Bridging Java Practices with DevOps Culture for Success
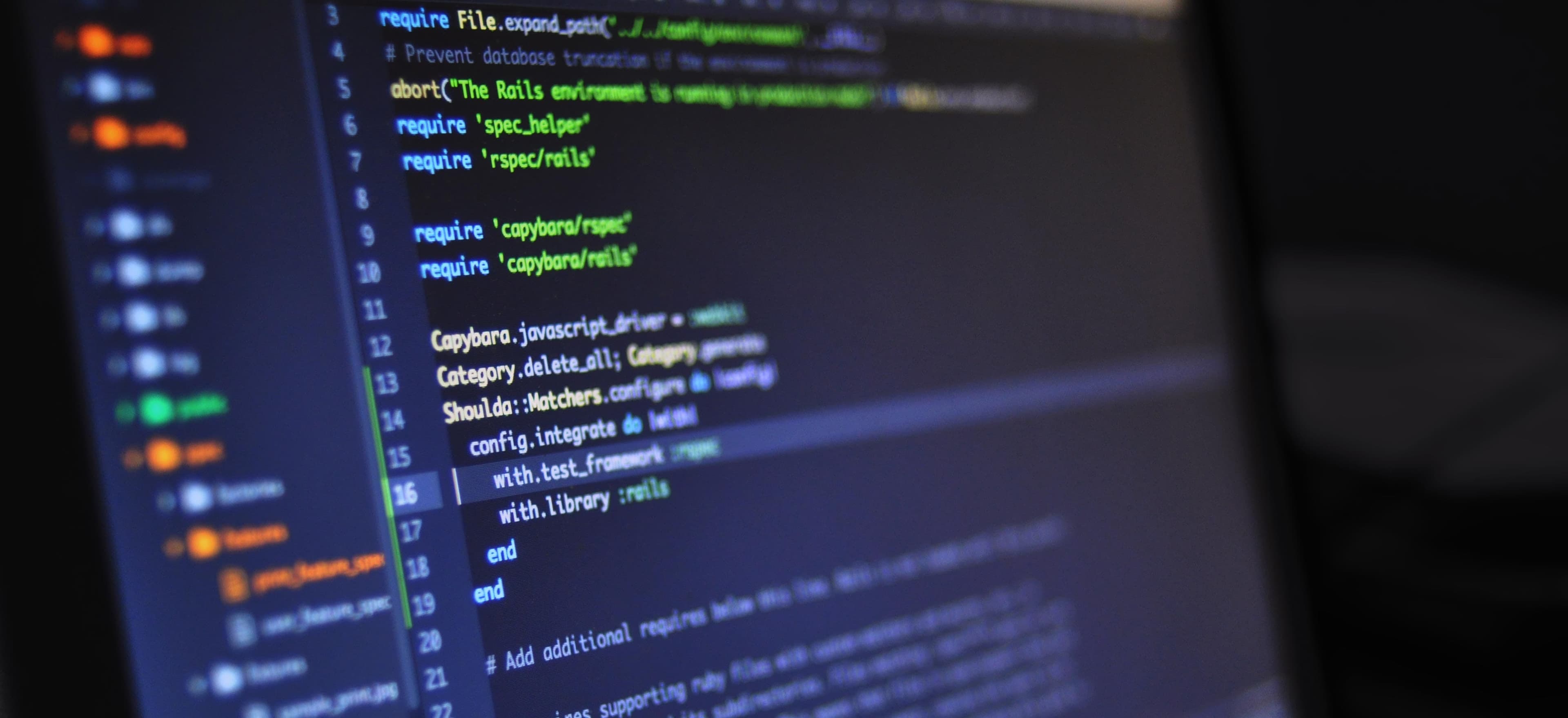
- Published on
Bridging Java Practices with DevOps Culture for Success
In the modern development landscape, merging programming practices with an evolving cultural framework is crucial. For Java developers, embracing DevOps principles can enhance their workflows, improve collaboration, and accelerate delivery pipelines. This post will explore how Java practices can be integrated within a DevOps culture, ensuring that developers not only write efficient code but also contribute to a harmonious operational environment.
Understanding DevOps Culture
What is DevOps?
DevOps is a set of practices designed to enhance collaboration between development and operations teams. It encourages a culture of rapid iterations and seamless integration, significantly impacting how software is developed, tested, and released.
Why is Culture Key to DevOps?
As discussed in the article "The Role of Culture in DevOps Transformation", cultivating a supportive environment that values collaboration greatly influences the success of DevOps initiatives. Culture shapes team dynamics, communication, and ultimately impacts project outcomes.
Core Cultural Principles in DevOps
Some foundational elements of the DevOps culture include:
- Collaboration: Breaking down silos between teams.
- Continuous Learning: Encouraging teams to experiment and learn from failures.
- Automation: Streamlining processes to minimize manual intervention.
- Customer-Centricity: Prioritizing user needs through iterative feedback.
Incorporating these principles into your Java practices not only leads to seamless deployments but also fosters a productive working environment.
Java's Role in a DevOps Ecosystem
Java is a powerful and versatile programming language that has a vast array of frameworks and tools ideal for DevOps. It empowers teams to build scalable applications and integrate seamlessly into DevOps pipelines. Let's examine some critical Java practices essential for aligning with a DevOps culture.
1. Embracing Agile Methodologies
Agile development complements DevOps philosophies by promoting iterative progress and flexibility.
Code Example: Implementing Agile Concepts
public class UserStory {
private String title;
private String description;
public UserStory(String title, String description) {
this.title = title;
this.description = description;
}
public void implement() {
System.out.println("Implementing user story: " + title);
// Further code for implementing user stories
}
}
Commentary: Here, we define a simple UserStory class. Each user story represents a feature requirement and can be implemented incrementally. The agile approach allows developers to focus on delivering small, functional, and valuable increments. This aligns perfectly with the DevOps ethos of frequent, reliable releases and minimizes risks.
2. Test-Driven Development (TDD)
TDD focuses on writing tests before writing the corresponding application code, enhancing code quality and reliability.
Code Example: TDD Implementation
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
class Calculator {
public int add(int a, int b) {
return a + b; // Simple addition operation
}
}
Commentary: This example showcases a simple TDD approach with the JUnit testing framework. By defining test cases first, developers ensure that their code meets specific requirements. TDD aligns well with DevOps, where automated testing is a pivotal process in Continuous Integration (CI).
3. Continuous Integration and Continuous Deployment (CI/CD)
CI/CD pipelines automate the process of integration and deployment. Java developers should leverage CI/CD tools to establish robust workflows.
Code Example: Jenkins Pipeline Script
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
echo 'Building the Java application...'
sh 'javac -d out src/**/*.java'
}
}
}
stage('Test') {
steps {
script {
echo 'Running tests...'
sh 'java -cp out org.junit.runner.JUnitCore CalculatorTest'
}
}
}
stage('Deploy') {
steps {
script {
echo 'Deploying the application...'
sh 'deploy_script.sh' // Custom deployment script
}
}
}
}
}
Commentary: This Jenkins pipeline outlines stages for building, testing, and deploying a Java application. Automating these stages reduces human error and speeds up delivery. Aligning CI/CD practice with DevOps fosters an environment of reliability and quick feedback, essential for innovation.
4. Infrastructure as Code (IaC)
IaC enables developers to manage and provision infrastructure through code, making the setup reproducible and manageable.
Code Example: Terraform Configuration
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "java_app" {
ami = "ami-12345678" // Specified AMI for Java application
instance_type = "t2.micro"
}
Commentary: Here, we define a Terraform configuration for deploying a Java application on AWS. Using IaC promotes consistency and reduces errors associated with manual setups, blending perfectly into the DevOps culture where automation is key.
5. Monitoring and Logging
Implementing effective monitoring and logging practices ensures that applications run smoothly in production.
Code Example: Using SLF4J for Logging
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Application {
private static final Logger logger = LoggerFactory.getLogger(Application.class);
public static void main(String[] args) {
logger.info("Starting application...");
// Application code...
logger.info("Application running successfully.");
}
}
Commentary: Using a logging library like SLF4J provides easy access to application logs, improving observability. Monitoring performance is essential in a DevOps setup, allowing teams to identify and rectify issues proactively.
Key Takeaways
- Culture Shapes Success: Adopt a collaborative and learning-oriented approach to maximize success rates in DevOps.
- Adopt Agile Practices: Break down projects into manageable user stories to foster iterative delivery.
- Utilize Test-Driven Development: Ensure code quality by implementing TDD rigorously.
- Embrace CI/CD Pipelines: Automate building, testing, and deploying processes for quicker feedback loops.
- Implement Infrastructure as Code: Simplify infrastructure management while promoting reproducibility and version control.
- Focus on Monitoring and Logging: Monitor application performance to ensure its reliability in production.
The Last Word
Incorporating Java practices into a DevOps culture is not just beneficial; it is essential for organizations aiming for increased agility and efficiency. By closing the gap between development and operations, teams can deliver high-quality software faster and with more reliability.
As Java developers, embracing the cultural aspects of DevOps will drive success not just in technical delivery but also in fostering a collaborative environment. By aligning our practices with DevOps principles, we pave the way for innovation and continuous improvement.
For further insights on building a robust DevOps culture, refer to the article "The Role of Culture in DevOps Transformation".