Common Pitfalls in Building NoSQL REST Apps with Hibernate OGM
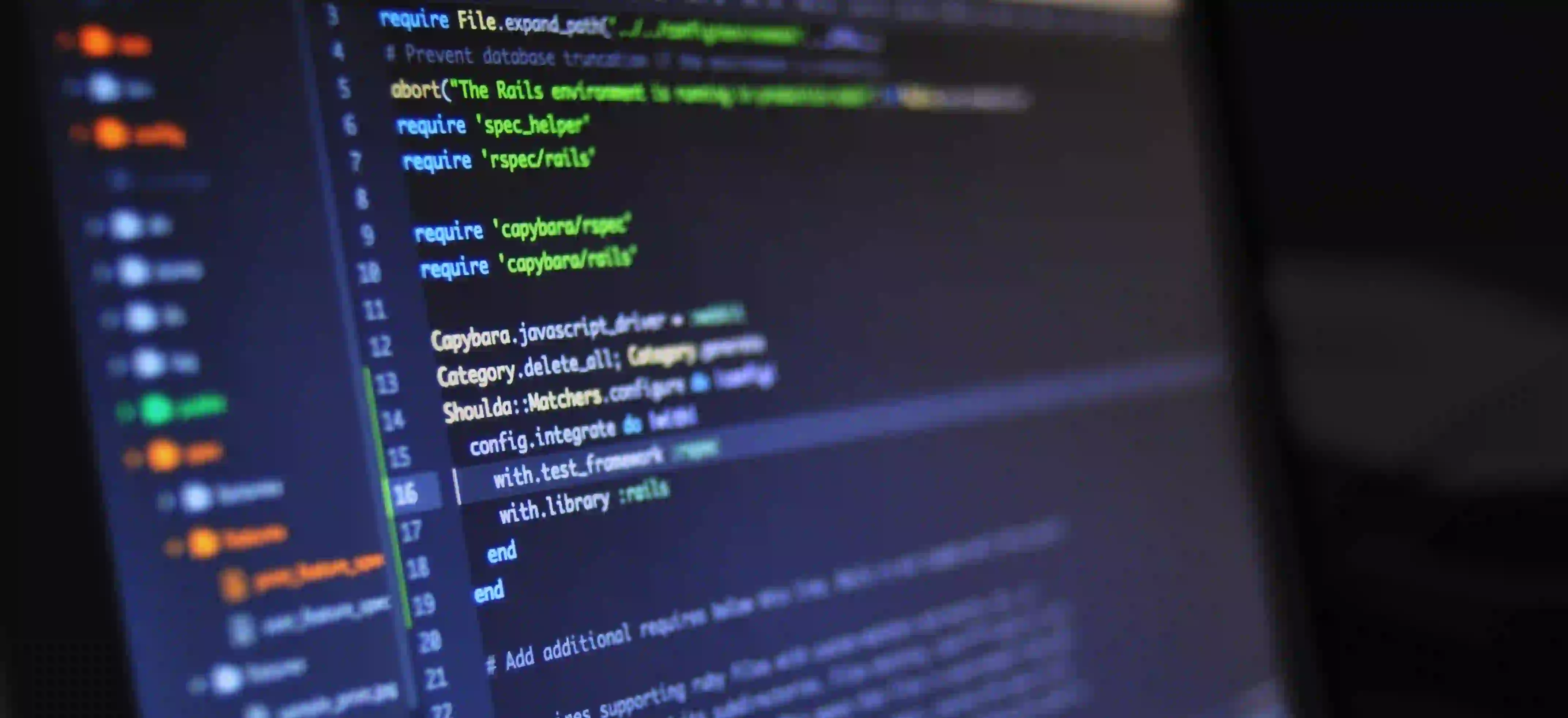
Common Pitfalls in Building NoSQL REST Apps with Hibernate OGM
Building modern web applications often involves the integration of various technologies. Among these, the combination of NoSQL databases and Java frameworks stands out due to its flexibility and scalability. In this blog post, we will explore the common pitfalls developers encounter when building NoSQL REST applications with Hibernate OGM (Object/Grid Mapper).
Hibernate OGM provides a way to interact with NoSQL databases through a similar programming approach as traditional Hibernate ORM, making it a popular choice for Java developers. However, it's essential to be aware of potential issues that can arise during development. Let’s dive into the key pitfalls and how to avoid them.
Understanding Hibernate OGM
Hibernate OGM is an extension of the well-known Hibernate ORM. It offers an API for working with NoSQL databases while leveraging the object-oriented paradigm that developers are already familiar with. This enables seamless integration of NoSQL databases, such as MongoDB, CouchDB, and others, into Java applications.
Before we explore the pitfalls, let's briefly outline the advantages of using Hibernate OGM:
- Consistency with JPA: Hibernate OGM uses Java Persistence API (JPA) annotations, making it easier for developers transitioning from relational databases.
- Flexible Schema: NoSQL databases are schema-less, allowing for easy alterations of the data model.
- Scalability: NoSQL databases often provide high scalability potential compared to traditional RDBMS.
These are critical benefits, but they also come with their own set of challenges.
Pitfall 1: Misunderstanding NoSQL Data Models
One of the most common mistakes developers make is mistakenly applying relational data models to NoSQL designs. NoSQL databases have different data structures, like key-value pairs, documents, and graphs, which call for different thinking.
Example:
@Entity
public class User {
@Id
private String id;
@Field
private List<Address> addresses;
// Getters and setters
}
In the above example, a User entity is defined with a list of addresses represented as separate embedded entities. If a developer tries to model this with strict relational thinking, they might end up creating unnecessary joins or complex structures, which defeats the purpose of working with NoSQL.
Why?
Understanding the actual benefits of NoSQL involves embracing its adaptability. By thinking in terms of documents rather than tables, developers can design schemas that better fit the use case.
Pitfall 2: Ignoring Performance Issues
Performance is a crucial aspect of any application. However, when using Hibernate OGM, developers sometimes overlook how queries translate into operations in the NoSQL store. ORM frameworks generally introduce a layer of abstraction that can lead to performance degradation if not handled correctly.
Example:
List<User> users = session.createQuery("FROM User", User.class).getResultList();
This line appears straightforward, but its execution can lead to fetching large datasets from the NoSQL store. Without proper pagination or filtering, the application may suffer from slow response times.
Why?
Always be mindful of how queries are constructed and executed. Using pagination and optimizing queries can lead to significant performance improvements.
Pitfall 3: Lack of Understanding of Transaction Management
In traditional RDBMS, developers rely on ACID properties to ensure consistent transactions. However, NoSQL databases often lean towards BASE (Basically Available, Soft state, Eventually consistent) properties, which introduces complexity in transaction handling.
Good Practice:
Utilizing the capability of transactions in databases like MongoDB is vital. Here's how you can initiate a transaction in Hibernate OGM:
Transaction transaction = session.getTransaction();
try {
transaction.begin();
session.persist(user);
session.persist(address);
transaction.commit();
} catch (Exception e) {
transaction.rollback();
}
Why?
It’s essential to have a solid understanding of the underlying database’s capabilities and limitations regarding transactions. This knowledge will better align your application design with the characteristics of NoSQL.
Pitfall 4: Not Using Correct Data Annotations
In Hibernate OGM, the use of JPA annotations is pivotal for mapping your objects to the NoSQL data structures correctly. Failing to use the right annotations can cause issues with data persistence and retrieval.
Example:
@Entity
public class User {
@Id
private String id;
@Column
private String name;
@Field
private Date registrationDate;
// Getters and setters
}
For example, forgetting to annotate a field with @Field
could mean that it is not included in the document structure in MongoDB, leading to runtime errors or data loss.
Why?
Cognizance of how annotations map to the NoSQL data model is vital for ensuring data integrity and application reliability.
Pitfall 5: Not Considering Data Access Patterns
Another common error arises when developers overlook the specific access patterns of their application. NoSQL databases are designed to excel in particular scenarios—like fast reads or writes—so aligning your data model with your access patterns is crucial.
Good Practice:
Suppose you have a read-heavy application; you should structure your data model to facilitate quick reads:
@Document
public class Article {
@Id
private String id;
@Field
private String title;
@Field
private String content;
@Field
private List<String> tags;
// Getters and setters
}
In this case, articles are easily indexed through tags, allowing for efficient retrieval based on user searches.
Why?
Understanding your application's behavior will guide how you structure your data. Designing with access patterns in mind results in optimal application performance.
Wrapping Up
Building NoSQL REST applications using Hibernate OGM can be an incredibly rewarding experience when done correctly. However, avoiding the common pitfalls discussed in this post—from understanding NoSQL data models to honing in on transaction management—is essential for success.
The key to success lies in diligent planning and understanding your chosen NoSQL database's unique features and behaviors. For further reading on best practices when working with NoSQL, you may find the following links useful:
- Hibernate OGM Documentation
- NoSQL Databases Overview
By embracing the advantages of NoSQL while avoiding these pitfalls, you can build scalable, efficient applications that meet the needs of modern users. Happy coding!