Common Pitfalls When Starting with ANTLR: Avoid These Mistakes
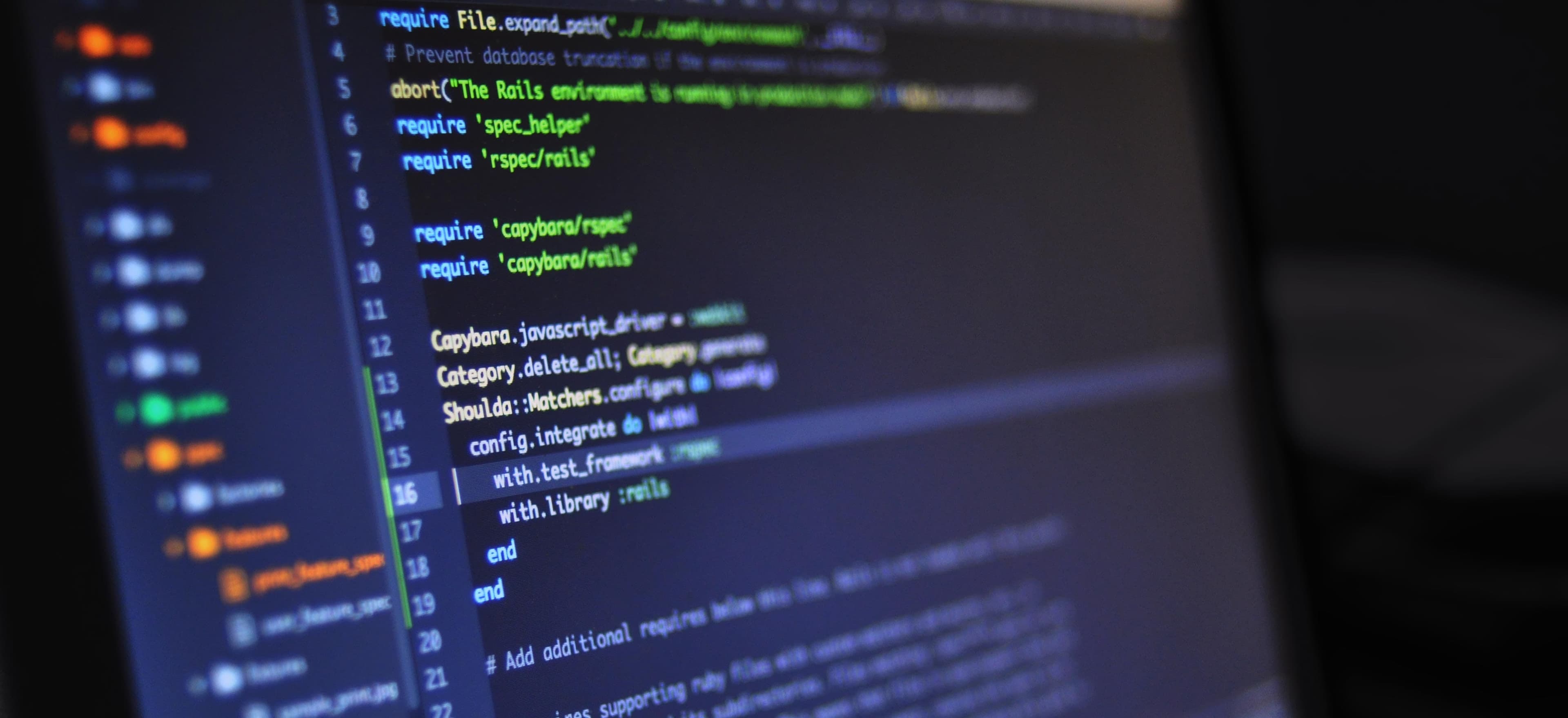
- Published on
Common Pitfalls When Starting with ANTLR: Avoid These Mistakes
ANTLR (Another Tool for Language Recognition) is a powerful tool used for building language parsers, interpreters, and compilers. Whether you’re crafting a new programming language, a domain-specific language (DSL), or streamlining config file parsers, ANTLR can simplify the process. However, starting with ANTLR can be challenging, especially for those new to grammar development. This blog post will highlight some common pitfalls you might face when working with ANTLR and provide tips on how to avoid them.
Understanding ANTLR Basics
Before diving into common pitfalls, let's quickly review what ANTLR is and how it works. ANTLR transforms the grammar you write into parser code, which can then handle input according to the rules you define. This makes it a popular choice for:
- Creating compilers for programming languages
- Implementing interpreters
- Developing configuration file processors
A Simple Example
To illustrate how ANTLR works, here's a basic example of a grammar for a simple expression language:
grammar Expr;
// The entry point of the grammar
expr: term (('+' | '-') term)*;
// Define terms
term: factor (('*' | '/') factor)*;
// Define factors
factor: INT | '(' expr ')';
// Define tokens
INT: [0-9]+;
WS: [ \t\n\r]+ -> skip; // Skip whitespaces
In this example, expr
is the starting point of the grammar. It defines an expression that can include addition, subtraction, multiplication, and division. The use of whitespace skipping improves usability by ignoring spaces and tabs.
Why This Example is Important
This example sets the foundation for understanding how ANTLR parses input, but it is also indicative of where beginners can go wrong. Let’s explore the common pitfalls that developers encounter when starting with ANTLR.
1. Ignoring Token Types
One common mistake beginners make is neglecting the definition of token types adequately. Token types are essential because they dictate how the parser recognizes input text.
Solution: Be Explicit
When creating your grammar, ensure that you define token types clearly and adequately. Here’s an improved approach to token definitions:
// Define tokens clearly
ID: [a-zA-Z_][a-zA-Z_0-9]*; // Identifiers must start with a letter or underscore
FLOAT: [0-9]+ '.' [0-9]+; // Floating point numbers
Why It Matters
Defining token types clearly aids in error reporting and makes your grammar more resilient. For instance, if your language includes identifiers, numbers, and floats, being explicit about their syntax helps ANTLR give you precise error messages and avoid misinterpretations.
2. Overcomplicating the Grammar
Beginners sometimes write overly complicated grammars that are hard to read and maintain. ANTLR can handle complex expressions, but simplicity is key when getting started.
Solution: Start Simple, Refine Later
Begin with a simple version of your grammar that captures the core functionality. For instance, instead of combining multiple complex rules, break it down into smaller, manageable pieces. This approach will help in debugging and maintenance.
expr: term (('+' | '-') term)*; // Keep it simple
Why Less is More
Simplicity leads to clarity. A clear, simple grammar is easier to debug, understand, and extend later on as your needs grow.
3. Neglecting Error Handling
Many beginners overlook error handling, which can lead to confusion during parsing when inputs do not comply with grammar rules.
Solution: Implement Robust Error Handling
You can customize error handling in ANTLR effectively by overriding the default error methods in the generated parser. Here’s an example:
@Override
public void recover(RecognitionException e) {
System.out.println("Syntax error at line " + e.getOffendingToken().getLine());
// Custom recovery logic
}
Why Error Handling is Crucial
Robust error handling ensures that your parser behaves predictably in the face of malformed input. Good error messages will help users of your language understand what went wrong.
4. Not Taking Advantage of ANTLR's Features
ANTLR provides a wide range of features that can facilitate parser development, but beginners often overlook these capabilities.
Solution: Familiarize Yourself with ANTLR Features
Spend some time exploring ANTLR features such as:
- Listener and Visitor patterns: To traverse parse trees
- Syntactic predicates: To deal with ambiguities in grammar
Here’s how you might implement a listener:
public class CustomListener extends ExprBaseListener {
@Override
public void enterExpr(ExprParser.ExprContext ctx) {
System.out.println("Entering expression: " + ctx.getText());
}
}
Why Utilize Features
Taking advantage of ANTLR’s built-in features can significantly reduce development time and increase code readability. Using listeners and visitors is a structured way to separate your actions from your grammar, leading to cleaner code.
5. Skipping Testing
Testing is a vital part of software development, yet many beginners overlook it when working with ANTLR grammars.
Solution: Develop a Testing Strategy Early
Create test cases for your grammar that cover various edge cases. Test for both valid inputs (to ensure the grammar behaves as expected) and invalid inputs (to check error handling).
Simple Test Example
You can structure unit tests using a framework like JUnit:
@Test
public void testSimpleExpression() {
String input = "3 + 5";
ExprLexer lexer = new ExprLexer(CharStreams.fromString(input));
CommonTokenStream tokens = new CommonTokenStream(lexer);
ExprParser parser = new ExprParser(tokens);
Assert.assertNotNull(parser.expr());
}
Why Testing is Essential
Thorough testing ensures that any changes to your grammar or business logic will not break existing functionality. Moreover, it builds confidence in the reliability and accuracy of your parser.
Bringing It All Together
Starting with ANTLR can be gratifying, but it is crucial to avoid common pitfalls. By defining token types explicitly, simplifying grammar, implementing robust error handling, utilizing ANTLR's features, and creating a thorough testing strategy, you can create effective language parsers.
For more resources on ANTLR, I recommend checking out the ANTLR documentation and ANTLR GitHub examples. These resources will provide further insights and examples to guide your journey into language parsing. Remember, practice and patience are your best tools as you dive into the world of ANTLR.
With these tips and strategies, you can avoid common mistakes and successfully harness the power of ANTLR to create parsing solutions tailored to your needs. Happy coding!