Why Ignoring Value Types Can Sabotage Your Code Quality
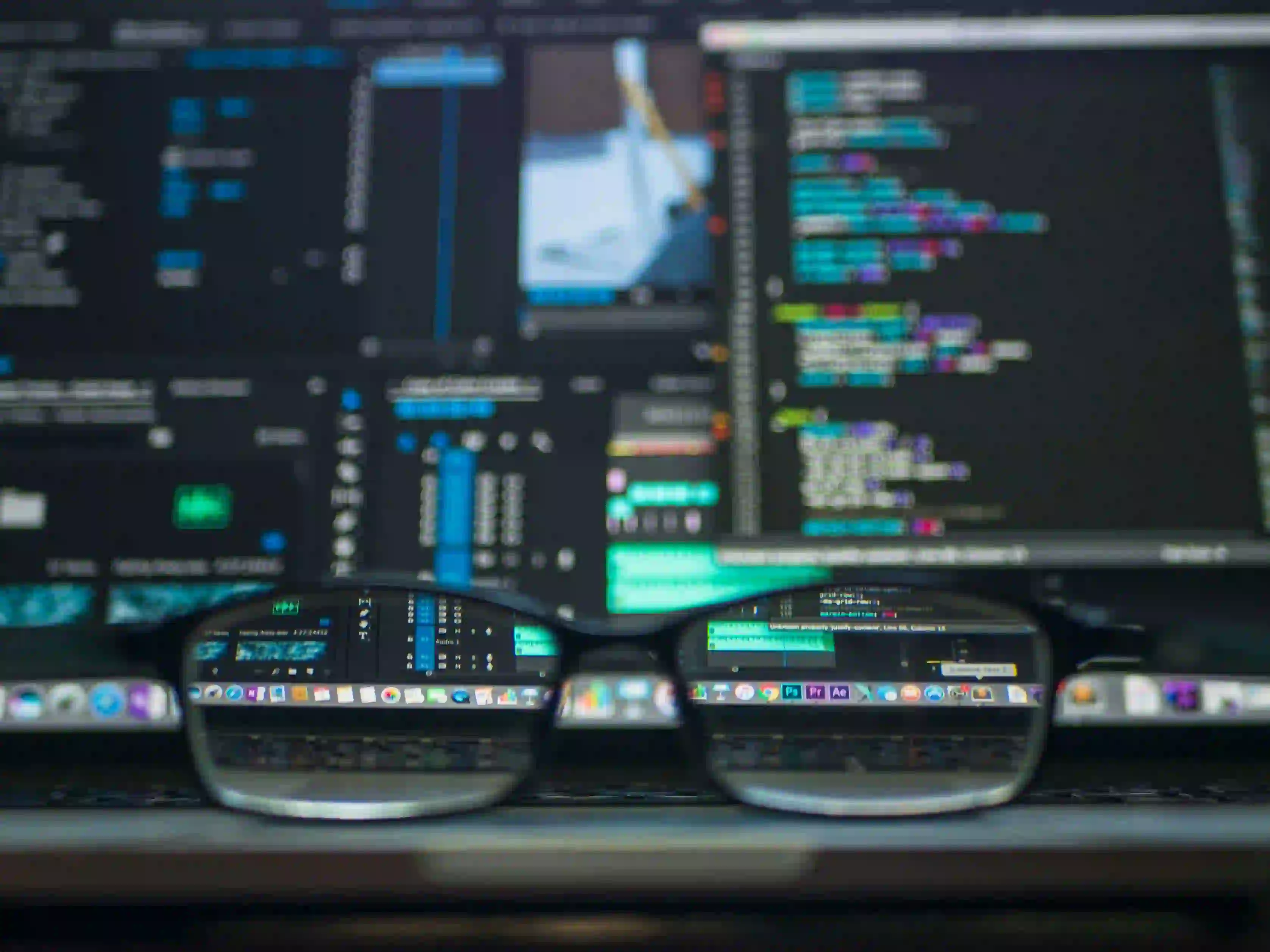
Why Ignoring Value Types Can Sabotage Your Code Quality
Java is a statically typed language, and understanding its type system is foundational for writing high-quality code. Among its various types, value types hold a prominent place. Ignoring value types often leads to subtle bugs, performance issues, and code that is difficult to maintain. In this blog post, we will explore what value types are, their importance in Java programming, and why neglecting them can be detrimental to your projects.
What Are Value Types?
In Java, data types can be categorized as either primitive types or reference types. Primitive types include int
, char
, boolean
, and others. Reference types include objects created from classes and arrays. Each of these types serves a different purpose, but when we discuss value types, we primarily refer to the primitive types.
Understanding Primitive Types
Primitive types are fundamental building blocks of data manipulation in Java. They have a fixed size and directly store their own values. Here’s a quick summary of commonly used primitive types in Java:
| Type | Size (in bytes) | Default Value |
|---------|------------------|---------------|
| byte
| 1 | 0 |
| short
| 2 | 0 |
| int
| 4 | 0 |
| long
| 8 | 0L |
| float
| 4 | 0.0f |
| double
| 8 | 0.0d |
| char
| 2 | '\u0000' |
| boolean
| 1 bit | false |
The Importance of Value Types
-
Performance: Primitive types are more efficient when memory allocation and access speed are considered. Being stack-allocated, primitive types are usually faster compared to reference types, which may involve additional memory overhead.
-
Simplicity: Value types allow for straightforward operations without needing to create object instances. This reduces the boilerplate code that typically accompanies reference types.
-
Avoid Null Pointers: Values of primitive types inherently cannot be null, reducing the likelihood of
NullPointerException
errors. This makes your code cleaner and easier to debug.
Here’s an example to illustrate their performance benefits:
public class ValueTypeExample {
public static void main(String[] args) {
int result = 0; // Primitive type: int initialized to 0
for (int i = 0; i < 1000000; i++) {
result += i; // simple arithmetic operation
}
System.out.println("Sum: " + result);
}
}
This code efficiently calculates the sum of integers from 0 to 999,999 using the primitive int
. The speed of this operation is due to direct value manipulation and stack allocation.
The Risks of Ignoring Value Types
1. Performance Penalties
When developers ignore value types in favor of reference types (like Integer
for int
), they may inadvertently introduce a performance bottleneck. For example, consider this code snippet:
public class PerformanceIssue {
public static void main(String[] args) {
Integer total = 0; // Reference type: Integer
for (int i = 0; i < 1000000; i++) {
total += i; // Autoboxing conversion occurs here
}
System.out.println("Total: " + total);
}
}
In this example, each addition requires the creation of a new Integer
object due to autoboxing. This overhead can lead to increased CPU and memory usage compared to using an int
.
2. Increased Complexity and Bugs
Using reference types often introduces complexity. If you're not careful, it's easy to get unexpected null
values, leading to potential bugs that are challenging to trace. This can make your code not only less robust but harder to read and maintain.
For example:
public class NullPointerExample {
public static void main(String[] args) {
Integer num = null; // Potentially problematic if not checked
System.out.println("Number: " + num.toString()); // Throws NullPointerException
}
}
In this case, dereferencing a null value leads to an error, harming program stability. Relying on primitive types can prevent this.
3. Code Readability and Maintainability
Value types, being simple and straightforward, enhance code readability. When you stay clear of unnecessary complexities, your code is easier for others (and your future self) to comprehend.
Consider this contrasting example:
import java.util.List;
import java.util.ArrayList;
public class MaintainableCode {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>(); // Reference type
for (int i = 0; i < 10; i++) {
numbers.add(i); // Addition involves boxing
}
for (Integer number : numbers) {
System.out.print(number + " ");
}
}
}
Using a list of Integer
may seem convenient, but it can lead to code that is less clear about its intent. Utilizing an array of primitive int
can often avoid confusion inherent in the use of references:
public class AlternativeMaintainability {
public static void main(String[] args) {
int[] numbers = new int[10]; // Array of primitive
for (int i = 0; i < 10; i++) {
numbers[i] = i; // Direct assignment
}
for (int number : numbers) {
System.out.print(number + " ");
}
}
}
The intent and purpose of the latter code are clearer and more direct.
Final Thoughts
Ignoring value types in Java can have detrimental effects on your code quality. From performance and memory consumption to enhancing readability and avoiding bugs, the advantages of using primitive types can be significant. While reference types have their place—particularly when you require the functionality provided by classes—the proper use of primitive types should be a fundamental skill for every Java developer.
Understanding the strengths and limitations of both primitive and reference types, and opting to use them judiciously, is vital for writing high-quality, maintainable Java code.
For more information on Java data types, visit Oracle’s Java Documentation. Additionally, if you're keen to learn more about performance optimization in Java, check out Baeldung's guide.
By heeding the lesson of value types and embracing their use where applicable, you will set yourself up for success in your Java programming journey. Happy coding!