Effortlessly Load JSON Images in Android with Http Libraries
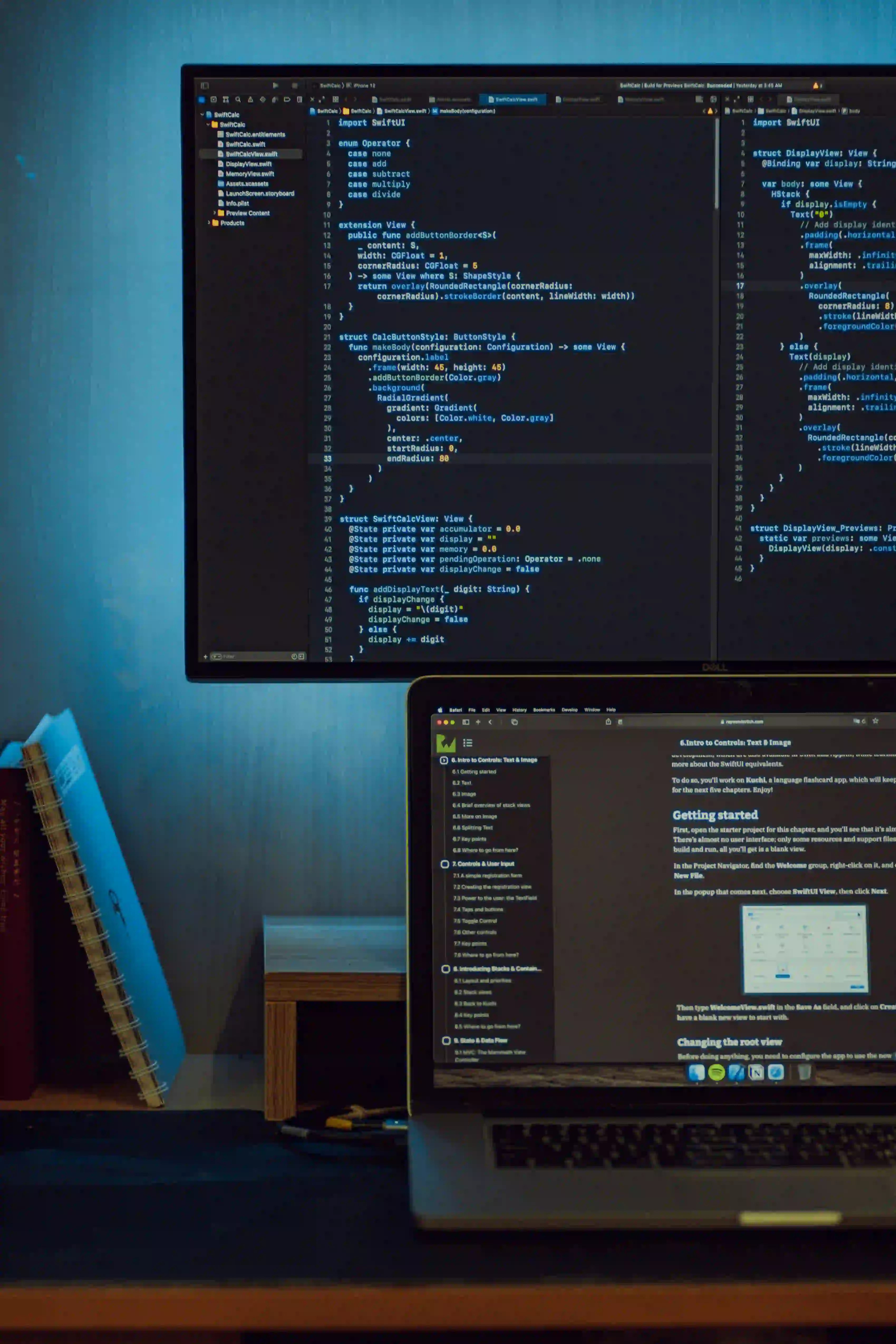
Effortlessly Load JSON Images in Android with Http Libraries
In today's mobile-first world, the need for seamless handling of image data is paramount. Whether you're building an e-commerce app, a social media platform, or any application that relies on an extensive gallery of images, your framework must handle JSON efficiently. This blog post will delve into how to effortlessly load images provided in a JSON format using popular HTTP libraries in Android.
Why JSON for Image Data?
JavaScript Object Notation (JSON) is a lightweight data interchange format that’s easy for humans to read and write and easy for machines to parse and generate. In Android development, JSON serves as a common format to exchange data with RESTful APIs. It’s simple and efficient, making it widely adopted.
Libraries at Your Disposal
When it comes to fetching and loading images in Android, there are several libraries available that simplify the process. Here are three of the most popular choices:
- Volley: An HTTP library that focuses on ease of use, performance, and flexibility.
- Glide: A fast and efficient library specifically designed for image loading and caching.
- Picasso: A powerful image downloading and caching library.
Getting Started
Before diving into code, ensure you have the necessary dependencies added in your app's build.gradle
file. We will focus on using both Glide and Volley for our example.
For Glide, add the following dependency:
implementation 'com.github.bumptech.glide:glide:4.12.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.12.0'
For Volley, include this dependency:
implementation 'com.android.volley:volley:1.2.0'
JSON Structure Example
Here’s a sample JSON structure that includes image URLs:
{
"images": [
{
"id": "1",
"url": "https://example.com/image1.jpg"
},
{
"id": "2",
"url": "https://example.com/image2.jpg"
}
]
}
Using Volley to Fetch JSON Data
Step 1: Create a Network Request
First, let's define a method to fetch the JSON data using Volley.
private void fetchImages() {
String url = "https://api.example.com/images";
JsonArrayRequest jsonArrayRequest = new JsonArrayRequest(Request.Method.GET, url, null,
new Response.Listener<JSONArray>() {
@Override
public void onResponse(JSONArray response) {
processImageData(response);
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.e("Volley Error", error.toString());
}
});
RequestQueue requestQueue = Volley.newRequestQueue(this);
requestQueue.add(jsonArrayRequest);
}
Explanation
- JsonArrayRequest: This class simplifies JSON array requests.
- processImageData(response): After successfully fetching the data, we're calling this method to handle the images.
Step 2: Process the Response
In the processImageData
method, we'll parse the JSON data and use Glide to load images.
private void processImageData(JSONArray jsonArray) {
List<String> imageUrls = new ArrayList<>();
for (int i = 0; i < jsonArray.length(); i++) {
try {
JSONObject jsonObject = jsonArray.getJSONObject(i);
String imageUrl = jsonObject.getString("url");
imageUrls.add(imageUrl);
} catch (JSONException e) {
e.printStackTrace();
}
}
loadImages(imageUrls);
}
Step 3: Load Images with Glide
The last step in this process is to display the images using Glide.
private void loadImages(List<String> imageUrls) {
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
ImageAdapter imageAdapter = new ImageAdapter(imageUrls);
recyclerView.setAdapter(imageAdapter);
}
Here is a simple adapter class:
public class ImageAdapter extends RecyclerView.Adapter<ImageAdapter.ImageViewHolder> {
private List<String> imageUrls;
public ImageAdapter(List<String> imageUrls) {
this.imageUrls = imageUrls;
}
@NonNull
@Override
public ImageViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.image_item, parent, false);
return new ImageViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ImageViewHolder holder, int position) {
String url = imageUrls.get(position);
Glide.with(holder.imageView.getContext())
.load(url)
.into(holder.imageView);
}
@Override
public int getItemCount() {
return imageUrls.size();
}
public static class ImageViewHolder extends RecyclerView.ViewHolder {
ImageView imageView;
public ImageViewHolder(@NonNull View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.imageView);
}
}
}
Why Glide?
- Caching: Glide caches images to reduce the number of network requests, making scrolling smoother.
- Performance: It automatically resizes images to fit the device's display, thereby optimizing memory consumption.
- Ease of Use: Using Glide to display images is as simple as a single line of code.
Key Takeaways
In this post, we've explored how to effortlessly load images from a JSON API in Android using Volley and Glide. The combination of these two libraries allows developers to quickly fetch, parse, and display images while ensuring smooth user experience through efficient caching.
For further insights into similar patterns, consider exploring the documentation for Glide and Volley for enhanced understanding.
Remember that optimizing networking and image loading is critical in today’s fast-paced application landscape. Implementing best practices will not only improve performance but also keep your users happy.
With that, you'll be well-equipped to handle JSON images in your Android projects effortlessly! Happy coding!