Troubleshooting WebLogic 12c Setup with NetBeans
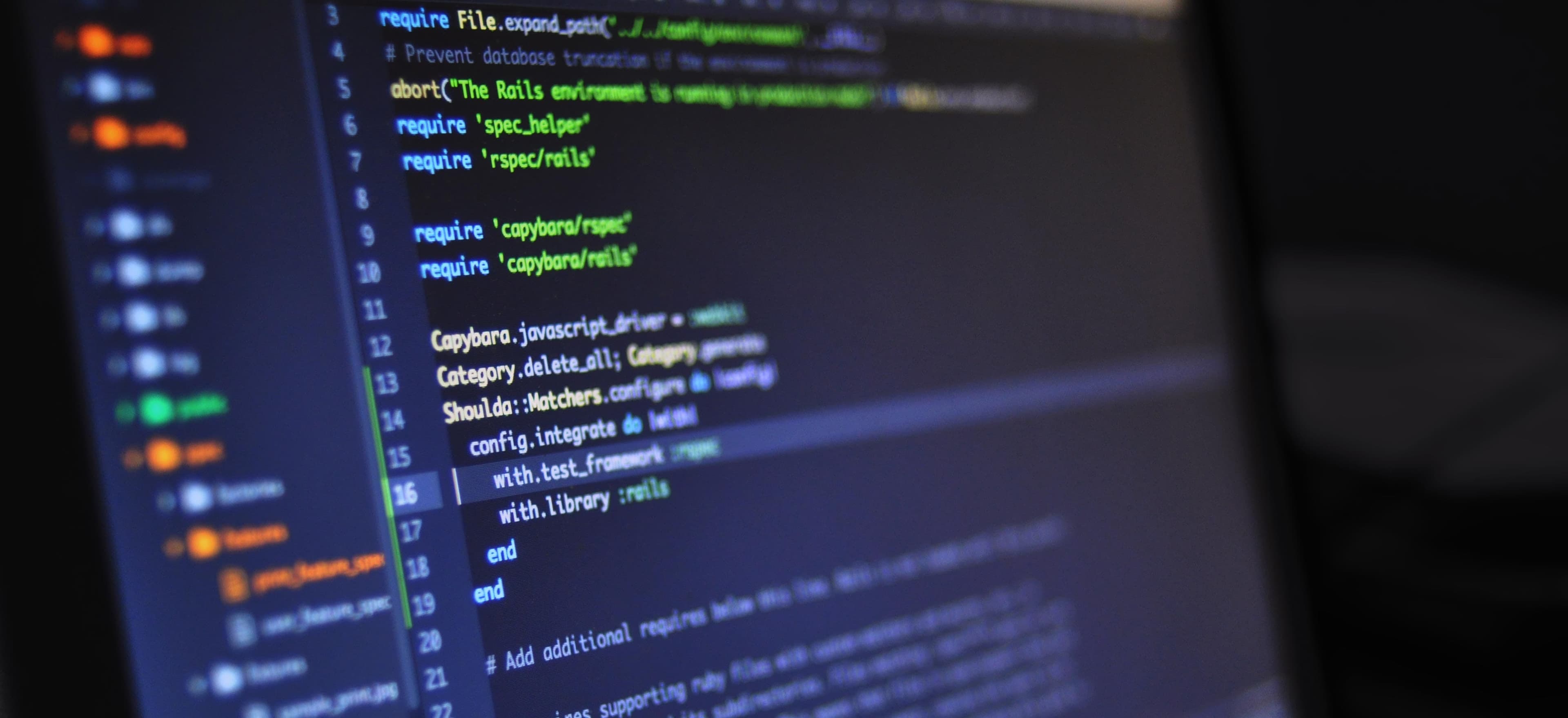
- Published on
Troubleshooting WebLogic 12c Setup with NetBeans
Setting up an Oracle WebLogic Server using NetBeans can be a straightforward process for developers, but occasionally, you might run into hiccups. This blog post serves to guide you through the setup and troubleshooting of WebLogic 12c in conjunction with NetBeans, ensuring a seamless development experience.
Prerequisites
Before diving into the setup and troubleshooting process, it is essential to have the following prerequisites in place:
-
Java Development Kit (JDK): Make sure you have JDK 8 or higher installed. You can download it from Oracle's official website.
-
NetBeans IDE: Download and install the latest version of NetBeans that supports Java EE (Enterprise Edition) development. You can find it here.
-
WebLogic Server 12c: Download the WebLogic Server 12c from the Oracle Technology Network.
Setting Up WebLogic Server in NetBeans
To successfully run and manage WebLogic applications from within NetBeans, follow these steps for setup:
Step 1: Install WebLogic
-
Extract the WebLogic zip file that you downloaded.
-
Navigate to your extraction directory and run the
wls_12.x.x.x.jar
file.java -jar wls_12.x.x.x.jar
-
Follow the installation instructions provided in the installer.
Step 2: Create a Domain
-
After installation, navigate to the
domain
directory in your WebLogic installation, usually found at:<WL_HOME>/wlserver/common/bin
-
Run the following command to create your WebLogic domain:
./config.sh
-
Follow the on-screen instructions to create the domain.
Step 3: Configure NetBeans for WebLogic
- Launch NetBeans and navigate to Tools > Servers.
- Click on Add Server and select Oracle WebLogic Server.
- Enter your WebLogic Home directory and set up necessary configurations.
Step 4: Test the Installation
At this point, you should be able to deploy a simple application to your WebLogic server. Create a new Java EE project in NetBeans, deploy it, and ensure it'll run without issues.
Common Issues and Their Solutions
Even with thorough preparation, issues may arise during installation or usage. Below are some common issues developers face when setting up WebLogic 12c in NetBeans and their solutions.
Issue 1: Database Connection Errors
One of the most common errors developers encounter is a failure to connect to a database. This usually occurs due to incorrect settings in your data source.
Solution:
- Check your JDBC driver. Ensure that it's included in the WebLogic server library.
- Configure your datasource correctly by going to the WebLogic Console (
http://localhost:7001/console
):- Navigate to Services > Data Sources.
- Click on New to create a new data source. Make sure to specify the right JNDI name and connection properties.
Issue 2: The Server Won't Start
If your WebLogic server fails to start from NetBeans, it can be due to several reasons, including port conflicts and environmental variables.
Solution:
- Ensure that the ports used by WebLogic are not already occupied. Default ports are 7001 and 7002 (SSL).
- Check your JAVA_HOME and PATH environment variables. They should be pointing to the JDK directory.
- Review the server logs found in the
logs
directory of your domain folder to diagnose the issue.
Example Code Snippet
Here's a simple code snippet demonstrating how to create a Data Source in WebLogic using the Java Naming and Directory Interface (JNDI):
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class DataSourceDemo {
public static void main(String[] args) {
try {
Context ctx = new InitialContext();
DataSource ds = (DataSource) ctx.lookup("java:comp/env/jdbc/MyDataSource");
// Use the DataSource to retrieve a connection
Connection con = ds.getConnection();
System.out.println("Connection established successfully");
// Always remember to close the connection
con.close();
} catch (NamingException | SQLException e) {
e.printStackTrace();
}
}
}
Why This Code is Important:
- The above snippet shows how to look up a JNDI resource to connect to your database.
- Review error handling practices in Java to appropriately manage exceptions during connection attempts.
Issue 3: Memory Issues
If your application is consuming too much memory, WebLogic might crash.
Solution:
-
Increase the Java heap size by launching the server with
-Xms
(initial memory) and-Xmx
(maximum memory) options.set JAVA_OPTIONS=-Xms512m -Xmx2048m
-
Verify that you’re not allocating too much memory compared to your machine specifications.
Debugging Techniques
When things go wrong, you need effective debugging techniques. Here are a few:
-
Logging: Always log your configurations and exceptions using Log4j or SLF4J. This gives you instant feedback on what went wrong.
-
Verbose Garbage Collection: Adjust the JVM to give you verbose GC information which can help diagnose memory-related issues.
-verbose:gc
-
NetBeans Profiler: Use the built-in profiling features in NetBeans to diagnose performance issues within your application.
Key Takeaways
Setting up and managing WebLogic 12c in conjunction with NetBeans can be efficient if you follow the correct procedures and heed important troubleshooting tips. Remember to check common issues, keep an eye on logs, and utilize debugging techniques to ensure your application runs smoothly.
For any further reading or deeper insights into concepts like JNDI, JDBC, or memory management in Java, consider exploring sites such as Oracle Documentation and Baeldung.
With the right tools and approaches, you’ll find developing on WebLogic an enriching experience!
Feel free to comment below if you have any questions or need additional assistance!