Wildcards: The Hidden Risks You Need to Know!
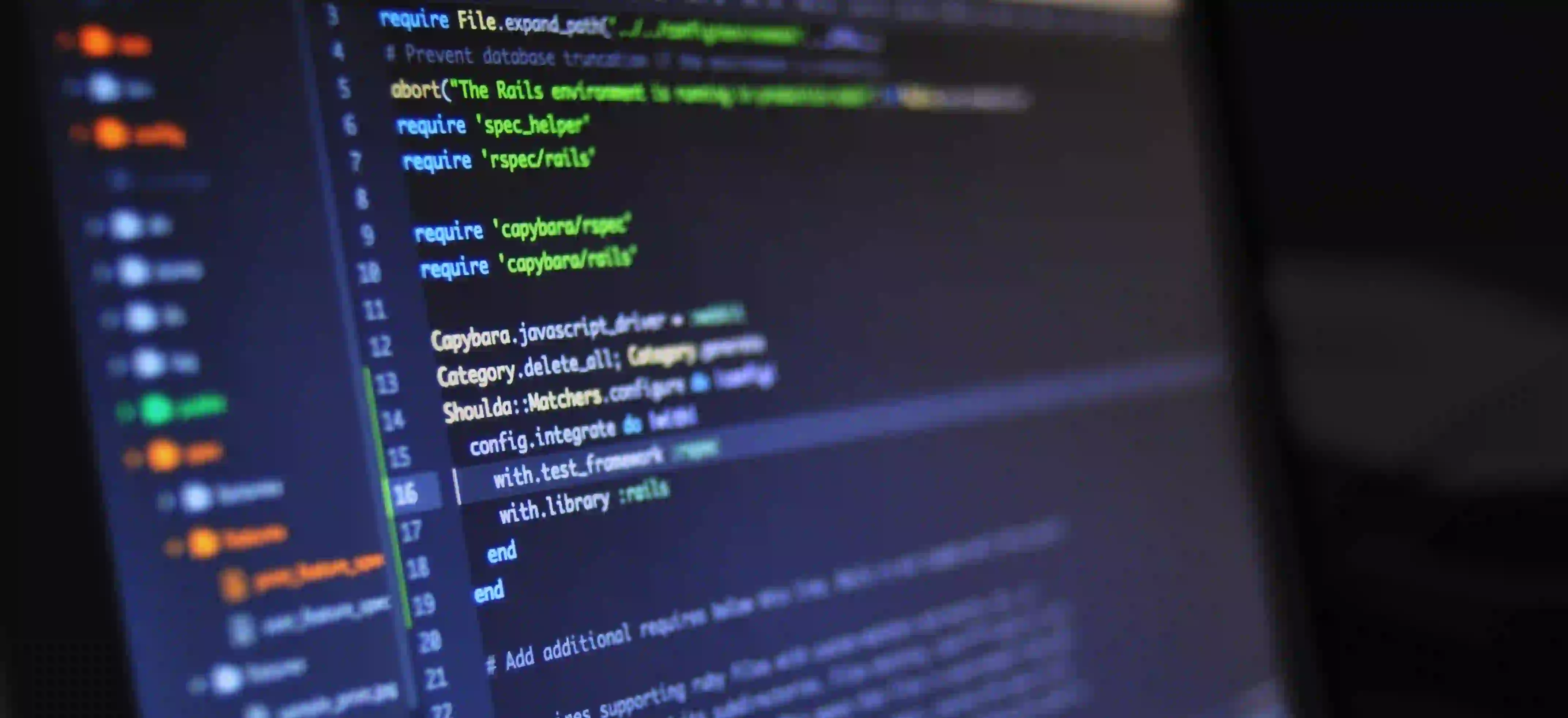
Wildcards: The Hidden Risks You Need to Know!
When it comes to programming and software development, certain features, while powerful, can introduce potential risks if not handled properly. One such feature is the use of wildcards. In this blog post, we'll explore what wildcards are, their applications, and the hidden risks associated with them, particularly in Java.
What Are Wildcards?
In Java, wildcards are a part of the generics feature, which allows developers to write flexible and reusable code. Wildcards enable you to work with unknown types, making your code more versatile while providing type safety. The primary wildcard types in Java generics are:
- Unbounded Wildcard (
<?>
): Represents an unknown type. - Upper Bounded Wildcard (
<? extends T>
): Represents any type that is a subtype of T. - Lower Bounded Wildcard (
<? super T>
): Represents any type that is a supertype of T.
Why Use Wildcards?
The primary advantage of using wildcards in Java is flexibility. They allow methods to accept a wider variety of arguments. For instance, if you want to write a method that can operate on lists of various types, wildcards enable that without compromising type safety.
Here's a simple example:
public static void printList(List<?> list) {
for (Object element : list) {
System.out.println(element);
}
}
In the above code, printList
can accept a List
of any type. This offers great flexibility in how the method can be used.
The Hidden Risks of Using Wildcards
While wildcards can make your code more flexible and reusable, they come with certain risks that developers must be aware of. Below, let's delve into some of the common pitfalls:
1. Type Safety Issues
One of the main advantages of Java's generics is type safety. However, wildcards can sometimes undermine this principle. For example, consider the following case:
List<? extends Number> list = new ArrayList<Integer>();
list.add(3.14); // Compiler Error
In this situation, you cannot add a Double
to a List<? extends Number>
, even though it seems like it should work. The Java Compiler prevents this to maintain type safety.
Why This Matters
If the compiler allows you to add objects of incompatible types, it could lead to runtime exceptions which are far more challenging to debug.
2. Insufficient Readability and Maintainability
When wildcards are overused, they can lead to code that is difficult to read and maintain. Developers unfamiliar with a codebase might find it challenging to understand the intended types being used. Keeping the code simple and straightforward should always be a priority.
3. Performance Overheads
Wildcards can introduce minor performance overheads. This is because Java may need to perform extra type checks or boxing/unboxing when working with wildcards, especially in a large-scale application where performance is a key consideration.
Employing Generics Wisely
To mitigate some of the risks associated with wildcards, it's essential to employ generics wisely. Focus on clarity and explicitness in your type declarations.
Example of Good Practice:
public static <T> void addToList(List<T> list, T item) {
list.add(item);
}
In this case, addToList
accepts any list type but ensures that only items of the same type can be added. This promotes safer and more readable code.
Additional Resources
For more information about wildcards and generics in Java, check out the following resources:
- Java Generics - Oracle's Official Documentation
- Understanding Wildcards in Generics
How to Address and Mitigate Risks
While risks are inherent, they can be managed effectively. Keeping the following practices in mind can lead to more reliable usage of wildcards:
-
Limit the Scope: Use wildcards only where necessary. Overuse can complicate code without providing significant benefits.
-
Verbose Type Declarations: Make your type requirements explicit. Avoid ambiguous wildcard usage when possible.
-
Code Review Practices: Encourage peer reviews focusing on the use of generics. This can introduce multiple perspectives to catch potential issues early.
-
Test Thoroughly: The more you test, the better your confidence in your code. Use automated tests to ensure that your code behaves as expected when using wildcards.
Wrapping Up
Wildcards in Java offer incredible flexibility but come with hidden risks that can compromise type safety and code maintainability. By understanding these risks and practicing sound coding standards, you can leverage wildcards effectively without falling into common traps.
Always remember: with great power comes great responsibility. By being mindful of how you utilize wildcards, you will write cleaner, safer, and more maintainable code.
Happy coding!