Unlocking Alternatives: When JPA/Hibernate Falls Short
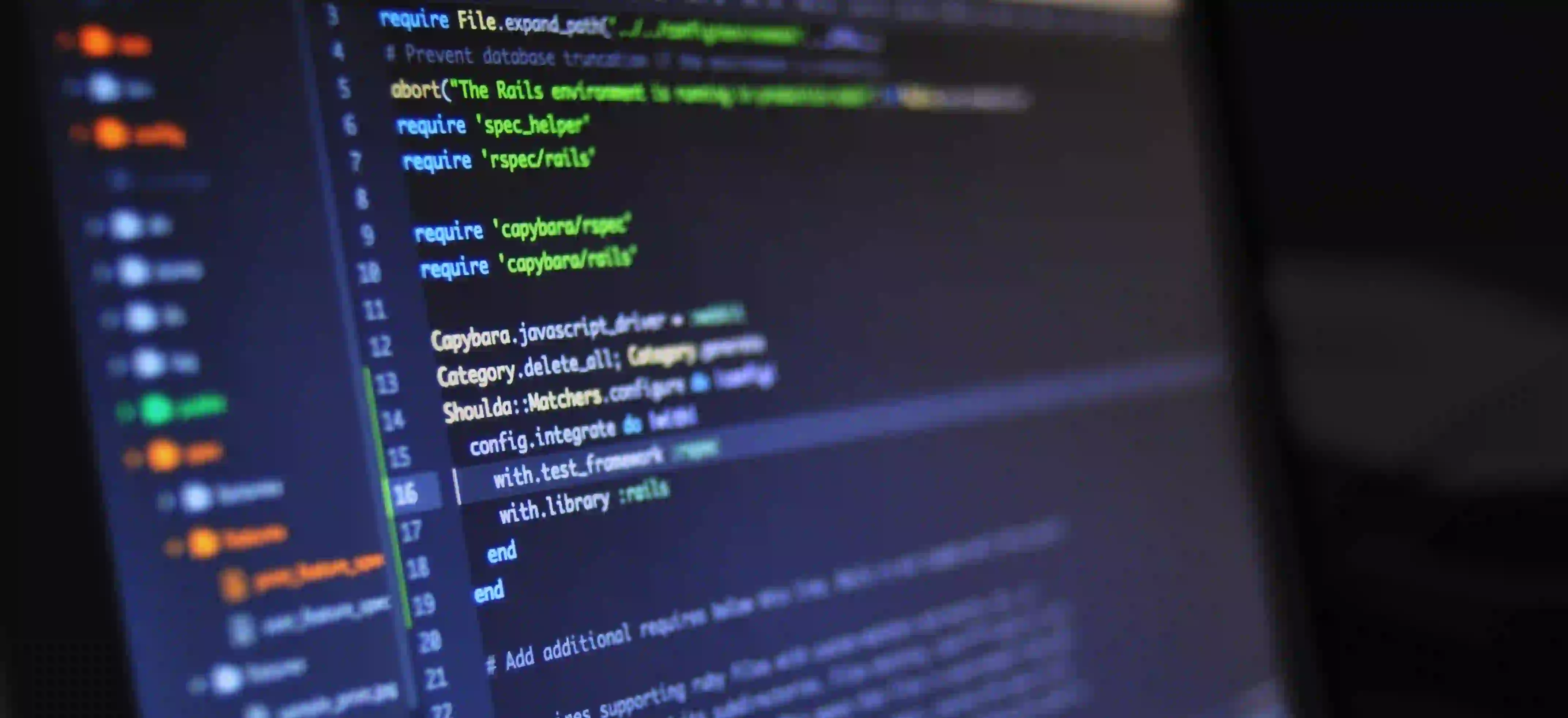
Unlocking Alternatives: When JPA/Hibernate Falls Short
Java Persistence API (JPA) and Hibernate are powerful tools for managing database operations in Java applications. They provide a simple interface to interact with databases, leveraging object-relational mapping (ORM) techniques. However, there are scenarios where JPA/Hibernate may not fulfill the requirements of your project. In this blog post, we will explore such scenarios, delve into the limitations of JPA and Hibernate, and discuss effective alternatives.
Understanding JPA and Hibernate
Before we address the limitations, it is essential to understand what JPA and Hibernate bring to the table. JPA is a Java specification for accessing, persisting, and managing data between Java objects and relational databases. Hibernate, on the other hand, is one of the most popular implementations of JPA, providing additional features beyond the standard specification.
Benefits of JPA/Hibernate
- Simplified Database Operations: JPA/Hibernate abstracts the complexities of database interactions, allowing developers to focus on the application logic.
- Data Caching: Hibernate provides a caching mechanism that significantly improves performance by reducing the number of database hits.
- Support for Complex Queries: JPA offers the Criteria API and JPQL (Java Persistence Query Language) for building complex queries in a type-safe manner.
With these benefits aside, let’s analyze the limitations that can compel developers to seek alternative solutions.
Limitations of JPA/Hibernate
1. Performance Overhead
Reason: JPA and Hibernate introduce an additional layer of abstraction. This can lead to slower performance compared to raw JDBC.
Example: For high-performance applications that require optimized database interactions, the overhead of ORM can become a bottleneck.
// Basic JDBC Example
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users WHERE id = ?")) {
statement.setInt(1, userId);
ResultSet resultSet = statement.executeQuery();
// Process result...
} catch (SQLException e) {
e.printStackTrace();
}
In this raw JDBC example, we achieve performance by directly working with the database, without the added overhead of ORM frameworks.
2. Complex Queries and Specifications
Reason: While JPQL is powerful, it can become cumbersome for very complex SQL queries and may not support every database feature without custom native queries.
Example: Try implementing a subquery in JPA, and you might find it challenging.
3. Lack of Flexibility
Reason: ORM frameworks impose certain restrictions on how you structure your domain model, which might not suit all applications.
Example: For applications with complex database designs that do not naturally map to object models, ORM can become an awkward fit.
4. Impedance Mismatch
Reason: There will always be a conceptual difference between relational databases and object-oriented programming. This gap can cause complications when fitting one into the other.
5. Learning Curve
Reason: Although JPA and Hibernate simplify a lot of tasks, they also introduce their own set of concepts and configurations that can be overwhelming, especially for newcomers.
Alternatives to JPA/Hibernate
1. JDBC (Java Database Connectivity)
When it comes to fine-grained control over SQL execution, JDBC is a solid alternative. It's a standard API for Java that allows you to interact directly with the database without the overhead of an ORM framework.
Advantages of JDBC
- Performance: Directly connects to the database, typically offering better performance in high-load scenarios.
- Control: You have complete control over SQL, leading to more optimized queries.
2. MyBatis
MyBatis is another popular alternative, providing a semi-ORM approach. Unlike full ORM frameworks, MyBatis allows you to write your raw SQL queries while still mapping the results to Java objects.
Advantages of MyBatis
- Flexibility: You control your SQL queries entirely and can easily leverage database-specific features.
- Less Complexity: Since it does not abstract the database layer too much, the learning curve is easier for those familiar with SQL.
Code Snippet Example using MyBatis
Here’s a simple MyBatis example demonstrating how to use it to fetch user information.
<!-- UserMapper.xml -->
<mapper namespace="com.example.mapper.UserMapper">
<select id="getUserById" parameterType="int" resultType="User">
SELECT * FROM users WHERE id = #{id}
</select>
</mapper>
// UserMapper.java
public interface UserMapper {
User getUserById(int id);
}
// MyBatis Configuration
SqlSession session = sqlSessionFactory.openSession();
UserMapper mapper = session.getMapper(UserMapper.class);
User user = mapper.getUserById(1);
session.close();
3. Spring Data JDBC
Spring Data JDBC is a Spring-based alternative designed to keep things simple while providing a similar ease of use as JPA. It's more straightforward and does not include all the complexities associated with ORM.
Advantages of Spring Data JDBC
- Simplicity: It adheres closely to JDBC principles while providing a repository and template pattern.
- Less Configuration: Requires less configuration than JPA/Hibernate, making it easier to set up.
4. Raw SQL with Java
For those who understand SQL and want precise control over data access, executing raw SQL queries using a Connection
can be advantageous. It’s suitable for small-scale applications where the overhead of ORM is unnecessary.
// Executing raw SQL
String query = "SELECT * FROM users WHERE age > ?";
try (Connection conn = DriverManager.getConnection(url);
PreparedStatement stmt = conn.prepareStatement(query)) {
stmt.setInt(1, 18);
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
System.out.println("User: " + rs.getString("name"));
}
}
The Last Word
While JPA and Hibernate have revolutionized data persistence in Java applications, there are certainly scenarios where they may not be the most effective fit. Performance concerns, complex queries, flexibility issues, and other limitations can necessitate alternative approaches. JDBC, MyBatis, Spring Data JDBC, or even raw SQL can provide more efficient, straightforward, and adaptable solutions based on your application's specific requirements.
By exploring these alternatives, you can choose the best persistence technology that aligns with your project goals and ensures optimal performance. Always weigh the intricacies of your application needs against the robust capabilities of JPA/Hibernate to determine the right tool for the job.
For further reading on alternatives to JPA/Hibernate, check out:
Ultimately, the choice of technology should enhance the development experience, maintainability, and performance of your application. Happy coding!