Streamline OSGi Bundles with a Common CXF Request Interceptor
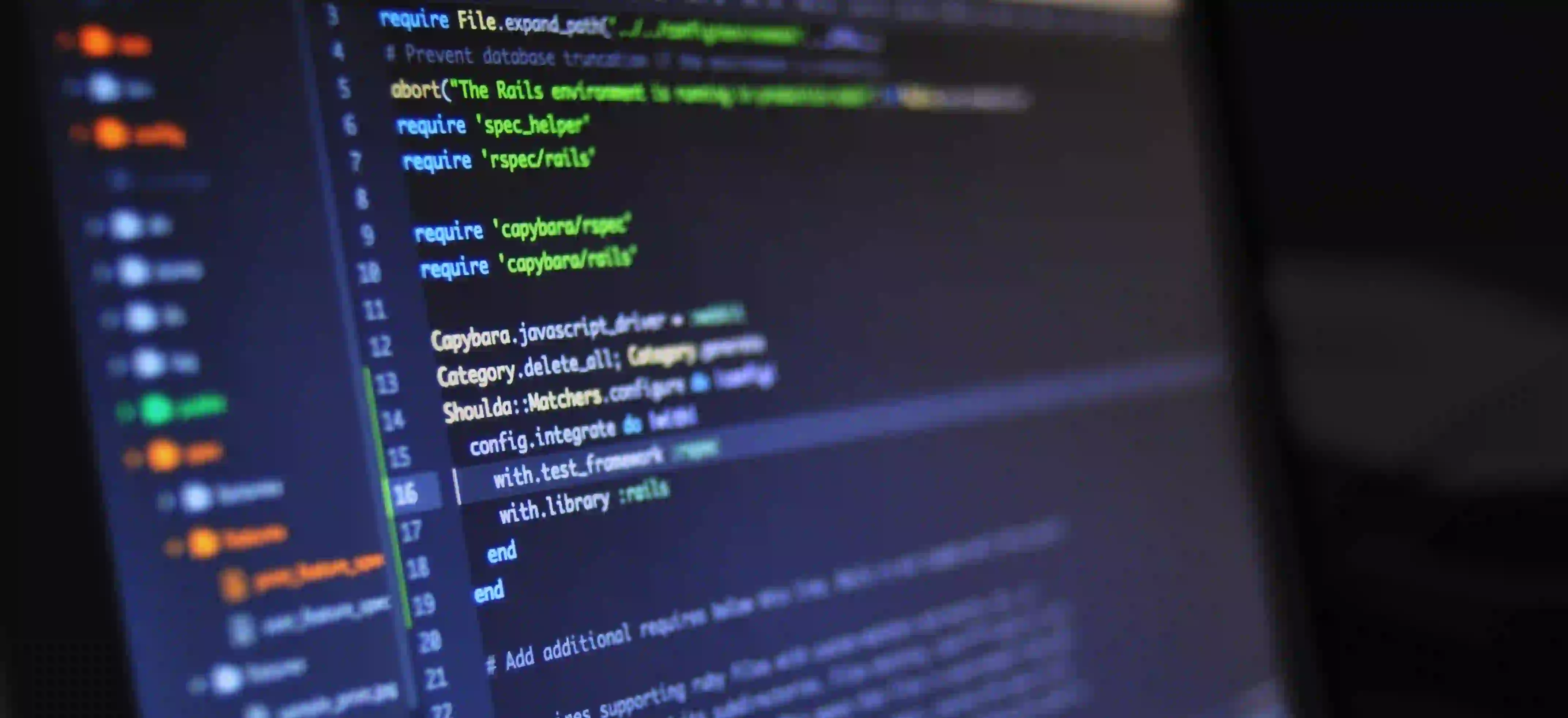
Streamline OSGi Bundles with a Common CXF Request Interceptor
In the rapidly evolving world of software architecture, efficient communication between components is paramount. Among the frameworks that facilitate such communication is Apache CXF, a popular choice for building web services. When combined with the OSGi (Open Service Gateway Initiative) framework, developers can create modular applications that are easier to manage and scale. However, redundant code often creeps in as you develop more bundles. This is where a common CXF request interceptor comes into play.
This blog post will guide you through the implementation of a common CXF request interceptor that can be shared across OSGi bundles, thereby streamlining your development process. We will cover the key concepts of OSGi and CXF, discuss what interceptors are, and eventually lead to an example of creating a simple interceptor.
Understanding OSGi and Apache CXF
What is OSGi?
OSGi is a framework that allows developers to create Java applications as a collection of loosely coupled modules, known as bundles. These bundles can be loaded and unloaded dynamically, making OSGi a powerful platform for modular application development.
For more in-depth knowledge about OSGi, check out the Official OSGi Specification.
What is Apache CXF?
Apache CXF is a robust framework for building RESTful and SOAP-based web services. It offers a plethora of features such as data binding, service hosting, and support for various protocols, which make it suitable for enterprise applications.
You can learn more about CXF from the Apache CXF Documentation.
The Role of Interceptors in CXF
Interceptors in Apache CXF serve as hooks that allow you to customize the way requests and responses are processed. They can access and modify messages, perform logging, handle security, and more. In our case, creating a common request interceptor can eliminate redundancy across multiple bundles.
Designing a Common CXF Request Interceptor
Creating a common CXF request interceptor involves several steps:
- Define the Interceptor: Implement the interface provided by Apache CXF for interceptors.
- Register the Interceptor as an OSGi Service: Make it available to other OSGi bundles.
- Consume the Interceptor in Your CXF Service: Ensure that it processes incoming requests before they reach the actual service logic.
Step 1: Implement the CXF Interceptor Interface
Here’s a basic code example that demonstrates how to implement a CXF interceptor:
import org.apache.cxf.interceptor.AbstractPhaseInterceptor;
import org.apache.cxf.interceptor.Fault;
import org.apache.cxf.message.Message;
import org.apache.cxf.phase.Phase;
public class CommonRequestInterceptor extends AbstractPhaseInterceptor<Message> {
public CommonRequestInterceptor() {
super(Phase.PRE_PROTOCOL); // Executed before the protocol layer
}
@Override
public void handleMessage(Message message) throws Fault {
// Log the incoming request
System.out.println("Received request: " + message.toString());
// You can add more logic here, e.g., validation, authentication, etc.
}
}
Explanation of the Code
- AbstractPhaseInterceptor: This class helps in creating interceptors that can act in various protocols. By extending this class, you automatically gain access to the interceptor lifecycle.
- Phase.PRE_PROTOCOL: This phase allows our interceptor to execute before the message is processed by the protocol layer. It is crucial for tasks such as logging and message modification.
- handleMessage Method: Here, we implement the core logic that processes incoming messages.
Step 2: Register the Interceptor as an OSGi Service
Now that we’ve defined the interceptor, we need to register it as an OSGi service. This enables other bundles to consume it easily.
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
@Override
public void start(BundleContext context) throws Exception {
CommonRequestInterceptor requestInterceptor = new CommonRequestInterceptor();
context.registerService(CommonRequestInterceptor.class.getName(), requestInterceptor, null);
}
@Override
public void stop(BundleContext context) throws Exception {
// Clean up resources if needed
}
}
Explanation of the Code
- BundleActivator: This interface is part of the OSGi framework. Implementing it allows us to control the lifecycle of our OSGi bundle.
- start Method: In this method, we create an instance of our interceptor and register it as a service in the OSGi container.
Step 3: Consume the Interceptor in Your CXF Service
Finally, we need to make sure our CXF service uses the registered interceptor. This typically happens in the service configuration.
Example configuration using Spring:
<jaxws:server id="myService" address="/myService">
<jaxws:service-class>com.example.MyService</jaxws:service-class>
<jaxws:outInterceptors>
<ref bean="commonRequestInterceptor"/>
</jaxws:outInterceptors>
</jaxws:server>
Explanation of the Code
In this XML configuration:
- jaxws:server: This element defines our web service.
- jaxws:outInterceptors: This allows us to add interceptors that will be invoked on the outgoing messages. We refer to our common request interceptor here.
Testing the Setup
Once your configuration is complete, deploy the OSGi bundles. To ensure that your interceptor works correctly, send a request to the web service and monitor the logs. You should see the output from your interceptor indicating that it has processed the request.
curl -X GET http://localhost:8080/myService
Lessons Learned
By implementing a common CXF request interceptor that can be shared across OSGi bundles, you streamline your service layer considerably. This approach not only reduces code redundancy but also centralizes your logic for logging, validation, or other cross-cutting concerns.
For further reading, consider checking out additional resources on:
- Apache CXF Interceptors
- OSGi Service Registry
With this foundation, you can enhance your OSGi bundles and improve the robustness of your CXF web services efficiently. Thank you for reading, and happy coding!