How to Diagnose JVM File Descriptor Leaks Efficiently
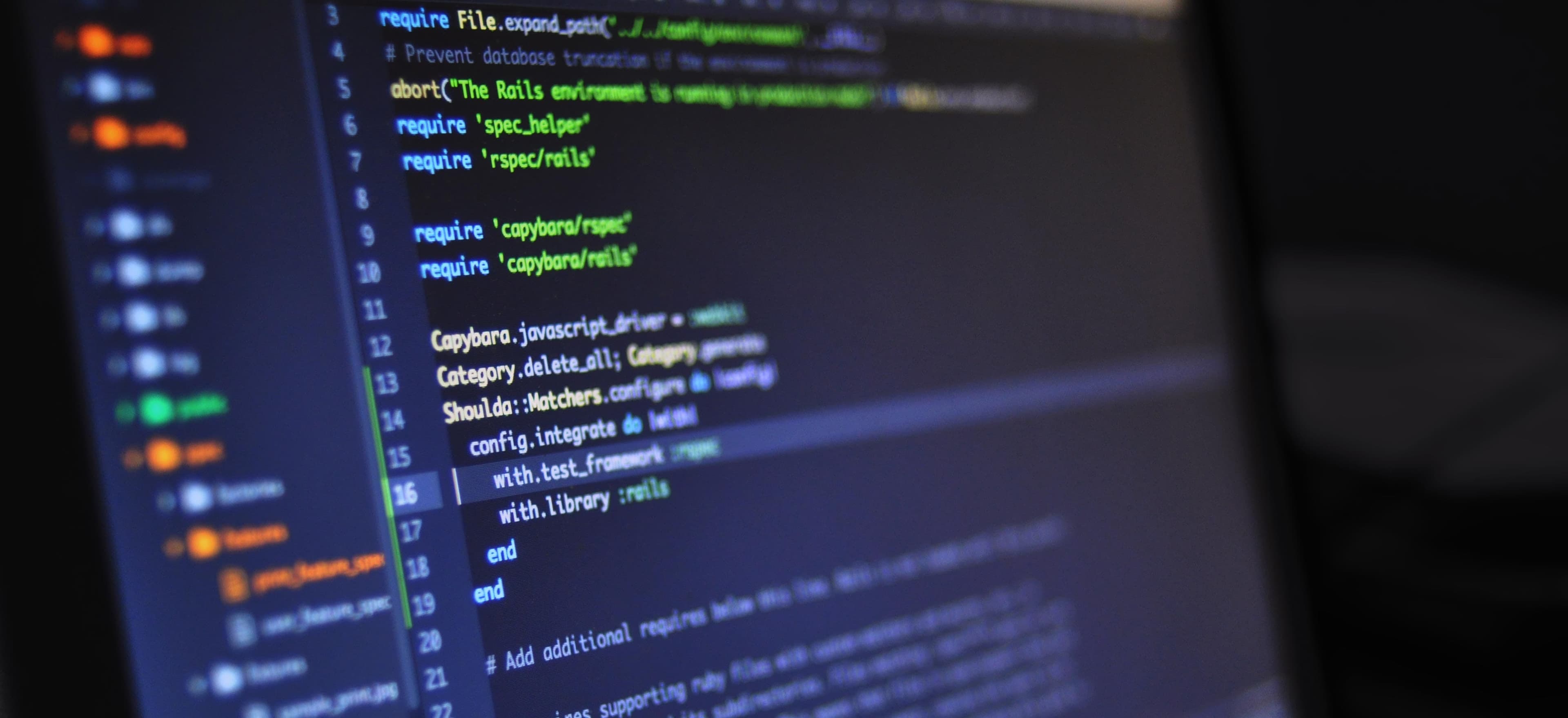
- Published on
How to Diagnose JVM File Descriptor Leaks Efficiently
The Java Virtual Machine (JVM) is robust, but it can experience file descriptor leaks leading to performance degradation or even application failures. Diagnosing these leaks isn't always straightforward, but with a methodical approach, it can be tackled effectively. In this post, we will dive deep into JVM file descriptor leaks, how to detect them, and strategies for efficient diagnosis and resolution.
Understanding File Descriptors
File descriptors are critical resources in any operating system. They represent open files, sockets, and pipes—anything that can be read from or written to. In the context of the JVM, each connection or file handle your application opens consumes a file descriptor.
The operating system usually limits the number of file descriptors an application can open. For instance, in Linux, the default limit for a single process is often set at 1024. If your application exceeds this limit due to leaking file descriptors, it can trigger an IOException
.
Key Signs of File Descriptor Leaks
- Increased Exception Frequency: Applications start throwing
Too many open files
exceptions. - Performance Degeneration: Performance stats drop as available descriptors run low.
- Resource Exhaustion: Eventually, your application may halt due to resource exhaustion.
Why Does This Happen?
File descriptor leaks often occur when resources aren't properly managed. Typically, developers forget to close resources after usage. For instance, if a stream is opened but never closed, the descriptor remains active and counts against the limit.
Example Java Code
Let's consider a simple Java snippet that opens a file but forgets to close it. We'll analyze why this is an issue.
import java.io.FileReader;
import java.io.IOException;
public class FileDescriptorLeakDemo {
public static void main(String[] args) {
try {
FileReader reader = new FileReader("file.txt");
// Processing the file...
// Not closing the reader leads to a leak!
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the above code, the FileReader
is opened but not closed. This oversight can lead to a file descriptor leak, especially if this code runs in a loop or multiple threads.
Diagnosing File Descriptor Leaks
Diagnosing file descriptor leaks involves a few steps. Here’s an efficient methodology:
1. Monitor File Descriptor Count
On Unix-like systems, you can monitor file descriptors using commands like:
lsof -p <pid>
or for a continuous count, use:
watch -n 1 'lsof -p <pid> | wc -l'
This command lists open files for a specific process. If you see this number continuously increasing without decreasing, it's a clear indicator of a leak.
2. Use Java Tools
Java provides several tools to help diagnose leaks.
a. JVisualVM
JVisualVM is a powerful monitoring tool that comes with the JDK. You can monitor memory usage, CPU load, and thread states. Specifically, you can also track open file descriptors.
- Launch JVisualVM.
- Connect to your Java application.
- Inspect the "Threads" and "Sampler" tabs.
b. JConsole
Similar to JVisualVM, JConsole can monitor the JVM and track MBeans. You can visualize the number of file descriptors being used. This implicit monitoring can help identify abnormal patterns indicating leaks.
3. Profiling Code
Profile your code using a Java profiler like YourKit, JProfiler, or VisualVM to identify where file descriptors are being opened and if they are being closed properly.
Example Profiling Code:
Here’s a corrected version of our earlier example demonstrating proper resource management using the Try-With-Resources statement introduced in Java 7:
import java.io.FileReader;
import java.io.BufferedReader;
import java.io.IOException;
public class FileDescriptorLeakFixed {
public static void main(String[] args) {
try (FileReader reader = new FileReader("file.txt");
BufferedReader bufferedReader = new BufferedReader(reader)) {
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
// Reader is automatically closed.
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code, the try-with-resources
statement ensures that the FileReader
and BufferedReader
are properly closed after use, thereby preventing leaks.
Tools for Leak Detection
Using tools designed for detecting leaks can significantly reduce debugging time. Some recommended ones include:
- LeakCanary: Although primarily aimed at Android, it serves as a model for detecting resource leaks.
- FindBugs: A static analysis tool for Java that can identify potential leak issues.
- Eclipse Memory Analyzer (MAT): Ideal for deep analysis to find memory leaks and analyze heap dumps.
Best Practices to Prevent File Descriptor Leaks
Prevention is always better than cure. Here are some best practices:
-
Always Close Resources: Use Try-With-Resources or ensure that
finally
blocks are used to close resources. -
Set Limits: On some systems, you can explicitly set file descriptor limits for your Java application. This can serve as a temporary buffer against leaks.
-
Regular Code Reviews: Encourage code reviews that focus on resource management.
-
Automated Testing: Incorporate test cases that simulate various resource loads and check for potential leaks during integration testing.
Key Takeaways
Diagnosing JVM file descriptor leaks can often feel overwhelming, but with the right tools and practices, it is manageable. By monitoring your application's file descriptor usage, improving your code to manage resources effectively, and employing profiling tools, you can tackle these leaks efficiently.
For more insight into JVM performance tuning, check out JVM Performance Tuning Best Practices and Understanding Garbage Collection.
Happy coding!
Checkout our other articles