Common Issues When Consuming JSON REST APIs in ADF
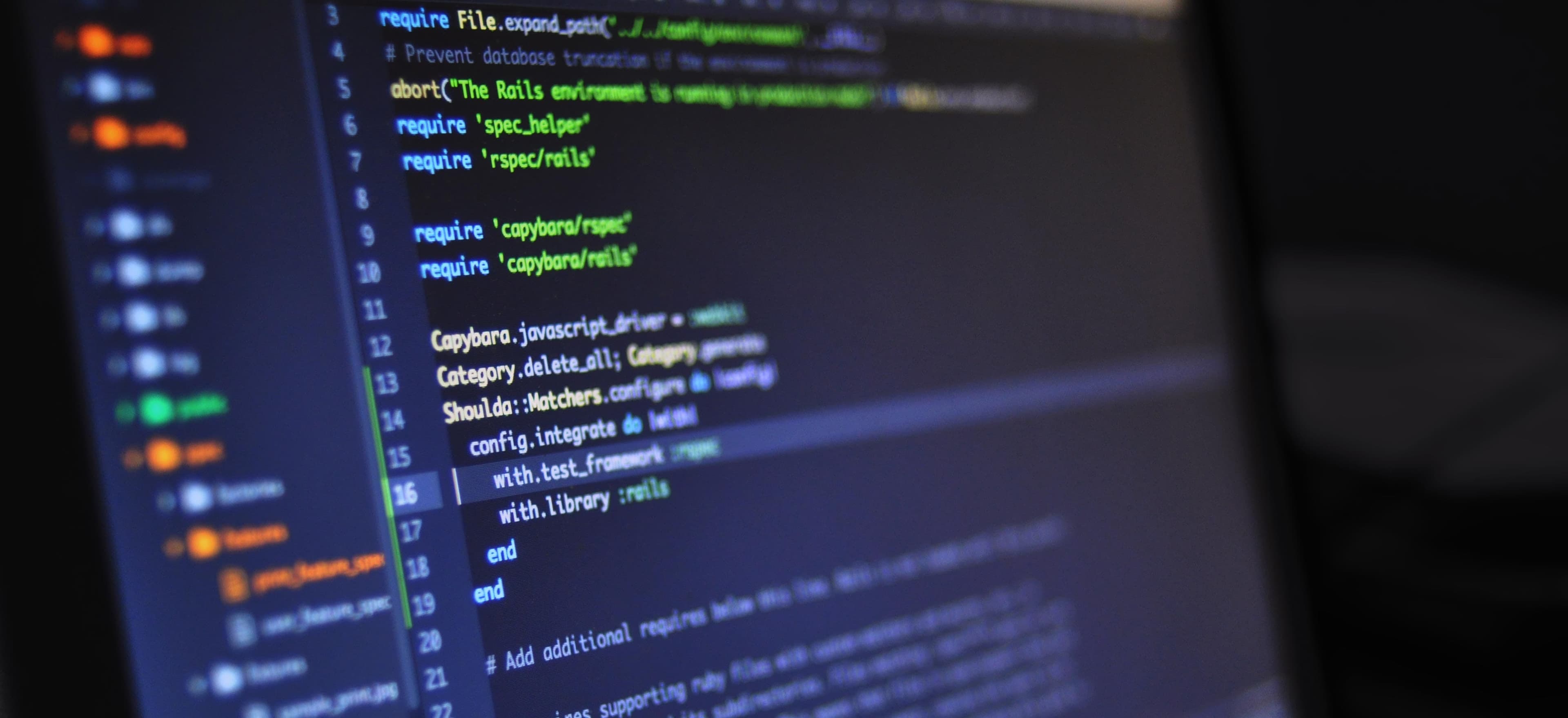
- Published on
Common Issues When Consuming JSON REST APIs in ADF
In recent years, the integration of web services has become essential for many applications. Among these services, RESTful APIs that deliver data in JSON format are the most prevalent. However, when incorporating JSON REST APIs in Oracle ADF (Application Development Framework), developers often encounter a variety of issues. In this blog post, we will explore common challenges faced while consuming JSON REST APIs in ADF and discuss effective strategies to overcome them.
Understanding ADF and JSON
Oracle ADF is a layer of Java EE-based frameworks that help developers build enterprise applications quickly. One of the key features of ADF is its ability to integrate with various data sources including RESTful APIs.
JSON (JavaScript Object Notation), being lightweight and easy to understand, serves as a standard format for data transfer between a client and server in REST APIs. While the combination of ADF and JSON API integrations can be powerful, it often leads to specific complications.
Common Issues
1. Data Mapping Challenges
When consuming a JSON REST API, one of the first challenges developers face is mapping JSON data to ADF data structures. ADF's default mechanisms may not always align with the JSON schema.
Example of JSON Data
{
"user": {
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
}
How to Handle Mapping
Implementing custom JSON data mapping can bridge the gap between JSON responses and ADF entities. The Jackson
library is commonly employed for this purpose.
Here's a simple implementation:
import com.fasterxml.jackson.databind.ObjectMapper;
public class UserMapper {
public User mapJsonToUser(String jsonString) throws IOException {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonString, User.class);
}
}
Why this works? The Jackson
library provides a flexible way to map JSON strings into Java objects seamlessly, removing boilerplate code and enabling easier maintenance.
2. Handling Nested JSON Structures
Nested JSON objects pose another significant challenge. In cases where you need to extract deeply nested information, ADF may struggle without additional configuration.
Consider a JSON response structured as follows:
{
"response": {
"status": "success",
"data": {
"user": {
"id": 1,
"profile": {
"name": "John Doe",
"age": 30
}
}
}
}
}
Solution
You can leverage custom views and entity objects that reflect the nested structure:
public class Profile {
private String name;
private int age;
// Getters and Setters
}
public class User {
private int id;
private Profile profile;
// Getters and Setters
}
Why this matters? By mapping nested objects explicitly, you gain fine control over data manipulation and enhance readability.
3. Exception Handling
REST API responses can often return errors, which requires robust error handling mechanisms in your ADF application. Improper handling of these responses can lead to app instability.
Implementing Proper Exception Handling
public void invokeApi() {
try {
// API call logic
} catch (IOException e) {
// Handle network-related errors
} catch (ApiException e) {
// Handle API-specific errors
displayErrorMessage(e.getMessage());
}
}
Why this is important? Effective error handling ensures a seamless user experience. It catches potential issues before they disrupt application flow.
4. Performance Issues Due to Large Payloads
Handling large JSON responses can lead to performance bottlenecks, affecting both loading times and responsiveness of the ADF application.
Optimize Data Loading
To mitigate this issue, consider implementing pagination in your API calls if supported. This will limit the amount of data returned in a single call.
String apiUrl = "https://api.example.com/users?page=1&limit=10";
Why use pagination? This approach minimizes data processing loads on both the API and client-side, resulting in faster performance and improved user satisfaction.
5. CORS Issues
Cross-Origin Resource Sharing (CORS) can be a significant roadblock. When your ADF application attempts to access an external JSON REST API from a different origin, it may be blocked by the browser due to security policies.
Solution to CORS Problems
You will need to set appropriate headers in your API to allow cross-origin requests. Here's what a server-side implementation in Node.js might look like:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors()); // Enable CORS for all routes
app.get('/api/users', (req, res) => {
res.json({ users: [] });
});
app.listen(3000);
Why implement CORS? Configuring CORS correctly ensures that requests to your API are respected and processed, enabling a smooth integration experience.
6. Security Concerns
When consuming external APIs, handling sensitive data securely is paramount. Failing to do so can expose vulnerabilities in your application.
Implement Security Best Practices
- Use HTTPS: Always communicate with the API over HTTPS to safeguard data integrity.
- Token Authentication: Implement OAuth2 or other token-based authentication methods.
Why prioritize security? ADF apps often handle sensitive business information. Securing API interactions protects both the application and the user.
7. Versioning of APIs
Finally, APIs often undergo updates. If your ADF application depends on a specific version of an API, a change in that API could break functionality.
Approach to Versioning
It is vital to build your application in a way that isolates API versions. Use URL versioning where possible, like so:
String apiUrl = "https://api.example.com/v1/users";
Why manage versions? By specifying versions, you ensure that changes in the API don't disrupt existing functionality, helping maintain application stability.
Closing Remarks
While consuming JSON REST APIs in ADF can be fraught with challenges, understanding these common issues and implementing proper solutions can pave the way for the successful integration of web services into your applications. From proper data mapping and error handling to considering performance optimizations and security measures, each step plays a vital role in ensuring developer efficiency and a smooth user experience.
For more insights on ADF and REST API integrations, check out Oracle ADF Documentation and RESTful API Design.
Happy Coding!
Checkout our other articles