5 Common Pitfalls That Sabotage Developer Performance
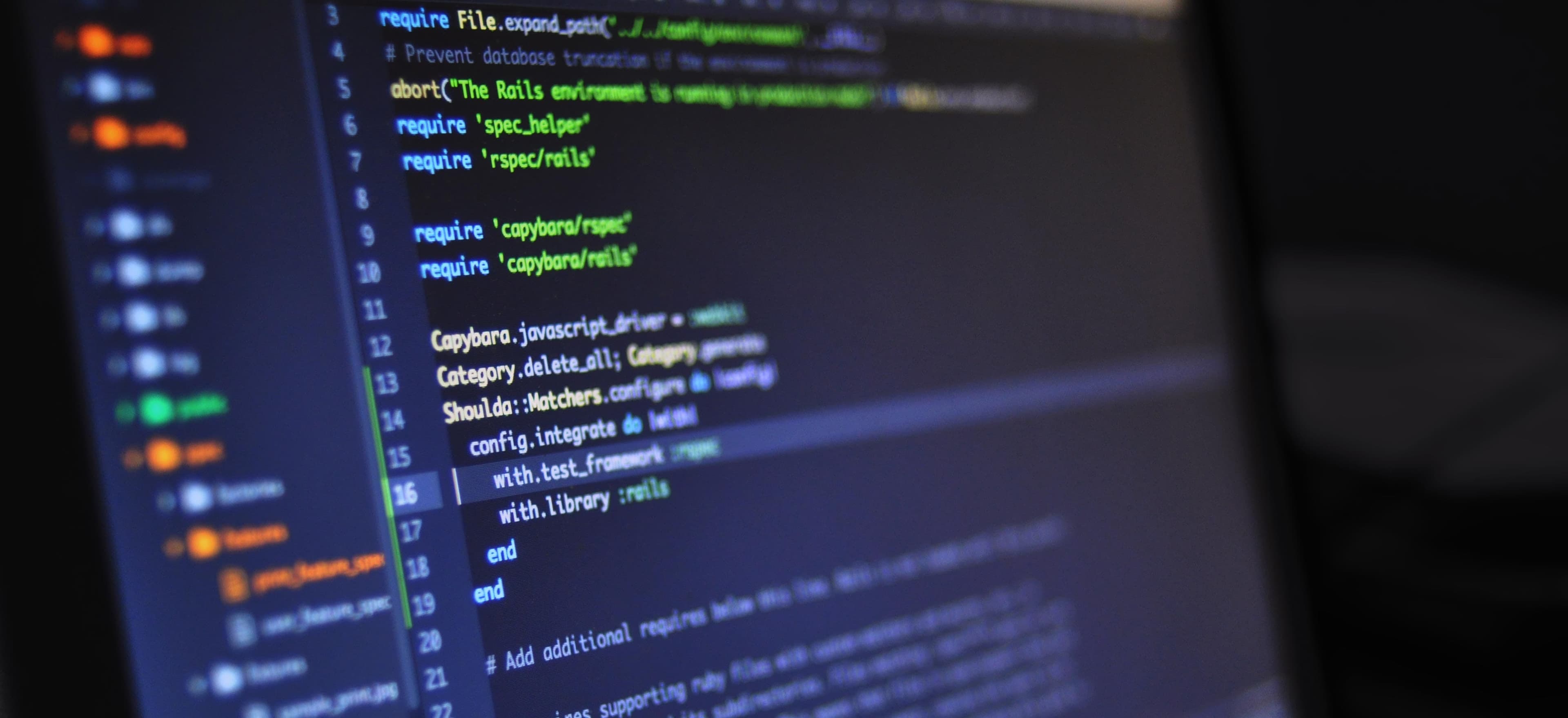
- Published on
5 Common Pitfalls That Sabotage Developer Performance
In the ever-evolving world of software development, developers often face numerous challenges that can hinder their productivity and performance. While coding is at the forefront of their responsibilities, various external and internal factors can play significant roles in shaping their work quality and efficiency.
In this blog post, we’ll discuss five common pitfalls that can sabotage developer performance and provide actionable insights to help you navigate these issues effectively.
1. Poor Time Management
Why It Matters
Good time management allows developers to plan their tasks effectively, ensuring they meet deadlines without compromising the quality of their code. Developers often juggle multiple tasks such as coding, debugging, and attending meetings, making time management crucial.
Common Issues
- Overcommitting: Developers may frequently take on too many tasks, leading to burnout.
- Distractions: Frequent interruptions can distort a developer's focus.
Solutions
- Prioritization Techniques: Use methods like the Eisenhower Matrix, which helps you distinguish between urgent and important tasks.
import java.util.*;
public class Task {
String name;
boolean isUrgent;
boolean isImportant;
public Task(String name, boolean isUrgent, boolean isImportant) {
this.name = name;
this.isUrgent = isUrgent;
this.isImportant = isImportant;
}
// Getter methods
public String getName() {
return name;
}
public boolean isUrgent() {
return isUrgent;
}
public boolean isImportant() {
return isImportant;
}
}
class TaskManager {
List<Task> tasks = new ArrayList<>();
public void addTask(Task task) {
tasks.add(task);
}
public void prioritizeTasks() {
Collections.sort(tasks, new Comparator<Task>() {
@Override
public int compare(Task t1, Task t2) {
if (t1.isUrgent() && t2.isUrgent()) {
return 0; // Both are urgent
} else if (t1.isUrgent()) {
return -1; // t1 is more significant
} else {
return 1; // t2 is more significant
}
}
});
}
public void displayTasks() {
for (Task task : tasks) {
System.out.println(task.getName());
}
}
}
In the above Java snippet, we define a Task
class to manage tasks with urgency and importance flags. The TaskManager
class sorts these tasks, emphasizing the need to address urgent and important ones first.
Wrapping Up
Investing time in effective task management tools and techniques can lead to noticeable improvements in performance and output quality.
2. Lack of Knowledge and Skills
Why It Matters
Technology areas shift rapidly, and staying updated is key for developers. Skill gaps can result in inefficient coding practices, leading to bugs and poorly structured code.
Common Issues
- Falling behind on new technologies: Developers may not be familiar with the latest tools or frameworks.
- Neglecting best practices: Without a commitment to ongoing learning, developers can produce less maintainable code.
Solutions
- Regular Training: Allocate time each week for learning new programming languages, techniques, or frameworks relevant to your work.
- Mentorship Programs: Having a mentor can help bridge the knowledge gap.
Wrapping Up
Investing in continuous learning not only improves individual performance but also enhances team capabilities, leading to better software products.
3. Lack of Collaboration
Why It Matters
In today's Agile environment, teamwork is essential. Developers often work within cross-functional teams, making collaboration critical for delivering projects on time.
Common Issues
- Siloed Work: When developers work in isolation, communication breakdowns occur.
- Core Information Not Shared: Developers may miss essential updates or context by not collaborating effectively.
Solutions
- Daily Standups: Encourage short meetings to discuss progress, identify blockers, and share information.
- Code Reviews: Promote joint code review sessions to foster knowledge sharing and improve code quality.
// Imagine this as a typical code review template
public class ReviewFeedback {
public static void checkCodeStyle(String code) {
if (code.length() < 5) {
System.out.println("Code snippet is too short.");
} else {
System.out.println("Looks good!");
}
}
}
In this simple Java method, we are mocking a code review feedback system. It highlights the role of communal knowledge in enhancing code quality while showing developers are engaged in the review process.
Wrapping Up
Fostering collaboration not only builds trust but also results in a unified vision for project success.
4. Inadequate Tools and Resources
Why It Matters
The right tools can significantly enhance productivity and streamline workflows. Using outdated tools or inefficient workflows can create unnecessary obstacles.
Common Issues
- Obsolete IDEs or Libraries: Developers may struggle with bugs that newer tools could have resolved.
- Poorly Configured Development Environments: Inefficient setups waste precious development time.
Solutions
- Evaluate Tools Regularly: Periodically assess whether the tools you are using meet your needs.
// Pseudo code for evaluating tools
public class ToolsEvaluation {
public static void evaluate(String toolName, String feature) {
List<String> features = Arrays.asList("debugging", "integration", "UI", "performance");
if (features.contains(feature)) {
System.out.println(toolName + " supports " + feature);
} else {
System.out.println(toolName + " lacks " + feature);
}
}
}
This simple evaluation method in Java helps highlight whether existing tools meet current feature requirements, encouraging developers to adapt to newer, more efficient alternatives.
Wrapping Up
Continuously upgrading your tools and resources not only boosts individual performance but also creates a more efficient project environment.
5. Ineffective Feedback Mechanisms
Why It Matters
Regular feedback is an essential component of growth and improvement. Without constructive feedback, developers remain unaware of their weaknesses or areas that require enhancement.
Common Issues
- Lack of Regular Check-ins: Developers may go long periods without receiving feedback on their work.
- Unclear Performance Metrics: Inconsistent evaluation criteria can lead to confusion.
Solutions
- Establish Feedback Loops: Incorporate a feedback process into development cycles, such as Agile sprints or retrospectives.
- Set Clear Metrics: Define success metrics that are transparent and attainable.
// Sample code to represent setting a development metric
public class FeedbackMetrics {
private int linesOf codeWritten;
private int bugsFixed;
public FeedbackMetrics(int lines, int bugs) {
this.linesOfCodeWritten = lines;
this.bugsFixed = bugs;
}
public void displayMetrics() {
System.out.println("Lines of code written: " + linesOfCodeWritten);
System.out.println("Bugs fixed: " + bugsFixed);
}
}
This basic structure illustrates a way to encapsulate key metrics that developers can track over time, offering clarity and direction for performance evaluations.
Wrapping Up
Implementing strong feedback mechanisms not only guides developers but also strengthens team dynamics, creating a culture of growth and improvement.
Wrapping Up
Navigating the challenges of a developer's role requires more than just coding expertise. Time management, continuous learning, collaboration, proper tools, and effective feedback are all vital components that can either hinder or enhance developer performance.
By acknowledging and addressing these common pitfalls, developers can better position themselves for success, optimized productivity, and ultimately, superior software quality. Embrace these strategies to cultivate an environment that fosters development excellence.
For further information on enhancing developer performance, check out Software Engineering Daily and Dev.to for valuable community insights and professional articles.