Understanding Performance Issues with StringBuffer in Java
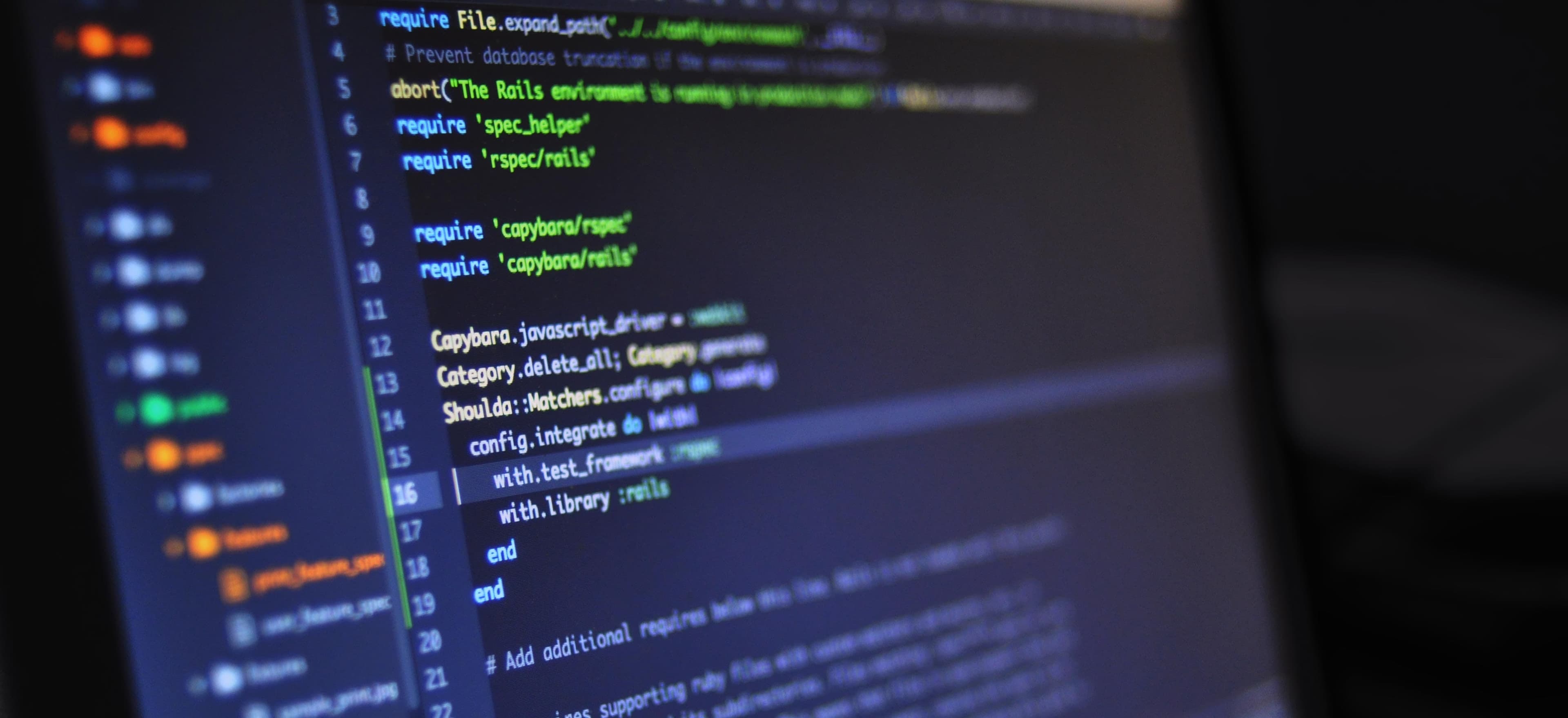
- Published on
Understanding Performance Issues with StringBuffer in Java
Java is a versatile and powerful programming language that provides developers with various options to manage and manipulate strings. One such option is StringBuffer
, which is designed to create mutable strings. While it appears to be a convenient choice for string manipulation, performance issues can arise when it is not used appropriately. In this post, we'll delve into the intricacies of StringBuffer
, explore its performance challenges, and present best practices for effective usage.
What is StringBuffer?
StringBuffer
is a class in Java that allows you to create mutable sequences of characters. Unlike the immutable String
class, which creates a new object for any change, StringBuffer
provides methods to manipulate strings without creating new instances. This makes it particularly useful in scenarios requiring frequent modifications.
Example of StringBuffer Usage
StringBuffer stringBuffer = new StringBuffer("Hello");
stringBuffer.append(" World");
System.out.println(stringBuffer); // Output: Hello World
In this example, we create an instance of StringBuffer
and append additional text to it without creating a new string object.
Why Use StringBuffer?
The primary reasons to use StringBuffer
include:
- Mutability: As mentioned,
StringBuffer
allows for modification of character data without creating multiple objects. - Thread-Safety: It is synchronized, which means it is safe for use in multiple threads.
However, the very characteristics that make StringBuffer
appealing can also lead to performance bottlenecks, particularly in environments where speed is of the essence.
Performance Issues with StringBuffer
1. Synchronization Overhead
Since StringBuffer
is synchronized, each method call involves an overhead from the locking mechanism. In single-threaded applications, this added complexity is unnecessary and can lead to slower performance compared to using StringBuilder
, which is a non-synchronized alternative.
StringBuffer stringBuffer = new StringBuffer();
for (int i = 0; i < 10000; i++) {
stringBuffer.append(i);
}
In the above code snippet, although synchronizing the method calls ensures thread safety, it also contributes to performance degradation in single-thread scenarios.
2. Capacity Management
Internally, StringBuffer
maintains a character array to store the string data. When the specified capacity of the StringBuffer
is exceeded, a new, larger array is created and the data is copied over. This resizing operation can be costly in terms of performance.
StringBuffer stringBuffer = new StringBuffer(10); // Initial Capacity
for (int i = 0; i < 100; i++) {
stringBuffer.append(i);
}
// Resizing occurs here as the entries exceed capacity.
In this example, the performance impact increases with the frequency of resizing operations.
3. Overhead of Method Calls
Every operation with StringBuffer
invokes a method call which adds overhead compared to simpler constructs. For intensive operations involving many manipulations, the accumulated overhead can significantly slow down execution.
Performance Comparison: StringBuffer vs. StringBuilder
To provide better context, let’s compare StringBuffer
and StringBuilder
.
- Thread Safety:
StringBuffer
is synchronized, whileStringBuilder
is not. - Performance: In single-threaded scenarios,
StringBuilder
outperformsStringBuffer
due to the absence of synchronization overhead.
Example Code
StringBuilder stringBuilder = new StringBuilder();
for (int i = 0; i < 10000; i++) {
stringBuilder.append(i);
}
In this code snippet with StringBuilder
, the performance will typically be superior compared to an equivalent StringBuffer
.
When to Use StringBuffer?
Understanding when to use StringBuffer
is crucial:
- Multi-threaded Applications: Use
StringBuffer
when you are certain that multiple threads will try to modify the same string. - Legacy Code: In some legacy systems,
StringBuffer
might have been thego-to
option. If rewriting is not an option, it can still be relevant. - Larger String Operations: If a string grows beyond its initial capacity significantly but is accessed by multiple threads.
Best Practices
Here are some best practices to keep in mind when working with StringBuffer
:
- Prefer StringBuilder for Single-threaded Scenarios: As mentioned,
StringBuilder
provides a better performance profile when thread safety is not an issue. - Preallocate Capacity Utilizing Constructors: If you know the expected length of the final string, initialize
StringBuffer
with that capacity to minimize resizing.
StringBuffer stringBuffer = new StringBuffer(100); // Preallocated capacity for performance
- Use ToString Wisely: Convert
StringBuffer
to aString
lazily, only when necessary.
Example of Conversion
String result = stringBuffer.toString(); // Converts StringBuffer to String
This conversion is acceptable when you are sure no further modifications will be performed on the StringBuffer
.
Lessons Learned
While StringBuffer
offers advantages in terms of mutability and thread safety, understanding its performance implications is crucial for developing efficient Java applications. In most scenarios, opting for StringBuilder
is the better choice unless thread safety is a paramount concern.
For more information regarding string manipulation in Java, feel free to check out the Java Documentation.
By thoughtfully applying the concepts discussed, you can enhance your Java applications' performance and maintainability.
Keep the conversation going! How do you manage string manipulation in your projects? Have you faced performance issues with StringBuffer
or switched to StringBuilder
? Share your experiences in the comments below!
Checkout our other articles