Avoid These Common Testing Scenarios in Selenium Automation
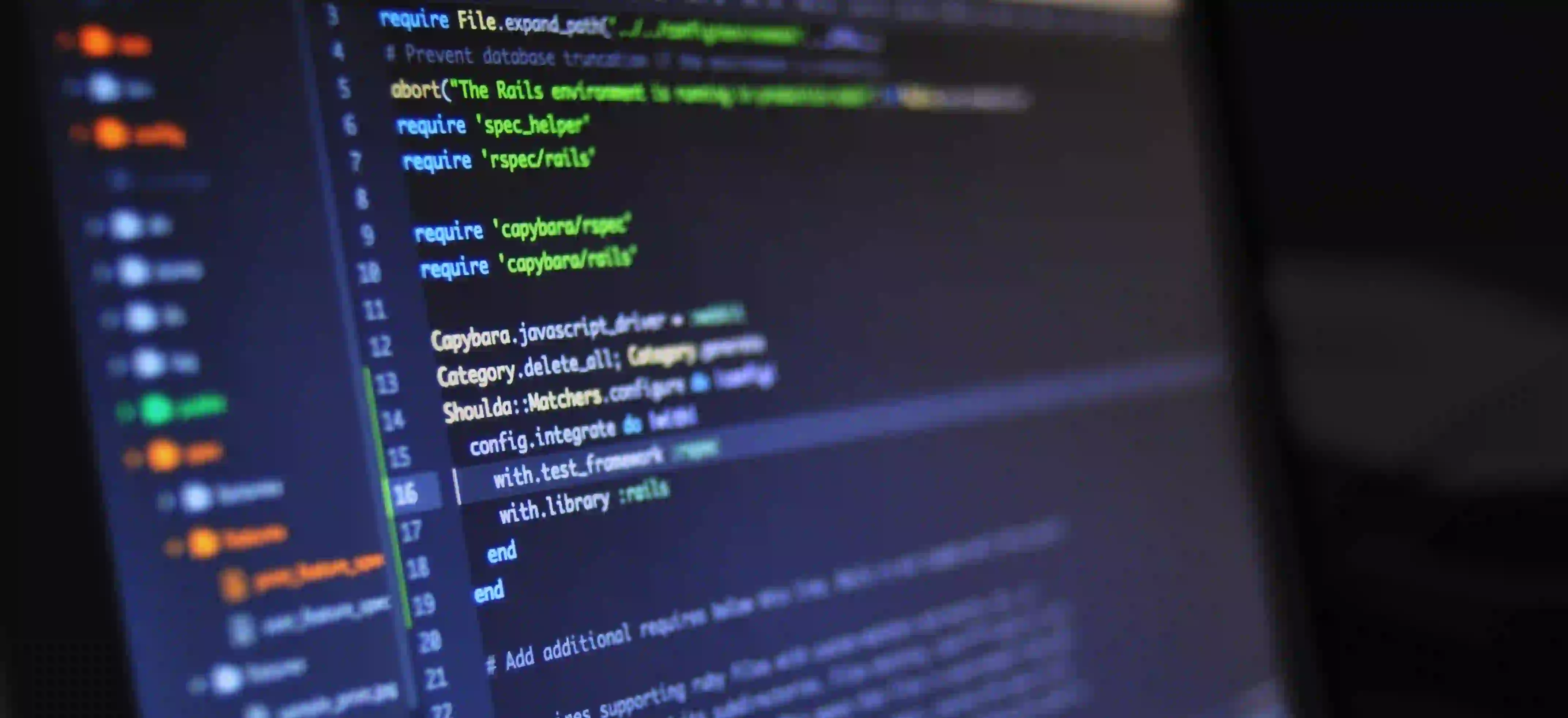
Avoid These Common Testing Scenarios in Selenium Automation
Selenium has become the go-to framework for automating web applications. However, despite its robust capabilities, many testers often encounter pitfalls in their automation efforts. This blog post will discuss common testing scenarios that can lead to problems in Selenium automation and provide insights on avoiding these issues.
Understanding Selenium Automation
Selenium is a widespread open-source tool for automating web applications. It supports various programming languages, including Java, and provides several features, such as:
- Browser Compatibility: Selenium supports multiple browsers, including Chrome, Firefox, and Safari.
- Language Support: Developers can write tests in languages like Java, C#, Ruby, and Python.
- Flexibility: Selenium integrates seamlessly with other tools and frameworks for enhanced functionality.
However, improper use of Selenium can lead to flaky tests, increased maintenance, and longer test execution time.
Common Testing Scenarios to Avoid
1. Hard-Coded Wait Times
Many testers fall into the trap of using hard-coded wait times in their Selenium scripts to handle asynchronous loading.
// Poor practice: using Thread.sleep
Thread.sleep(5000); // Wait for 5 seconds
Why to Avoid This: Hard-coded waits can lead to unnecessarily long test runs and do not adapt well to different network conditions. If elements load faster than expected, the test waits unnecessarily. Conversely, if they take longer, the test could fail.
Better Approach: Use WebDriver's implicit or explicit waits.
// Using WebDriverWait for dynamic wait
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Benefits: This ensures that the script only proceeds once the element is available, improving efficiency and reliability.
2. Ignoring Element Interactions Timing
Another common mistake is ignoring the timing of web element interactions, such as clicking buttons or entering text.
// Ignoring whether the element is clickable
driver.findElement(By.id("submitButton")).click();
Why to Avoid This: If the element is not interactable at the time of the action, it can lead to an exception or flaky tests.
Better Approach: Use WebDriverWait to ensure the element is both visible and clickable before interacting with it.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement button = wait.until(ExpectedConditions.elementToBeClickable(By.id("submitButton")));
button.click();
Benefits: This improves the stability of your tests, significantly reducing false negatives.
3. Not Using Page Object Model (POM)
Failing to implement the Page Object Model (POM) is a common mistake among testers using Selenium.
Why to Avoid This: Not using POM can lead to cumbersome and unmanageable code. If you need to update locators, you end up modifying the test scripts instead of a central class. This also leads to duplicated code.
Better Approach: Implement POM where each page of your application has an associated class.
public class LoginPage {
private WebDriver driver;
@FindBy(id = "username")
private WebElement usernameField;
@FindBy(id = "password")
private WebElement passwordField;
@FindBy(id = "loginButton")
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
loginButton.click();
}
}
Benefits: With POM, your tests become more structured, maintainable, and reusable, saving time and effort as your test suite grows.
4. Neglecting Error Handling
Neglecting to implement proper error handling can lead to unexpected failures and untraceable issues.
driver.findElement(By.id("nonExistentElement")).click();
Why to Avoid This: If the element is not found, the script will throw an exception and stop execution without giving you context on the error.
Better Approach: Use try-catch blocks to handle exceptions gracefully.
try {
driver.findElement(By.id("nonExistentElement")).click();
} catch (NoSuchElementException e) {
System.out.println("Element not found: " + e.getMessage());
}
Benefits: Implementing error handling allows for better debugging and makes your tests robust against unexpected conditions.
5. Overlooking Browser-Specific Features
Not accounting for browser-specific features can lead to inconsistencies in test results.
Why to Avoid This: Different browsers may implement web standards in slightly different ways. Relying on features not universally supported can lead to failures in specific browsers.
Better Approach: Test on multiple browsers and ensure compatibility for critical features.
// Example of switching to fireFoxDriver and ChromeDriver
WebDriver driver = new ChromeDriver(); // or new FirefoxDriver();
Benefits: You ensure that your application behaves consistently across different environments, which is essential for user experience.
6. Ignoring Test Data Management
Not managing test data can lead to tests that are interdependent and unpredictable.
Why to Avoid This: Hard-coded test data or not sanitizing data can result in unreliable tests that may pass or fail depending on the previous state.
Better Approach: Use external data sources or frameworks like TestNG's data providers or properties files.
@DataProvider(name="loginData")
public Object[][] loginDataProvider() {
return new Object[][] {
{"user1", "pass1"},
{"user2", "pass2"},
};
}
// Using test data in a test method
@Test(dataProvider="loginData")
public void testUserLogin(String username, String password) {
LoginPage loginPage = new LoginPage(driver);
loginPage.login(username, password);
}
Benefits: This promotes reusability. It also makes your tests clearer and consistent.
7. Not Writing Assertions
Failing to include assertions can lead to situations where tests pass without validating outcomes.
// No assertion made
driver.findElement(By.id("submitButton")).click();
Why to Avoid This: Simply clicking a button does not confirm that the next page loaded correctly or desired outcomes occurred.
Better Approach: Always follow actions with assertions to validate outcomes.
Assert.assertTrue(driver.getTitle().contains("Expected Title"), "Title did not match!");
Benefits: This ensures that your test not only runs but also validates that it met the expected conditions.
Bringing It All Together
By avoiding these common scenarios in Selenium automation, you can significantly improve the quality and reliability of your tests. Remember to focus on best practices, maintainability, and flexibility as your test suite evolves.
For more insights into Selenium automation techniques, check out SeleniumHQ or Test Automation University.
By following the principles outlined here, you will contribute to a more robust testing environment and help ensure your web applications maintain high standards of quality.
Happy Testing!