Common Mistakes in Requirements Gathering You Must Avoid
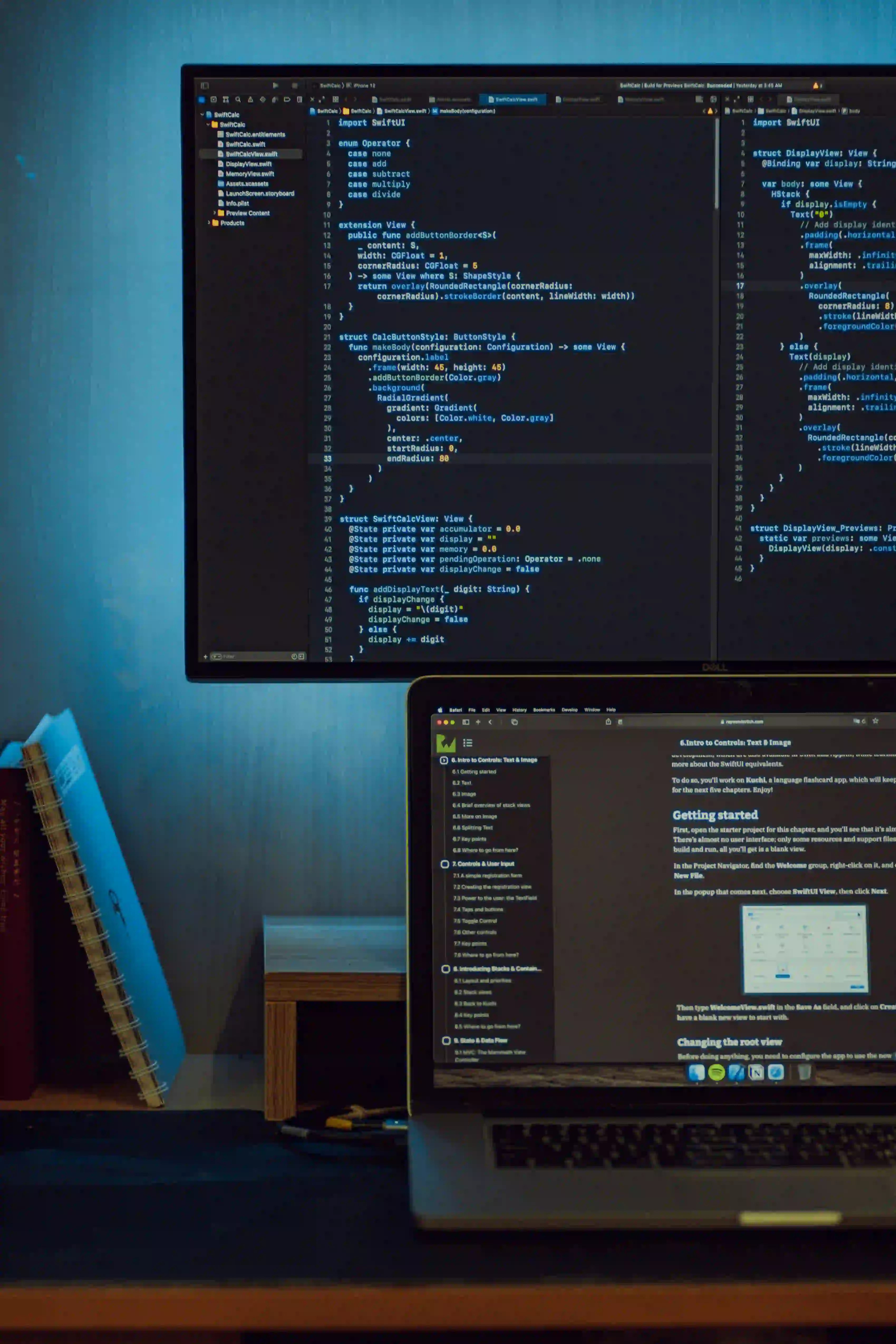
Common Mistakes in Requirements Gathering You Must Avoid
Gathering requirements is one of the most critical phases in the software development lifecycle. It sets the foundation for the entire project, impacting everything from design to deployment. However, this process is often riddled with pitfalls. In this blog post, we’ll explore the common mistakes in requirements gathering and provide insights on how to steer clear of them.
1. Skipping Stakeholder Involvement
Why It’s a Mistake: One of the biggest oversights is not involving all relevant stakeholders. This can include end-users, project sponsors, and team members. When various voices are absent from the conversation, you risk developing a product that does not meet its intended purpose.
Solution: Schedule meetings and facilitate discussions with all key stakeholders. Utilize methods like brainstorming sessions or interviews to gauge expectations and priorities. This collaborative effort will ensure that diverse perspectives are incorporated into the requirements.
import java.util.List;
public class StakeholderMeeting {
public void initiateMeeting(List<String> stakeholders) {
// Simulate meeting initiation
for (String stakeholder : stakeholders) {
System.out.println("Meeting initiated with: " + stakeholder);
}
}
}
// Usage
List<String> stakeholdersList = List.of("Product Manager", "End User", "Developer Lead");
new StakeholderMeeting().initiateMeeting(stakeholdersList);
Commentary:
In the code snippet above, we create a simple method that automates the initiation of stakeholder meetings. This emphasizes the importance of including diverse participants and ensures that no voice is left unheard.
2. Failing to Document Requirements Properly
Why It’s a Mistake: Poorly documented requirements can lead to significant misunderstandings down the line. Ambiguities can cause conflicts and misalignments between team members, leading to a product that fails to meet user expectations.
Solution: Employ standardized documentation techniques. Use templates or requirement management tools to keep everything organized. Maintain a version history for your requirements to track any changes or updates.
import java.util.ArrayList;
import java.util.List;
public class RequirementsDocumentation {
private List<String> requirementsList = new ArrayList<>();
public void addRequirement(String requirement) {
requirementsList.add(requirement);
System.out.println("Requirement added: " + requirement);
}
public void printRequirements() {
System.out.println("Documented Requirements:");
for (String req : requirementsList) {
System.out.println("- " + req);
}
}
}
// Usage
RequirementsDocumentation doc = new RequirementsDocumentation();
doc.addRequirement("User login must be secure.");
doc.addRequirement("System should support multiple languages.");
doc.printRequirements();
Commentary:
This code provides an example of a basic requirements documentation tool. It allows for easy addition and retrieval of requirements, emphasizing the necessity for clear documentation in the requirements gathering process.
3. Ignoring User Experiences and Pain Points
Why It’s a Mistake: Developers often focus on technical requirements without understanding user experiences. This lack of focus can lead to features that do not adequately address real user pain points.
Solution: Engage in user research. Conduct surveys, interviews, or usability tests to gather insights on users' challenges. Understanding their needs will help shape a product that genuinely solves problems.
4. Not Prioritizing Requirements
Why It’s a Mistake: Every requirement may seem essential, but not all are created equal. Failing to prioritize them can lead to scope creep and delays during development.
Solution: Implement prioritization techniques such as MoSCoW (Must have, Should have, Could have, Won't have). This approach allows teams to focus on the most critical requirements first.
5. Overlooking Non-Functional Requirements
Why It’s a Mistake: Non-functional requirements, like performance, security, and scalability, are often neglected in favor of functional requirements. Ignoring these can jeopardize the system's effectiveness and user satisfaction.
Solution: Ensure that your requirements gathering process encompasses both functional and non-functional aspects. Create a checklist to maintain a balanced approach.
6. Using Ambiguous Language
Why It’s a Mistake: Using vague terms can lead to various interpretations among different stakeholders. This confusion creates a gap between what developers think they should build and what the users actually need.
Solution: Be specific in your language. This includes defining any terms or jargon that may not be universally understood.
Example of Specific vs. Ambiguous Requirement:
// Ambiguous requirement
The system should be fast.
// Specific requirement
The system should load the user dashboard in under 2 seconds on a standard broadband connection.
Commentary:
Being specific eliminates ambiguity. The clearer your requirements, the less room for interpretation, ensuring that the end product aligns with user needs and expectations.
7. Neglecting to Review and Validate Requirements
Why It’s a Mistake: Requirements can evolve over time. Failing to validate them periodically leads to the development of features that may have become irrelevant or less important.
Solution: Set a schedule for regular reviews of requirements with your stakeholders. This iterative approach allows you to adapt to any changes in user needs or business objectives.
Closing Remarks
Effective requirements gathering is a cornerstone of successful software projects. By avoiding common mistakes such as neglecting stakeholder involvement, poorly documenting requirements, ignoring user pain points, and using ambiguous language, you lay the groundwork for a more streamlined development process.
Engagement, clarity, and regular review are vital elements in this phase. Remember, the value of robust requirements cannot be overstated; they not only dictate the project's direction but also enhance the final product's quality.
For more information on this topic, you can check 10 Common Mistakes in Software Requirements Gathering and Understanding the Importance of Requirements Gathering, where you will find additional tips and detailed perspectives.
By taking careful measures against these pitfalls, you not only improve the requirements gathering process but also increase the likelihood of creating a successful product that meets both user and business needs.