Overcoming Challenges in Java Latency Benchmarking
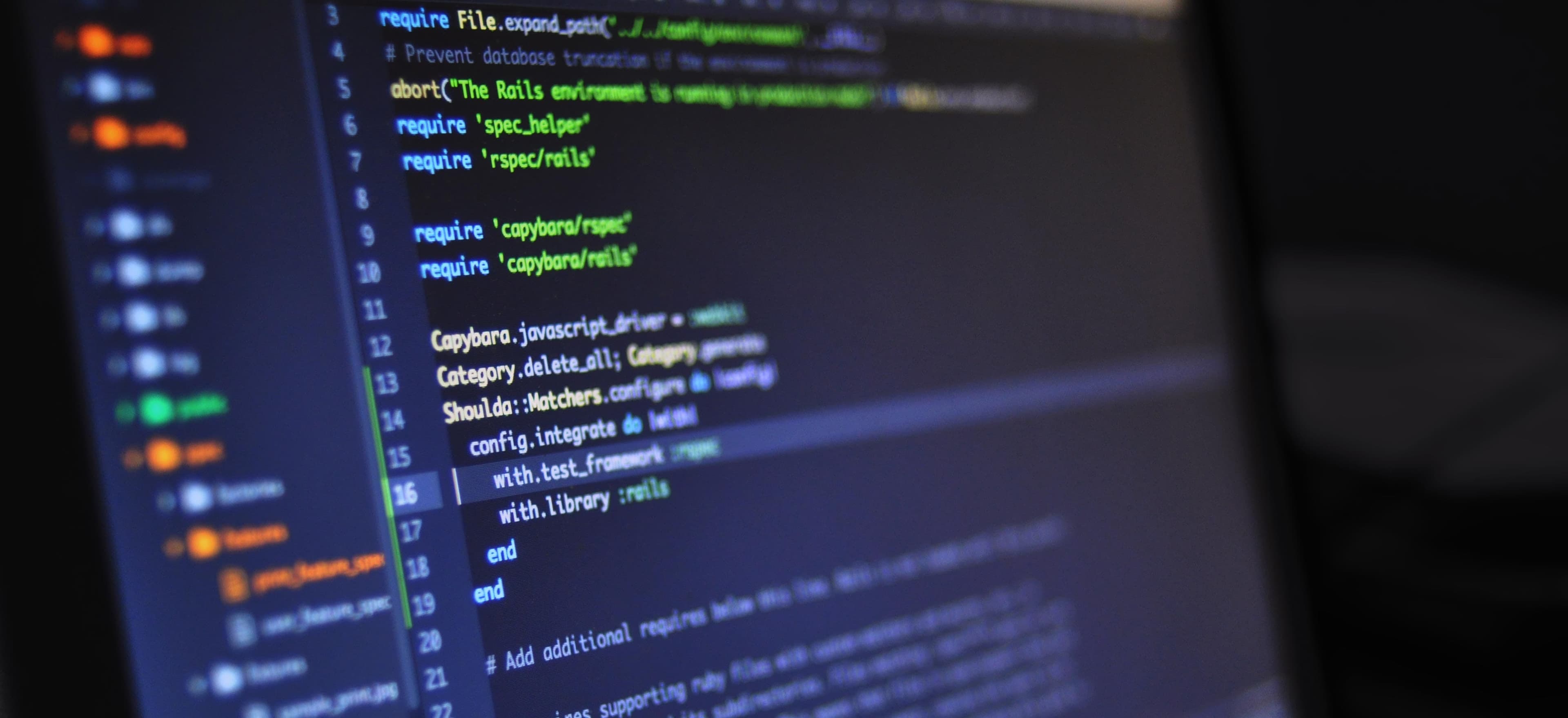
- Published on
Overcoming Challenges in Java Latency Benchmarking
Latency benchmarking is a critical aspect of evaluating the performance of applications in Java. In a world where response times can be the difference between user satisfaction and frustration, it becomes essential for developers to conduct thorough benchmarking. This post delves into common challenges encountered during Java latency benchmarking and offers practical solutions to overcome them.
Understanding Latency Benchmarking
Latency refers to the time it takes for a system to respond to a request. In Java applications, this could relate to various components, such as database calls, network requests, or internal processing. The goal of latency benchmarking is to identify bottlenecks and ensure that the application can handle the expected load with minimal delays.
Key Concepts:
- Throughput vs. Latency: While throughput measures the number of requests processed over time, latency is concerned with the time taken for a single request.
- Warm-up Period: Latency measurements should not begin until the Java Virtual Machine (JVM) is fully warmed up to avoid misleading results due to Just-In-Time (JIT) compilation.
Common Challenges in Latency Benchmarking
1. Variability in Execution Time
Java applications can exhibit significant variability in execution time due to many factors:
- JIT compilation
- Garbage collection (GC)
- Thread contention
Solution: Use appropriate constraints and configurations to minimize variations. For example, executing multiple iterations under a steady state can yield more consistent results.
2. The Impact of Garbage Collection
Garbage collection pauses can distort latency metrics. Even short pauses can significantly affect response times in applications that require low latency.
Solution: Use low-pause garbage collection algorithms, such as the G1 garbage collector, or conduct runs during off-peak hours when GC events are less frequent.
public class G1GCExample {
public static void main(String[] args) {
System.out.println("Running with G1 Garbage Collector...");
// Simulate workload here
}
}
// Run with JVM options: -XX:+UseG1GC
3. Network Latency
When benchmarking applications that involve network calls, the variability of external network responses can introduce significant challenges.
Solution: Isolate benchmarks by using mock servers or stubbing network responses. Tools like WireMock allow you to simulate HTTP services, creating a more predictable environment during testing.
import com.github.tomakehurst.wiremock.WireMockServer;
public class MockServer {
public static void main(String[] args) {
WireMockServer wireMockServer = new WireMockServer();
wireMockServer.start();
wireMockServer.stubFor(get(urlEqualTo("/api/data"))
.willReturn(aResponse()
.withStatus(200)
.withBody("Mocked response")));
// Your test code here
wireMockServer.stop();
}
}
Best Practices for Effective Java Latency Benchmarking
1. Use Proper Benchmarking Tools
Leverage established tools such as JMH (Java Microbenchmark Harness) for micro-benchmarking. JMH provides accurate measurement and can take care of many benchmarking pitfalls.
import org.openjdk.jmh.annotations.*;
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MILLISECONDS)
public class BenchmarkExample {
@Benchmark
public void testMethod() {
// Code to benchmark
}
}
// To run the benchmark, use the command line with the proper flags
2. Multiple Warm-up Rounds
Warm-up rounds help the JVM optimize code execution. Set up multiple warm-up iterations in JMH to prepare the JVM before the actual measurements.
@Warmup(iterations = 5)
@Measurement(iterations = 10)
public class BenchmarkWithWarmup {
@Benchmark
public void testMethod() {
// Benchmark code
}
}
3. Analyze Results with Precision
Collect data over time and use statistical analysis to interpret the results accurately. Tools like Grafana or Prometheus can help visualize the data neatly.
4. Consistent Testing Environment
Ensure that the environment is consistent between runs. This includes:
- Same hardware specifications
- Minimal background processes
- Similar workload characteristics
A Final Look
Latency benchmarking in Java serves as a vital practice to ensure robust application performance. With the right strategies and methodologies, developers can overcome common challenges associated with latency testing.
By recognizing the importance of controlled environments and using effective tools like JMH, developers can obtain reliable and meaningful metrics. Always remember the significance of detailed analysis to make informed enhancements to the application.
For further reading on JVM tuning and performance, consider checking Oracle's official Java Performance Tuning guide.
Greater focus on these practices ensures that future applications developed on the Java platform are optimized for performance, catering to an increasingly demanding user base. Happy coding!
This post aims not only to guide you through the challenges but also to inspire confidence in handling Java latency benchmarking effectively. Let us know how these strategies work for you, and feel free to share your experiences!