Conquering the Frustration of Non-Reproducible Bugs
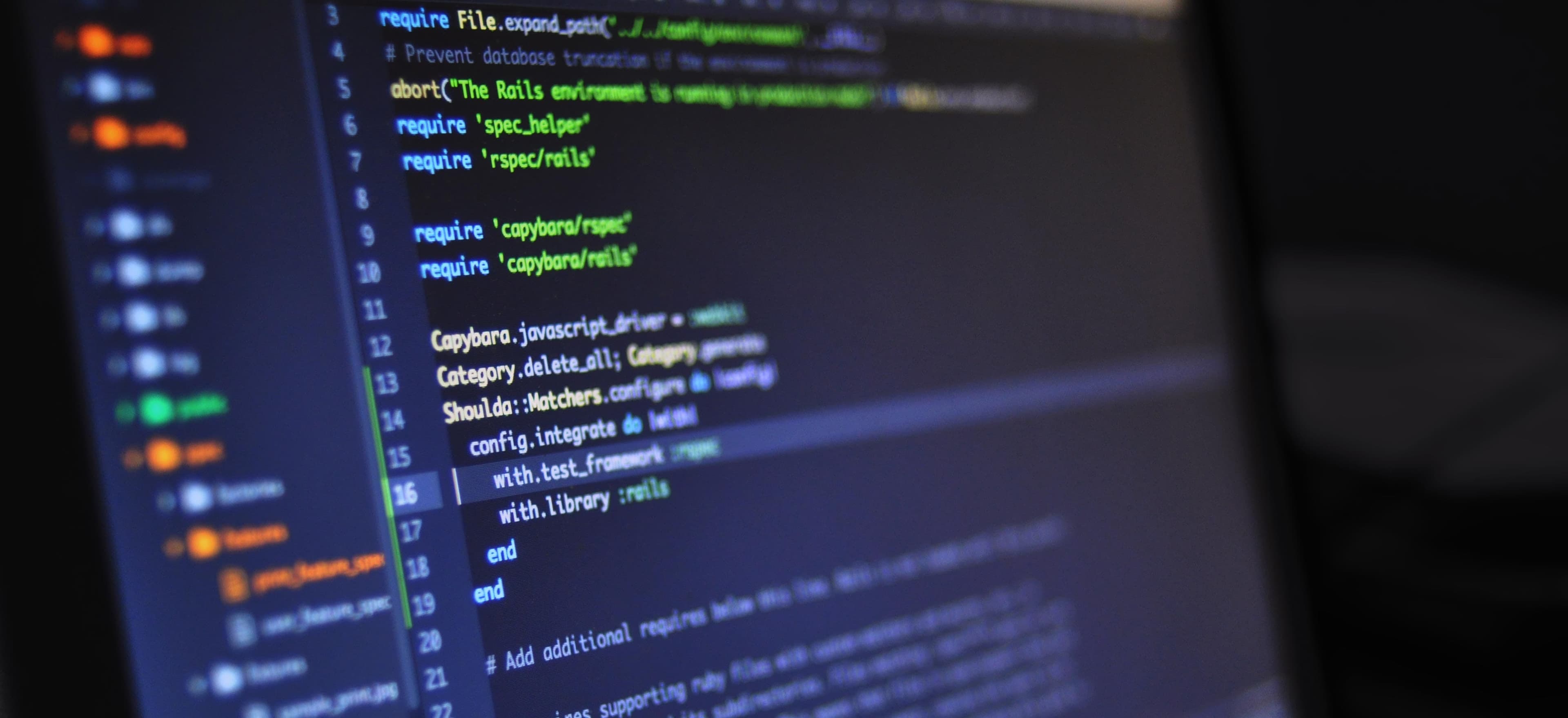
- Published on
Conquering the Frustration of Non-Reproducible Bugs in Java
As Java developers, we often face a particularly vexing issue: non-reproducible bugs. These bugs seem to appear out of nowhere, occasionally bite us during testing, and can leave us feeling helpless when they don't manifest under our conditions. In this blog post, we'll explore what non-reproducible bugs are, why they occur, and strategies to troubleshoot and ultimately conquer these aggravating issues.
Understanding Non-Reproducible Bugs
What Are Non-Reproducible Bugs?
Non-reproducible bugs are issues that cannot be consistently duplicated in a testing environment. They may occur during live production but fail to appear under the controlled conditions of a development or testing setting. This inconsistency can stem from various factors—environmental differences, data disparities, or timing issues, to name a few.
Why Do They Occur?
- Timing Issues: Some bugs may arise from race conditions where multiple threads interact unpredictably.
- Environmental Factors: The configuration of servers, libraries, or even operating systems can lead to varying behaviors.
- Data State: The state of the application’s data can influence how certain methods behave—especially in databases.
Case Study: A Common Example
Consider an online shopping cart application written in Java. When a user adds an item but their connection times out, they might experience a double-charge due to a race condition that affects the transaction process. Here is a snippet of code illustrating this concept:
public synchronized void addToCart(Item item) {
if (cart.contains(item)) {
// If item already exists, increment the quantity
cart.incrementQuantity(item);
} else {
// Add new item to cart
cart.add(item);
}
}
Why This Code?
- Synchronized Keyword: This ensures that the method can be accessed by only one thread at a time, thus preventing race conditions. However, it's essential to consider performance due to thread contention.
The Challenge
Imagine testing this code by simulating a user adding an item. However, if your test simulates only one user at a time, the bug can go unnoticed. When put into production, multiple threads might trigger the method simultaneously, revealing a potentially harmful flaw.
Troubleshooting Non-Reproducible Bugs
Now that we have a perspective on what non-reproducible bugs are, let's delve into some strategies to troubleshoot and overcome these issues.
1. Logging
Implement comprehensive logging to capture what is happening in your application before, during, and after a bug occurs. Java provides several logging libraries, with java.util.logging
and Log4j being two common choices.
Here’s a simple example:
import java.util.logging.Logger;
public class ShoppingCart {
private static final Logger logger = Logger.getLogger(ShoppingCart.class.getName());
public synchronized void addToCart(Item item) {
logger.info("Attempting to add item to cart: " + item.getName());
// Rest of the code...
}
}
Why Logging?
- Understanding Context: By logging critical variables and states at various points in your code, you gain insight into potential failure points.
- Tracking Issues: Log entries can help establish patterns or environmental settings when a bug occurs.
Learn more about effective logging in Java here.
2. Use a Debugging Tool
Java debugging tools like IntelliJ IDEA or Eclipse can provide features like breakpoints and step-through debugging. When a bug occurs, high-level debugging coupled with enabling options like "Concurrency Visualizer" or "Thread Dumps" can often reveal the state of your threads and synchronized methods.
3. Automated Tests
Write integration tests that simulate a more realistic environment. Tools like JUnit can be used creatively to emulate high-load situations:
@RunWith(ConcurrentJunitRunner.class)
public class ShoppingCartTest {
@Test
public void testAddToCartWithMultipleThreads() {
// Simulate multiple threads attempting to add an item to the cart
ExecutorService executor = Executors.newFixedThreadPool(10);
Item item = new Item("Sample Item");
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
cart.addToCart(item);
});
}
executor.shutdown();
executor.awaitTermination(1, TimeUnit.MINUTES);
// Assert expected outcomes
}
}
Why Use Automated Tests?
- Reproduce the Bug: Writing tests that intentionally overlap thread usage helps surface race conditions and discover bugs proactively.
- Regression Safety: Stable tests will enforce correctness as you modify code in the future.
4. Review Code and Configuration
Organizing peer reviews of your code can often shine a light on overlooked issues. Involving team members allows fresh eyes to catch potentially unlikely bugs. Additionally, ensure configuration settings maintain consistency between development, testing, and production environments.
5. Capture Environment States
sometimes recording the state of your environment can provide insights. Tools like Docker allow you to create a containerized version of your application replicable across different setups.
The Bottom Line
Non-reproducible bugs may seem daunting; however, with strategic troubleshooting approaches, understanding the underlying causes, and leveraging tools and practices, you can minimize them effectively. Always remember that logging, debugging, testing, and proper code review are essential components in ridding your Java applications of these elusive bugs.
By conquering the frustration of non-reproducible bugs, you not only enhance your code's reliability but also bolster your confidence as a developer. Our tools will always evolve, but a solid understanding of debugging practices will continue to be a cornerstone of effective software development.
For further reading, check out The Pragmatic Programmer, which offers invaluable insights into becoming a better programmer and troubleshooting challenges you may encounter.
By mastering the art of debugging, you will greatly enhance your productivity, ensuring a smoother development process and happier end-users. Happy coding!
Checkout our other articles