Why Overprovisioned Heap Can Still Trigger OutOfMemoryError
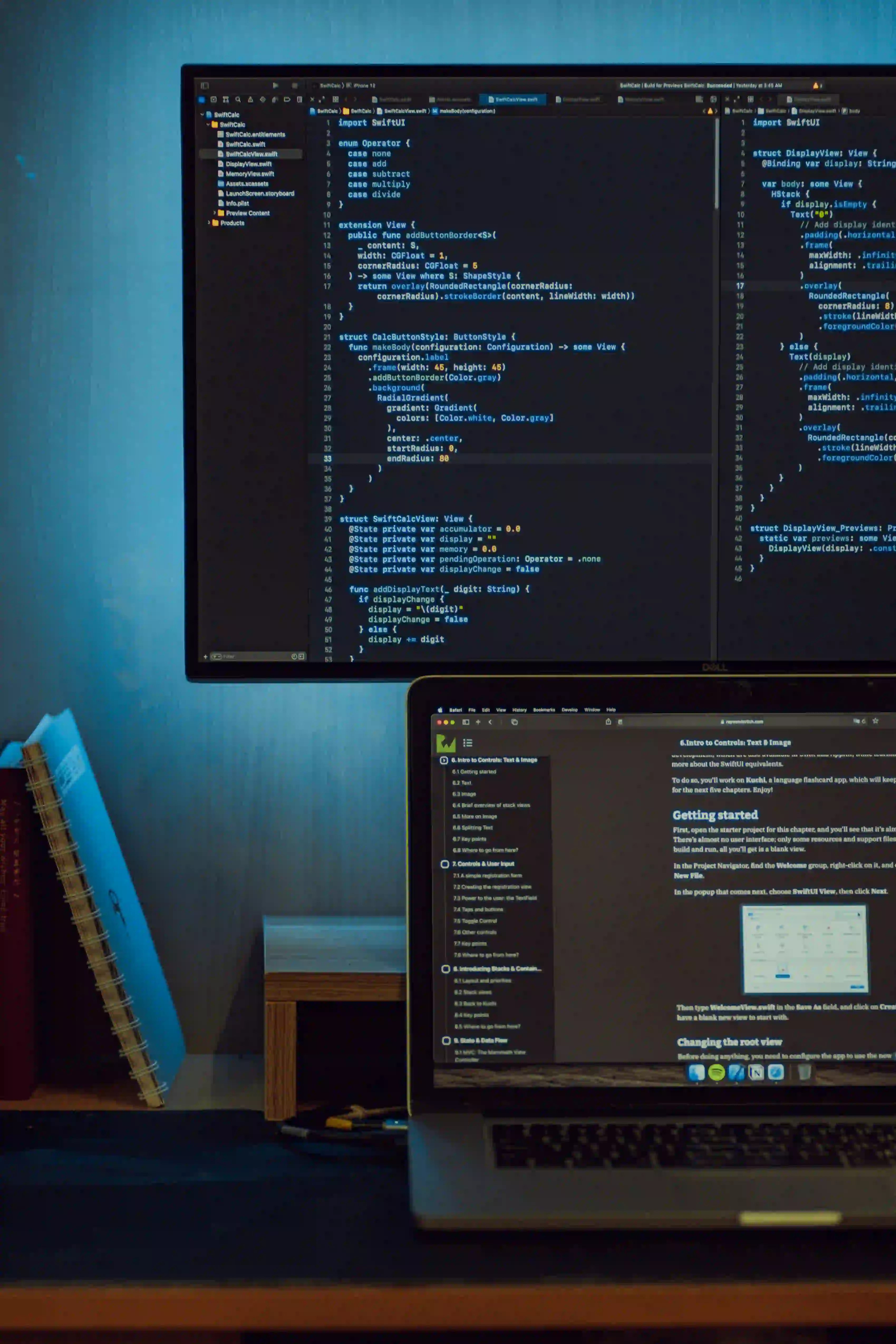
Understanding Why Overprovisioned Heap Can Still Trigger OutOfMemoryError
When developing Java applications, one of the primary concerns is managing memory efficiently. A common misconception is that if you allocate a sufficiently large heap size, OutOfMemoryError will not occur. However, this is not always the case. In this blog post, we will explore why overprovisioning the heap can still lead to OutOfMemoryError in Java, alongside practical strategies to manage memory effectively.
What Is Heap Memory in Java?
In Java, the heap is a region of memory used for dynamic memory allocation. Objects created in Java are stored here, and their lifespan is controlled by the Garbage Collector (GC). When applications are run, they can request additional memory from the Java Virtual Machine (JVM), leading us to the practice of setting heap sizes.
Setting Heap Size
You can adjust the heap size using the JVM options -Xms
(initial heap size) and -Xmx
(maximum heap size).
java -Xms512m -Xmx2048m -jar yourapp.jar
In this example, a minimum heap size of 512 MB is set, while a maximum of 2048 MB is allowed.
The Paradox of Overprovisioning
Even when you've allocated a large heap memory, you can still encounter OutOfMemoryError. Here are some key reasons that may lead to this paradox:
- Memory Leaks
- Excessive Object Creation
- JNI Memory Exhaustion
- GC Overhead Limit Exceeded
- Native Memory Limits
Memory Leaks
Memory leaks occur when an application holds references to objects that are not needed anymore. The Garbage Collector cannot reclaim the memory since it thinks those objects are still in use, leading to gradual memory consumption until the JVM runs out of heap space.
public class MemoryLeakDemo {
private static List<Object> leakedList = new ArrayList<>();
public static void addObject() {
leakedList.add(new Object());
}
}
In this code snippet, the leakedList
keeps growing indefinitely because it retains references to all objects added to it, causing potential OutOfMemoryError.
Excessive Object Creation
Creating too many objects, especially in a loop or a recursive function, can lead to rapid memory consumption. For example:
public class ExcessiveObjectCreation {
public void createMemoryHog() {
while (true) {
String largeObject = new String(new char[1000000]); // 1 MB string
}
}
}
In this code, a new string of size 1 MB is created repeatedly without any limit, ultimately exhausting heap space and triggering OutOfMemoryError.
JNI Memory Exhaustion
If your Java application integrates with native libraries through Java Native Interface (JNI), memory allocated in the native heap can also exceed available space. The JVM does not have control over this memory, which might result in an OutOfMemoryError even if your Java heap is not full.
GC Overhead Limit Exceeded
When the Java Virtual Machine spends excessive time reclaiming memory while only managing to free a small amount, it can throw a GC overhead limit exceeded exception. This can happen even with a large heap size:
java.lang.OutOfMemoryError: GC overhead limit exceeded
To resolve this, analyze application performance and optimize code to minimize object creation, or potentially increase available memory.
Native Memory Limits
Even with a large JVM heap size, if native memory (not managed by the JVM) is exhausted, you will encounter an OutOfMemoryError. This is especially relevant for applications utilizing extensive native resources, such as image processing or databases.
How to Avoid OutOfMemoryError
-
Profiling and Monitoring With Tools
Utilize tools like VisualVM, JConsole, or profilers such as YourKit to monitor memory usage.
☕snippet.java// Example to add a memory listener MemoryMXBean memoryBean = ManagementFactory.getMemoryMXBean(); memoryBean.setVerbose(true);
Check for memory leaks and hotspots where excessive allocations occur.
-
WeakReferences for Caching
Use
WeakReference
for object pools or caches so that if the GC needs memory, it can reclaim these objects when they are not in use anymore.☕snippet.javaWeakHashMap<String, Object> weakCache = new WeakHashMap<>();
-
Proper Exception Handling
Always catch exceptions and release resources. For example, explicit nulling helps with GC.
☕snippet.javatry { Object obj = new Object(); } finally { obj = null; // Explicitly allow GC }
-
Optimize Data Structures
Pick data structures that are less memory-intensive. Choose collections wisely, for instance, using
ArrayList
overLinkedList
can help in certain scenarios due to lower memory overhead. -
Tune JVM Options
Adjust your JVM parameters based on application needs, including the garbage collection strategy and heap size configurations.
In Conclusion, Here is What Matters
Overprovisioning heap space is not a silver bullet against OutOfMemoryError in Java applications. Memory management requires a comprehensive understanding of how your application utilizes memory and proper application design. By leveraging tools for monitoring, utilizing weak references, and optimizing data handling, you can avoid common pitfalls associated with memory management.
For further reading on Java memory management and troubleshooting, check out the official Java documentation and how to properly optimize garbage collection.
If you have any questions or wish to share your experiences with memory management in Java, feel free to leave a comment below!