Common Pitfalls When Using Gradle for AST Transformations
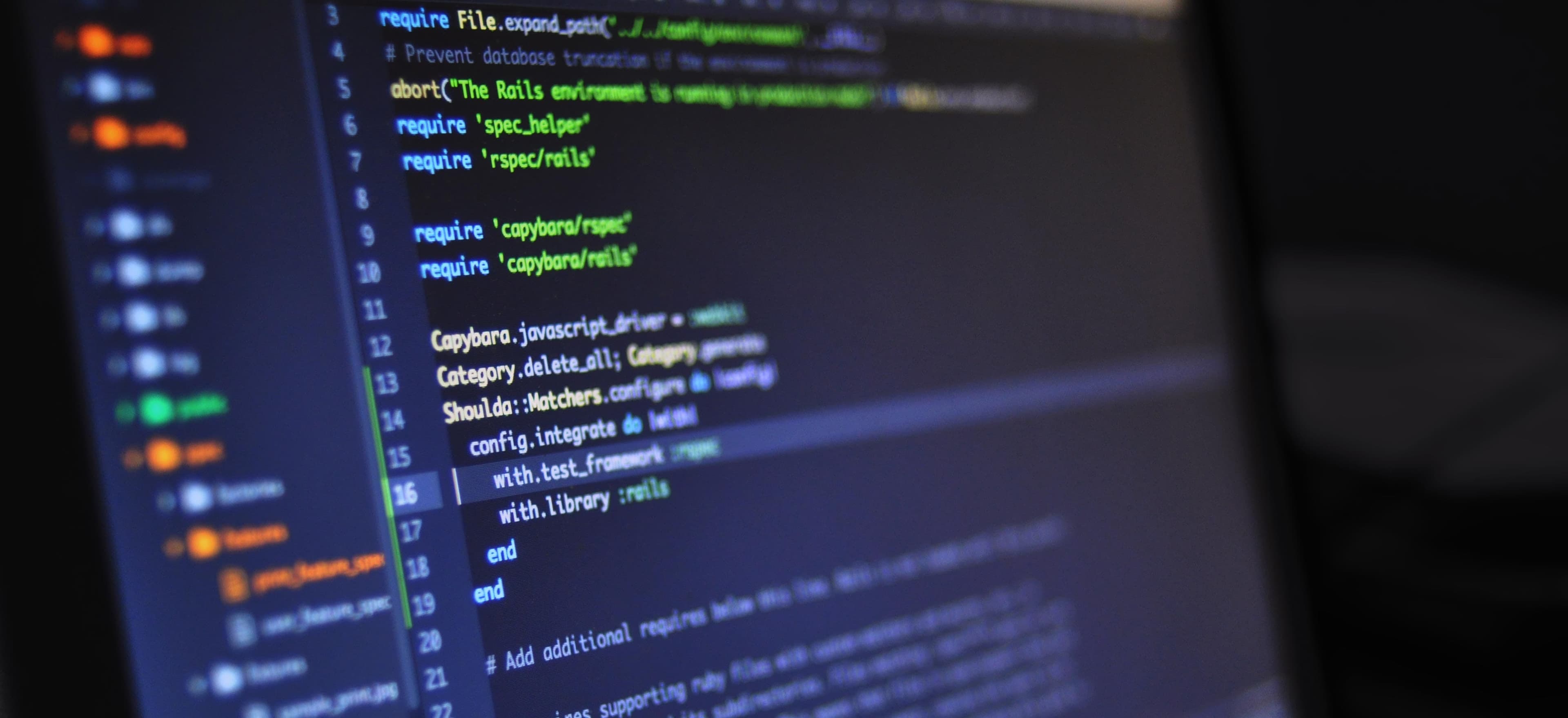
- Published on
Common Pitfalls When Using Gradle for AST Transformations
Gradle has become the go-to build automation tool for many Java developers. It is highly flexible, supports incremental builds, and integrates seamlessly with existing Java projects. However, when it comes to AST (Abstract Syntax Tree) transformations, developers often encounter several challenges. This post aims to highlight common pitfalls when using Gradle for AST transformations and provide solutions to navigate these issues effectively.
What Are AST Transformations?
AST transformations allow developers to manipulate Java source code programmatically. They involve creating a semantic representation of the structure of code—known as an abstract syntax tree—and modifying it before compile time. This technique is useful for tasks such as code generation, source code validation, and even applying design patterns more efficiently.
Why Use Gradle for AST Transformations?
Leveraging Gradle for AST transformations provides several benefits:
- Automation: Automate repetitive tasks and dependencies.
- Flexibility: Configure your build lifecycle as per specific project requirements.
- Integration: Easily integrate other tools, such as code analyzers and linters.
Despite these benefits, many developers find themselves tangled in pitfalls when trying to implement AST transformations. Let's explore these common issues and how to solve them.
Pitfall 1: Incorrect Configuration of Gradle Plugin
One of the initial stumbling blocks in using Gradle for AST transformations is improper plugin configuration. Gradle uses build plugins to extend its capabilities, but if these are not set up properly, they can lead to various issues.
Solution
Ensure that you correctly apply the required AST transformation plugins. If you are using the popular Java Plugin, your configuration should look something like this:
plugins {
id 'java'
id 'groovy' // If you're working with Groovy AST Transformations
}
Make sure to configure the sourceSets
to specify the correct source directories:
sourceSets {
main {
java {
srcDirs = ['src/main/java', 'src/main/groovy']
}
}
}
This configuration will ensure that Gradle recognizes both Java and Groovy sources for AST transformations.
Pitfall 2: Complicated Dependencies
AST transformations often require additional libraries to handle code manipulation effectively. If your dependencies are not declared correctly, you could run into issues during compilation.
Solution
Always ensure your dependencies are correctly defined in the build.gradle
file. Here’s an example of how to define dependencies:
dependencies {
compile 'org.codehaus.gpars:gpars:1.2.1'
compile 'org.apache.commons:commons-lang3:3.11'
}
One common issue arises when transitive dependencies are not resolved correctly. In such cases, consider using implementation
instead of compile
, as follows:
dependencies {
implementation 'org.codehaus.gpars:gpars:1.2.1'
implementation 'org.apache.commons:commons-lang3:3.11'
}
Using implementation
ensures that only the required classes are included in your runtime environment.
Pitfall 3: Inadequate Testing of Transformations
Testing is a critical part of any build process, but AST transformations often slip through the cracks. Developers may forget to create unit tests for their transformations or fail to validate the transformed code.
Solution
Make use of testing frameworks such as JUnit or Spock to create tests specifically for your AST transformations. Here’s an example of how you might set up a simple test for an AST transformation:
@RunWith(JUnit4.class)
public class MyASTTransformationTest {
@Test
public void testTransformation() {
// Code to set up the test.
String source = "class TestClass{}";
String transformed = MyASTTransformer.transform(source);
assertTrue(transformed.contains("public class TestClass {"));
}
}
Make sure to stress test your transformations with various input scenarios to anticipate potential edge cases.
Pitfall 4: Gradle Daemon Issues
The Gradle daemon can sometimes create an environment that does not capture changes made to the AST transformations properly. This can lead to stale builds, where the output does not reflect the latest changes in your code.
Solution
Always ensure that after significant changes, you stop the Gradle daemon to clear any cached configurations. Run the following command:
./gradlew --stop
This command stops any running Gradle daemons. You can also consider adding the following to your gradle.properties
file to disable the daemon temporarily:
org.gradle.daemon=false
Pitfall 5: Misconfigured Project Structure
When implementing AST transformations, maintaining a clean project structure is paramount. Disorganized code can result in conflicting paths, leading to compile-time errors.
Solution
Organize your project into a well-defined structure. A typical Gradle project might look like this:
my-project
├── build.gradle
└── src
├── main
│ ├── java
│ └── groovy
└── test
├── java
└── groovy
Using this structure makes it easier to locate sources and tests, reducing the likelihood of misconfigurations.
Pitfall 6: Performance Issues During Compilation
Last but not least, performance issues can arise when using Gradle for AST transformations, particularly if transformations are computationally intensive.
Solution
To avoid performance bottlenecks, consider optimizing your transformation code. Batched processing can help. Here’s a simple example illustrating this concept:
public class MyASTTransformer extends AbstractASTTransformer {
@Override
public void transformCode(CompilationUnit cu) {
cu.getTypes().forEach(type -> {
// Perform operations on the type.
optimizeType(type);
});
}
private void optimizeType(TypeDeclaration type) {
// Efficiency enhancement here.
}
}
Additionally, run builds with profiling to identify slow tasks:
./gradlew build --profile
This flag generates a report showing which tasks are consuming the most time.
Final Thoughts
Using Gradle for AST transformations can be highly effective if you remain aware of the common pitfalls. From proper plugin configuration to maintaining a consistent project structure, every small detail matters.
Make sure to test your transformations thoroughly, manage dependencies effectively, and optimize the performance of your transformations. By doing so, you not only enhance your own workflow but also make significant contributions to the project as a whole.
For further reading, check out the Gradle User Guide and the AST Transformations documentation to deepen your understanding of these topics.
Happy coding!
Checkout our other articles