Mastering Load Balancing in Apache Camel: Common Pitfalls
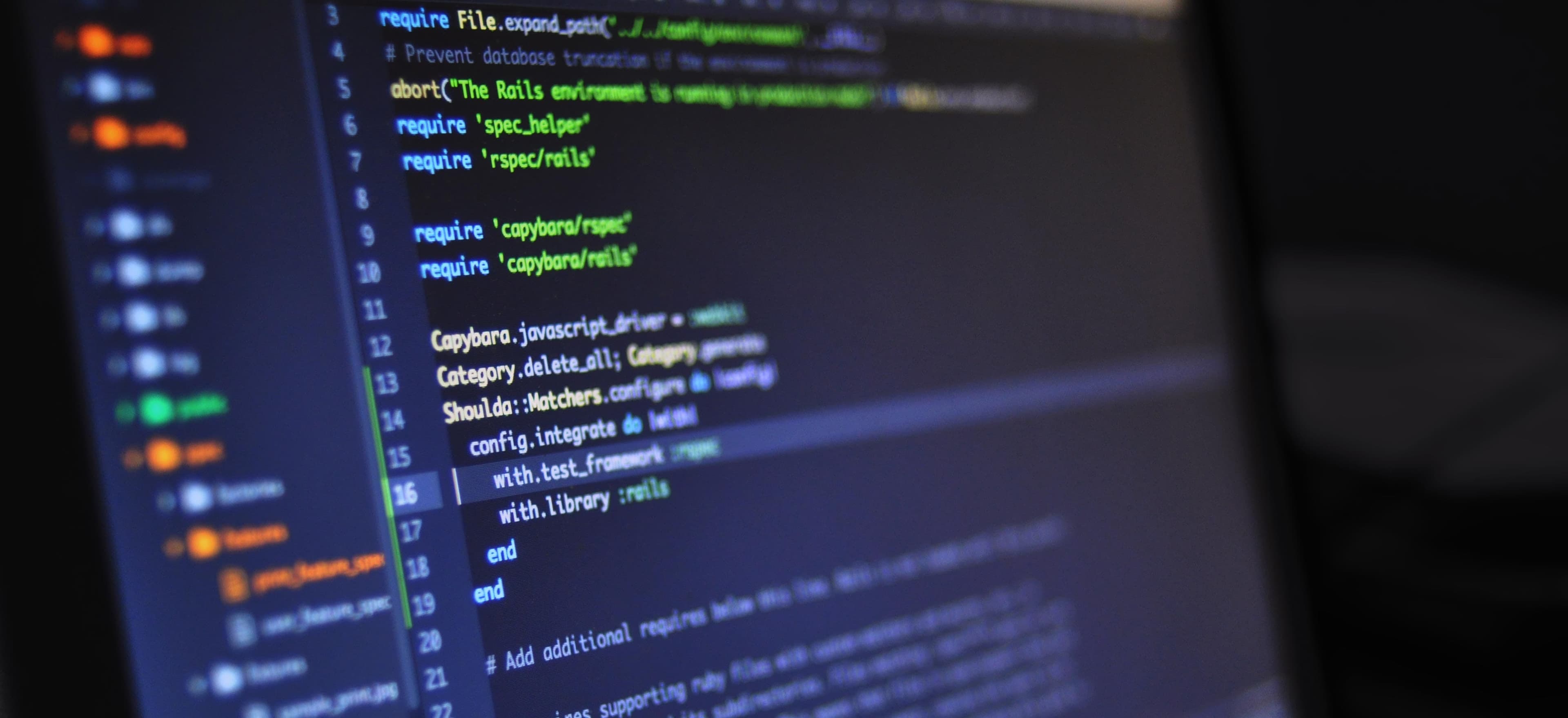
- Published on
Mastering Load Balancing in Apache Camel: Common Pitfalls
Load balancing is crucial for building robust, scalable, and fault-tolerant applications. When working with Apache Camel, it’s essential to understand how load balancing works and the common pitfalls that developers face. This blog post will dive deep into how to effectively use load balancing in Apache Camel, illustrate potential issues, and provide solutions, all while including code snippets for clarity.
What Is Load Balancing?
Load balancing is the distribution of workloads across multiple computing resources, like servers or clusters. It helps in optimizing resource use, maximizing throughput, minimizing response time, and avoiding overloads. In the realm of Apache Camel, load balancing can be achieved through various patterns, including round-robin, sticky sessions, and more.
Load Balancing in Apache Camel
Apache Camel provides several load balancing strategies through its EIP (Enterprise Integration Patterns) framework. Some of the common load balancing strategies include:
- Round Robin
- Random
- Sticky (Session Affinity)
- Weighted Load Balancing
Each strategy has its unique use cases and can be implemented easily with Camel's DSL.
Example: Round Robin Load Balancer
from("direct:start")
.loadBalance()
.roundRobin()
.to("seda:serviceA")
.to("seda:serviceB")
.to("seda:serviceC");
Why Use Round Robin? Round Robin is a straightforward method where requests are distributed evenly in a cyclical manner. This method is effective in scenarios where all servers have equal capability and can handle similar loads.
Common Pitfalls in Apache Camel Load Balancing
Despite its advantages, configuring load balancing in Apache Camel can lead to common pitfalls. Let's explore these issues and how to mitigate them.
Pitfall 1: Not Monitoring Load Distribution
Failing to monitor how load is distributed can lead to unbalanced workloads and potential bottlenecks.
Solution: Use Camel’s built-in metrics to monitor the load distribution. Integrating with tools like Prometheus and Grafana allows for visualizing the load across your routes.
from("direct:start")
.loadBalance()
.roundRobin()
.to("seda:serviceA")
.to("seda:serviceB")
.to("seda:serviceC")
.log("Load sent to: ${header.CamelToEndpoint}");
This logging statement gives you visibility into which endpoint is receiving what load, aiding in troubleshooting and adjustment.
Pitfall 2: Inefficient Load Balancing Strategies
Choosing the wrong load balancing strategy can result in wasted resources or uneven load distribution.
Solution: Understand the characteristics of different balancing strategies. For example, use sticky sessions for user sessions where maintaining the same server for requests is critical.
Example of using sticky sessions:
from("direct:start")
.loadBalance()
.stickyHeader("JSESSIONID")
.to("seda:serviceA")
.to("seda:serviceB");
Pitfall 3: Ignoring Timeout Settings
Timeout settings on your endpoints can lead to issues when one of your services becomes slow or unresponsive.
Solution: Configure timeout settings on your Camel endpoints appropriately to ensure smooth operation.
from("direct:start")
.loadBalance()
.roundRobin()
.to("seda:serviceA?timeout=3000")
.to("seda:serviceB?timeout=3000");
Setting a timeout ensures that requests do not hang indefinitely, maintaining the performance of your application.
Pitfall 4: Scalability Issues with a Single Load Balancer
Relying on a single load balancer can introduce a single point of failure.
Solution: Utilize multiple instances of your load balancer, or employ a clustering solution that distributes the load effectively across multiple load balancer nodes.
Pitfall 5: Lack of Error Handling
Failing to implement robust error handling can cause the entire system to fail when one endpoint encounters issues.
Solution: Implement error handling at the Camel route level to manage exceptions gracefully.
from("direct:start")
.loadBalance()
.roundRobin()
.to("seda:serviceA")
.to("seda:serviceB")
.errorHandler(deadLetterChannel("log:deadLetter"));
This approach ensures that messages that cannot be processed are routed to a designated error channel, allowing for further diagnosis and action.
The Closing Argument
Load balancing in Apache Camel is a powerful tool that, when implemented correctly, can lead to highly available and resilient applications. However, several common pitfalls can hinder success.
By being proactive about monitoring, taking care in strategy selection, configuring proper timeout settings, ensuring scalability, and facilitating effective error handling, developers can build a more robust integration solution.
For further reading on Apache Camel and load balancing strategies, consider exploring the Apache Camel Documentation and Enterprise Integration Patterns.
By mastering these best practices, developers can confidently implement load balancing in their applications and avoid common issues that can arise along the way. Happy coding!