Common Pitfalls When Using Google Guava Cache
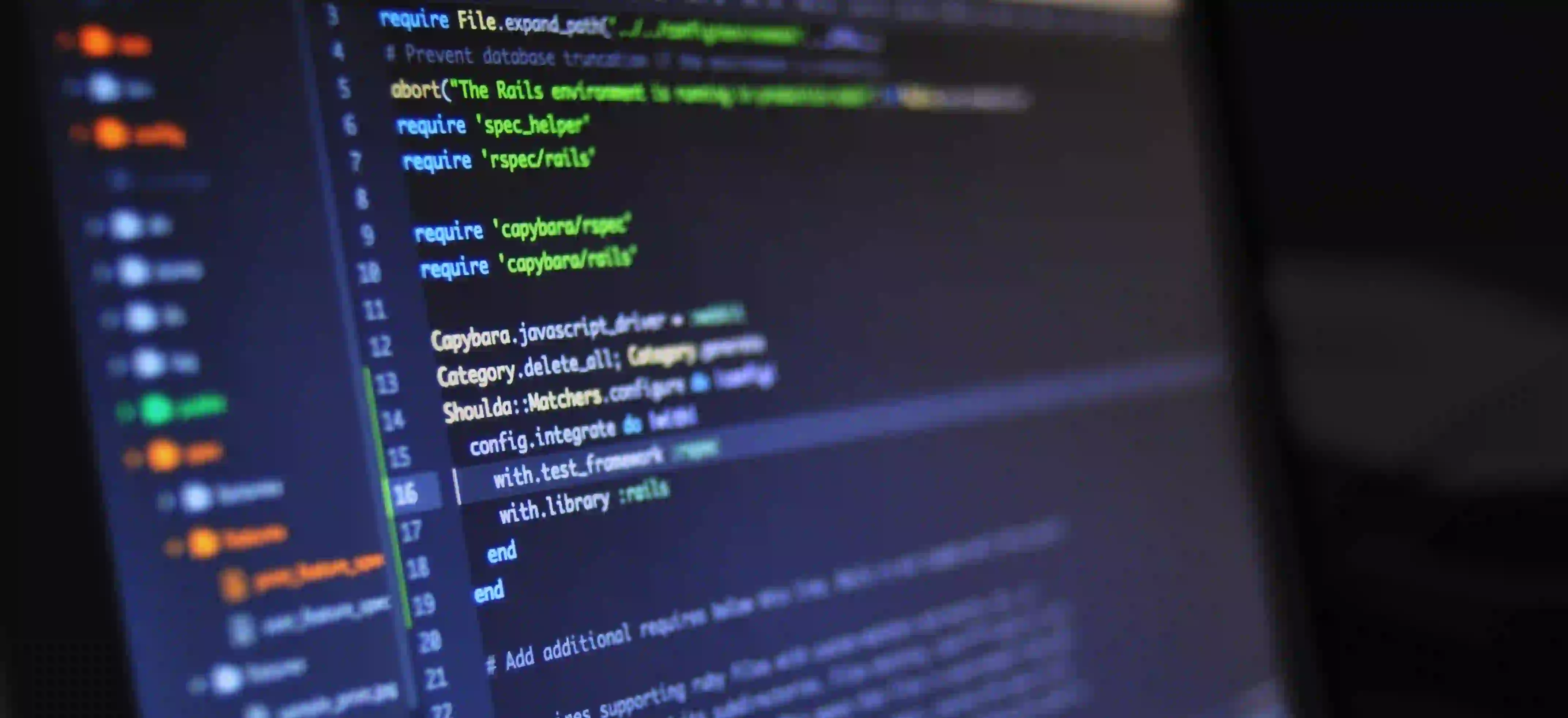
Common Pitfalls When Using Google Guava Cache
Google Guava is a popular open-source Java library that provides numerous utility functionalities, among which its caching capabilities stand out. The Guava Cache allows developers to store data temporarily in memory, enhancing performance by reducing latency on repeated data fetches. However, as with any powerful tool, there are common pitfalls developers may encounter when using the Guava Cache. This blog will delve into these pitfalls while providing solutions and best practices.
What is Google Guava Cache?
Before diving into the pitfalls, let's take a moment to understand what Google Guava Cache is and how it operates. Guava Cache provides a local in-memory cache for Java applications, allowing for efficient data retrieval and storage. Its features include:
- Automatic eviction: Items can expire after a particular time or be removed when the maximum size is exceeded.
- Asynchronous loading: Cache entries can be loaded asynchronously as needed.
- Support for weak references: Helping to prevent memory leaks by allowing cached objects to be garbage collected.
Basic Example of Using Guava Cache
Here's a simple example demonstrating how to set up and use Guava Cache:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.ExecutionException;
public class GuavaCacheExample {
public static void main(String[] args) throws ExecutionException {
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(100) // Limit cache size to 100 entries
.expireAfterWrite(10, java.util.concurrent.TimeUnit.MINUTES) // Data expires after 10 minutes
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
return fetchDataFromDatabase(key); // Simulate fetching data from a database
}
});
// Fetch data for the first time
String data1 = cache.get("key1");
System.out.println("Data: " + data1);
// Fetch the same data again
String data2 = cache.get("key1");
System.out.println("Data: " + data2); // Should be cached
}
private static String fetchDataFromDatabase(String key) {
// Simulating costly database operation
return "Fetched Data for " + key;
}
}
In this example, a CacheLoader is defined to fetch data when it's not available in the cache. Properly managing the cache reduces the likelihood of data fetch overhead.
Common Pitfalls
Now that we've established a basic understanding of the Guava Cache, let’s outline some common pitfalls and how to navigate them effectively.
1. Ignoring Cache Size Limitations
One of the most frequent mistakes developers make is not configuring maximum cache size properly. If the cache doesn’t have a limit, it risks consuming too much memory, which can lead to performance degradation.
Solution: Set a maximum size based on your application's needs.
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(1000) // Set a reasonable cache size
.build(new CacheLoader<String, String>() { /* CacheLoader implementation */ });
By explicitly setting a maximum size, we ensure that memory consumption is monitored and controlled.
2. Caching Everything
While it might be tempting to cache every piece of data, indiscriminately caching everything can lead to unnecessary memory usage and potential data staleness.
Solution: Be selective about what data is cached. Cache data that is frequently accessed and costly to retrieve.
if (shouldCache(data)) {
cache.put(key, data);
}
In the code example, only data that qualifies based on specific criteria is added to the cache.
3. Not Handling Cache Evictions
Another common oversight is failing to account for cache evictions. When the cache reaches its size limit, or data expires, it is essential to handle what happens next. Unmanaged evictions can lead to application errors if critical data is removed.
Solution: Implement listeners or callbacks.
cache = CacheBuilder.newBuilder()
.maximumSize(1000)
.removalListener(notification -> {
System.out.println("Removed key: " + notification.getKey());
})
.build();
By adding a removal listener, you can take necessary actions when items are evicted, such as logging or updating a backing store.
4. Blocking Calls
When using CacheLoader
, people often forget that cache loading can block threads if not handled asynchronously. This can result in slower response times, especially under heavy load.
Solution: Use AsyncCacheLoader
.
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.build(Caffeine.newBuilder()
.maximumSize(1000)
.buildAsync((key) -> fetchDataFromDatabase(key)));
Using asynchronous loading helps to provide a non-blocking approach to data fetching, improving overall performance.
5. Inadequate Testing of Expirations
Expiration settings can sometimes be overlooked during development. A cache that never expires can hold onto stale data, leading to inconsistent application behavior.
Solution: Write tests for cache expiration scenarios.
@Test
public void testCacheExpiration() throws InterruptedException {
LoadingCache<String, String> cache = setupCacheWithExpiration();
cache.put("key", "value");
Thread.sleep(60 * 1000); // Wait for expiration time
assertNull(cache.getIfPresent("key")); // Assert that the key has expired
}
This test scenario simulates the conditions under which cached data may expire, promoting better reliability.
6. Overly Complicated Caching Strategies
Sometimes developers overcomplicate their caching strategy, leading to confusion and maintenance overhead. Opt for simplicity where possible.
Solution: Start with basic caching and evolve as needed.
// Simple cache usage example
String value = cache.get(key); // Simple, straightforward fetching
As requirements evolve, you can layer complexity incrementally.
7. Failure to Monitor Cache Performance
Ignoring performance metrics related to cache usage can hide issues until they become significant problems. For example, frequent cache misses might indicate a poorly configured cache.
Solution: Utilize Guava's built-in metrics or consider third-party monitoring tools.
import com.google.common.cache.CacheStats;
CacheStats stats = cache.stats();
System.out.println("Cache Hits: " + stats.hitCount());
System.out.println("Cache Misses: " + stats.missCount());
By periodically checking cache statistics, you can diagnose performance issues proactively.
The Bottom Line
Google Guava Cache is a powerful tool that, when used correctly, can significantly improve application performance. Avoiding the common pitfalls we've discussed will ensure that you use this caching mechanism effectively. By configuring proper cache size, selectively caching data, handling evictions, and keeping performance in check, you can harness the full potential of Guava Cache without falling into traps that could hinder your application.
For more on caching strategies, check out Caching best practices and the Guava documentation, which provide deeper insights into creating efficient caching systems.
Happy coding!