETE") // Specify allowed HTTP methods
.allowCredentials(true) // Allow credentials (cookies, authorization headers, etc.)
.allowedHeaders("*") // Allow all headers
.maxAge(3600); // Cache preflight requests for 1 hour
}
}
Why Use This Configuration?
- The
addMapping("/**")
line enables CORS for all endpoints in your application, allowing cross-origin requests. - By specifying
allowedOrigins
, only requests from this origin will succeed. - Setting
allowCredentials(true)
allows the inclusion of credentials (like cookies).
Step 3: Securing with Annotations
For more granular control, you can also use @CrossOrigin
annotations directly on your REST controllers:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin(origins = "https://example.com")
public class MyController {
@GetMapping("/data")
public String getData() {
return "This is secure data!";
}
}
Why Use Annotations? This allows targeted CORS policies on specific endpoints rather than globally, enhancing security by limiting exposure.
CORS in Jakarta EE
If you are working with Jakarta EE, you can configure CORS in a Filter
:
import javax.servlet.*;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CORSFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletResponse response = (HttpServletResponse) res;
response.setHeader("Access-Control-Allow-Origin", "https://example.com");
response.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
response.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization");
response.setHeader("Access-Control-Max-Age", "3600");
chain.doFilter(req, res);
}
public void init(FilterConfig filterConfig) {}
public void destroy() {}
}
Deploying on Azure
Once your Java application is set up and you’ve implemented the CORS policies, the next step is deployment. Azure provides seamless integration for deploying Java web applications.
- Create Azure Web App: Navigate to Azure Portal and create a new Web App.
- Deploy Your Application: Use Azure CLI, Git, or any CI/CD tool to deploy your Java application.
- Test CORS Implementation: Use tools like Postman or your browser’s developer console to test if CORS headers are correctly set when calling your API.
Troubleshooting CORS Issues
CORS issues can often frustrate developers, especially in production environments. Here are some steps to troubleshoot:
- Check Browser Console: Look for CORS errors in the console. They often tell you what’s missing.
- Verify Allowed Origins: Ensure that your specified origins match the calling domain (including ports).
- Preflight Requests: If using custom headers or methods, confirm that your server correctly handles preflight (OPTIONS) requests.
- Examine Server Logs: Sometimes CORS failures stem from server-side errors. Check the logs for more information.
For an in-depth understanding of how CORS issues can arise on the Azure platform specifically, refer to the article Understanding CORS Issues in Azure: A Simple Guide.
Final Thoughts
Mastering CORS in Java applications, especially when deployed on Azure, is crucial for ensuring security while providing seamless user experiences. By following the configuration techniques illustrated above and employing best practices when dealing with cross-origin requests, you can efficiently manage and troubleshoot CORS in your applications.
Whether using Spring Boot or Jakarta EE, the key takeaway is to pay close attention to your CORS settings. Allow only the necessary origins and methods to minimize security risks, ensuring your applications remain robust and secure.
By understanding and leveraging CORS correctly, you unlock the full potential of modern web applications to enhance user satisfaction and promote smoother integrations with external services.