Bridging Java Development and DevOps Culture Shift
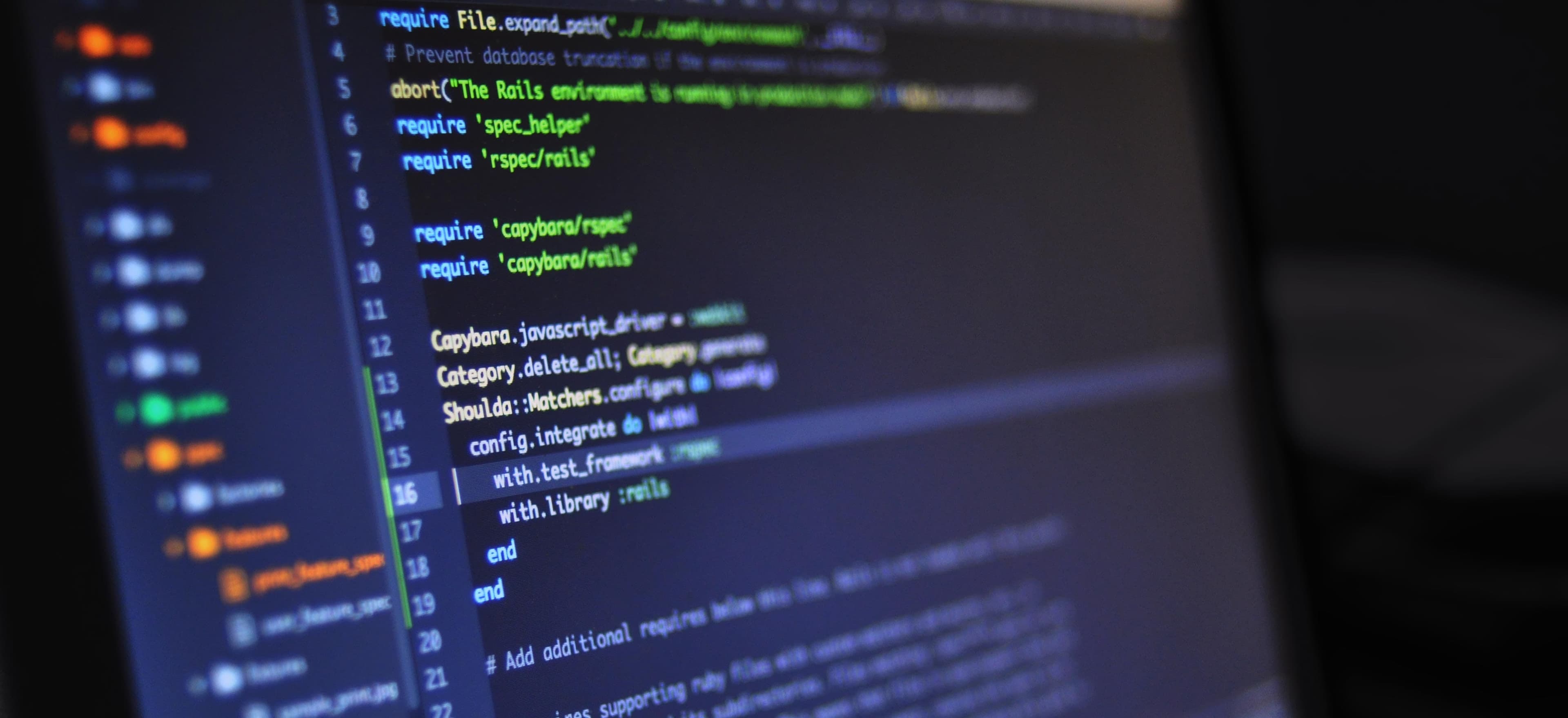
- Published on
Bridging Java Development and DevOps Culture Shift
In the fast-paced world of software development, bridging the gap between Java development and a DevOps culture shift is crucial for successful project delivery. Java, a long-standing stalwart in the programming community, offers robust frameworks and tools. However, to thrive in today’s environment, Java developers must embrace the principles of DevOps—a culture that emphasizes collaboration, automation, and continuous integration.
Understanding DevOps and Culture Transformation
DevOps, a fusion of "Development" and "Operations," is a methodology aimed at integrating software development and IT operations. It promotes shorter development cycles, increased deployment frequency, and more dependable releases, in alignment with business objectives.
Culture plays a pivotal role in this transformation. A strong DevOps culture encourages a mindset shift, where teams are motivated to collaborate across traditionally siloed roles. This synergy is essential, as highlighted in the insightful article The Role of Culture in DevOps Transformation. Understanding this cultural aspect sets the stage for Java developers to adopt DevOps practices effectively.
Why Java Developers Should Adapt to DevOps
Java is versatile and widely used in enterprise environments. Adopting DevOps practices can significantly enhance Java development projects by:
- Fostering Collaboration: Breaking down silos between development and operations.
- Enhancing Efficiency: Streamlining processes through automation.
- Improving Quality: Encouraging continuous testing and integration.
- Accelerating Time-to-Market: Enabling faster delivery of features and fixes.
Code Example: Continuous Integration with Jenkins
To illustrate how Java developers can leverage DevOps practices, let’s take a closer look at how to set up a CI/CD pipeline using Jenkins. Jenkins is an open-source automation server that supports building, deploying, and automating any project.
Here’s a simple Jenkins pipeline script written in Groovy for a Java application:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
// Running Maven build tool to compile the application
sh 'mvn clean package'
}
}
}
stage('Test') {
steps {
script {
// Running unit tests using Maven
sh 'mvn test'
}
}
}
stage('Deploy') {
steps {
script {
// Deploying the application to the server
sh 'scp target/myapp.jar user@server:/path/to/deploy'
}
}
}
}
}
Commentary
-
Pipeline Structure: The pipeline is structured into three fundamental stages: Build, Test, and Deploy. This mirrors the DevOps philosophy of continuous integration and delivery.
-
Build Stage: The mvn clean package command compiles the Java application. Artifact creation is essential in DevOps for tracking changes easily.
-
Test Stage: The mvn test command runs unit tests, ensuring that code changes don’t break existing functionality. Automation of testing is crucial for quick feedback.
-
Deploy Stage: The command scp target/myapp.jar user@server:/path/to/deploy achieves automated deployment to a server, which enhances efficiency.
By utilizing Jenkins in this manner, Java developers establish a robust CI/CD pipeline that aligns with DevOps principles.
Embracing Agile Methods for Effective Development
The Agile methodology complements DevOps by emphasizing iterative development and frequent delivery. Java developers can implement Agile practices in various ways:
-
Daily Stand-up Meetings: Regular communication enhances collaboration and quickly identifies blockers.
-
Sprint Planning: Focuses on a defined scope of work over a short period, facilitating rapid delivery and adaptation.
-
Retrospectives: Reflecting on past sprints allows teams to learn and improve processes continuously.
Code Example: Agile User Story Representation
Let's consider a User Story that a Java development team might use to drive Agile practice:
**User Story: As a user, I want to be able to reset my password so that I can regain access to my account.**
**Acceptance Criteria:**
- User should receive a reset link via email.
- Link should expire after 24 hours.
- Password must meet minimum security requirements.
**Tasks:**
1. Implement email service for sending reset links.
2. Create REST API endpoint for password reset.
3. Develop front-end interface for user interaction.
Discussion
Utilizing user stories like this one fosters clear communication between developers and stakeholders while providing a foundation for development.
Integration of Monitoring Tools
Another critical aspect of bridging Java development with a DevOps culture shift is the integration of monitoring tools. Tools such as Prometheus and Grafana can be instrumental in tracking application performance and user behavior in real-time.
Code Example: Adding Prometheus Metrics in a Spring Boot Application
For a Java Spring Boot application, integrating Prometheus can be straightforward. First, add the following dependency to your pom.xml
:
<dependency>
<groupId>io.prometheus</groupId>
<artifactId>simpleclient_springboot</artifactId>
<version>2.3.1</version>
</dependency>
Next, expose metrics by adding the following configuration in your Spring Boot application:
import io.prometheus.client.spring.autoconfigure.EnablePrometheus;
@EnablePrometheus
@SpringBootApplication
public class YourApplication {
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
}
Commentary on Monitoring Integration
This configuration exposes important metrics that can be scraped by Prometheus.
-
Performance Monitoring: Being able to analyze performance in real-time is essential for quick decision-making.
-
Alerting: When thresholds are exceeded (e.g., high CPU usage), alerts can be generated, prompting immediate investigation.
Key Takeaways and Next Steps
To effectively bridge the gap between Java development and a DevOps culture, developers should focus on:
-
Culture Shift: Embrace collaboration and open communication.
-
Automation: Utilize CI/CD tools like Jenkins to automate the software delivery process.
-
Agile Practices: Implement Agile methodologies to remain adaptive and iterative.
-
Monitoring: Integrate tools like Prometheus and Grafana for real-time insights.
By recognizing and implementing these strategies, Java developers can become integral players in their organizations' transformation toward DevOps. This, in turn, allows them to not only contribute to their projects effectively but also align with the evolving needs of the software industry.
For further insights on the culture aspect of DevOps transformation, readers can refer to the article The Role of Culture in DevOps Transformation.
By practicing these recommendations and actively participating in the cultural shift, Java developers can leverage their skills to thrive in the modern software landscape, ultimately resulting in improved project delivery and satisfaction for both developers and stakeholders alike.