Common Java Serialization Pitfalls to Avoid
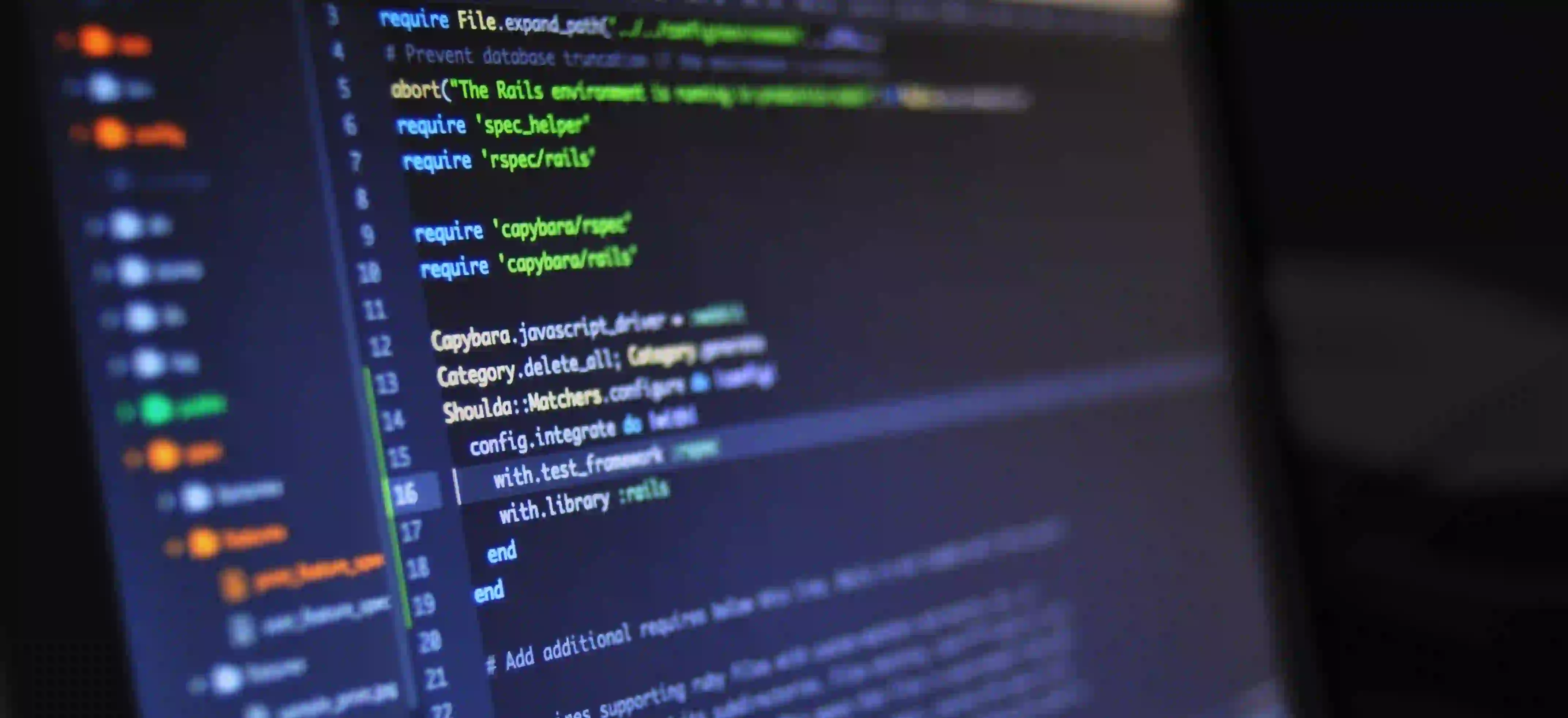
Common Java Serialization Pitfalls to Avoid
Serialization in Java is a powerful mechanism that allows you to convert an object into a byte stream for storage or transmission. While it provides incredible utility, several pitfalls can inflict chaos if not managed properly. In this blog post, we'll delve into common serialization pitfalls while highlighting strategies to avoid them.
What is Java Serialization?
Serialization is the process of converting an object into a format that can be easily stored or transmitted and then reconstructed later. In Java, this is typically done using the Serializable
interface.
import java.io.Serializable;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private String email;
// Constructor, getters, and setters...
}
The serialVersionUID
serves as a version control mechanism. It helps ensure that during serialization and deserialization, both sides are using compatible classes. If they aren't, you'll face an InvalidClassException
.
Why Use serialVersionUID
?
Using a serialVersionUID
is crucial:
- Control Versioning: Changes in your class structure can lead to deserialization issues.
- Readability: It documents the version of your classes.
- Avoiding Runtime Errors: It prevents hard-to-diagnose incompatibility issues when classes are altered.
For more on the Serializable
interface, check the official Java documentation.
Common Pitfall 1: Not Defining serialVersionUID
Failing to define a serialVersionUID
can lead to unexpected issues, especially as your application evolves. By default, Java creates a serialVersionUID
based on the class structure, which could lead to mismatches when you alter the class.
Solution
Always explicitly declare your serialVersionUID
, as shown above. This act of caution can prevent many serialization headaches.
Common Pitfall 2: Transient Fields
Not all fields in a class need to be serialized. Java provides the transient
keyword to exclude fields from serialization. If you fail to mark sensitive or unnecessary fields as transient
, you may unintentionally serialize them.
Example
public class Session implements Serializable {
private static final long serialVersionUID = 1L;
private String sessionId;
private transient String password;
// Constructor, getters, and setters...
}
In this example, the password
field will not be serialized, ensuring sensitive information remains protected.
Why Mark Fields as transient
?
- Security: It prevents sensitive data from being compromised.
- Efficiency: Reduces size of the serialized object.
Common Pitfall 3: Inherited Classes and Interfaces
When working with inheritance, it's common to forget whether the superclass is Serializable
. If a superclass is not serializable, it can lead to serialization failure, resulting in an NotSerializableException
.
Solution
Make sure all classes in the hierarchy implement Serializable
, or use the transient
keyword for non-serializable parts.
Example
import java.io.Serializable;
class Animal implements Serializable {
private static final long serialVersionUID = 1L;
String type;
// Constructor, getters, and setters...
}
public class Dog extends Animal {
private String breed; // This is just serialized with Dog instance.
// Constructor, getters, and setters...
}
Here, both Animal
and Dog
are serializable, preserving the object structure during serialization.
Common Pitfall 4: Circular References
Java’s serialization handles circular references gracefully, but they can lead to performance issues or stack overflows if mismanaged.
Solution
Be cautious with circular references by ensuring that the objects are as simple as necessary. Such complexity can lead to bloated serialized forms.
Example
import java.io.Serializable;
class Parent implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private Child child;
// Constructor, getters, and setters...
}
class Child implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private Parent parent; // Circular reference
// Constructor, getters, and setters...
}
While valid, this structure can lead to complications in large applications. Always evaluate the necessity of such design choices.
Common Pitfall 5: Deserialization Vulnerabilities
One of the most significant risks of serialization is introducing vulnerabilities, especially if untrusted input is deserialized. Attackers can craft malicious serialized objects.
Solution
- Validate Input: Always validate serialized data before deserialization.
- Use Safe Libraries: Prefer libraries like Kryo or Protostuff for safer serialization processes.
Example of Input Validation
import java.io.*;
public class SafeDeserialization {
public static User safeDeserialize(byte[] data) throws IllegalArgumentException {
try (ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(data))) {
User user = (User) ois.readObject();
// Validate user fields
if(user.getEmail().equals("unsafe@example.com")) {
throw new IllegalArgumentException("Unsafe email detected!");
}
return user;
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
return null;
}
}
}
In this example, we check the email before proceeding with the deserialized User
object, helping mitigate potential risks.
Lessons Learned
Serialization is a useful tool in Java development, but pitfalls are lurking if you aren't careful. Remember to define serialVersionUID
, properly use the transient
keyword, be aware of class hierarchies, manage circular references wisely, and always validate your input.
By following these guidelines, you protect your application from subtle bugs and potential security vulnerabilities. For an in-depth understanding, refer to the Java Serialization tutorial on Baeldung.
Happy coding!