Common Pitfalls When Containerizing Spring Boot with Podman
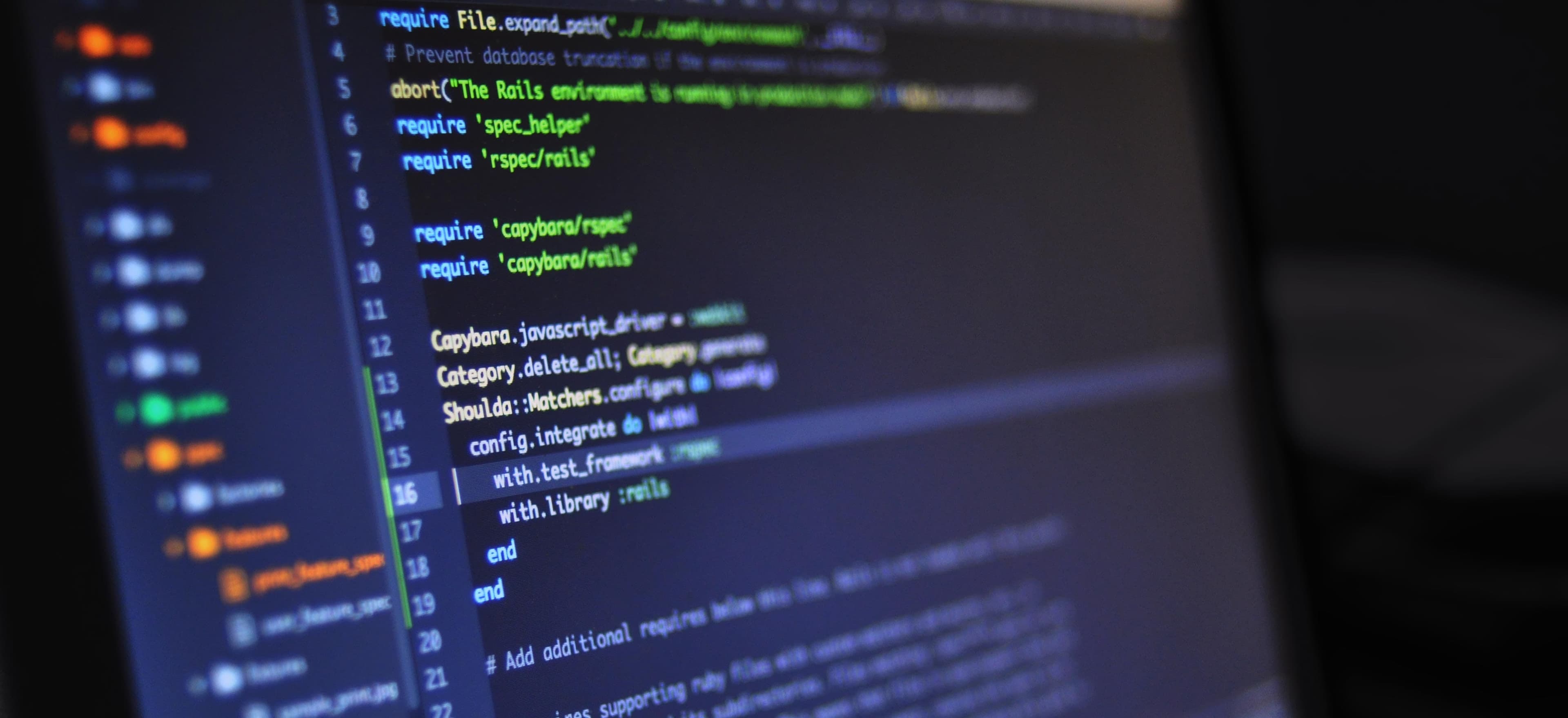
- Published on
Common Pitfalls When Containerizing Spring Boot with Podman
In today's microservices architecture, containerization is not just a trend; it's a necessity. Developers and organizations are constantly on the lookout for efficient ways to deploy applications. Two tools that stand out in this realm are Spring Boot, a popular Java framework, and Podman, a daemonless container engine that offers a refreshing alternative to Docker.
While containerization simplifies deployment, it often comes with its set of challenges. In this blog post, we'll explore common pitfalls developers face when containerizing Spring Boot applications with Podman, along with practical solutions to address these issues.
Understanding Podman
Before we dive into the pitfalls, it’s essential to understand what Podman brings to the table. If you’re familiar with Docker, you’ll recognize many similarities. However, Podman stands out by eliminating the need for a daemon and enabling rootless containers. This is not just a minor difference—it can impact how you manage security and the user environment in which your containers run.
For more information on Podman’s architecture, check out Podman Official Documentation.
Pitfall 1: Base Image Selection
The Problem
Many developers start with generic base images when containerizing their Spring Boot applications. Choosing a base image can drastically affect not only the size of your container but also its performance and security. Many Java applications are resource-heavy, and using a bloated base image can lead to slow startup times.
The Solution
Opt for a minimal base image that suits your application needs. A common choice for Java applications is the adoptopenjdk
or other OpenJDK variants.
FROM adoptopenjdk:11-jre-hotspot as base
# Copy the dependency JAR
COPY target/myapp.jar /app/myapp.jar
# Label for improving readability
LABEL maintainer="your_email@example.com"
In this snippet, we are using a specific JRE image rather than a full JDK. This reduces the final image size and maintains a good balance between functionality and overhead.
Pitfall 2: Mismanagement of Dependencies
The Problem
Spring Boot applications often have numerous dependencies, and if not managed correctly, they can lead to bloated images and unnecessary overhead. This is especially true if you package your JAR with its dependencies included.
The Solution
Use the maven
or gradle
build tools wisely. Here’s how you can efficiently package your application:
# For Maven users
mvn clean package -DskipTests
# For Gradle users
./gradlew build -x test
Using flags to skip tests during the build process can save time when you're containerizing your application for initial testing.
Pitfall 3: Configurations Hardcoded in the JAR
The Problem
Hardcoding configurations (like database URLs, API keys, etc.) in your Spring Boot JAR can lead to multiple issues during container orchestration. Such practices reduce portability and make the development cycle very cumbersome.
The Solution
Utilize environment variables or external configuration files to manage settings. Spring Boot supports externalized configuration, which can be loaded at runtime.
Example of using environment variables:
# application.yml
spring:
datasource:
url: ${DB_URL:jdbc:mysql://localhost:3306/mydb}
username: ${DB_USERNAME:root}
password: ${DB_PASSWORD:password}
In this example, Spring Boot will use the provided environment variables. If those variables aren’t set, it falls back to default values.
Pitfall 4: Inefficient Layering
The Problem
When building images, caching can play a significant role in speeding up build times. However, inefficient layering can force you to rebuild layers that haven't changed, leading to slower builds and longer deployment times.
The Solution
Order the commands in your Dockerfile
to maximize layer caching. Place rarely changing commands above frequently changing ones.
# First, add the Maven dependencies (they change less frequently)
COPY pom.xml /app/
RUN mvn dependency:go-offline
# Then copy the rest of your application
COPY src /app/src
COPY target/myapp.jar /app/myapp.jar
# Run the application
CMD ["java", "-jar", "/app/myapp.jar"]
In this example, we first copy the pom.xml
file and install dependencies. This way, if your source code changes but your dependencies do not, there is no need to re-run the expensive mvn dependency:go-offline
command.
Pitfall 5: Poor Error Handling in Containerized Environment
The Problem
During deployment, developers sometimes forget to implement adequate error handling within the application or when executing commands in the container. This can lead to untraceable errors, making debugging a nightmare.
The Solution
Ensure you have robust logging in place within your Spring Boot application and capture the stderr and stdout when running the container.
Here's how you could run your Podman container with logging enabled:
podman run --name myapp -d -e DB_URL=jdbc:mysql://mydb:3306 -p 8080:8080 myapp:latest
Adding flags like -d
to run in detached mode (background), and -e
to pass environment variables allows you to monitor what happens in case something goes wrong.
Pitfall 6: Ignoring Security Best Practices
The Problem
Security can often take a back seat when it comes to containerization. Exposing unnecessary ports or using root privileges can leave your application vulnerable.
The Solution
Run your Podman containers with non-root users and limit the exposed ports.
Here’s how to structure your Podman command:
podman run --user 1001:1001 -p 8080:8080 myapp:latest
Using the --user
flag helps in running the application as a user with a specified UID and GID rather than the default root user.
Bringing It All Together
Containerizing Spring Boot applications with Podman can streamline your development and deployment workflow, but it's fraught with challenges that can quickly turn into roadblocks. By staying vigilant about selecting a suitable base image, managing dependencies, avoiding hardcoded values, maximizing layer caching, implementing error handling, and adhering to security best practices, you can build efficient and secure containers.
By understanding and circumventing these common pitfalls, you'll be better equipped to leverage the powerful synergy between Spring Boot and Podman, ensuring a smooth containerization experience.
For further reading, consider exploring Best Practices for Docker to complement your knowledge on containerization, and check out Spring Boot Official Documentation for more details on how to optimize your Spring Boot applications.
Happy containerizing!