Common Java Deployment Pitfalls and How to Avoid Them
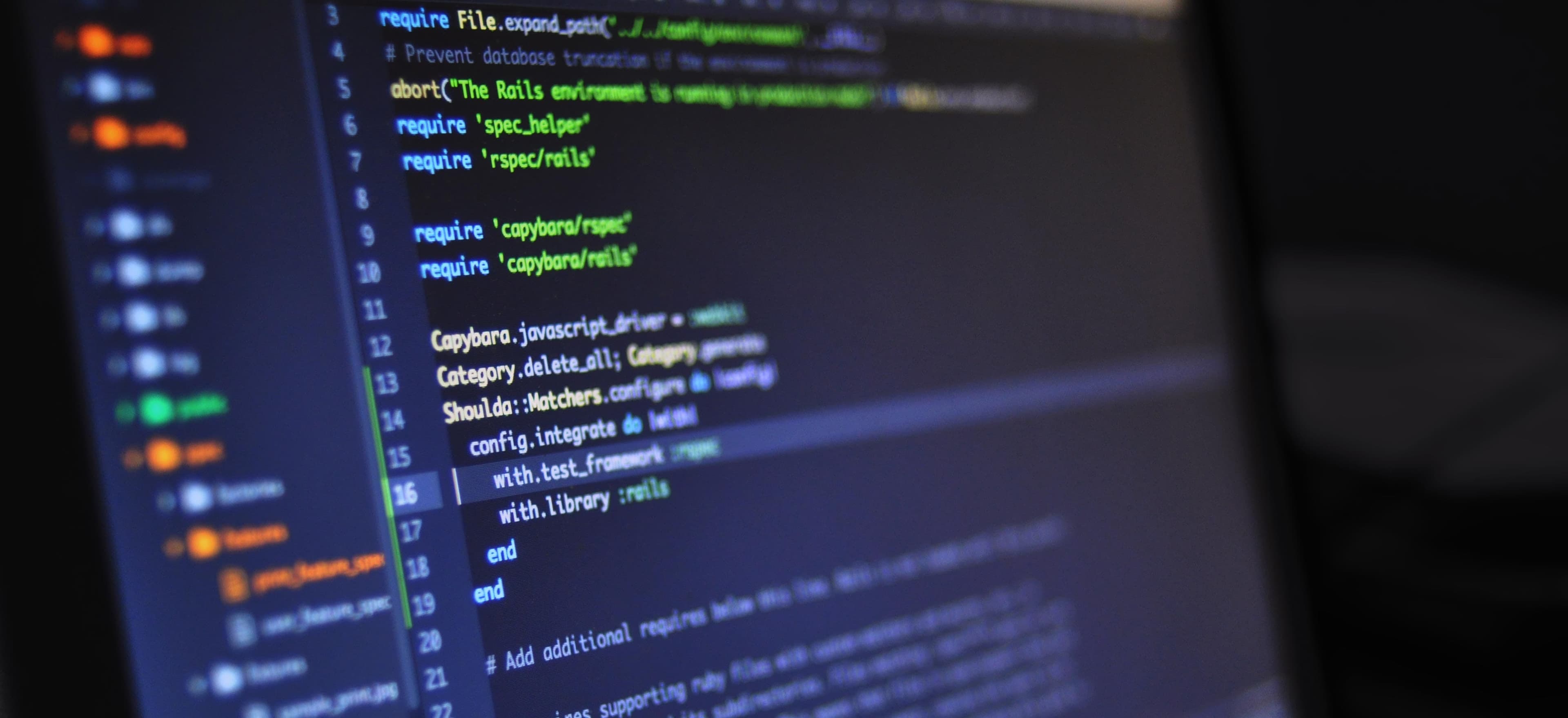
- Published on
Common Java Deployment Pitfalls and How to Avoid Them
Deploying Java applications can often be riddled with challenges, even for seasoned developers. While Java is a powerful and flexible language, the deployment phase can expose various pitfalls that, if not addressed, can lead to significant downtime and lost productivity. In this blog post, we will explore common deployment pitfalls in Java development and provide actionable strategies to sidestep these challenges. Let’s dive in!
Pitfall 1: Inadequate Testing Before Deployment
Why It's Critical
Testing is an integral part of any software development lifecycle. A hasty deployment without thorough testing can lead to application failures, bugs, and user dissatisfaction. Java applications can be particularly complex, making testing all the more essential.
Solution
-
Adopt a Comprehensive Testing Approach: Implement unit tests, integration tests, and end-to-end tests. Each of these testing types plays a unique role:
- Unit Tests gauge the functionality of individual components.
- Integration Tests check how different modules work together.
- End-to-End Tests simulate real user scenarios to ensure that everything functions correctly within the application.
-
Continuous Integration (CI): Using a CI pipeline, such as Jenkins or GitHub Actions, allows running tests automatically with every build. This ensures that only tested code gets deployed.
// Example of a simple JUnit test case.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "Adding 2 and 3 should equal 5");
}
}
Resources
For an in-depth understanding of testing frameworks, check out JUnit 5 documentation for guidelines on effective testing practices.
Pitfall 2: Ignoring Environment Discrepancies
Why It's Critical
Running an application in a local or development environment that significantly differs from the production setup can lead to application failure upon deployment. For instance, different versions of the Java Runtime Environment (JRE) or database configurations may yield varying results.
Solution
- Containerization: Tools like Docker can help simulate the production environment. They ensure that the application runs in the same way on any machine that has Docker installed.
# A simple Dockerfile for a Java application.
FROM openjdk:11
# Set the working directory
WORKDIR /app
# Copy the jar file
COPY target/myapp.jar myapp.jar
# Run the application
CMD ["java", "-jar", "myapp.jar"]
-
Configuration Management: Use configuration management tools like Ansible or Chef to maintain consistency in environments by managing configurations centrally.
-
Testing on Staging: Always deploy to a staging environment that mimics production as closely as possible. It allows for real-world testing before the final release.
Pitfall 3: Poor Logging and Monitoring
Why It's Critical
In the event of failure, a lack of proper logging and monitoring can make diagnosing issues a Herculean task. Without sufficient data, debugging becomes a guessing game.
Solution
- Effective Logging Framework: Use a robust logging framework, like Log4j or SLF4J, to log vital information. Log levels (INFO, WARN, ERROR) help categorize logs.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApplication {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public static void main(String[] args) {
logger.info("Application is starting...");
try {
// do something
} catch (Exception e) {
logger.error("Error occurred: {}", e.getMessage());
}
}
}
- Monitoring Tools: Tools like Prometheus coupled with Grafana, or ELK Stack, assist in monitoring the application’s performance. They can warn you about potential issues before they escalate.
Resources
For further reading on setting up an effective logging strategy, refer to Log4j documentation.
Pitfall 4: Inefficient Scaling Strategies
Why It's Critical
Java applications can sometimes become bottlenecked under increased load, especially if they are not designed to scale. Inadequate scaling can lead to slow response times and system crashes.
Solution
-
Microservices Architecture: Instead of monolithic applications, explore a microservices architecture which allows parts of the application to scale independently based on load.
-
Load Testing: Before peak traffic periods, perform load testing to ensure your application can handle the expected user load. Tools like JMeter can help simulate various levels of user engagement.
// Example of a simple load test script using JMeter
ThreadGroup {
numThreads = 100
rampTime = 100
duration = 600
}
GetSampler {
url = "http://localhost:8080/api/resource"
}
Pitfall 5: Missing Dependencies or Mismanaged Libraries
Why It's Critical
Java applications often depend on various libraries and frameworks. Forgetting or mismanaging these dependencies can break the application at runtime.
Solution
-
Dependency Management Tools: Use tools such as Maven or Gradle for managing libraries. They automatically resolve, download, and include dependencies when you build your application.
-
Creating a "fat" JAR: A "fat" or "uber" JAR bundles all dependencies into a single JAR file, ensuring everything is available during execution.
<project>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<executions>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
</executions>
</executions>
</plugin>
</plugins>
</build>
</project>
Resources
Explore the Maven documentation for additional support on how to manage dependencies effectively.
The Bottom Line
Java deployment can encompass numerous hurdles, but by addressing common pitfalls, developers can smoothly navigate the challenges of rolling out their applications. By embracing comprehensive testing, maintaining environment consistency, and ensuring robust logging and monitoring, you can significantly enhance the deployment process. Additionally, leveraging tools for dependency management and effective scaling ensures that your application remains healthy and performant, regardless of user traffic.
Remember, preparation is crucial in a successful deployment. By implementing best practices from this guide, you can enhance the resilience of your Java applications and deliver a better experience for your users.
Stay tuned for more articles discussing Java development methodologies, best practices, and community insights!