Challenges of Migrating to Java 8 Long-Term Support
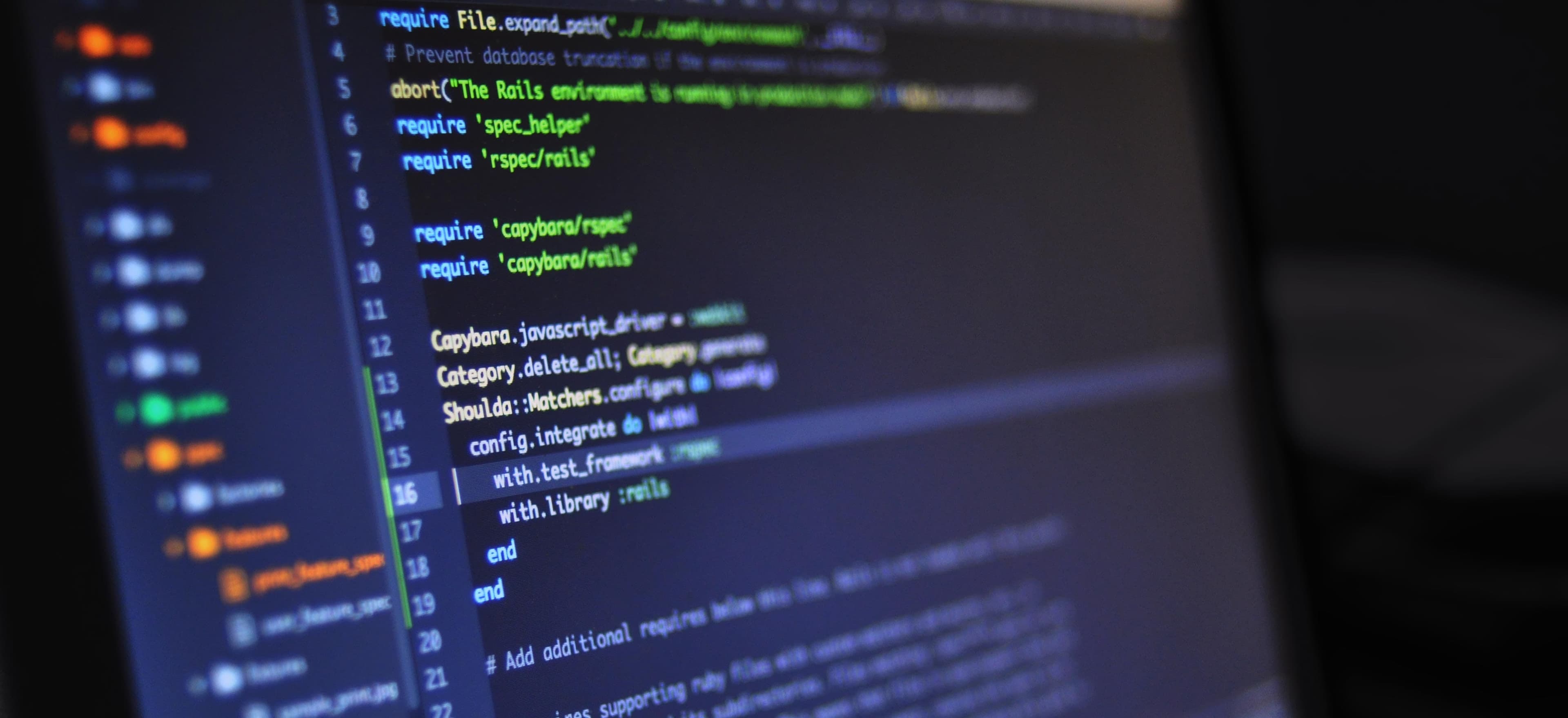
- Published on
Challenges of Migrating to Java 8 Long-Term Support
The evolution of programming languages brings many advantages but also presents unique challenges. When we look at Java, a widely-used programming language known for its stability and security, migrating to a new version can be daunting. Java 8, released in March 2014, introduced several significant features that transformed how developers approach coding. The Long-Term Support (LTS) version of Java 8 has proven to be especially alluring due to its extended support and stability. Nevertheless, the migration process involves hurdles that developers need to overcome.
In this blog post, we will delve into the challenges of migrating to Java 8 LTS, discussing the implications it has on legacy systems, incorporating modern features, and understanding dependencies and environmental considerations.
1. Understanding Legacy Code
One of the biggest challenges of migration lies in the legacy code that organizations have been using for years, if not decades. Older versions of Java, especially Java 6 and 7, have formed the backbone of many enterprise applications.
Code Compatibility
Many existing Java applications may rely on outdated libraries or specific Java features that have been deprecated or altered in newer versions. For instance, the new Date and Time API, introduced in Java 8, offers a more comprehensive and flexible way to manage time, but it also means that any legacy code using the older Date class will require significant rewrites.
// Legacy Date usage
import java.util.Date;
public class LegacyDate {
public static void main(String[] args) {
Date legacyDate = new Date();
System.out.println("Legacy Date: " + legacyDate.toString());
}
}
This could pose compatibility issues, especially if third-party libraries are involved. A good strategy is to establish a compatibility layer that can help transition older systems to handle the new Date and Time API.
Migration Strategy
Developers should conduct thorough testing for deprecated features and identify which parts of the codebase might need refactoring. Creating a migration plan that prioritizes critical components is critical. This plan should include a rollback strategy in case any migration issues arise during implementation.
2. Implementation of Modern Features
The introduction of Java 8's modern features, such as Lambda expressions, Streams, and the new Optional class, means developers not only need to rewrite old code but to also embrace new paradigms.
Embracing Lambdas
Lambda expressions simplify the way you write functional interfaces. For example, instead of creating anonymous inner classes, you can use a clean, concise syntax to express instances of single-method interfaces.
// Using Lambda Expressions
import java.util.Arrays;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach(name -> System.out.println(name)); // Lambda expression in action
}
}
While this example is straightforward, existing teams may face resistance to adopting Lambda-style programming. To alleviate this, integrating workshops, training sessions, or reading resources can help ease the transition.
Streams API
Another revolutionary feature is the Stream API, which allows for functional-style operations on collections. Instead of looping through collections, developers can write cleaner, more declarative code.
// Using Streams
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream().mapToInt(Integer::intValue).sum();
System.out.println("Sum: " + sum);
}
}
Transitioning to a Stream-based approach may require breaking existing programming habits, which could be a struggle for some teams. Advocating for code reviews and pair programming can also ease the acceptance of these new methodologies.
3. Managing Dependencies
Migration can sometimes be complicated by dependencies on libraries and frameworks that may not yet support or fully integrate with Java 8.
Third-Party Libraries
Many libraries that were built for earlier Java versions may not seamlessly transition to Java 8. This situation can lead to a dependency hell scenario where libraries conflict with each other or are no longer updated.
When migrating, it is crucial to interactively audit your project dependencies. Tools like Maven or Gradle can automate this process, helping to identify outdated libraries and suggesting alternatives.
Framework Issues
If your Java application is built upon popular frameworks such as Spring or Hibernate, ensuring compatibility with Java 8 is imperative. Upgrading the framework version may be necessary.
For instance, Spring 4.0 introduced support for Java 8 features, but applications running on older versions would need to be carefully planned for migration to ensure smooth operations.
4. Environmental Considerations
Software Environment
Java migration isn't just about the code. Your software environment carries as much weight. It's essential to ensure that your server, IDEs, and build tools are compatible with Java 8.
Testing Environments
Setting up testing environments that reflect the production environment accurately is vital. Virtual Machines (VMs) or Docker containers can be extremely beneficial here, allowing you to simulate the production environment after migrating to Java 8, ultimately reducing surprises down the line.
Continuous Integration / Continuous Deployment (CI/CD)
If your team is leveraging CI/CD pipelines, ensure that your build tools, such as Jenkins or Travis CI, support Java 8. This migration may require changes in configurations, scripts, or even the setup of build agents.
Key Takeaways
Migrating to Java 8 Long-Term Support presents several challenges, but with careful planning and collaborative teamwork, these challenges can be met effectively. Understanding legacy code and its particularities, embracing the modern features Java 8 offers, managing dependencies diligently, and ensuring environmental compatibility will pave the way for a successful migration.
The rewards for undertaking this journey are immense. Improved code efficiency, reduced technical debt through modern coding practices, and extended support for the Java ecosystem are just some of the benefits that organizations can enjoy.
If you want to deepen your understanding of Java 8 features, you can always refer to Oracle's official documentation or explore insightful articles on notable Java functionalities to prepare you for your migration initiative.
By ensuring your team is adequately equipped and your strategy is solid, you will harness the power of Java 8 LTS, enhancing both productivity and application performance in the long run.