Resolving Dependency Conflicts in MicroProfile and Jakarta EE
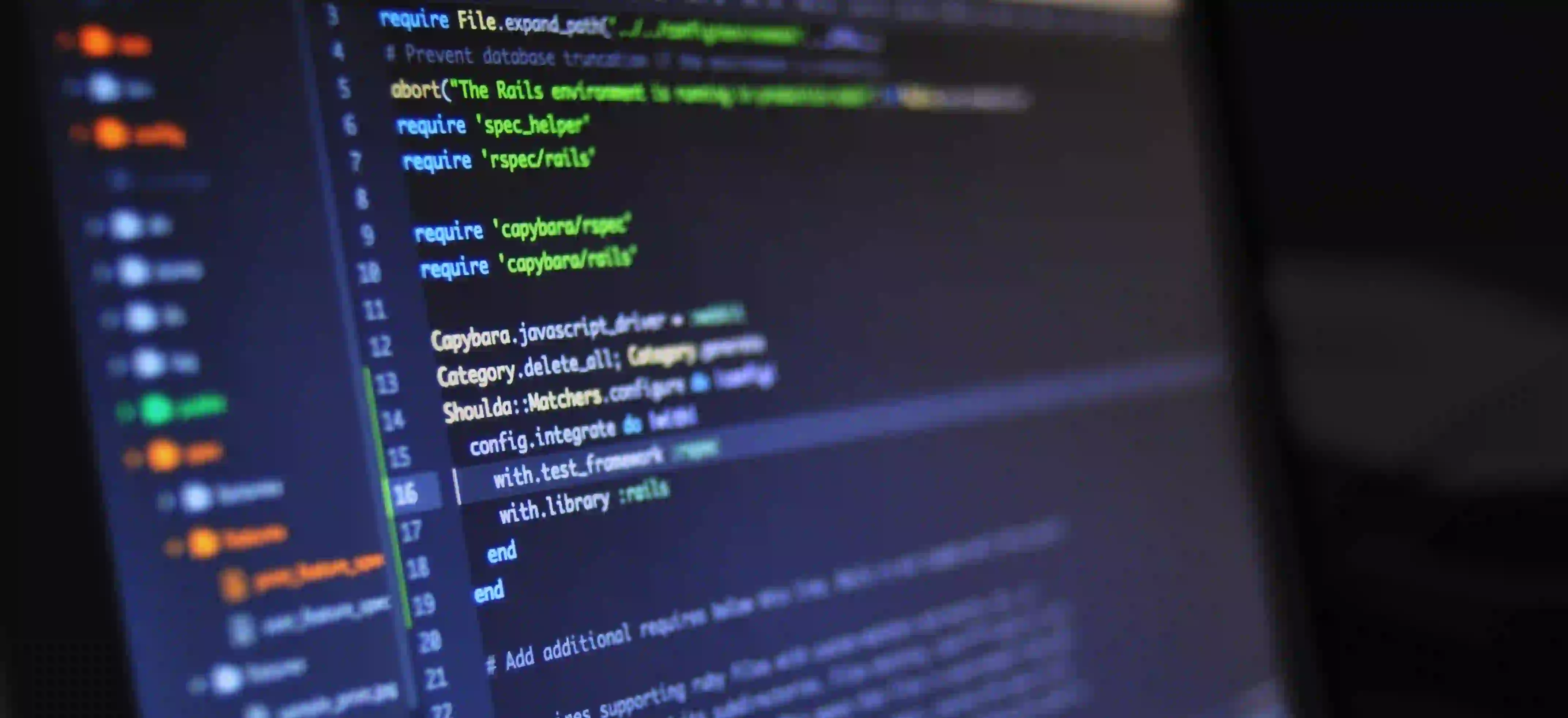
Resolving Dependency Conflicts in MicroProfile and Jakarta EE
In modern Java development, one of the most significant challenges developers face is managing dependencies, particularly in large-scale applications built using MicroProfile and Jakarta EE. Dependency conflicts can lead to runtime issues, class loading problems, or unexpected behaviors that can derail a project's success. In this blog post, we’ll explore ways to effectively resolve these conflicts and ensure that your Java applications are stable and efficient.
Understanding MicroProfile and Jakarta EE
Before diving into resolving dependency conflicts, let’s clarify what MicroProfile and Jakarta EE are.
MicroProfile is an open-source initiative that optimizes enterprise Java for microservices architecture. It builds upon existing Java EE (now Jakarta EE) technologies, providing a set of APIs and specifications targeted for microservices.
On the other hand, Jakarta EE is the evolution of Java EE, which has transitioned under the Eclipse Foundation. It emphasizes a more modular approach, aiming to make enterprise Java lightweight and scalable for modern applications.
Why Dependency Management Matters
Proper management of dependencies is crucial in enterprise applications due to several reasons:
- Conflicting libraries: Different libraries may rely on different versions of the same library, leading to conflicts.
- Performance issues: Redundant dependencies can inflate the size of your application, reducing performance.
- Security vulnerabilities: Outdated libraries may leave your application open to security exploits.
When developing with MicroProfile or Jakarta EE, it’s essential to have a strategy in place to handle these issues.
Common Causes of Dependency Conflicts
Version Mismatches
With multiple libraries often depending on specific versions of shared dependencies, it is not unusual to encounter version mismatches. For instance:
- If Library A requires version 1.0 of a dependency, and Library B requires version 2.0, you may end up with conflicts if your application uses both.
Transitive Dependencies
A primary library might pull in its own dependencies, which can also lead to conflicts. This phenomenon is known as transitive dependencies. For example:
<dependency>
<groupId>com.example</groupId>
<artifactId>libraryA</artifactId>
<version>1.0</version>
</dependency>
<dependency>
<groupId>com.example</groupId>
<artifactId>libraryB</artifactId>
<version>2.0</version>
<exclusions>
<exclusion>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
</exclusion>
</exclusions>
</dependency>
In this code snippet, we see an exclusion of commons-lang3
from libraryB
. This approach helps in avoiding transitive dependency conflicts but must be handled carefully to ensure that needed functionality isn't omitted.
Strategies for Resolving Dependency Conflicts
1. Dependency Management
Both Maven and Gradle provide mechanisms to enforce dependency versions across your project. You can define a dependencyManagement
section in Maven or set up a resolutionStrategy
in Gradle.
For Maven:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
</dependencyManagement>
In Gradle:
configurations.all {
resolutionStrategy {
force 'org.apache.commons:commons-lang3:3.12.0'
}
}
2. Understanding and Reviewing Dependencies
Use tools to visualize your dependency tree in both Maven and Gradle projects. These tools can help identify where conflicts arise:
- For Maven, use
mvn dependency:tree
. - For Gradle, use
./gradlew dependencies
.
Understanding how your libraries relate to one another is key to resolving conflicts effectively.
3. Update and Align Versions
One effective strategy is to ensure that you are using the latest versions of your dependencies. Each new release usually contains not only new features but fixes for known issues, including vulnerabilities and bugs.
4. Minimizing Direct Dependencies
Where possible, prefer using well-maintained libraries that come with fewer dependencies. For instance, if using MicroProfile Rest Client, check if there are lightweight alternatives aligned with similar functions but fewer transitive dependencies.
5. Exclusions
As shown in the earlier section, selectively excluding transitive dependencies can prevent conflicts. However, this oversight must be considered carefully so as not to remove essential functionalities.
Example: Resolving Conflict in MicroProfile Rest Client
Let’s consider a scenario where a conflict arises between two versions of an HTTP client required by two different libraries. Assume libraryA
is updated to include an HTTP client, and libraryB
rolls back to an older version due to compatibility issues.
Here is the code snippet addressing this conflict:
<dependencies>
<dependency>
<groupId>org.eclipse.microprofile.rest.client</groupId>
<artifactId>microprofile-rest-client-api</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
<exclusions>
<exclusion>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpcore</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
In the snippet above, we are connecting the MicroProfile REST Client with a specific version of the httpclient
. We also excluded httpcore
which can lead to compatibility issues.
The Last Word
Dependency conflicts in MicroProfile and Jakarta EE applications can arise from various scenarios, such as version mismatches or issues with transitive dependencies. Through effective dependency management, visualization tools, judicious use of exclusions, and alignment of library versions, developers can mitigate most challenges.
It's essential to stay updated with the latest library versions as the ecosystem continuously evolves. For more intricate needs and to keep abreast with best practices, be sure to consult the Eclipse MicroProfile and Jakarta EE documentation for updates and thorough guidelines.
By taking these steps, you can ensure your Java applications run smoothly and are primed for the demands of modern development. Always remember, proactive dependency management is the key to a trouble-free microservices architecture. Happy coding!