Mastering Range Types in Java: Common Pitfalls Explored
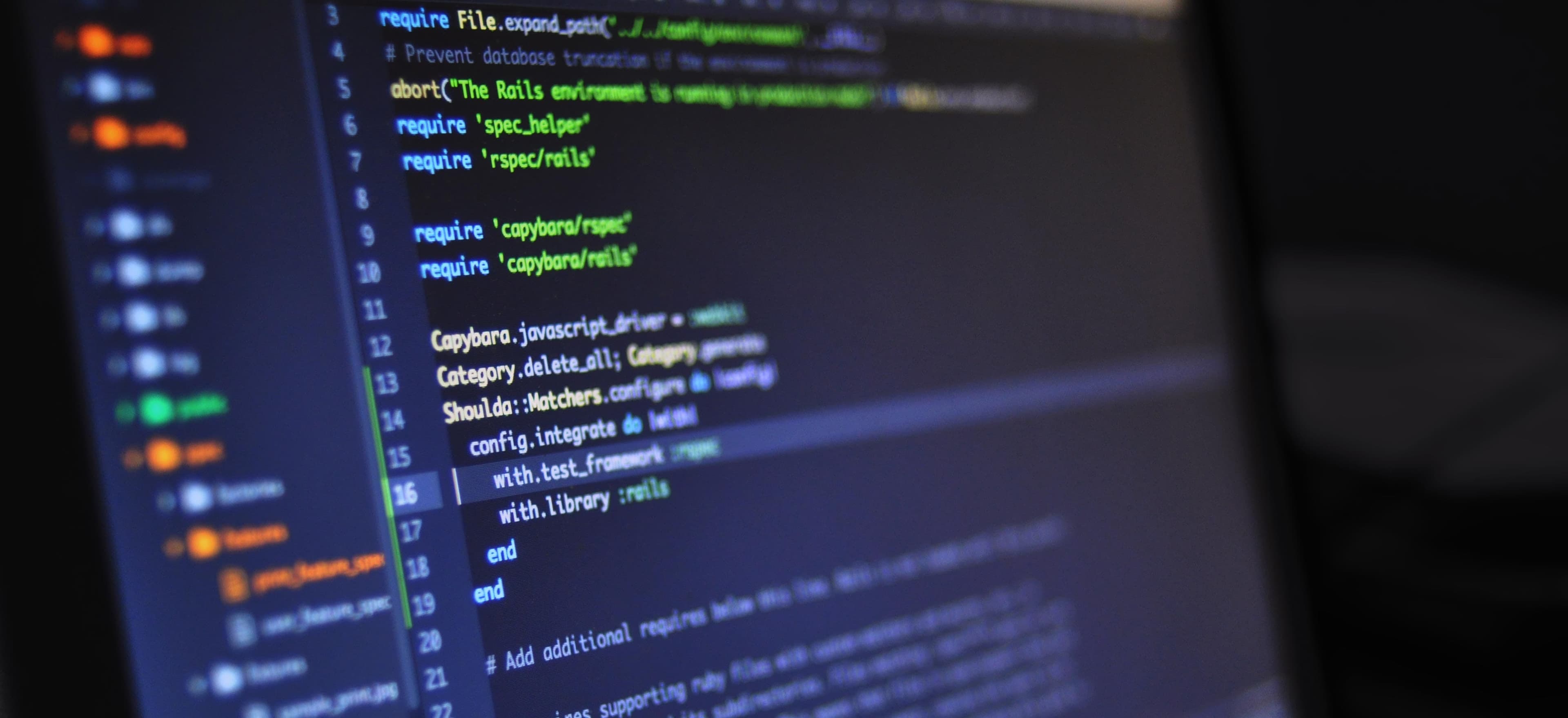
- Published on
Mastering Range Types in Java: Common Pitfalls Explored
Java has long been a bastion of strong typing and explicit behaviors. However, complexities sometimes arise, particularly in how we handle ranges. In today's post, we will delve deep into range types in Java, investigate common pitfalls, and arm you with the knowledge to avoid these issues.
What Are Range Types?
In programming, a "range" typically represents a set of values between a starting point and an endpoint. Java does not have a built-in range type, but we can effectively implement ranges using classes like IntStream
or by creating our custom classes.
Why Use Range Types?
Using range types can:
- Simplify your code by avoiding repetitive boundaries.
- Increase readability, making it easier for others (and your future self) to understand your intentions.
- Provide enhanced control over value checks, especially in algorithms where boundaries matter.
Basic Implementation
Let’s first look at a simple range implementation using custom classes.
public class NumberRange {
private final int start;
private final int end;
public NumberRange(int start, int end) {
if (start > end) {
throw new IllegalArgumentException("Start must be less than or equal to end.");
}
this.start = start;
this.end = end;
}
public boolean includes(int value) {
return value >= start && value <= end;
}
@Override
public String toString() {
return "Range: [" + start + ", " + end + "]";
}
}
Commentary
This code snippet defines a basic NumberRange
class with:
- A constructor that checks constraints (ensures the start is less than or equal to the end).
- A method
includes
to check if a number falls within the defined range.
Common Pitfall 1: Inclusive vs. Exclusive Ranges
One of the first pitfalls is confusing inclusive and exclusive ranges. In programming, an inclusive range means both endpoints are part of the range (e.g., [1, 10]), while an exclusive range does not include the endpoints (e.g., (1, 10)).
Example of Confusion
NumberRange range = new NumberRange(1, 10);
System.out.println(range.includes(1)); // true
System.out.println(range.includes(10)); // true
In this case, our NumberRange
is inclusive by design. If you needed it to be exclusive, you would modify the includes
method:
public boolean includesExclusive(int value) {
return value > start && value < end;
}
Common Pitfall 2: Off-by-One Errors
Off-by-one errors are notorious in programming. When defining ranges, it's essential to ensure your code correctly includes or excludes boundaries to avoid these pitfalls.
Consider the following example:
for(int i = 0; i <= 100; i++) {
if (range.includes(i)) {
System.out.println(i);
}
}
The loop includes 100
when your intention was to only include values from 0
to 99
. To avoid this, ensure your range definitions match the problem domain you’re working within.
Using Streams for Ranges
Java Streams introduced the IntStream
class, which can manage ranges with ease.
Here’s how to print even numbers in a range:
IntStream.rangeClosed(1, 10)
.filter(i -> i % 2 == 0)
.forEach(System.out::println); // Prints: 2, 4, 6, 8, 10
Common Pitfall 3: Off-By-One in Streams
When using IntStream.range()
, it's critical to understand that it is exclusive of the endpoint.
IntStream.range(1, 10) // This is [1, 2, ..., 9]
.forEach(System.out::println);
In contrast, if you want it inclusive, use IntStream.rangeClosed()
.
Even More Pitfalls: Empty Ranges
Handling empty ranges can also lead to issues. If you create a range from a larger number to a smaller number, this unexpected case could lead to runtime exceptions.
NumberRange range = new NumberRange(10, 1);
To prevent this, always validate your input ranges before using them.
A Final Look
Mastering range types in Java adds a level of robustness and clarity to your coding practices. However, it is essential to be mindful of common pitfalls such as understanding inclusive vs. exclusive boundaries, avoiding off-by-one errors, and validating range input to remain in control of your data flow.
Further Reading
For more insights into effective ranges in Java, consider checking out these resources:
- Oracle Java Documentation on Streams
- Effective Java by Joshua Bloch, particularly Section on Generics
- Java Design Patterns - Range
By employing these lessons and resources, you will elevate your Java programming skills to a new level, avoiding pitfalls along the way. Happy coding!
Checkout our other articles