Troubleshooting Zipkin Integration in Spring Applications
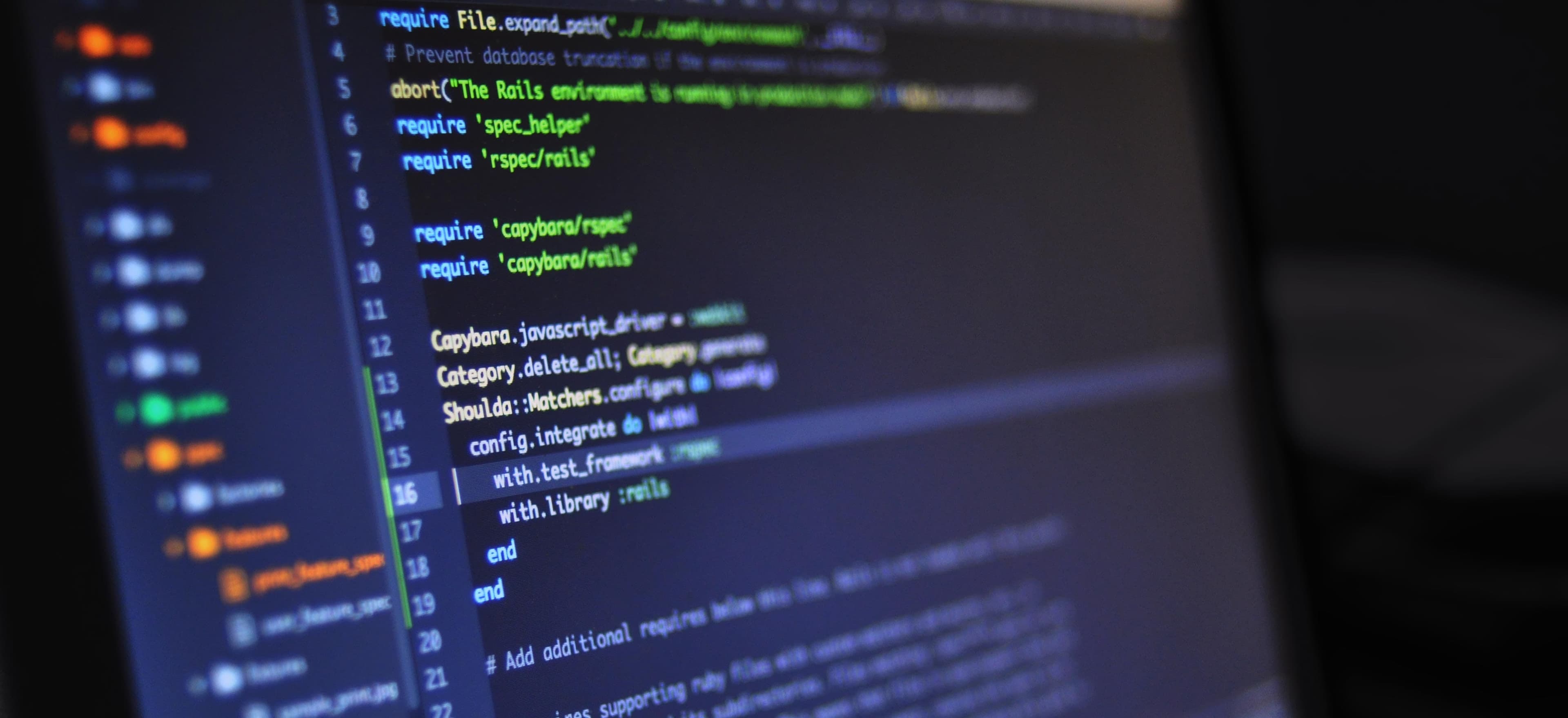
- Published on
Troubleshooting Zipkin Integration in Spring Applications
Zipkin is a distributed tracing system that helps developers gather timing data needed to troubleshoot latency problems in microservices. While integrating Zipkin with Spring applications generally works seamlessly, there may be cases where developers face challenges. In this blog post, we will explore common issues encountered during Zipkin integration and how to resolve them effectively.
Understanding Zipkin Architecture
Before diving into troubleshooting, it's essential to understand how Zipkin fits into your Spring application architecture. Zipkin collects trace data from various services connected in a distributed system. It visualizes the data, helping you pinpoint where latency occurs.
Here's a simplified architecture:
- Zipkin server: Collects and stores trace data.
- Spring applications: Generate trace data, often configured with Spring Cloud Sleuth.
- Instrumentation: Helps in capturing the data from your application.
Key Components of Zipkin:
- Span: Represents a single unit of work.
- Trace: A collection of spans that work together to complete a request.
- Annotations: Tags associated with spans that provide information about the operations.
Basic Setup of Zipkin in Spring Applications
Integrating Zipkin in Spring usually involves adding dependencies and configuration settings. Here's a straightforward setup to start:
Dependencies
If you're using Maven, add the following dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-sleuth</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-zipkin</artifactId>
</dependency>
For Gradle users, you would include:
implementation 'org.springframework.cloud:spring-cloud-starter-sleuth'
implementation 'org.springframework.cloud:spring-cloud-starter-zipkin'
Application Properties
Configure Zipkin in your application.properties
or application.yml
:
spring.zipkin.base-url=http://localhost:9411
spring.sleuth.sampler.probability=1.0
Why these configurations matter:
spring.zipkin.base-url
: This tells your application the Zipkin server's location.spring.sleuth.sampler.probability
: Controls the percentage of traces sent to Zipkin. A value of1.0
means all traces will be collected.
Common Troubleshooting Scenarios
1. Zipkin Not Receiving Any Traces
Symptoms: You don't see any trace data on the Zipkin UI.
What to Check:
- Is Zipkin Running?: Ensure that the Zipkin server is up and running. You can start it with Docker easily:
docker run -d -p 9411:9411 openzipkin/zipkin
- Configuration Issues: Double-check that the
spring.zipkin.base-url
is correctly pointing to your running Zipkin instance. Use tools likecurl
to verify the endpoint:
curl http://localhost:9411/
- Sampler Probability: If your
spring.sleuth.sampler.probability
is too low (e.g.,0.0
), no traces will be sent. Make sure it is set adequately for testing.
2. Tracing Latency
Symptoms: Data is being sent, but there's significant latency in the traces.
What to Check:
-
Network Issues: Check the network latency between your application and the Zipkin server. Slow networks can delay trace submissions.
-
Asynchronous Calls: Span completion may be deferred in asynchronous calls. If your service uses asynchronous patterns, ensure that spans are completed promptly.
-
Error Handling: Proper error handling ensures that spans aren't prematurely closed. Use the following code to add error handling to your tracing:
try {
// Your business logic here
} catch (Exception e) {
// Log the error and add it to the span
Span span = Tracer.currentSpan();
if (span != null) {
span.tag("error", "true");
span.log("Exception occurred: " + e.getMessage());
}
}
3. Missing Service Identification
Symptoms: You see some traces, but service names are not correctly identified.
What to Check:
- Service Name Conflicts: Ensure your service name isn't being overwritten or set to a generic value. You can explicitly set the service name in your application properties:
spring.application.name=my-service
- Correct usage of
@NewSpan
: If you are using@NewSpan
annotations, make sure they are applied correctly to your methods.
4. Trace Data Aggregation Issues
Symptoms: Traces from various services are not grouped properly in Zipkin.
What to Check:
- Trace Context Propagation: Make sure the trace context is propagated across service boundaries. Use
RestTemplate
orWebClient
in Spring to automatically carry trace information.
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
- Using the Right HTTP Client: If you are using custom HTTP clients, ensure they integrate with Sleuth for trace context propagation.
Additional Resources
For more in-depth resources on Zipkin and Sleuth, check the following:
Final Considerations
Integrating Zipkin into a Spring application offers invaluable insight into your distributed systems. Despite some common issues that could arise during the setup, a clear understanding of the components and configuration can enhance your troubleshooting capabilities. By following best practices and leveraging Spring's capabilities, you can ensure that you capture necessary trace data effectively and efficiently.
Feel free to dive into these troubleshooting techniques, and remember that with each hurdle you overcome, your application's performance will only improve. Happy tracing!
Checkout our other articles