Unlocking Guava 15: The Top 5 Features You Must Know!
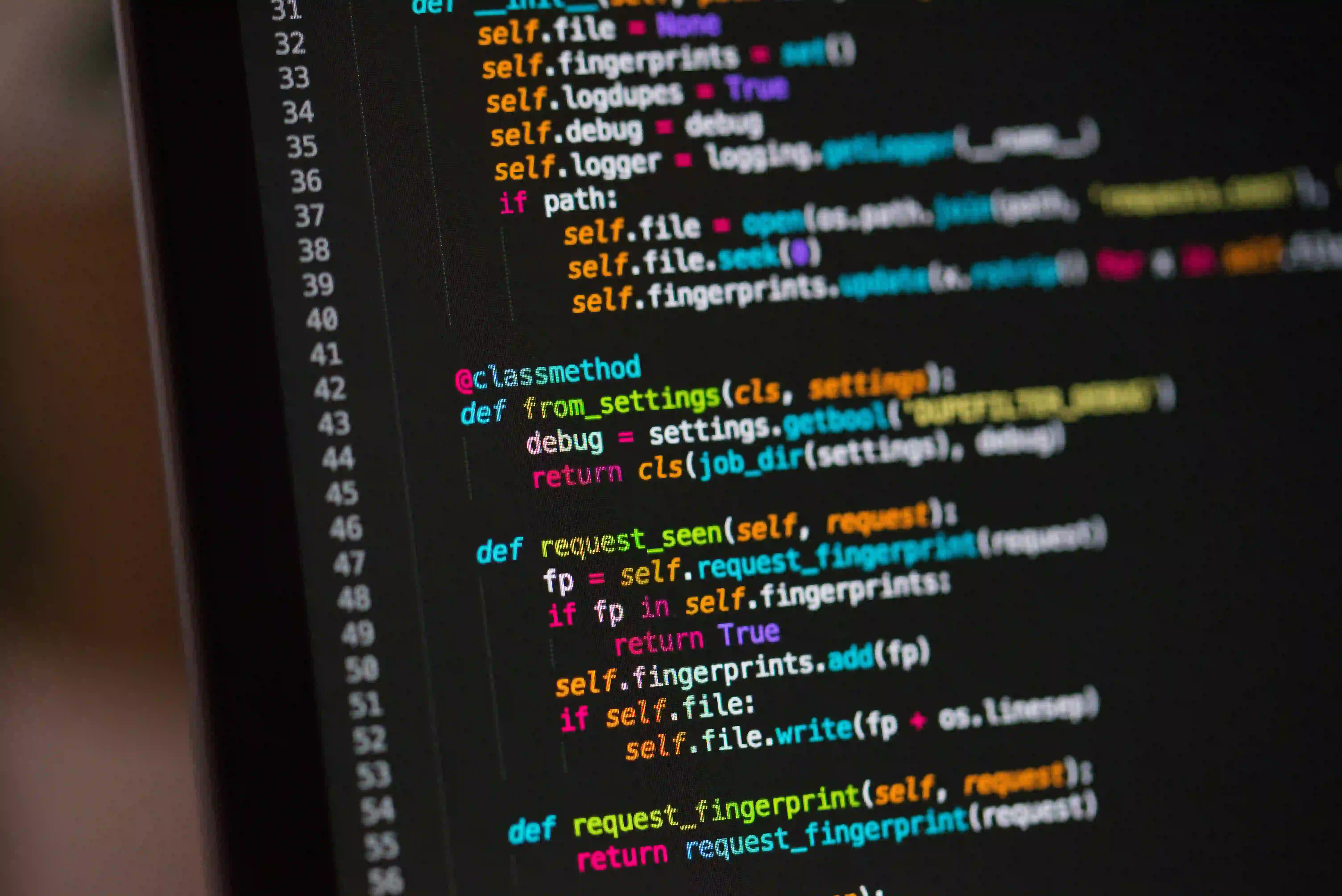
Unlocking Guava 15: The Top 5 Features You Must Know
Google's Guava library has become an essential toolkit for Java developers, providing a wealth of utilities to simplify coding tasks. In this post, we will dive into the top five features introduced in Guava 15. Understanding these features will not only improve your code quality but also make your development process more efficient.
What is Guava?
Guava is a set of core libraries developed by Google for Java. It leverages the strengths of the Java Collections framework while providing powerful utilities for collections, I/O, caching, primitives support, concurrency, and more. Guava helps developers to write cleaner and more efficient code, saving time and effort.
1. Immutable Collections
One of the standout features of Guava 15 is its enhanced support for immutable collections. Immutable collections are collections that cannot be modified after they are created. This concept might sound trivial, but the benefits it brings are profound.
Why Use Immutable Collections?
- Thread Safety: Immutable collections are inherently thread-safe, meaning they can be shared freely between threads without synchronization.
- Simplicity: They eliminate bugs related to unexpected modifications.
- Performance: They can deliver better performance for read operations.
Example
Here is how to create an immutable list using Guava:
import com.google.common.collect.ImmutableList;
public class ImmutableListExample {
public static void main(String[] args) {
ImmutableList<String> immutableList = ImmutableList.of("Apple", "Banana", "Cherry");
System.out.println(immutableList);
// Output: [Apple, Banana, Cherry]
// immutableList.add("Date"); // This line would throw an UnsupportedOperationException
}
}
In the example above, once immutableList
is created, any attempt to modify it will result in an exception. This safety feature is fundamental in concurrent applications.
2. Multiset
Guava 15 introduced the Multiset
, a collection that allows for duplicate elements. It expands on the traditional Set interface by allowing multiple occurrences of an element.
Why Use Multiset?
- Count Occurrences: You can easily count how many times an item occurs in a collection.
- Flexible Operations: Operations like
add
andremove
influence the count rather than just the presence of the item.
Example
Let's look at how to use a Multiset
:
import com.google.common.collect.HashMultiset;
import com.google.common.collect.Multiset;
public class MultisetExample {
public static void main(String[] args) {
Multiset<String> multiset = HashMultiset.create();
multiset.add("Apple");
multiset.add("Banana");
multiset.add("Apple");
System.out.println(multiset);
// Output: [Apple x 2, Banana x 1]
System.out.println("Count of Apples: " + multiset.count("Apple")); // Output: 2
}
}
The HashMultiset
showcased above allows tracking the number of duplicates effortlessly. This can be particularly useful in scenarios like counting words in a document.
3. Functional Programming Capabilities
Guava 15 introduces functional programming capabilities that align with how modern Java applications are developed. You can utilize functional interfaces and lambda expressions (introduced in Java 8) to write clearer and more concise code.
Why Embrace Functional Programming?
- Improved Readability: Makes it easier to understand the intention behind the code.
- Reduced Boilerplate: Minimizes the amount of code required for certain operations.
Example
Here's an example of using function interfaces with Guava's FluentIterable
:
import com.google.common.collect.FluentIterable;
import java.util.Arrays;
import java.util.List;
public class FunctionalOperationsExample {
public static void main(String[] args) {
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry", "Date");
List<String> filteredFruits = FluentIterable.from(fruits)
.filter(fruit -> fruit.startsWith("A"))
.toList();
System.out.println(filteredFruits);
// Output: [Apple]
}
}
In this code, FluentIterable
allows for a functional-style of filtering collections. This approach enhances clarity and expressiveness, leading to easier maintenance.
4. Cache Support
Caching plays a crucial role in applications requiring quick data retrieval. Guava 15 provides a powerful caching API, allowing developers to cache results for improved performance.
Why Use Caching?
- Improved Performance: Reduces latency for frequently accessed data.
- Simplicity: Easy to set up and use.
Example
Here’s how to implement a simple cache using Guava:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.TimeUnit;
public class CacheExample {
public static void main(String[] args) {
LoadingCache<String, String> cache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.build(new CacheLoader<>() {
@Override
public String load(String key) {
return fetchDataFromDatabase(key);
}
});
System.out.println(cache.getUnchecked("user1")); // Fetches data from the database
}
private static String fetchDataFromDatabase(String key) {
return "Data for " + key; // Simulating database fetch
}
}
This implementation of a cache is efficient, automatically invalidating entries after 10 minutes, which is crucial for maintaining up-to-date data.
5. EventBus
The Guava EventBus provides an easy way to facilitate communication between components in a decoupled manner. It serves as a mediator, allowing subscribers to receive events published by producers.
Why Use EventBus?
- Decoupling Components: Reduces direct dependencies between components.
- Simplicity: Easy to implement and use for simple event-driven designs.
Example
Here’s a simple demonstration of how to use EventBus:
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
public class EventBusExample {
static class MessageEvent {
String message;
MessageEvent(String message) {
this.message = message;
}
}
static class MessageListener {
@Subscribe
public void handleMessage(MessageEvent event) {
System.out.println("Received message: " + event.message);
}
}
public static void main(String[] args) {
EventBus eventBus = new EventBus();
MessageListener listener = new MessageListener();
eventBus.register(listener);
eventBus.post(new MessageEvent("Hello, EventBus!"));
// Output: Received message: Hello, EventBus!
}
}
In this example, the MessageListener
listens for MessageEvent
posts. The use of EventBus simplifies communication and helps to keep your classes decoupled.
Closing the Chapter
Guava 15 brings forth a myriad of powerful features that can assist Java developers in fostering optimized, cleaner, and more maintainable code. Whether you are looking for immutable collections, advanced caching strategies, or event-driven design patterns, Guava has you covered.
For additional resources on Guava, you can explore the Guava GitHub Repository and the Guava Documentation.
Incorporate these features into your next project — it could be the difference between an average application and one that excels. Happy coding!