Overcoming Complexity: Mastering the Template Design Pattern
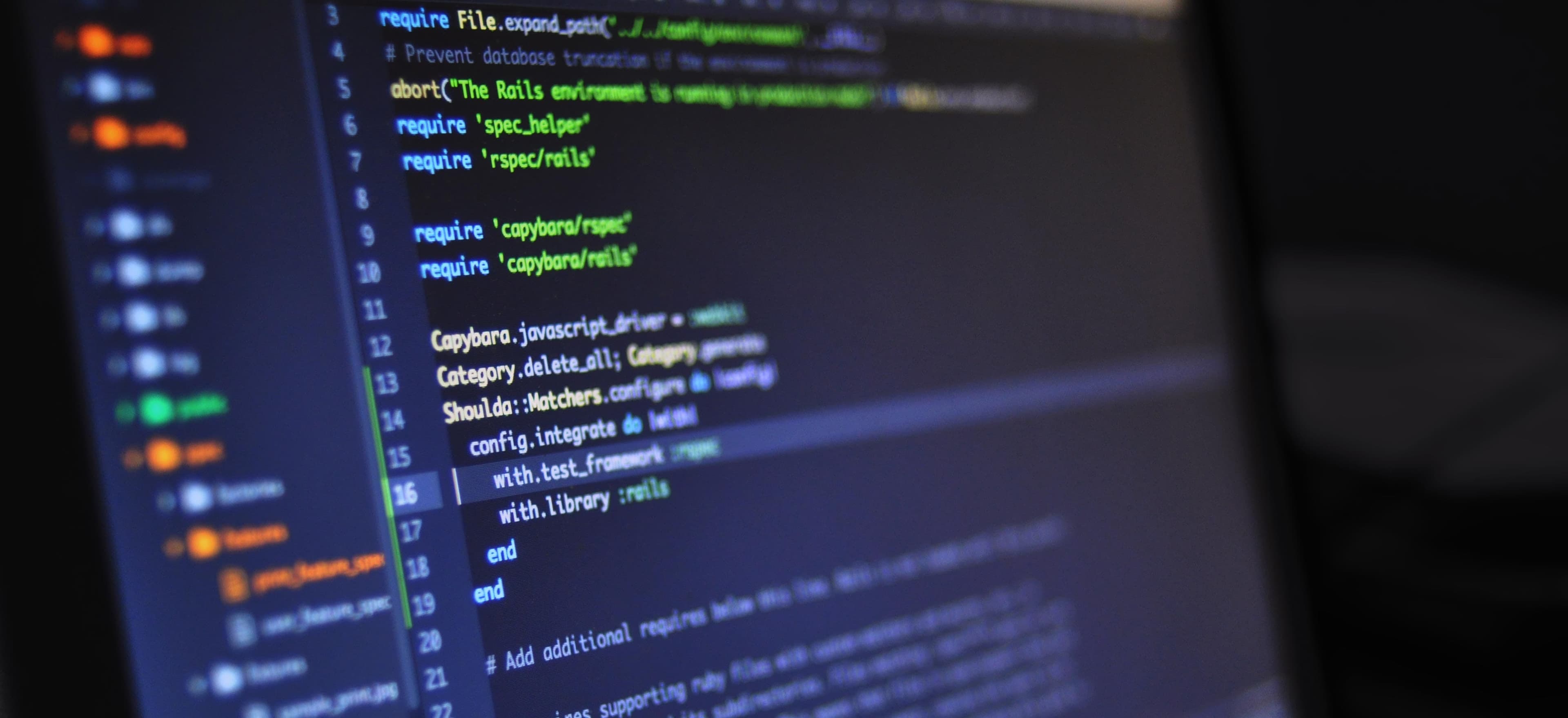
- Published on
Overcoming Complexity: Mastering the Template Design Pattern
In the realm of software design, simplicity and clarity are the ultimate goals. Yet, as projects grow in complexity, managing this complexity can become overwhelming. One of the most effective design patterns to combat this is the Template Design Pattern. In this blog post, we will explore what the Template Design Pattern is, why it is valuable, and how to implement it in Java with practical, engaging examples.
Understanding the Template Design Pattern
At its core, the Template Design Pattern provides a blueprint for organizing code while allowing for some level of customization. This pattern helps in defining the skeleton of an algorithm in an operation, deferring some steps to subclasses.
Key Benefits of the Template Design Pattern
- Code Reusability: By defining common operations in a base class, you allow subclasses to reuse this code without redundancy.
- Increased Maintainability: Changes made in the base class are automatically reflected in derived classes.
- Separation of Concerns: The pattern promotes better organization by separating common behavior from specific implementations.
When to Use the Template Design Pattern
Use this pattern when:
- There are multiple classes which have similar structures and behaviors.
- You want to encapsulate common logic and allow for variability in specific steps.
Now, let's get into how to implement the Template Design Pattern in Java.
Example Implementation
Step 1: Define the Template Class
Let's say you're building a series of classes for different types of beverages. They all share a preparation process but differ in their specifics. We can create an abstract class that defines the template.
abstract class Beverage {
// Template method
public final void prepareRecipe() {
boilWater();
brew();
pourInCup();
addCondiments();
}
protected abstract void brew();
protected abstract void addCondiments();
private void boilWater() {
System.out.println("Boiling water");
}
private void pourInCup() {
System.out.println("Pouring beverage into cup");
}
}
Commentary:
In the Beverage
class:
- The
prepareRecipe
method is a final method — no subclasses can override it. This enforces the exact sequence of steps. brew
andaddCondiments
are left abstract for subclasses to implement. This flexibility allows for specific beverage details without changing the overall flow.
Step 2: Create Concrete Subclasses
Now, let’s create two subclasses: Tea
and Coffee
.
class Tea extends Beverage {
@Override
protected void brew() {
System.out.println("Steeping the tea");
}
@Override
protected void addCondiments() {
System.out.println("Adding lemon");
}
}
class Coffee extends Beverage {
@Override
protected void brew() {
System.out.println("Dripping coffee through filter");
}
@Override
protected void addCondiments() {
System.out.println("Adding sugar and milk");
}
}
Commentary:
In these subclasses:
- Both
Tea
andCoffee
implement thebrew
andaddCondiments
methods. While they follow the same preparation template, the output varies based on the specific beverage type.
Step 3: Running the Code
Let's run a small test to see our pattern in action.
public class TemplateMethodTest {
public static void main(String[] args) {
Beverage tea = new Tea();
Beverage coffee = new Coffee();
System.out.println("Making tea...");
tea.prepareRecipe();
System.out.println("\nMaking coffee...");
coffee.prepareRecipe();
}
}
Expected Output:
Making tea...
Boiling water
Steeping the tea
Pouring beverage into cup
Adding lemon
Making coffee...
Boiling water
Dripping coffee through filter
Pouring beverage into cup
Adding sugar and milk
Commentary:
This output demonstrates that although both beverages follow the same preparation template, differing steps (like brewing and adding condiments) allow for unique processes.
Real-World Use Cases
Enhancing our understanding requires looking into real-world applications of the Template Design Pattern. Here are some scenarios:
- Document Generation: In report generation systems, the overall document structure is typically consistent, but the content differs per report type.
- Game Development: In games, different character behaviors can follow a general sequence while allowing specific actions (like attacking or defending) to vary.
- Data Processing Pipelines: Many data processing operations share the same preparation steps but differ in data handling specifics.
Lessons Learned
The Template Design Pattern is a powerful tool in our software engineering toolkit. By providing a consistent and clear structure, this pattern not only simplifies the code but also leads to applications that are more maintainable and reusable.
In summary, when tackling complex systems or codebases, consider the Template Design Pattern as a way to impose order, leverage shared behavior, and make your code more manageable.
Further Reading
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al.
- Refactoring Guru: Template Method Pattern
By adopting design patterns, we can not only overcome complexity but also elevate our skills as developers. Happy coding!