Taming Sparse JSON: Effective Mapping Strategies Explained
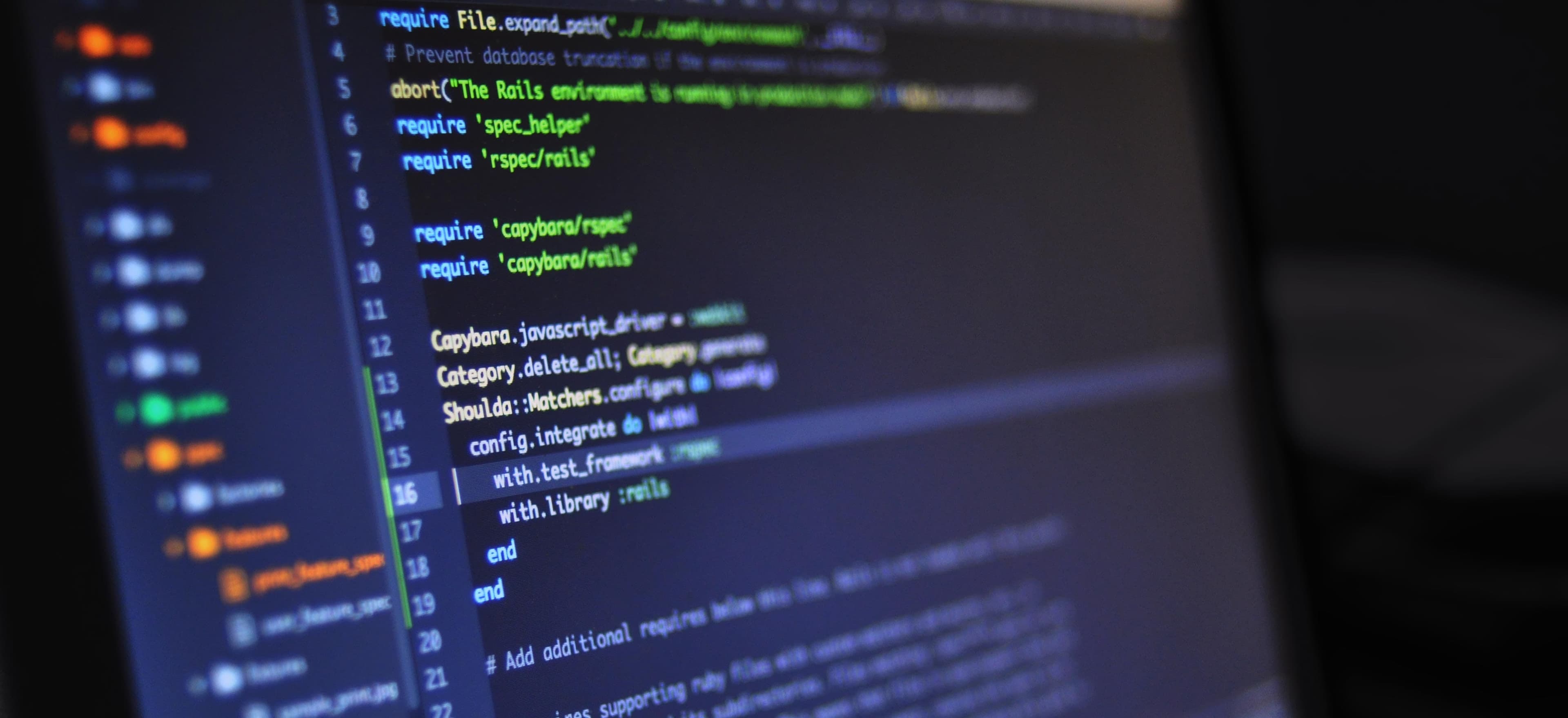
- Published on
Taming Sparse JSON: Effective Mapping Strategies Explained
In a world where data is more abundant than ever, JSON (JavaScript Object Notation) has emerged as the go-to format for exchanging data between a client and server. However, JSON can sometimes be sparse – that is, it may contain a significant number of empty fields, redundant data, or underpopulated nested structures. This article will explore effective mapping strategies for managing sparse JSON data in Java.
Understanding Sparse JSON
Sparse JSON refers to JSON objects that carry minimal or incomplete information. These objects may have:
- Many null or undefined values
- Nested data structures that are only partially filled
- Irrelevant fields that are not necessary for the intended operation
Here’s an example of a sparse JSON object:
{
"user": {
"name": "John Doe",
"age": null,
"email": "",
"address": {
"street": null,
"city": "New York",
"zip": null
}
}
}
In this example, notice that several keys are filled with either null
or empty strings. When working with such data, it's crucial to consider how to map these values effectively in your Java application.
Why Care About Sparse JSON?
Handling sparse JSON can be replete with challenges. It can lead to:
- Increased latency during data retrieval or processing
- Higher memory consumption
- Complexity in data validation and integrity checks
Addressing these issues with effective mapping strategies can significantly enhance the efficiency and readability of your code.
Best Practices for Mapping Sparse JSON in Java
1. Use Strongly Typed Data Models
Having a well-defined Java class structure can help manage sparse JSON more effectively:
public class User {
private String name;
private Integer age; // Use Integer instead of int to allow null
private String email;
private Address address;
// Getters and Setters
}
Why this matters: Using strongly typed classes allows for better data validation and handling of nulls. An optional age can be treated as absent rather than zero, leading to cleaner and more intuitive code.
2. Leverage Java Libraries
Using libraries like Jackson or Gson can streamline the JSON parsing and serialization processes. Here's how you might use Jackson to convert a JSON string to a Java object:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonMapper {
public static User mapJsonToUser(String jsonString) throws Exception {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonString, User.class);
}
}
Why Jackson? Jackson provides a simple interface to parse JSON directly into Java objects. It also has built-in support for handling null values and can ignore unknown properties, which is essential for parsing sparse JSON.
3. Utilize Annotations for Custom Serialization
Sometimes, the default behavior of libraries won’t suit your needs. Consider using annotations for customizing how fields are serialized or deserialized.
import com.fasterxml.jackson.annotation.JsonInclude;
@JsonInclude(JsonInclude.Include.NON_NULL) // Skip null values during serialization
public class User {
private String name;
private Integer age;
private String email;
private Address address;
// Getters and Setters
}
Why use annotations? Annotations like @JsonInclude
allow for more control over your serialization/deserialization process, ensuring that only meaningful data is processed and transmitted.
4. Filtering Out Unnecessary Fields
There are situations when specific fields might be irrelevant or not needed for a particular context. You can create different versions of your data models tailored for specific use cases.
public class UserSummary {
private String name;
private String email;
// Getters and Setters
}
In this scenario, if you only require the user's name and email when displaying a summary, it's efficient to represent this with a UserSummary
class.
Why filter fields? This reduces bandwidth and processing, ensuring that only necessary data is transferred and handled, which is especially critical in mobile applications or low-bandwidth situations.
5. Lazy Loading Nested Structures
Nested structures in sparse JSON can lead to complications during deserialization. A strategy to manage this is to use lazy loading for nested objects.
public class User {
private String name;
private transient Address address; // Prevent immediate loading
public Address getAddress() {
if (address == null) {
address = fetchAddress(); // Lazy load
}
return address;
}
private Address fetchAddress() {
// Code to fetch the address
}
}
Why lazy loading? This technique can significantly improve performance, especially when you're dealing with deeply nested and sparsely populated JSON objects.
A Final Look
Navigating the complexities of sparse JSON is a skill every Java developer should possess. Effective mapping strategies not only streamline the code but also promote better data integrity and a smoother user experience.
By leveraging strongly typed data models, using libraries like Jackson, filtering out unnecessary fields, and applying lazy loading principles, developers can minimize the chaos often associated with handling sparse JSON.
For further reading on mapping JSON in Java, check out Jackson's official documentation and Google's Gson library.
With the right strategies and tools, you can tame sparse JSON effectively, ensuring that your applications remain efficient, maintainable, and user-friendly.