Debugging GWT Apps: Common Pitfalls with Chrome Dev Tools
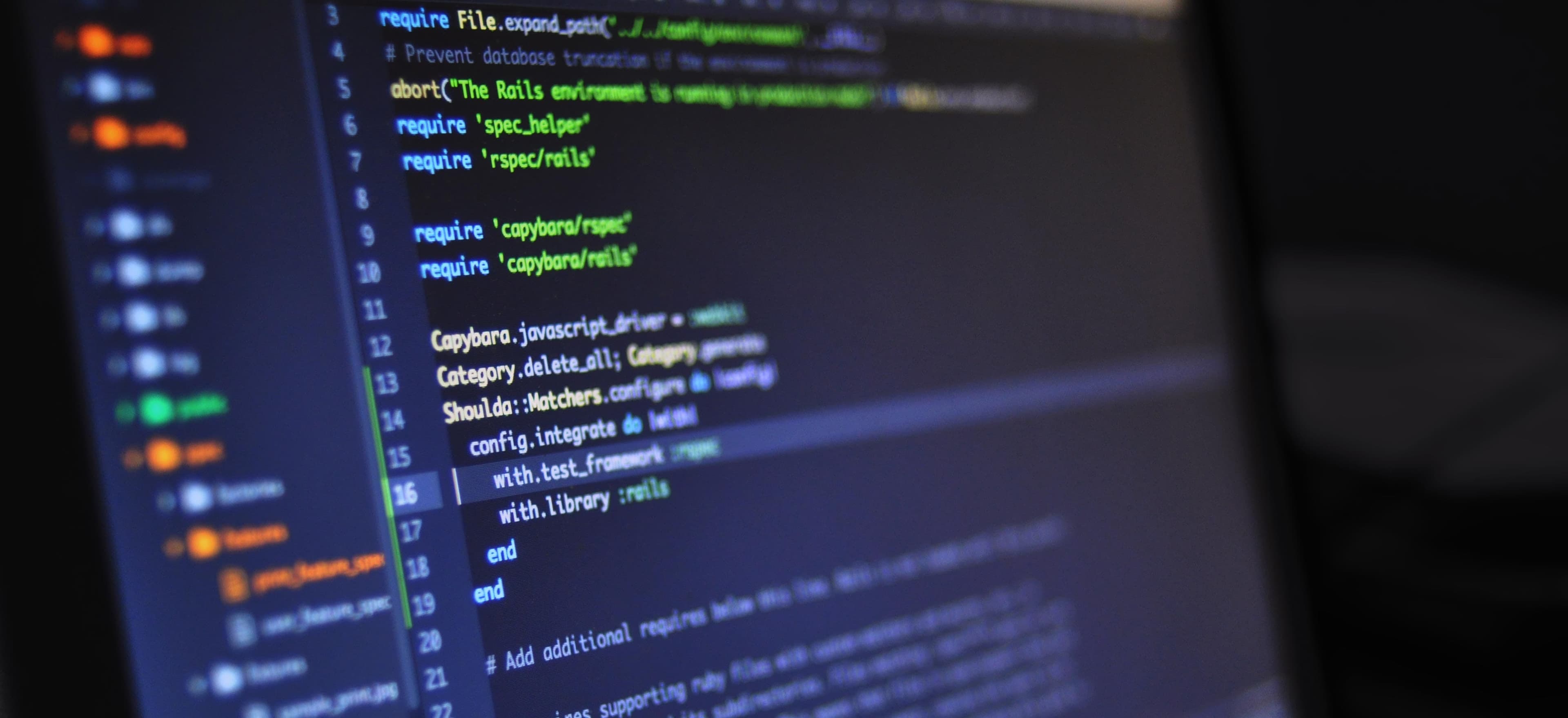
- Published on
Debugging GWT Apps: Common Pitfalls with Chrome Dev Tools
Google Web Toolkit (GWT) allows developers to write client-side code in Java and translates it into JavaScript. This powerful framework helps in building complex web applications seamlessly. However, while developing GWT applications, debugging can sometimes feel like navigating a maze. Fortunately, Chrome Dev Tools provides a set of utilities that can ease this process. In this post, we'll explore common pitfalls encountered while debugging GWT apps and provide tips and tricks to navigate them effectively.
Understanding Chrome Dev Tools
Before we dive into the specifics, it’s crucial to understand what Chrome Dev Tools offers. This robust set of web developer tools helps inspect pages, debug JavaScript, and optimize performance. You can access Chrome Dev Tools by right-clicking on any part of the page and selecting "Inspect" or by pressing Ctrl + Shift + I
(or Cmd + Option + I
on Mac).
Common Pitfalls in Debugging GWT Apps
1. Source Map Misconfiguration
A common issue developers face is the incorrect configuration of source maps. Source maps allow you to see your original Java code instead of the compiled JavaScript, making debugging significantly easier. If source maps are not set up correctly, debugging becomes exceedingly difficult as your breakpoints may not line up with the actual code.
Why You Should Care
When you compile your Java code, GWT generates JavaScript along with a source map. If the source maps are misconfigured, breakpoints in Chrome Dev Tools might not correspond to the right locations in your source files, leading to confusion.
public class MyApplication implements EntryPoint {
public void onModuleLoad() {
// The actual application logic
System.out.println("Application Loaded");
}
}
Tip
Ensure your gwt.xml
file is correctly configured with:
<set-property name="enableSourceMaps" value="true"/>
This tells GWT to generate source maps, enabling accurate debugging.
2. JavaScript Obfuscation and Minification
GWT automatically obfuscates and minifies JavaScript code to improve loading times and make reverse-engineering difficult. When hunting down bugs, this can create a challenge, as the variable names in your code become unrecognizable.
Why You Should Care
Obfuscated code is less readable, making it hard to trace errors back to their source. This could lead to an increased time spent debugging.
Tip
Use the gwt.compile.debug
option by setting it in your gwt.xml
file:
<set-property name="compiler.disableInlining" value="true"/>
<set-property name="compiler.enableDebug" value="true"/>
This command prevents the code from being minified in the development phase, allowing you to debug more easily.
3. Missing Exception Details
When an exception occurs in GWT applications, especially during asynchronous calls, the error message in the console may often lack detail. This can make it challenging to pinpoint the issue.
Why You Should Care
Lack of detailed exception information can waste valuable debugging time and frustrate developers.
Tip
To handle exceptions more gracefully, implement custom error handling in your GWT app:
public void onModuleLoad() {
try {
// Perform actions that might throw exceptions
} catch (Exception ex) {
Window.alert("Error: " + ex.getMessage());
}
}
By presenting a user-friendly error message, you can quickly understand what went wrong.
4. Complex GWT RPC Calls
Debugging Remote Procedure Calls (RPCs) can be tricky. Often, you would need to check request and response formats, especially when misconfigurations occur at the server end.
Why You Should Care
If the format of data being sent does not match that expected by your server, debugging can lead you down a rabbit hole of errors.
Tip
Utilize the Network panel in Chrome Dev Tools to inspect asynchronous RPC calls:
- Open Dev Tools and navigate to the Network tab.
- Make sure to preserve log to keep data during page reloads.
- Observe any RPC requests and their responses.
This direct peek into what’s being sent and received can provide insight into malformed communication.
5. CSS and UI Layout Issues
Sometimes, the problem lies not in the Java application code itself but in how CSS is being interpreted by the browser. Misalignment and styling issues can easily arise without proper inspections.
Why You Should Care
Visual bugs can detract from user experience, leading to frustrating scenarios if not addressed promptly.
Tip
Use the Elements tab in Dev Tools to inspect and edit styles on-the-fly. You can view the computed styles for any element and see which CSS rules are being applied.
The Closing Argument
Debugging GWT applications can be quite the trek, filled with pitfalls that can frustrate even the most seasoned developers. By leveraging the power of Chrome Dev Tools effectively, you can smooth out this journey, enhancing your debug strategies.
In Summary:
- Ensure your source maps are correctly set up for easier debugging.
- Control JavaScript minification for a clearer view of your code.
- Implement better error handling for clearer exception messages.
- Use the Network panel to validate your RPC communications.
- Inspect and experiment with CSS layouts in real-time.
For more advanced debug techniques in GWT, consider visiting the GWT Documentation which provides extensive details about the framework's capabilities, or check out forums dedicated to GWT and Java web development for community insights and troubleshooting tips. With the right tools and tips, debugging your GWT applications will become a less painful experience.
Remember, every bug is an opportunity to learn and improve your application. Happy debugging!