Securing Java Web Apps: Combat Session Security Risks
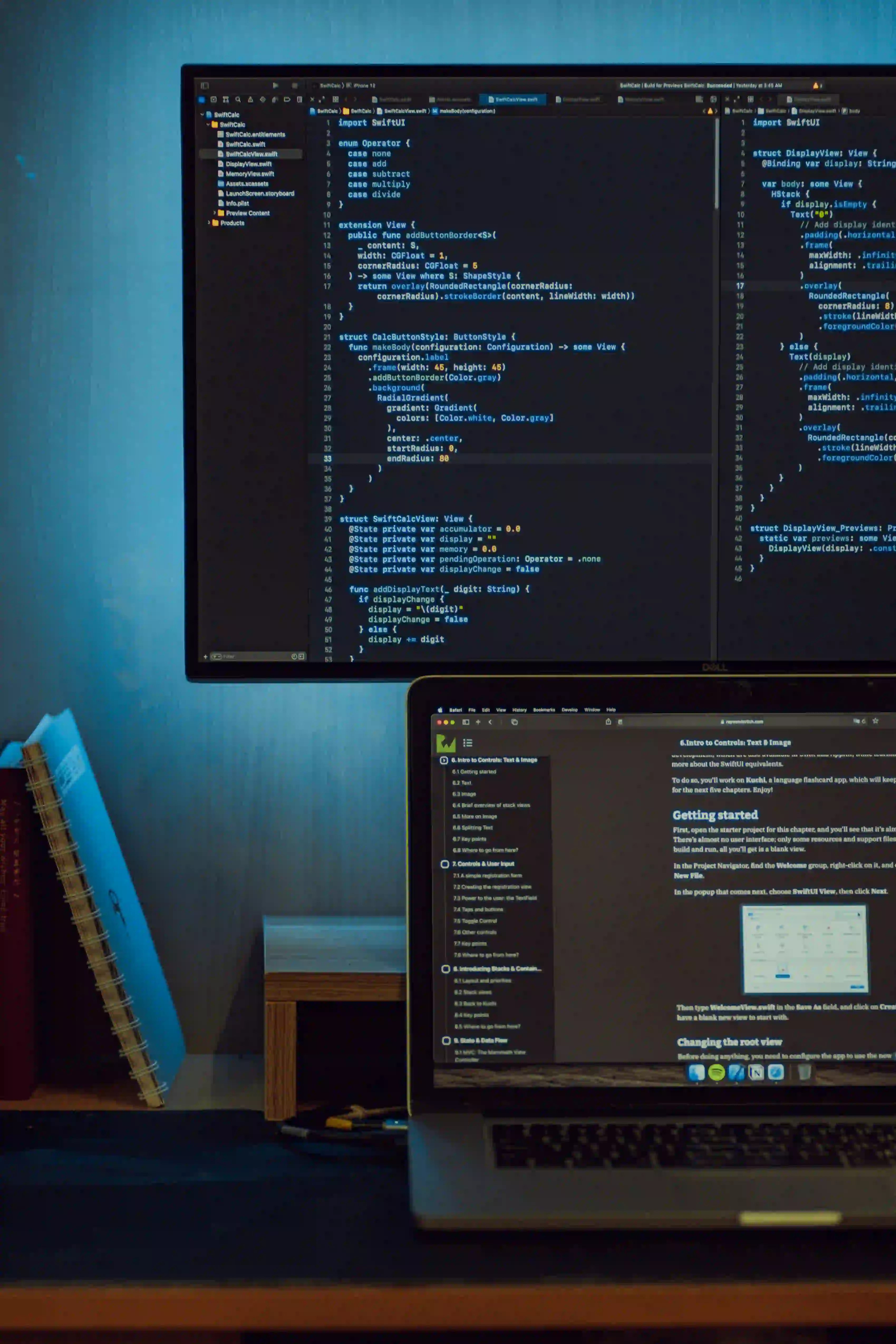
Securing Java Web Apps: Combat Session Security Risks
As the digital landscape evolves, so do the threats that challenge the security of web applications. A critical area of focus for developers is session security. A poorly designed session management system can lead to significant vulnerabilities, opening doors for attackers. In this blog post, we will dive into best practices and strategies to enhance session security in Java web applications. We will also touch upon the insights from the article titled "Is Your Login Safe? Unveiling Session Security Flaws," which can be found at infinitejs.com/posts/login-safety-unveiling-session-security-flaws.
Understanding Session Security Flaws
What is a session? A session is a stateful interaction between a user and a web application. When you log into a web application, the server creates a session to keep track of your interactions. However, if session management lacks robustness, unauthorized access can occur.
Common session security vulnerabilities include:
- Session Hijacking: When an attacker steals a user's session ID, they can impersonate them.
- Session Fixation: An attacker forces a user to authenticate with a known session ID.
- Cross-Site Request Forgery (CSRF): Attackers trick users into executing unwanted actions on trusted sites.
To mitigate these vulnerabilities, a foundational understanding of secure session management is essential.
1. Use Secure Session IDs
Why Secure Session IDs?
Session IDs are the keys to a user's session; if they are intercepted, attackers gain access to user accounts.
Implementation Example:
Here is a code snippet to demonstrate how to generate a secure session ID in Java:
import javax.servlet.http.HttpSession;
import java.security.SecureRandom;
import java.util.Base64;
public class SessionUtil {
private static final SecureRandom secureRandom = new SecureRandom();
public static String generateSessionId() {
byte[] randomBytes = new byte[24];
secureRandom.nextBytes(randomBytes);
return Base64.getUrlEncoder().withoutPadding().encodeToString(randomBytes);
}
public static void createSession(HttpSession session) {
session.setId(generateSessionId());
session.setMaxInactiveInterval(30 * 60); // 30 minutes
}
}
Commentary:
- SecureRandom is used for generating random bytes to enhance randomness.
- Base64 encoding ensures that the session IDs are URL safe.
- Setting a max inactive interval prevents sessions from lingering indefinitely.
2. Enforce HTTPS
Why HTTPS?
Transporting session IDs over HTTP makes them susceptible to eavesdropping. HTTPS encrypts the data in transit, safeguarding sensitive information.
Implementation Tips:
- Acquire an SSL certificate from a trusted Certificate Authority (CA).
- Ensure all HTTP requests redirect to HTTPS using server configurations or frameworks.
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>user</role-name>
</auth-constraint>
<user-data-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</user-data-constraint>
</security-constraint>
Commentary:
In this web.xml configuration, we enforce HTTPS by specifying that all traffic to the protected area should be confidential. This approach protects session IDs and user authentication tokens.
3. Implement Session Timeout
Why Session Timeout?
Automatically logging users out after a period of inactivity minimizes the risk of unauthorized access from abandoned sessions.
Implementation Example:
import javax.servlet.http.HttpSessionListener;
import javax.servlet.http.HttpSessionEvent;
public class SessionListener implements HttpSessionListener {
@Override
public void sessionCreated(HttpSessionEvent se) {
se.getSession().setMaxInactiveInterval(15 * 60); // 15 minutes
}
@Override
public void sessionDestroyed(HttpSessionEvent se) {
// Cleanup resources
}
}
Commentary:
Implementing a HttpSessionListener
allows you to specify session timeout directly as sessions are created. In this example, sessions expire after 15 minutes of inactivity.
4. Use SameSite Cookies
Why SameSite Attributes?
SameSite cookies help mitigate CSRF attacks by preventing browsers from sending cookies along with cross-site requests.
Implementation Example:
When creating your cookies, set the SameSite attribute appropriately:
import javax.servlet.http.Cookie;
public static void createSessionCookie(HttpServletResponse response, String sessionId) {
Cookie cookie = new Cookie("SESSIONID", sessionId);
cookie.setHttpOnly(true);
cookie.setSecure(true);
cookie.setPath("/");
cookie.setMaxAge(30 * 60);
cookie.setAttribute("SameSite", "Strict"); // CSRF Protection
response.addCookie(cookie);
}
Commentary:
- HttpOnly and Secure ensure that the cookie is not accessible via JavaScript and is transmitted over HTTPS only.
- The SameSite attribute adds an additional layer of protection against CSRF.
5. Monitor Session Activity
Why Monitoring?
Log and track user sessions to detect anomalies that may indicate session hijacking attempts.
Implementation Recommendation:
Implement logging within your session management strategy. Consider logging actions such as login attempts, session creation, and session termination.
public void logSessionActivity(String sessionId, String action) {
System.out.println("Session ID: " + sessionId + " performed action: " + action + " at " + LocalDateTime.now());
}
Commentary:
Logging session actions provides valuable insights for identifying unusual patterns and initiating timely responses to potential threats.
6. Educate Users on Security
Why User Education?
Users are often the weak link in security. Informing them about common security practices will empower them to help protect their accounts.
Strategies for User Education:
- Regularly update users on security best practices.
- Encourage the use of strong, unique passwords.
- Explain the importance of logging out after use, especially on shared devices.
Closing Remarks
Securing session management in Java web applications is critical in mitigating risks associated with session security flaws. By implementing secure session IDs, enforcing HTTPS, utilizing session timeouts, applying SameSite attributes to cookies, monitoring session activity, and educating users, you can significantly reduce vulnerabilities.
For more information on identifying potential security risks within session management, refer to the article "Is Your Login Safe? Unveiling Session Security Flaws," available at infinitejs.com/posts/login-safety-unveiling-session-security-flaws.
As developers, our responsibility is to create applications that are not only user-friendly but also secure. Prioritize session security as a foundational element of your web application development process, and empower your users to be part of the solution.
By staying informed and proactive, together we can combat session security risks effectively. Happy coding!