Common Pitfalls When Writing Your First Spring Cloud Microservice
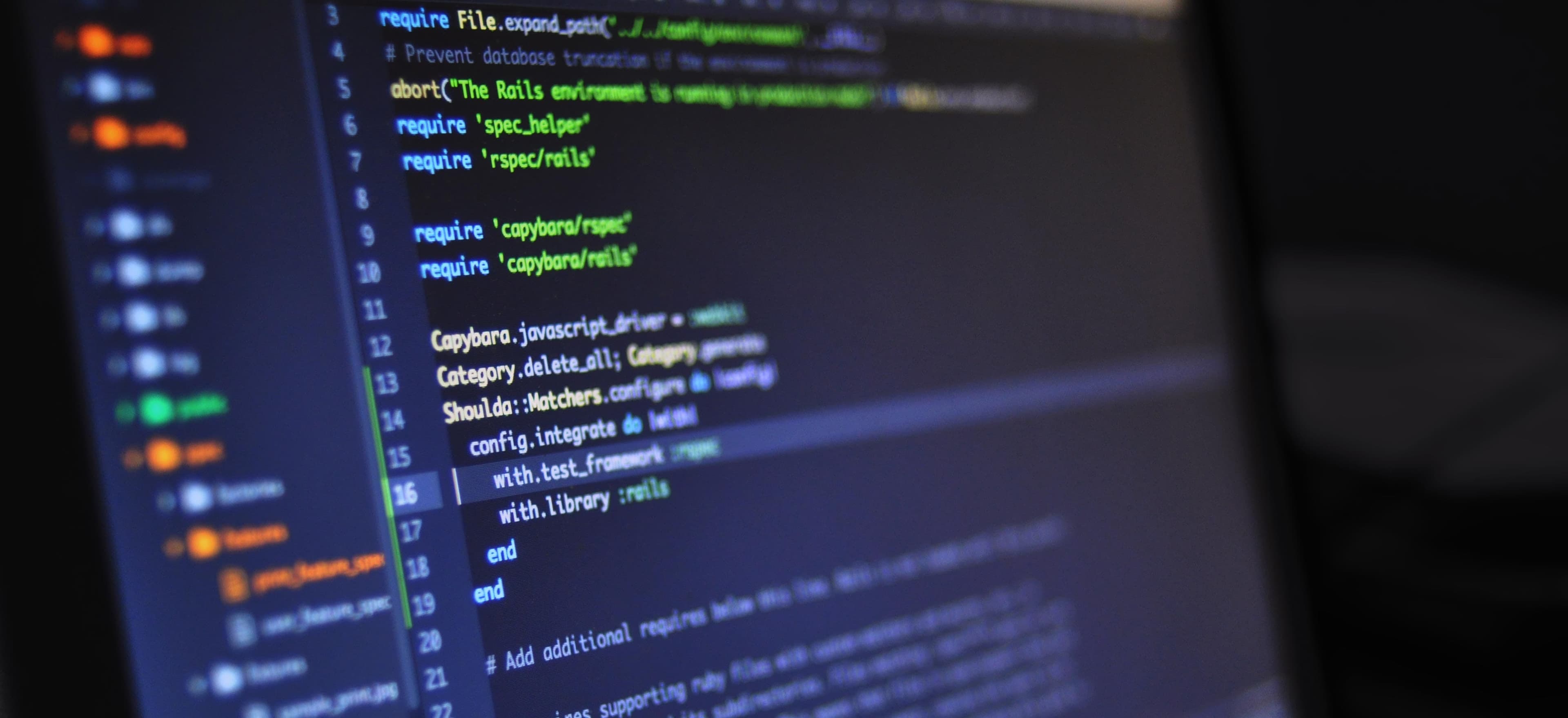
- Published on
Common Pitfalls When Writing Your First Spring Cloud Microservice
Building microservices is an exciting journey, especially with Spring Cloud, which significantly simplifies the development of distributed systems. However, new developers often make common mistakes that can lead to difficulties in deployment, maintenance, and scalability. In this blog post, we'll explore these pitfalls and provide guidance on how to avoid them.
1. Not Understanding Microservices Architecture
The first and perhaps the most fundamental mistake is not fully comprehending what microservices architecture entails. Microservices emphasize decentralized management, scalability, and modularity. Here are a few common misinterpretations:
-
Microservices are not just small services: It's crucial to realize that the size of a service is not what defines a microservice. Instead, it's about the service's autonomy, domain-driven design, and how it integrates with other services in an ecosystem.
-
Microservices require robust communication mechanisms: Relying on synchronous calls between services can lead to latency issues. As a solution, consider employing asynchronous methods, such as message queues.
Example of Asynchronous Communication in Spring Cloud
import org.springframework.cloud.stream.annotation.EnableBinding;
import org.springframework.cloud.stream.messaging.Source;
import org.springframework.messaging.support.MessageBuilder;
@EnableBinding(Source.class)
public class MessageProducer {
private final Source source;
public MessageProducer(Source source) {
this.source = source;
}
public void sendMessage(String payload) {
source.output().send(MessageBuilder.withPayload(payload).build());
}
}
In the code above, we enable binding to Spring Cloud Stream and create a method to send messages asynchronously. This helps to decouple services by minimizing direct dependencies.
2. Ignoring Configuration Management
Microservices come with a new challenge concerning configuration management. Each microservice often has unique configurations. Neglecting to manage these configurations properly can lead to hard-to-trace issues.
Best Practices for Configuration Management
- Use Spring Cloud Config: Centralizing configuration with Spring Cloud Config Server allows you to manage application settings for multiple services efficiently.
- Profile-Specific Configurations: Utilize profiles to provide different configurations based on the environment (development, testing, production).
Example of Spring Cloud Config Server
In your application.yml
, you can define the config server properties:
spring:
cloud:
config:
server:
git:
uri: https://github.com/your-org/config-repo
Using a Git repo for storing configurations not only enables version control but also promotes centralization.
3. Improper Service Registration and Discovery
When dealing with microservices, service registration and discovery become vital—a common pitfall is neglecting to implement these features correctly. A misconfigured Eureka server can lead to issues where services cannot discover each other, causing cascading failures.
Configuring Eureka for Service Discovery
Add the following dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
Next, annotate your main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Starting the Eureka server allows microservices to register themselves. Ensure all services are configured to register with the appropriate Eureka server URL.
4. Neglecting API Gateway Configuration
Another common mistake is underestimating the importance of API gateways in a microservices architecture. An API gateway serves as the single endpoint for clients, helping to reduce complexity by providing features like request routing, load balancing, and authentication.
Implementing Spring Cloud Gateway
Add the Spring Cloud Gateway dependency:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
Here's how to configure the gateway in your application.yml
:
spring:
cloud:
gateway:
routes:
- id: user-service
uri: lb://USER-SERVICE
predicates:
- path=/users/**
The above configuration routes incoming requests to the appropriate service based on the path specified.
5. Inadequate Error Handling
Microservices introduce various points of failure, and proper error handling mechanisms are often overlooked. Without effective error handling, failures in one service can cascade across the entire system.
Implementing Circuit Breaker with Resilience4j
Consider using a circuit breaker pattern with Resilience4j. First, include the dependency:
<dependency>
<groupId>io.github.resilience4j</groupId>
<artifactId>resilience4j-spring-boot2</artifactId>
</dependency>
Next, annotate your service method:
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker;
@Service
public class UserService {
@CircuitBreaker
public User getUser(String userId) {
// Call to external service which may fail
}
}
The circuit breaker will help prevent the application from continuously attempting to call a failing service, thus preserving resources and improving overall reliability.
6. Failing to Monitor and Log
Monitoring and logging are critical components of any microservices architecture. Without proper monitoring, you can't effectively troubleshoot issues or understand performance metrics.
Implementing Spring Boot Actuator
Add the Actuator dependency to your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
This will provide rich information about your microservice's metrics and health, helping you maintain performance and stability:
management:
endpoints:
web:
exposure:
include: "*"
Connect your microservices to centralized logging solutions like ELK Stack or Grafana for effective visibility across services.
7. Overcomplicating Deployment Strategies
Finally, don't complicate your deployment process. Using tools like Docker and Kubernetes can simplify deployment and scaling. However, trying to implement a cloud-native infrastructure from scratch before mastering microservices can lead to unnecessary complexity.
Containerizing a Spring Boot Application
Here’s a simple Dockerfile to containerize your Spring Boot application:
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/myapp.jar myapp.jar
ENTRYPOINT ["java", "-jar", "/myapp.jar"]
This Dockerfile defines how to assemble your Spring Boot project into a Docker container. It allows for easy deployment in different environments without modifying the application code.
The Last Word
Creating your first Spring Cloud microservice offers numerous challenges, but understanding common pitfalls can significantly ease the journey. From grasping microservices architecture to ensuring effective monitoring and logging, the potential for innovation is immense.
Key Takeaways:
- Understand the core principles of microservices.
- Implement proper configuration management using Spring Cloud Config.
- Use service discovery and API gateways appropriately.
- Integrate error handling strategies such as Circuit Breakers.
- Monitor and log effectively with tools like Spring Boot Actuator.
- Simplify deployment processes.
As you embark on your microservices journey, remember that the goal is not just to build services but to create robust, maintainable, and scalable applications. Stay curious and continue exploring the rich ecosystem of Spring Cloud—your future self will thank you!
For more information on Spring Cloud, consider checking the Spring Cloud documentation. Happy coding!
Checkout our other articles