Overcoming Common Pitfalls in RAG App Development
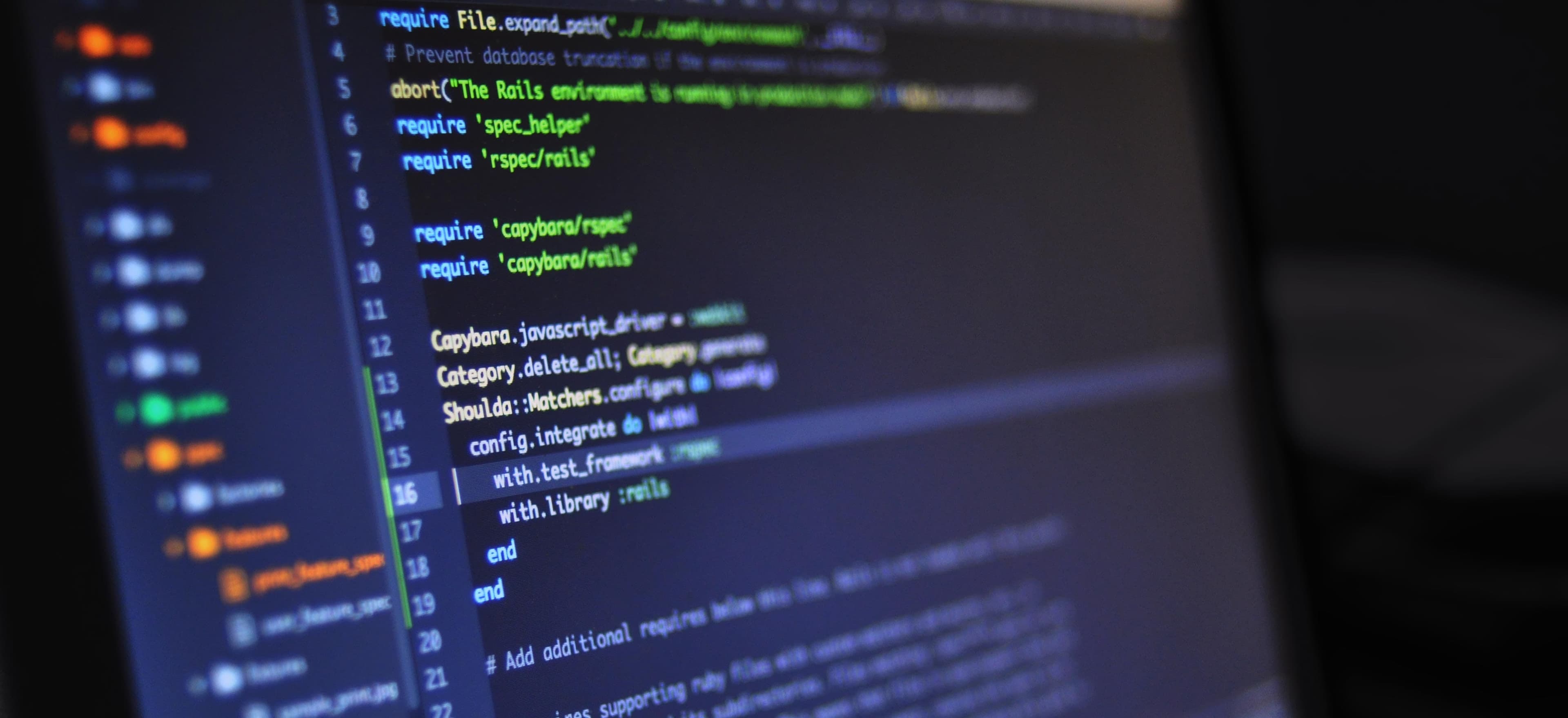
- Published on
Overcoming Common Pitfalls in RAG App Development
In the fast-paced world of application development, RAG (Red-Amber-Green) apps have gained traction due to their efficacy in visually representing data and progress. However, navigating their development can be fraught with pitfalls. This post aims to uncover these pitfalls and offer solutions to develop robust RAG applications.
Understanding RAG App Development
Before diving into common pitfalls, it's essential to understand what RAG apps are. RAG apps utilize a color-coded system to provide insight into the status of tasks, projects, or data sets. The colors represent:
- Red: Immediate attention needed
- Amber: Caution required
- Green: All is well
This simple yet effective approach aids decision-making, making RAG apps integral to project management, data analytics, and more.
Common Pitfalls in RAG App Development
1. Lack of Clear Requirements
One of the most significant oversights in RAG app development is failing to define clear requirements upfront. This can lead to scope creep, where additional features are constantly added, making the app more complex without clear value.
Solution
- Requirements Gathering Sessions: Conduct meetings with stakeholders to clarify objectives, target audience, and features. This guarantees all parties have aligned expectations.
2. Overcomplicating the UI
A common mistake is to create an overly complex user interface (UI). While offering numerous features may seem beneficial, it can overwhelm users and detract from the app's primary purpose.
Solution
- Simplicity is Key: Focus on creating an intuitive UI that emphasizes RAG status. For instance, employing a minimalist design will help in enhancing usability.
// Example Java code snippet for a simple RAG UI component
import javax.swing.*;
import java.awt.*;
public class RagStatusPanel extends JPanel {
public RagStatusPanel(String status) {
switch (status.toLowerCase()) {
case "red":
setBackground(Color.RED);
break;
case "amber":
setBackground(Color.ORANGE);
break;
case "green":
setBackground(Color.GREEN);
break;
default:
throw new IllegalArgumentException("Invalid status: " + status);
}
}
}
This code initializes a JPanel that dynamically changes its background color based on the RAG status. Simplifying color-coding boosts user visibility and comprehension.
3. Neglecting User Experience (UX)
Beyond visual simplicity, the overall UX design often takes a backseat. Ignoring the end-user's journey can lead to frustration and could ultimately cause users to abandon the app.
Solution
- User Testing: Conduct several rounds of user testing with prototypes. Pay close attention to how intuitive and satisfying the experience is for real users.
4. Data Integration Challenges
Data feeds that inform RAG statuses are typically sourced from multiple systems. If these integrations are handled poorly, they may result in inaccurate data representations or delayed updates.
Solution
- API Best Practices: Use RESTful APIs and ensure proper integration mechanisms. Consider implementing data reliability checks to validate incoming data.
Here's an example of how to fetch data from an API in Java:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class ApiDataFetcher {
public String fetchData(String apiUrl) throws Exception {
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
StringBuilder output = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
output.append(line);
}
conn.disconnect();
return output.toString();
}
}
This example demonstrates how to fetch data from an API while implementing error handling to manage issues effectively. Ensuring data quality is fundamental to delivering accurate RAG statuses.
5. Insufficient Testing
Insufficient testing is a significant risk that can derail RAG app success. If testing is rushed or ignored, bugs and usability issues can emerge post-launch, harming user acceptance.
Solution
- Robust Testing Strategy: Develop a comprehensive testing plan that includes unit testing, integration testing, and user acceptance testing. Make sure to cover edge cases that may not initially seem relevant.
6. Ignoring Feedback Mechanisms
Once the RAG app is live, ignoring user feedback can lead to stagnation. Continuous improvement through user suggestions is key to retaining users and enhancing the product.
Solution
- Feedback Loops: Implement in-app feedback forms, or use third-party platforms for gathering user opinions. Actively monitor this feedback and prioritize updates accordingly.
7. Security Oversights
In an era where data breaches make headlines regularly, neglecting security practices can be catastrophic. RAG apps often handle sensitive data, making security a priority.
Solution
- Security Protocols: Utilize secure coding practices, regular audits, and employ OAuth for user authentication. Protecting user data fosters trust and compliance with regulations like GDPR.
8. Not Planning for Scale
A lack of foresight concerning app scalability can be detrimental. If the app is successful, high traffic may lead to crashes or performance issues.
Solution
- Architecture Considerations: Use microservices or cloud-native architecture to facilitate scalability. Design the app to handle increased load effortlessly.
Closing Remarks
Overcoming pitfalls in RAG app development is possible with careful planning, user-centric approaches, and agile practices. By identifying common issues and implementing practical solutions, developers can create efficient, user-friendly, and scalable RAG applications.
For more insights on effective project management and application development, check out this resource on agile methodologies and best practices in UI/UX design.
Happy coding, and may your RAG apps shine bright in red, amber, and green!