Mastering AssertJ: Common Pitfalls in Unit Testing
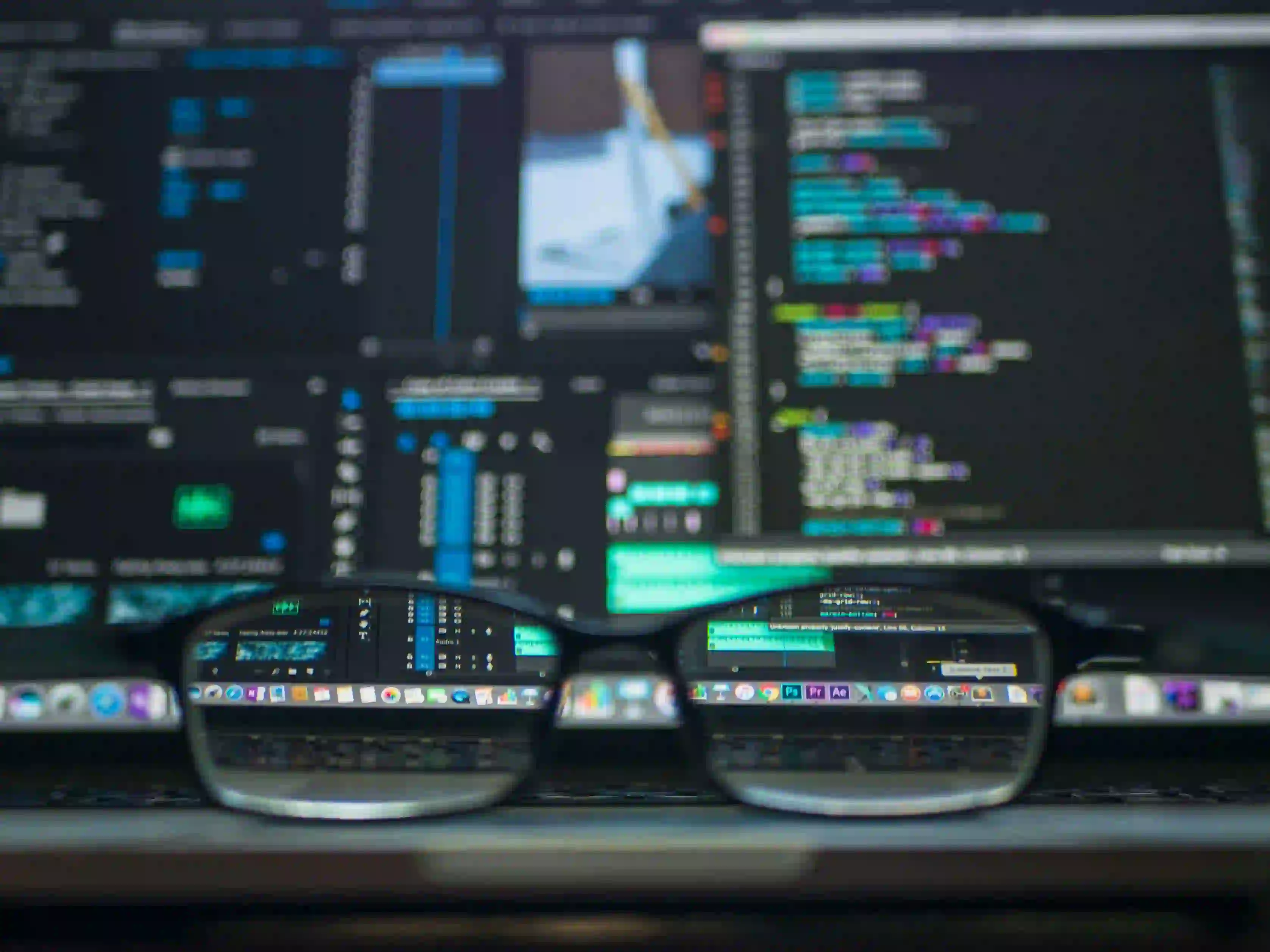
Mastering AssertJ: Common Pitfalls in Unit Testing
Unit testing is an integral aspect of software development, ensuring that individual components of your application function correctly. In Java, one of the most powerful libraries for unit testing is AssertJ. This fluent assertion library enhances readability and provides extensive features compared to traditional JUnit assertions. However, as with any tool, it's easy to fall into common pitfalls that can lead to inefficient or incorrect tests. In this blog post, we will explore some of these pitfalls and provide some insight into how to master AssertJ in your unit testing endeavors.
What is AssertJ?
AssertJ is a rich and fluent assertion library for Java. It provides a wide range of assertions that help developers write clearer and more expressive tests. The simplicity of its syntax makes unit tests easier to read and maintain.
Here's a basic example of using AssertJ:
import static org.assertj.core.api.Assertions.assertThat;
public class CalculatorTest {
@Test
public void shouldAddNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertThat(result).isEqualTo(5);
}
}
In the snippet above, we can see how AssertJ provides a fluent way to assert that the result of the addition is correct.
Common Pitfalls in Using AssertJ
1. Overusing Assertions
One common mistake when using AssertJ is overusing assertions to the point where tests become cluttered. AssertJ allows chaining assertions, but this can lead to confusion if not managed properly.
Avoid:
assertThat(result)
.isEqualTo(5)
.isGreaterThan(0)
.isLessThan(10)
.isNotNull();
Better Approach:
Instead of chaining multiple assertions on a single value, separate them for clarity:
assertThat(result).isEqualTo(5);
assertThat(result).isGreaterThan(0);
By separating assertions, you improve test readability and maintainability. If one of the assertions fails, it’s easier to identify which one.
2. Ignoring Exception Assertions
When testing for exceptions, developers often forget to assert that the correct exception is thrown. AssertJ provides a way to handle this elegantly.
Common Approach:
@Test(expected = IllegalArgumentException.class)
public void shouldThrowException() {
calculator.divide(1, 0);
}
This is a JUnit-style test that can lead to inconsistencies, especially if the expected exception isn’t thrown.
AssertJ Way:
Instead, use AssertJ to make the intent clear:
import static org.assertj.core.api.Assertions.assertThatThrownBy;
@Test
public void shouldThrowIllegalArgumentException() {
assertThatThrownBy(() -> calculator.divide(1, 0))
.isInstanceOf(IllegalArgumentException.class)
.hasMessageContaining("division by zero");
}
This approach makes the test explicit and easily understandable. The fluent style not only improves clarity but also allows for precise control over exception details.
3. Poor Use of Collections Assertions
AssertJ offers a variety of assertions for collections. When testing collections, it's critical to utilize the appropriate assertions to avoid confusion.
Inefficient Approach:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
assertThat(names).contains("Alice", "Bob", "Charlie", "David");
In this example, it’s unclear what the intent is. It checks for the existence of a name but doesn't clarify that it expects the exact contents.
Improved Approach:
Using containsExactly
to assert that the list has specific elements in the exact order improves the clarity of the test:
assertThat(names).containsExactly("Alice", "Bob", "Charlie");
This method ensures that not only the names exist but also that the order is correct.
4. Forgetting Null Checks
Another common oversight is failing to include valuable assertions related to null checks. AssertJ allows for expressive null and non-null checks.
Basic Check:
assertThat(user).isNotNull();
This is a good practice but can be improved with detailed checks:
Detailed Null Check:
assertThat(user)
.isNotNull()
.extracting("name")
.isNotNull()
.isEqualTo("Alice");
Here, we not only check if the user
object is not null, but we further investigate the properties of the object. This approach leads to more informed test scenarios.
5. Neglecting the Use of Custom Descriptions
When writing tests, it's crucial to provide context. AssertJ allows you to include custom descriptions that can help in understanding the purpose of each assertion.
Default Descriptions:
Leaving assertions without context can result in confusion when a test fails.
assertThat(result).isEqualTo(5);
Custom Descriptions:
Add meaningful descriptions to explain the intent behind each assertion:
assertThat(result).as("Sum of 2 and 3 should be 5").isEqualTo(5);
By including a description, you provide immediate context, making it easier to interpret the failure when it occurs.
Wrapping Up
Mastering AssertJ requires an understanding of both its features and potential pitfalls. The elegance of its fluent API can sometimes lead developers to overlook clarity and best practices.
By avoiding common pitfalls such as overusing assertions, ignoring exceptions, poorly using collection assertions, neglecting null checks, and not using custom descriptions, you'll be on your way to writing cleaner, more effective unit tests. AssertJ's power lies in its capability to make your tests more expressive and maintainable, ultimately leading to a more robust codebase.
For more information on unit testing in Java, you can check the official AssertJ documentation or refer to JUnit's guide for unit testing practices.
By incorporating these practices into your workflow, you will enhance your skillset in unit testing and, perhaps more importantly, improve the reliability of your applications. Happy testing!