Resolving UI Overlaps in Java Web Apps on Older Browsers
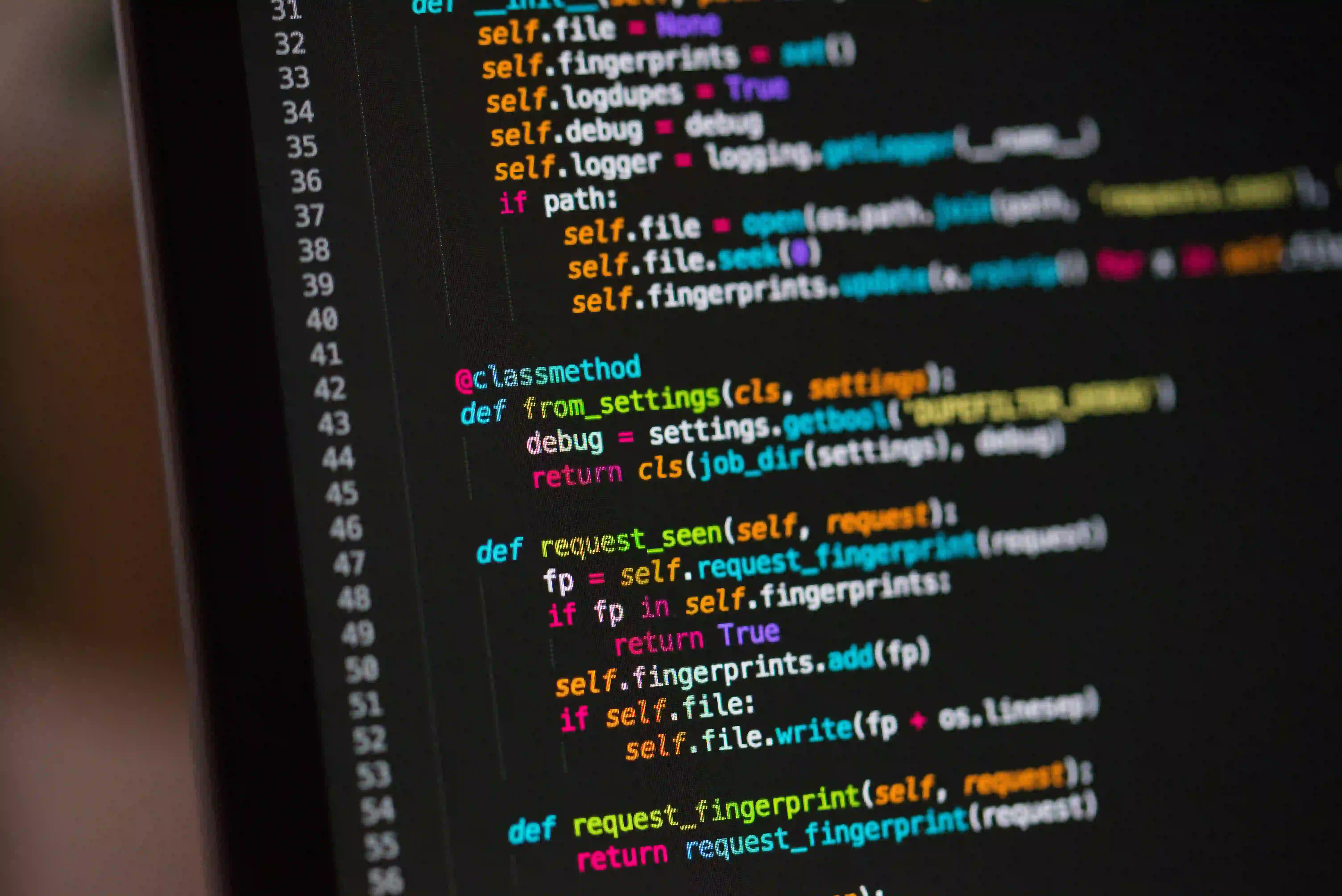
Resolving UI Overlaps in Java Web Apps on Older Browsers
Modern web applications often take advantage of advanced styling features, relying on CSS for responsive and visually appealing interfaces. However, when it comes to older browsers, these advanced features can lead to unexpected UI issues, particularly overlaps that make the application user experience poor. In this blog post, we will explore effective techniques to handle UI overlaps in Java web applications, especially in the context of older web browsers.
Understanding the Importance of UI
User Interface (UI) design is a crucial aspect of software development. A well-designed UI encourages user interaction and improves the overall experience. When deploying web applications, particularly business-critical ones, it's essential to consider how they will behave across different browsers, especially older ones like Internet Explorer.
Older browsers often lack support for modern CSS techniques that can create overlapping elements, breakdowns in responsivity, and other issues that impact usability. This problem can lead to frustration and, in some cases, lost revenue. In this article, we will focus on effective strategies for minimizing overlap issues and improving your web application’s cross-browser compatibility.
Common Causes of UI Overlaps
Before diving into solutions, let’s identify some common causes of UI overlaps in older browsers:
- CSS Layout Techniques: Certain CSS properties such as Flexbox and Grid may not be supported in older browsers.
- Z-Index Mismanagement: Using z-index improperly can cause elements to overlap unexpectedly.
- Poorly Configured Margins and Paddings: Elements with large margins or paddings can cause items to shift, creating overlaps.
- Opacity and Visibility Issues: Opacity settings may not render properly, especially if combined with position properties.
Example of a UI Overlap Scenario
Let’s consider a simple HTML layout:
<div class="header">Header</div>
<div class="content">Content</div>
<div class="footer">Footer</div>
In a modern environment, this layout might look fine, but in an outdated browser, you may experience overlaps if CSS is not handled properly:
.header {
position: fixed;
top: 0;
width: 100%;
}
.content {
margin-top: 50px; /* Header height */
padding: 20px;
}
.footer {
position: absolute;
bottom: 0;
width: 100%;
}
Practical Solutions to Prevent UI Overlaps
1. Utilize Feature Detection
To ensure that your web application behaves consistently across all browsers, implement feature detection using tools like Modernizr. This JavaScript library allows you to check for support of various CSS and JavaScript features:
if (!Modernizr.flexbox) {
// Load fallback CSS for those without Flexbox support
document.write('<link rel="stylesheet" href="fallback.css">');
}
Why:
This approach ensures that users are served a more compatible version of your styles, maintaining usability.
2. Rely on Compatible CSS Techniques
Instead of relying solely on Flexbox or CSS Grid, you can achieve layout with older techniques like floating or inline-block elements. Here's an adjusted example using floats:
.header {
float: left;
width: 100%;
}
.content {
margin-top: 50px; /* Ensure there is sufficient margin down */
padding: 20px;
overflow: hidden; /* Clear floats */
}
.footer {
float: left;
width: 100%;
}
Why:
Using floats provides wider compatibility, ensuring that layouts render correctly in older browsers.
3. Managing Z-Index Carefully
To avoid the confusion caused by overlapping elements, use z-index thoughtfully. Always define the position property:
.header {
position: relative;
z-index: 10;
}
.content {
position: relative;
z-index: 5; /* Lower z-index than header to prevent overlap */
}
.footer {
position: relative;
z-index: 1; /* The footer should have the lowest z-index */
}
Why:
Clearly defined z-index values help prevent accidental overlaps, making it easy to manage which elements appear on top.
4. Implement Conditional Comments for IE
If your users are primarily using older versions of browsers like IE, consider adding conditional comments directly in your HTML:
<!--[if lt IE 9]>
<link rel="stylesheet" type="text/css" href="ie8-and-down.css">
<![endif]-->
Why:
This will deliver a targeted CSS file to users on those browsers to ensure better rendering.
5. Minimize Absolute Positioning
While position: absolute
can be convenient, it can lead to unforeseen overlaps, especially when elements are placed in nested containers. Always strive to rely on relative positioning when possible.
.footer {
position: relative; /* Use relative instead */
}
Why:
This change keeps the footer in its natural flow and prevents it from overlapping other content.
Testing Across Browsers
Once you’ve implemented these solutions, always remember to test across different browsers, especially those you anticipate your users may employ. Tools like BrowserStack and Sauce Labs enable testing on multiple browsers and versions.
The Last Word
Addressing UI overlaps in Java web applications, particularly with older browsers, is crucial for ensuring a pleasant user experience. Through careful planning, CSS techniques, and appropriate use of JavaScript and HTML, you can build a cross-browser compliant application.
As discussed, applying practices like feature detection, using backward-compatible CSS, managing z-index, and avoiding excessive use of absolute positioning can drastically enhance your application's usability.
For more insights on fixing browser-specific issues, consider reading the article, Fixing Transparent Dropdown Menu Issues in Internet Explorer.
By integrating these methods into your development process, you will significantly mitigate the risk of UI overlaps, thereby creating a smoother experience for all users, regardless of the browsers they prefer.