Common Pitfalls When Passing Arrays to PL/pgSQL Functions
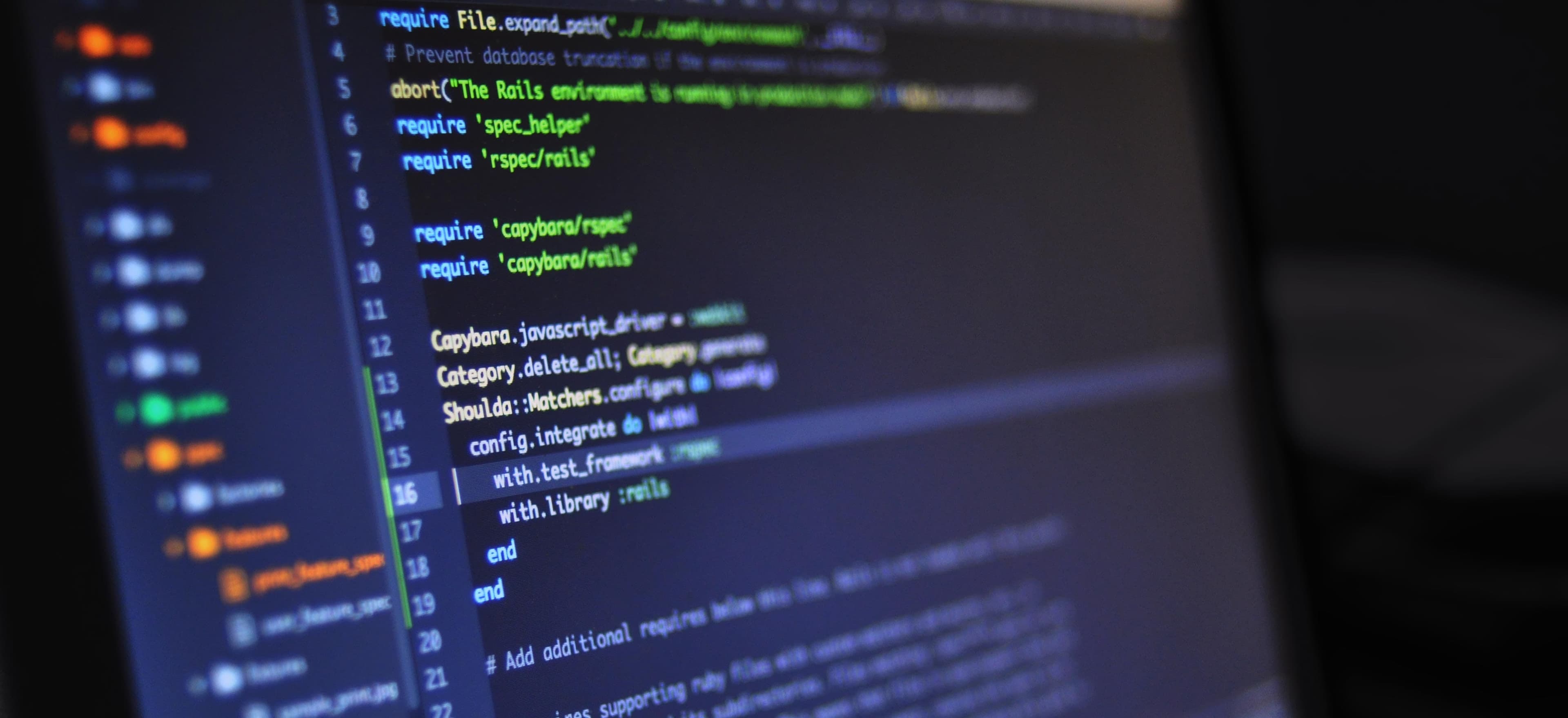
- Published on
Common Pitfalls When Passing Arrays to PL/pgSQL Functions
PostgreSQL is a powerful relational database management system that utilizes the procedural language PL/pgSQL for crafting advanced database functionalities. One of the standout features of this language is its capability to handle arrays, but as users dive into array handling, several common pitfalls emerge. In this article, we will explore these pitfalls in detail to equip you with a more nuanced understanding of how to work effectively with arrays in PL/pgSQL functions.
Understanding PL/pgSQL and Arrays
Before we dive into specific pitfalls, let's establish the context.
What is PL/pgSQL?
PL/pgSQL is a procedural language designed specifically for PostgreSQL. It allows developers to create complex SQL statements in a way that is efficient, maintainable, and expressive.
Working with Arrays
Arrays in PostgreSQL can hold multiple values of a single data type, allowing for the storage of structured data. However, working with arrays can sometimes lead to unexpected results, especially when passing them to PL/pgSQL functions.
Pitfalls When Passing Arrays to PL/pgSQL Functions
1. Incorrect Data Types
One of the first hurdles developers face when dealing with arrays in PL/pgSQL is ensuring the data types match. PL/pgSQL functions possess strict type checking, and any mismatch can lead to runtime errors.
Example
CREATE OR REPLACE FUNCTION process_integer_array(int_array INT[]) RETURNS VOID AS $$
DECLARE
item INT;
BEGIN
FOREACH item IN ARRAY int_array
LOOP
RAISE NOTICE 'Processing item: %', item;
END LOOP;
END;
$$ LANGUAGE plpgsql;
SELECT process_integer_array(ARRAY[1, 2, 3]);
In this example, the function expects an array of integers. Passing, say, an array of strings will lead to an error like:
ERROR: argument of WHERE must be type integer, not type character varying
Why It Matters: Always ensure that the data type of the array you are passing matches the expected data type of the function.
2. Not Handling NULL Values
Arrays in PostgreSQL can hold NULL values. Failing to handle these values appropriately can result in ambiguous behaviors during execution.
Example
CREATE OR REPLACE FUNCTION handle_nulls(int_array INT[]) RETURNS VOID AS $$
DECLARE
item INT;
BEGIN
FOREACH item IN ARRAY int_array
LOOP
IF item IS NOT NULL THEN
RAISE NOTICE 'Processing item: %', item;
ELSE
RAISE NOTICE 'Item is NULL';
END IF;
END LOOP;
END;
$$ LANGUAGE plpgsql;
SELECT handle_nulls(ARRAY[1, NULL, 2]);
The above function explicitly handles NULL values, allowing for clearer and more predictable output during processing.
Why It Matters: Avoid assumptions about values in your arrays. Handle NULLs clearly to prevent unexpected issues in your application logic.
3. Misunderstanding Array Indexing
Another common misconception involves the way PostgreSQL treats array indexing. Unlike many programming languages where indexing begins at 0, PostgreSQL arrays are 1-indexed.
Example
CREATE OR REPLACE FUNCTION access_first_element(int_array INT[]) RETURNS INT AS $$
BEGIN
RETURN int_array[1]; -- Correctly accesses the first element
END;
$$ LANGUAGE plpgsql;
SELECT access_first_element(ARRAY[10, 20, 30]); -- Returns 10
Attempting to access the first element with int_array[0]
will lead to:
ERROR: array subscript must be an integer
Why It Matters: Understanding PostgreSQL's 1-based indexing is crucial to ensure you are pulling the correct data from your arrays.
4. Performance Issues with Large Arrays
Handling large arrays within PL/pgSQL can lead to performance degradation. This is particularly true if you are using operations that iterate over each element.
Example
CREATE OR REPLACE FUNCTION compute_large_array(int_array INT[]) RETURNS VOID AS $$
DECLARE
sum INT := 0;
BEGIN
FOREACH item IN ARRAY int_array
LOOP
sum := sum + item; -- This can be costly for very large arrays
END LOOP;
RAISE NOTICE 'Total Sum: %', sum;
END;
$$ LANGUAGE plpgsql;
In such cases, it's crucial to evaluate whether there are set operations or other SQL functionalities that might perform better than manual iteration.
Why It Matters: Whenever you work with large datasets, consider performance. Opt for set-based operations when feasible.
5. Misusing Array Functions
PostgreSQL offers several built-in functions to work with arrays, such as array_length
, unnest
, and others. Misusing these functions can lead to inefficiencies and unexpected results.
Example
SELECT unnest(ARRAY[1, 2, 3]); -- Correct usage of unnest
Using unnest
can provide flattened results but can also cause confusion if you are explicitly expecting an array structure.
Why It Matters: Familiarize yourself with the built-in array functions to leverage their power effectively and to avoid redundant operations.
Best Practices for Passing Arrays to PL/pgSQL Functions
Following are some best practices to keep in mind:
- Type Consistency: Always verify that the data types match between the function definition and the array passed in.
- Handle NULLs: Foresee the presence of NULL values and dictate how your function should react to them.
- Use 1-Based Indexing: Remember that PostgreSQL arrays start indexing at 1 to avoid runtime errors.
- Optimize for Performance: Be conscious of performance when handling large arrays; prefer set operations over manual loops where possible.
- Leverage Built-in Functions: Use PostgreSQL's powerful array functions wisely to enhance performance and clarity.
Final Considerations
Passing arrays to PL/pgSQL functions can be fraught with pitfalls, but with a solid understanding of the underlying rules and best practices, you can navigate these challenges effectively.
For further reading on PL/pgSQL, consider exploring these resources:
By mastering these elements, you can make the most of PL/pgSQL's capabilities, creating robust and efficient database functions that can handle the intricacies of array processing.
Checkout our other articles